An In-depth Guide to the Map Interface
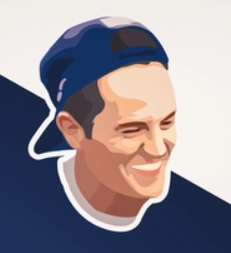
