Unlocking the Potential of Python: A Comprehensive Guide to Python Programming {Whitepaper}

Table of contents
- 1. Introduction
- 2. Getting Started with Python
- 3. Pythonic Programming and Best Practices
- 4. Object-Oriented Programming (OOP) in Python
- 5. Working with Python Libraries and Frameworks
- 6. Python for Data Science and Visualization
- 7. Asynchronous Programming and Concurrency
- 8. Testing and Debugging in Python
- 9. Python Security and Best Practices
- 10. Deploying Python Applications
- 11. The Future of Python
- 12. Conclusion
- 13. Bibliography
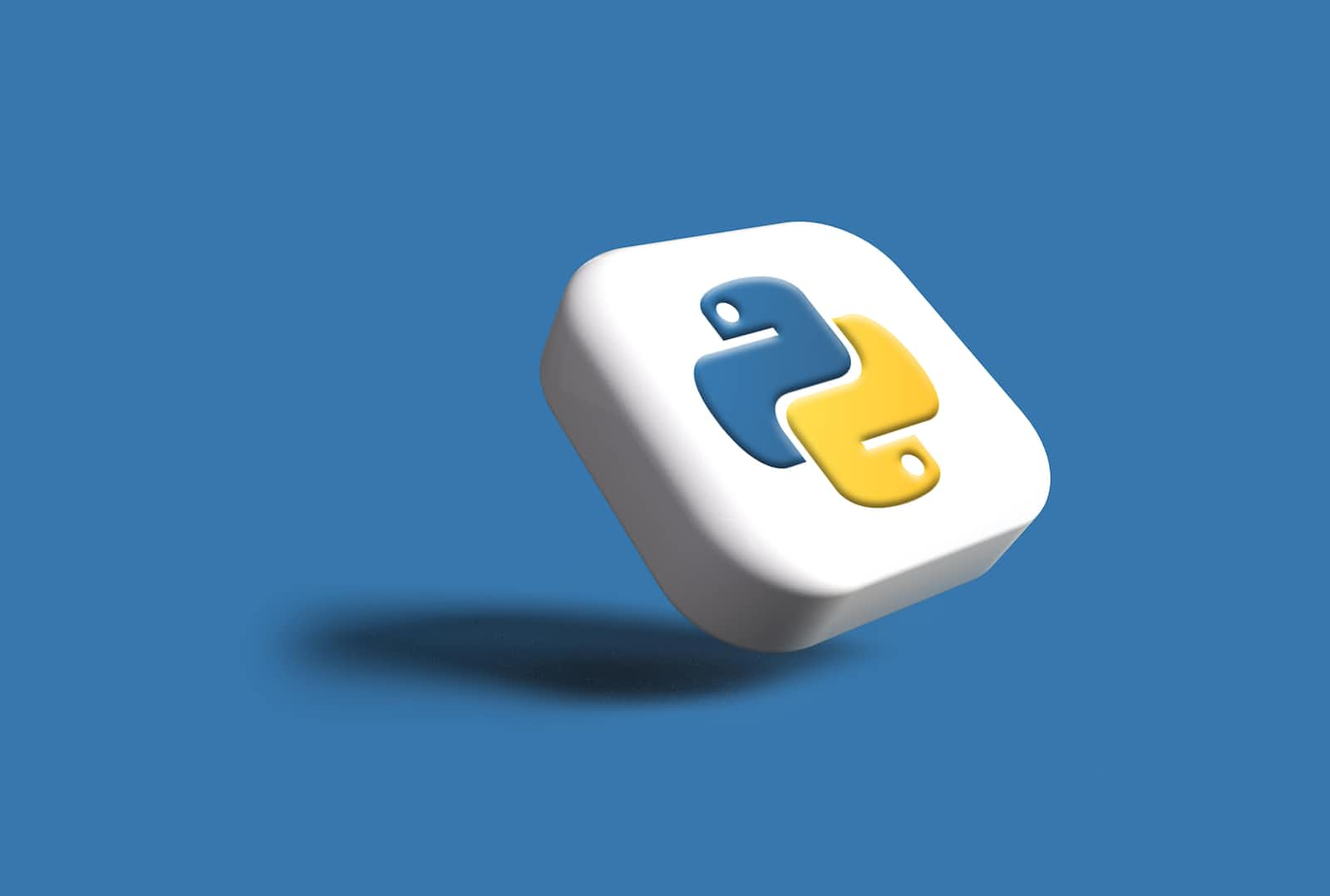
Abstract:
Python has emerged as one of the most popular and versatile programming languages in the software development landscape. This white paper aims to provide a comprehensive guide to Python programming, covering fundamental concepts, best practices, and advanced techniques. By exploring the diverse applications of Python and its rich ecosystem, this white paper equips developers with the knowledge and skills needed to leverage Python's power and unlock its full potential.
Table of Contents:
1. Introduction
1.1 The Rise of Python in the Programming World
1.2 Scope and Objectives
2. Getting Started with Python
2.1 Installing Python and Setting Up the Development Environment
2.2 Understanding Python's Syntax and Basic Data Types
2.3 Control Flow and Looping Constructs
2.4 Working with Functions and Modules
3. Pythonic Programming and Best Practices
3.1 Writing Clean and Readable Code
3.2 Using Built-in Data Structures and Libraries
3.3 Handling Exceptions and Error Handling
3.4 Adopting Python Coding Conventions and Style Guidelines
4. Object-Oriented Programming (OOP) in Python
4.1 Understanding the Principles of OOP
4.2 Creating Classes and Objects
4.3 Inheritance and Polymorphism in Python
4.4 Encapsulation and Abstraction
5. Working with Python Libraries and Frameworks
5.1 Exploring the Python Standard Library
5.2 Introduction to Third-Party Libraries and Package Management
5.3 Web Development with Flask and Django
5.4 Data Analysis and Scientific Computing with NumPy and Pandas
5.5 Machine Learning with scikit-learn and TensorFlow
6. Python for Data Science and Visualization
6.1 Data Manipulation and Cleaning with Pandas
6.2 Exploratory Data Analysis (EDA) with Matplotlib and Seaborn
6.3 Statistical Analysis with SciPy and StatsModels
6.4 Machine Learning Pipelines with scikit-learn
7. Asynchronous Programming and Concurrency
7.1 Understanding Asynchronous Programming Concepts
7.2 Working with asyncio and aiohttp
7.3 Parallel and Concurrent Programming Techniques
8. Testing and Debugging in Python
8.1 Writing Effective Unit Tests with unittest and pytest
8.2 Debugging Techniques and Tools
8.3 Code Profiling and Optimization
9. Python Security and Best Practices
9.1 Secure Coding Practices
9.2 Common Security Vulnerabilities and Mitigation Strategies
9.3 Securing Web Applications and APIs
10. Deploying Python Applications
10.1 Packaging and Distribution Options
10.2 Containerization with Docker
10.3 Deployment Strategies and Best Practices
11. The Future of Python
11.1 Exploring Python's Latest Features and Updates
11.2 Emerging Trends and Technologies in the Python Ecosystem
12. Conclusion
12.1 Key Takeaways
12.2 Embracing the Power of Python
This white paper aims to provide developers with a comprehensive guide to Python programming, covering essential concepts, best practices, and advanced techniques. By delving into various aspects of Python development, developers can harness the language's versatility and build robust and scalable applications. Please note that the white paper should be further expanded with detailed explanations, code examples, and relevant references to provide a comprehensive
1. Introduction
1.1 The Rise of Python in the Programming World
Python's Journey to Prominence
In the ever-evolving landscape of programming languages, Python has emerged as a shining star. Its journey to prominence has been nothing short of remarkable. Python, often referred to as a "glue" language, started its life in the late 1980s. Guido van Rossum, Python's creator, sought to create a language that prioritized simplicity and readability, making it accessible to both novice and experienced programmers.
Over the decades, Python's popularity soared for several key reasons:
Elegant and Readable Syntax: Python's syntax is clean, concise, and human-readable, making it an ideal choice for developers to express their ideas clearly and efficiently.
Versatility: Python is a versatile language that can be used for a wide range of applications, from web development and scientific computing to artificial intelligence and automation.
Thriving Ecosystem: Python boasts an extensive ecosystem of libraries, frameworks, and tools that streamline development and solve complex problems.
Community and Collaboration: Python's open-source nature fosters a vibrant and collaborative community. Developers worldwide contribute to its growth and development.
Education and Adoption: Python has found its way into classrooms and universities, becoming a preferred language for teaching programming and computer science.
In recent years, Python has become a driving force in various domains, including web development, data science, machine learning, and more. Its simplicity, versatility, and robustness have made it a go-to choice for startups, enterprises, and individual developers alike.
1.2 Scope and Objectives
Defining the Journey Ahead
The scope of this white paper is to provide a comprehensive guide to Python programming. From fundamental concepts to best practices and advanced techniques, this document aims to equip developers with the knowledge and skills necessary to harness Python's full potential.
Our objectives include:
Demystifying Python: We will unravel the elegance of Python's syntax and explore its basic data types, control flow, and modular structure.
Pythonic Practices: We will delve into Pythonic programming principles and best practices, emphasizing clean, readable code and adherence to coding conventions.
Object-Oriented Programming: A comprehensive exploration of Python's object-oriented programming capabilities, including inheritance, polymorphism, encapsulation, and abstraction.
Ecosystem Exploration: We will navigate the Python ecosystem, examining libraries, frameworks, and tools for web development, data analysis, machine learning, and more.
Data Science and Visualization: A deep dive into data manipulation, exploratory data analysis, statistical analysis, and machine learning with Python.
Asynchronous Programming and Concurrency: Understanding the concepts of asynchronous programming and concurrency and practical applications using asyncio.
Testing and Debugging: Strategies for effective testing, debugging techniques, and code profiling to optimize Python programs.
Security Best Practices: Guidance on secure coding practices, identifying vulnerabilities, and securing web applications and APIs developed in Python.
Deployment Strategies: Options for packaging, containerization with Docker, and deployment best practices for Python applications.
The Future of Python: A glimpse into Python's latest features, updates, emerging trends, and technologies in the Python ecosystem.
2. Getting Started with Python
Python is a versatile and widely-used programming language known for its simplicity and readability. In this guide, we will cover the basics of Python, including installation, syntax, data types, control flow, and functions.
2.1 Installing Python and Setting Up the Development Environment
Installation:
Python can be downloaded from the official website (python.org) and is available for various platforms (Windows, macOS, Linux).
Ensure that you download the latest version compatible with your operating system.
Development Environment:
Python can be run directly from the command line by typing
python
. You can exit by typingexit()
or pressing Ctrl+Z and Enter.Integrated Development Environments (IDEs) like PyCharm, Visual Studio Code, and Jupyter Notebook provide a more feature-rich environment for coding in Python.
Virtual environments (e.g., using
venv
orvirtualenv
) help manage dependencies and isolate projects.
2.2 Understanding Python's Syntax and Basic Data Types
Python Syntax:
Python uses indentation (whitespace) to define blocks of code, making it crucial for readability.
Statements are terminated with a newline character, and no semicolons are required.
Basic Data Types:
Integers (
int
): Whole numbers, e.g., 5, -10.Floating-point numbers (
float
): Numbers with decimals, e.g., 3.14, -0.5.Strings (
str
): Ordered sequences of characters, e.g., "Hello, Python!"Booleans (
bool
): Represents True or False.Lists (
list
): Ordered, mutable collections, e.g., [1, 2, 3].Tuples (
tuple
): Ordered, immutable collections, e.g., (1, 2, 3).Dictionaries (
dict
): Key-value pairs, e.g., {"name": "John", "age": 30}.
2.3 Control Flow and Looping Constructs
Conditional Statements:
if
,elif
,else
are used for decision-making.Example:
if condition: # Code to execute if condition is True elif another_condition: # Code to execute if another_condition is True else: # Code to execute if none of the conditions are True
Loops:
for
loop iterates over a sequence (e.g., list) or range.for item in sequence: # Code to execute for each item
while
loop continues until a condition becomes False.while condition: # Code to execute while condition is True
2.4 Working with Functions and Modules
Functions:
Functions are reusable blocks of code defined using the
def
keyword.Example:
def add_numbers(a, b): return a + b
Modules:
Modules are reusable Python files containing functions, variables, and classes.
Import modules using
import
statement.Example:
import math result = math.sqrt(25)
You can also create your own modules by saving Python code in a .py file and importing it in other scripts.
3. Pythonic Programming and Best Practices
Pythonic programming refers to writing code in a way that adheres to Python's style and conventions while also optimizing for readability, efficiency, and maintainability. Embracing Pythonic practices helps ensure your code is both elegant and efficient.
3.1 Writing Clean and Readable Code
Importance of Clean Code:
Clean code is easy to read, understand, and maintain.
It reduces the chances of bugs and improves collaboration with other developers.
Pythonic Practices for Clean Code:
Use descriptive variable and function names that convey their purpose.
Follow the PEP 8 style guide for naming conventions and code formatting.
Write docstrings to document functions and modules.
Keep functions and classes short and focused on a single task (the "Single Responsibility Principle").
Avoid deep nesting and excessive indentation.
Use whitespace and indentation consistently.
Comment code sparingly; focus on writing self-explanatory code instead.
Refactor and optimize code as needed for readability and performance.
3.2 Using Built-in Data Structures and Libraries
Leveraging Built-in Data Structures:
Python provides versatile data structures like lists, dictionaries, sets, and tuples.
Use the appropriate data structure for the task to optimize performance and readability.
Avoid reinventing the wheel by using built-in data structures for common tasks.
Working with Libraries:
Python has a vast standard library with modules for various purposes (e.g.,
math
,datetime
,os
,json
).Utilize third-party libraries from the Python Package Index (PyPI) to extend functionality.
Always ensure you install and import libraries properly to use their features.
3.3 Handling Exceptions and Error Handling
Exception Handling:
Use try-except blocks to handle exceptions and prevent program crashes.
Be specific about the exceptions you catch to avoid hiding unexpected errors.
Include meaningful error messages to aid in debugging.
Optionally, use a
finally
block to ensure certain code executes, regardless of exceptions.
Example of Exception Handling:
try:
# Code that may raise an exception
result = 10 / 0
except ZeroDivisionError as e:
# Handle the specific exception
print(f"Error: {e}")
except Exception as e:
# Handle other exceptions
print(f"An unexpected error occurred: {e}")
else:
# Code to execute if no exception is raised
finally:
# Cleanup code (always runs)
print("Cleanup")
3.4 Adopting Python Coding Conventions and Style Guidelines
PEP 8 Style Guide:
PEP 8 is the official Python style guide that provides coding conventions and recommendations.
It covers naming conventions, indentation, line length, and more.
Adhering to PEP 8 makes your code consistent and readable.
Tools for Style Checking:
Use tools like Flake8, Pylint, or Black to automate style checking and formatting.
These tools help ensure your code conforms to PEP 8 and maintain consistency.
Code Reviews:
Conduct code reviews with team members to maintain coding standards.
Code reviews help catch issues early and improve code quality.
4. Object-Oriented Programming (OOP) in Python
Object-Oriented Programming (OOP) is a programming paradigm that focuses on designing software using objects, which are instances of classes. In Python, OOP is a fundamental concept and is widely used for building complex and modular applications.
4.1 Understanding the Principles of OOP
Principles of OOP:
Classes: Classes are blueprints for creating objects. They define the structure and behavior of objects.
Objects: Objects are instances of classes and represent real-world entities with data (attributes) and actions (methods).
Encapsulation: Encapsulation restricts access to the internal state of an object and exposes a controlled interface.
Inheritance: Inheritance allows a class to inherit properties and methods from another class, promoting code reuse.
Polymorphism: Polymorphism enables objects of different classes to be treated as objects of a common superclass.
Abstraction: Abstraction simplifies complex systems by hiding unnecessary details and showing only essential features.
4.2 Creating Classes and Objects
Defining a Class:
Use the
class
keyword to define a class.The class may have attributes (variables) and methods (functions).
Creating Objects:
Objects are instances of a class.
You can create objects by calling the class constructor, usually
__init__
method.Example:
class Car: def __init__(self, make, model): self.make = make self.model = model car1 = Car("Toyota", "Camry")
Accessing Attributes and Methods:
Use dot notation to access object attributes and methods.
Example:
print(car1.make) # Accessing attribute car1.start() # Calling method
4.3 Inheritance and Polymorphism in Python
Inheritance:
Inheritance allows you to create a new class (subclass/derived class) that inherits properties and methods from an existing class (base class/parent class).
Example:
class ElectricCar(Car): # ElectricCar inherits from Car def __init__(self, make, model, battery_size): super().__init__(make, model) # Call the parent class constructor self.battery_size = battery_size electric_car = ElectricCar("Tesla", "Model 3", 75)
Polymorphism:
Polymorphism allows different objects to respond to the same method or attribute in a way that is specific to their class.
Example:
def get_vehicle_info(vehicle): print(f"Make: {vehicle.make}, Model: {vehicle.model}") get_vehicle_info(car1) # Prints Car information get_vehicle_info(electric_car) # Prints ElectricCar information
4.4 Encapsulation and Abstraction
Encapsulation:
Encapsulation restricts access to certain attributes and methods to prevent unauthorized modifications.
Use private attributes (names starting with
_
) to indicate that they should not be accessed directly.Example:
class Student: def __init__(self, name, age): self._name = name self._age = age def get_name(self): return self._name def set_age(self, age): if age >= 0: self._age = age student1 = Student("Alice", 25)
Abstraction:
Abstraction hides complex implementation details and exposes a simplified interface.
Abstraction allows you to focus on essential features while ignoring unnecessary complexity.
Example:
class Shape: def area(self): pass # Abstract method class Circle(Shape): def __init__(self, radius): self.radius = radius def area(self): return 3.14 * self.radius * self.radius
Object-oriented programming in Python enables you to design modular and organized code by creating classes and objects, using inheritance and polymorphism for code reuse and flexibility, and encapsulating data to control access. Abstraction helps simplify complex systems. These principles are fundamental for building scalable and maintainable software in Python.
5. Working with Python Libraries and Frameworks
Python offers a rich ecosystem of libraries and frameworks that extend its capabilities for various domains and tasks. Understanding how to work with these libraries is crucial for building robust applications.
5.1 Exploring the Python Standard Library
Python Standard Library:
The Python Standard Library is a collection of modules and packages that provide a wide range of functionality.
It includes modules for file I/O, regular expressions, networking, data serialization (JSON, XML), and more.
Example: Reading and Writing Files
# Reading a file
with open("example.txt", "r") as file:
content = file.read()
# Writing to a file
with open("output.txt", "w") as file:
file.write("Hello, Python!")
5.2 Introduction to Third-Party Libraries and Package Management
Third-Party Libraries:
Third-party libraries are developed by the Python community and provide specialized functionality.
These libraries extend Python's capabilities in areas like web development, data analysis, machine learning, etc.
Package Management with pip:
pip
is the package manager for Python.It allows you to install and manage third-party libraries easily.
Example:
Install a package:
pip install package_name
List installed packages:
pip list
Create a
requirements.txt
file to specify package dependencies for your project.
5.3 Web Development with Flask and Django
Flask:
Flask is a lightweight web framework for building web applications in Python.
It provides essential tools for routing, handling requests, and rendering templates.
Django:
Django is a high-level web framework that includes many built-in features like authentication, ORM (Object-Relational Mapping), and an admin interface.
It follows the "batteries-included" philosophy, making it suitable for large-scale applications.
Example with Flask:
from flask import Flask
app = Flask(__name__)
@app.route("/")
def hello_world():
return "Hello, Flask!"
if __name__ == "__main__":
app.run()
5.4 Data Analysis and Scientific Computing with NumPy and Pandas
NumPy:
NumPy is a library for numerical and scientific computing in Python.
It provides support for large, multi-dimensional arrays and matrices, along with mathematical functions.
Pandas:
Pandas is a data manipulation library built on top of NumPy.
It provides data structures like DataFrames and Series, making it easier to work with structured data.
Example with Pandas:
import pandas as pd
data = {
'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35]
}
df = pd.DataFrame(data)
print(df)
5.5 Machine Learning with scikit-learn and TensorFlow
scikit-learn:
scikit-learn is a popular machine learning library for Python.
It provides tools for classification, regression, clustering, dimensionality reduction, and more.
TensorFlow:
TensorFlow is an open-source machine learning framework developed by Google.
It is widely used for building deep learning models and neural networks.
Example with scikit-learn:
from sklearn import datasets
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
from sklearn.metrics import accuracy_score
# Load the iris dataset
iris = datasets.load_iris()
X, y = iris.data, iris.target
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2)
# Train a logistic regression model
model = LogisticRegression()
model.fit(X_train, y_train)
# Make predictions
predictions = model.predict(X_test)
# Calculate accuracy
accuracy = accuracy_score(y_test, predictions)
print(f"Accuracy: {accuracy}")
Working with Python libraries and frameworks allows developers to leverage existing tools and solutions to solve complex problems efficiently. Whether you're developing web applications, performing data analysis, or building machine learning models, Python's ecosystem offers a wide array of resources to support your work.
6. Python for Data Science and Visualization
Python is a powerful tool for data science and visualization, with libraries like Pandas, Matplotlib, Seaborn, SciPy, StatsModels, and scikit-learn providing essential functionality for data manipulation, analysis, and machine learning.
6.1 Data Manipulation and Cleaning with Pandas
Pandas for Data Manipulation:
Pandas is a popular library for data manipulation and analysis.
It provides two key data structures: DataFrames (2D tables) and Series (1D arrays).
Data Cleaning with Pandas:
Pandas offers functions to handle missing data, duplicate records, and outliers.
You can filter, transform, and aggregate data with ease.
Example: Handling Missing Data
import pandas as pd
data = {'A': [1, 2, None, 4],
'B': [5, None, 7, 8]}
df = pd.DataFrame(data)
# Drop rows with missing values
df.dropna(inplace=True)
# Fill missing values with a specific value
df['A'].fillna(0, inplace=True)
6.2 Exploratory Data Analysis (EDA) with Matplotlib and Seaborn
Matplotlib for Plotting:
Matplotlib is a widely-used library for creating static, animated, or interactive visualizations.
It offers fine-grained control over plot customization.
Seaborn for Statistical Visualization:
Seaborn is built on top of Matplotlib and simplifies the creation of aesthetically pleasing statistical plots.
It's especially useful for EDA.
Example: Creating a Scatter Plot with Seaborn
import seaborn as sns
import matplotlib.pyplot as plt
iris = sns.load_dataset("iris")
sns.scatterplot(data=iris, x="sepal_length", y="sepal_width", hue="species")
plt.title("Sepal Length vs. Sepal Width")
plt.show()
6.3 Statistical Analysis with SciPy and StatsModels
SciPy for Scientific Computing:
SciPy is built on top of NumPy and provides additional functionality for scientific computing.
It includes statistical tests, optimization, interpolation, and more.
StatsModels for Statistical Analysis:
StatsModels is a library dedicated to statistical analysis and hypothesis testing.
It is particularly useful for regression analysis and time series analysis.
Example: Linear Regression with StatsModels
import statsmodels.api as sm
import pandas as pd
data = pd.read_csv("data.csv")
X = data[['X1', 'X2']]
y = data['Y']
X = sm.add_constant(X) # Add a constant for the intercept
model = sm.OLS(y, X).fit()
print(model.summary())
6.4 Machine Learning Pipelines with scikit-learn
scikit-learn for Machine Learning:
scikit-learn is a powerful library for machine learning in Python.
It offers a wide range of algorithms for classification, regression, clustering, and more.
Building a Machine Learning Pipeline:
A machine learning pipeline includes data preprocessing, feature selection, model training, and evaluation.
scikit-learn provides tools for creating robust pipelines.
Example: Creating a Classification Pipeline
from sklearn.pipeline import Pipeline
from sklearn.preprocessing import StandardScaler
from sklearn.decomposition import PCA
from sklearn.svm import SVC
# Define the steps in the pipeline
steps = [('scaler', StandardScaler()),
('pca', PCA(n_components=2)),
('classifier', SVC(kernel='linear'))]
# Create the pipeline
pipeline = Pipeline(steps)
# Fit the pipeline to the data
pipeline.fit(X_train, y_train)
# Make predictions
y_pred = pipeline.predict(X_test)
# Evaluate the model
accuracy = pipeline.score(X_test, y_test)
Python's libraries and tools for data manipulation, visualization, statistical analysis, and machine learning make it a versatile choice for data science projects. Whether you need to clean and explore data, create visualizations, perform statistical analysis, or build machine learning models, Python's ecosystem has you covered.
7. Asynchronous Programming and Concurrency
Asynchronous programming and concurrency are essential concepts in modern software development, allowing applications to perform multiple tasks concurrently without blocking the main execution thread. Python provides tools and libraries to work with asynchronous programming effectively.
7.1 Understanding Asynchronous Programming Concepts
Synchronous vs. Asynchronous:
Synchronous code executes one task at a time, blocking until each task is completed.
Asynchronous code allows tasks to execute independently, freeing up the main thread for other work.
Concurrency vs. Parallelism:
Concurrency involves managing multiple tasks, potentially interleaving their execution.
Parallelism involves running multiple tasks simultaneously on multiple processors or cores.
Benefits of Asynchronous Programming:
Improved responsiveness in applications.
Efficient use of system resources.
Enhanced I/O-bound task performance.
7.2 Working with asyncio and aiohttp
asyncio:
asyncio is a Python library for writing asynchronous code.
It provides an event loop that manages asynchronous tasks (coroutines).
Coroutines are functions that can be paused and resumed, allowing non-blocking execution.
Example of asyncio:
import asyncio
async def main():
print("Hello")
await asyncio.sleep(1)
print("World")
asyncio.run(main())
aiohttp:
aiohttp is an asynchronous HTTP client/server framework.
It enables building high-performance, non-blocking web applications and services.
Example of aiohttp:
import aiohttp
import asyncio
async def fetch_url(url):
async with aiohttp.ClientSession() as session:
async with session.get(url) as response:
return await response.text()
async def main():
url = "https://example.com"
content = await fetch_url(url)
print(content)
asyncio.run(main())
7.3 Parallel and Concurrent Programming Techniques
Parallelism with multiprocessing:
Python's
multiprocessing
module allows running code in parallel processes.It is suitable for CPU-bound tasks that benefit from multiple CPU cores.
Example of multiprocessing:
from multiprocessing import Pool
def square(x):
return x * x
if __name__ == '__main__':
numbers = [1, 2, 3, 4, 5]
with Pool() as pool:
results = pool.map(square, numbers)
print(results)
Concurrency with threading:
Python's
threading
module provides a way to run tasks concurrently using threads.It is useful for I/O-bound tasks but may face Global Interpreter Lock (GIL) limitations for CPU-bound tasks.
Example of threading:
import threading
def print_numbers():
for i in range(1, 6):
print(f"Number {i}")
def print_letters():
for letter in 'abcde':
print(f"Letter {letter}")
thread1 = threading.Thread(target=print_numbers)
thread2 = threading.Thread(target=print_letters)
thread1.start()
thread2.start()
thread1.join()
thread2.join()
Understanding asynchronous programming and concurrency is crucial for optimizing performance in applications that handle multiple tasks simultaneously. Python provides asyncio for asynchronous programming and libraries like aiohttp for non-blocking I/O. Additionally, the multiprocessing
and threading
modules offer options for parallel and concurrent programming, respectively. Choosing the right approach depends on the specific requirements of your application.
8. Testing and Debugging in Python
Testing and debugging are critical aspects of software development. Python offers various tools and libraries to help developers write robust code and identify and fix issues effectively.
8.1 Writing Effective Unit Tests with unittest and pytest
Unit Testing:
Unit testing is the practice of testing individual components or units of code in isolation.
Python provides two popular testing libraries:
unittest
andpytest
.
unittest:
unittest
is Python's built-in testing framework.It is inspired by Java's JUnit and follows the xUnit style of testing.
Example using unittest:
import unittest
def add(a, b):
return a + b
class TestAddition(unittest.TestCase):
def test_add_positive_numbers(self):
result = add(2, 3)
self.assertEqual(result, 5)
def test_add_negative_numbers(self):
result = add(-2, -3)
self.assertEqual(result, -5)
if __name__ == '__main__':
unittest.main()
pytest:
pytest
is a third-party testing framework that simplifies test writing and offers advanced features.It automatically discovers and runs test functions.
Example using pytest:
def add(a, b):
return a + b
def test_add_positive_numbers():
result = add(2, 3)
assert result == 5
def test_add_negative_numbers():
result = add(-2, -3)
assert result == -5
8.2 Debugging Techniques and Tools
Debugging Process:
Debugging involves identifying and fixing errors or bugs in the code.
Common debugging techniques include using print statements and debugging tools.
Built-in Debugger (pdb
):
Python comes with a built-in debugger called
pdb
(Python Debugger).You can insert breakpoints in your code and inspect variables and control flow.
Example using pdb
:
import pdb
def divide(a, b):
result = a / b
return result
pdb.set_trace()
result = divide(10, 2)
print(result)
Debugging Tools:
Integrated Development Environments (IDEs) like PyCharm, Visual Studio Code, and Jupyter Notebook provide debugging features.
These tools offer visual debugging, breakpoints, variable inspection, and more.
8.3 Code Profiling and Optimization
Code Profiling:
Code profiling helps identify performance bottlenecks in your code.
Python offers the
cProfile
module for profiling.
Example using cProfile
:
import cProfile
def slow_function():
result = 0
for i in range(10_000_000):
result += i
return result
if __name__ == '__main__':
cProfile.run('slow_function()')
Optimization Techniques:
Once performance issues are identified, optimization techniques can be applied.
Strategies include algorithmic improvements, using built-in functions, and leveraging libraries like NumPy for numeric operations.
Example of Optimization:
# Non-optimized code
result = 0
for i in range(10_000_000):
result += i
# Optimized code using sum()
result = sum(range(10_000_000))
Testing and debugging are integral parts of the software development process. Effective unit testing with libraries like unittest
and pytest
helps ensure code correctness. Debugging involves identifying and fixing errors using techniques like print statements and debugging tools like pdb
. Code profiling with cProfile
can reveal performance bottlenecks, which can be addressed through optimization techniques to improve code efficiency.
9. Python Security and Best Practices
Python security is crucial to protect your applications and data from various threats. This includes writing secure code, identifying and mitigating vulnerabilities, and securing web applications and APIs.
9.1 Secure Coding Practices
Secure Coding Principles:
Input Validation: Always validate and sanitize user inputs to prevent SQL injection, Cross-Site Scripting (XSS), and other injection attacks.
Authentication and Authorization: Implement proper authentication mechanisms, such as strong password hashing and multi-factor authentication. Authorize users to access only what they need.
Session Management: Use secure session management techniques to protect user sessions and tokens.
Error Handling: Avoid displaying detailed error messages to users. Log errors securely.
Encryption: Use encryption (e.g., TLS/SSL) for data transmission and storage.
Secure Password Handling: Store passwords securely using salted and hashed algorithms like bcrypt.
File Uploads: Implement strict controls on file uploads to prevent arbitrary file execution and denial-of-service attacks.
9.2 Common Security Vulnerabilities and Mitigation Strategies
Common Vulnerabilities:
SQL Injection: Attackers can manipulate SQL queries to access or modify data. Prevent this by using parameterized queries or ORM frameworks like SQLAlchemy.
Cross-Site Scripting (XSS): Malicious scripts are injected into web pages viewed by other users. Sanitize user inputs and escape output to prevent XSS attacks.
Cross-Site Request Forgery (CSRF): Attackers trick users into performing actions without their consent. Implement anti-CSRF tokens and use the SameSite attribute for cookies.
Security Misconfigurations: Insecure default settings or poorly configured security settings. Regularly review configurations and use security tools like static code analyzers.
Insecure Dependencies: Using outdated or vulnerable libraries. Keep dependencies up-to-date and use tools like
pip
to check for vulnerabilities.Sensitive Data Exposure: Inadequate protection of sensitive data like passwords or credit card numbers. Use encryption, protect data at rest and in transit, and follow data protection regulations.
9.3 Securing Web Applications and APIs
Securing Web Applications:
Use web application firewalls (WAFs) and intrusion detection systems (IDS) to protect against attacks.
Implement proper access controls and session management.
Regularly update and patch web frameworks, libraries, and server software.
Validate and sanitize inputs and implement content security policies (CSP).
Securing APIs:
Use authentication mechanisms like OAuth2 or API keys.
Implement rate limiting and throttling to prevent abuse.
Validate and sanitize input data to prevent injection attacks.
Implement secure transport (TLS/SSL) for API communications.
Use API security tools for continuous monitoring and protection.
Security Testing:
Conduct regular security testing, including penetration testing and code reviews.
Use security testing tools like OWASP ZAP and Nessus.
Consider threat modeling to identify potential vulnerabilities in the design phase.
Security is an ongoing process, and it's essential to stay informed about the latest threats and mitigation strategies. Following secure coding practices, understanding common vulnerabilities, and taking steps to secure web applications and APIs are vital to safeguarding your Python applications and data. Regular audits and testing should be part of your security strategy to ensure ongoing protection.
10. Deploying Python Applications
Deploying Python applications involves packaging and distributing your code, containerization using Docker, and implementing deployment strategies and best practices to ensure your application runs reliably in production environments.
10.1 Packaging and Distribution Options
Packaging Python Code:
Python code is typically packaged into distributable units using tools like
setuptools
andpip
.Create a
setup.py
script to specify project metadata and dependencies.
Creating Python Packages:
Packages are directory structures containing modules and an
__init__.py
file.Use the
__init__.py
file to make a directory a Python package.
Distribution Options:
Distribute your Python code as source packages (
.tar.gz
) or binary packages (.whl
).Publish packages to the Python Package Index (PyPI) for easy distribution.
Use virtual environments to isolate dependencies.
10.2 Containerization with Docker
Docker Containers:
Docker is a containerization platform for packaging applications and their dependencies.
Containers provide consistent and isolated runtime environments.
Dockerfile:
Create a
Dockerfile
to define the steps to build a Docker image.Specify a base image, copy code, install dependencies, and configure the runtime environment.
Docker Compose:
Use Docker Compose to manage multi-container applications.
Define services, networks, and volumes in a
docker-compose.yml
file.
Example Dockerfile:
# Use an official Python runtime as a parent image
FROM python:3.7-slim
# Set the working directory to /app
WORKDIR /app
# Copy the current directory contents into the container at /app
COPY . /app
# Install any needed packages specified in requirements.txt
RUN pip install -r requirements.txt
# Make port 80 available to the world outside this container
EXPOSE 80
# Define environment variable
ENV NAME World
# Run app.py when the container launches
CMD ["python", "app.py"]
10.3 Deployment Strategies and Best Practices
Deployment Strategies:
Choose deployment strategies based on your application's requirements:
Blue-Green Deployment: Create a parallel environment and switch traffic when ready.
Canary Deployment: Gradually release new features to a subset of users.
Rolling Deployment: Update one instance at a time to minimize downtime.
Continuous Deployment: Automatically deploy changes when tests pass.
Deployment Automation:
Implement continuous integration and continuous deployment (CI/CD) pipelines.
Use CI/CD tools like Jenkins, Travis CI, or GitLab CI/CD for automated testing and deployment.
Monitoring and Logging:
Set up monitoring tools like Prometheus and Grafana to track application performance.
Use centralized logging solutions like ELK (Elasticsearch, Logstash, Kibana) for log aggregation and analysis.
Scalability:
Design your application to scale horizontally using load balancers and auto-scaling groups.
Use cloud services like AWS Elastic Beanstalk or Kubernetes for scalable deployments.
Security:
Keep your container images and application dependencies up to date to patch security vulnerabilities.
Implement security best practices like least privilege and network segmentation.
Deploying Python applications involves careful planning, including packaging and distribution, containerization with Docker, and selecting appropriate deployment strategies and best practices. Automating deployment processes, monitoring, and securing your application are essential steps to ensure smooth operation in production environments.
11. The Future of Python
Python is a dynamic programming language that continues to evolve with new features and updates. It also remains relevant and adaptable to emerging trends and technologies in the software development ecosystem.
11.1 Exploring Python's Latest Features and Updates
Python Enhancement Proposals (PEPs):
Python's development process is driven by PEPs, which propose new features, improvements, and updates.
PEPs are discussed and reviewed by the Python community, and some are accepted for implementation.
Latest Python Versions:
As of my last knowledge update in September 2021, Python 3.10 was the latest stable release.
Python 3.11 and subsequent versions have likely been released with new features and improvements.
Notable Features in Recent Python Versions (Python 3.10):
Pattern matching: Enhanced syntax for working with structured data.
Parenthesized Context Managers: Simplified management of multiple context managers.
Improved error messages and diagnostics.
Performance optimizations.
Upcoming Python Features:
- Python development roadmaps may include planned features like static typing enhancements, improved concurrency support, and more.
11.2 Emerging Trends and Technologies in the Python Ecosystem
Data Science and Machine Learning:
Python remains a dominant language for data science and machine learning with libraries like NumPy, Pandas, scikit-learn, and TensorFlow.
Emerging trends include AI ethics, responsible AI, and explainable AI.
Web Development:
Python frameworks like Django and Flask continue to be popular for web development.
Microservices architecture, serverless computing, and asynchronous web frameworks are emerging trends.
DevOps and Cloud Computing:
Python is used extensively in DevOps for automation and infrastructure as code (IaC) using tools like Ansible and Terraform.
Cloud-native development with Python is on the rise, leveraging cloud providers like AWS, Azure, and GCP.
Cybersecurity:
Python is used in cybersecurity for penetration testing, security analysis, and building security tools.
Security automation and threat detection are growing areas.
Quantum Computing:
Python libraries like Qiskit and Cirq are enabling quantum computing research and development.
Quantum computing is an emerging field with significant potential.
WebAssembly:
Python can be compiled to WebAssembly (Wasm), making it possible to run Python code in web browsers.
This allows Python to play a role in web applications and edge computing.
Blockchain and Cryptocurrency:
Python is used in blockchain development for creating smart contracts and decentralized applications (DApps).
Cryptocurrency projects often use Python for scripting and automation.
Python's adaptability, rich ecosystem, and active community contribute to its continued relevance and growth. As Python evolves with new features and updates, it remains a versatile language that can be applied to a wide range of domains, from web development and data science to emerging technologies like quantum computing and blockchain. Keeping up with Python's latest developments and staying informed about emerging trends is essential for Python developers.
12. Conclusion
Python is a versatile and powerful programming language with a rich ecosystem of libraries and frameworks. In this comprehensive guide, we've covered various aspects of Python, from its fundamentals to advanced topics, including data science, web development, and security. Here are some key takeaways:
12.1 Key Takeaways
Python's Versatility: Python can be used for a wide range of applications, including web development, data analysis, machine learning, scientific computing, and more.
Clean and Readable Code: Python's readability and elegant syntax make it a great language for both beginners and experienced developers.
Libraries and Frameworks: Python's extensive ecosystem of libraries and frameworks, such as Pandas, Flask, Django, NumPy, and scikit-learn, empowers developers to build robust and efficient solutions.
Asynchronous Programming: Python's asyncio and concurrent programming capabilities enable developers to write high-performance, non-blocking code for handling concurrent tasks.
Security and Best Practices: Secure coding practices, vulnerability mitigation, and secure web application development are essential to protect Python applications.
Deployment: Deploying Python applications can be done using containerization with Docker, various packaging options, and deployment strategies like Blue-Green, Canary, and Continuous Deployment.
Future Trends: Python continues to evolve with new features and updates, staying relevant in emerging fields like quantum computing, AI, web development, and more.
12.2 Embracing the Power of Python
Python's simplicity, readability, and versatility make it an ideal choice for both beginners and experienced programmers. As you continue your journey with Python, remember to:
Keep exploring new libraries and frameworks that align with your interests and projects.
Stay updated with the latest Python features and developments by following the Python community and official resources.
Embrace best practices in coding, security, and deployment to build robust and secure Python applications.
Python's future looks promising, and as you leverage its power, you'll find that it can be a valuable tool in solving a wide range of real-world problems and contributing to the ever-evolving landscape of technology and software development.
13. Bibliography
Here are books that I based my research on:
Subscribe to my newsletter
Read articles from Ebube Oguaju-Dike directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Ebube Oguaju-Dike
Ebube Oguaju-Dike
I am a developer from Nigeria. I am a Python developer and technical writer focused on data and machine learning. Tech Enthusiast in Blockchain, Hadoop, Python, Cyber-Security, Ethical Hacking. Interested in anything and everything about Computers.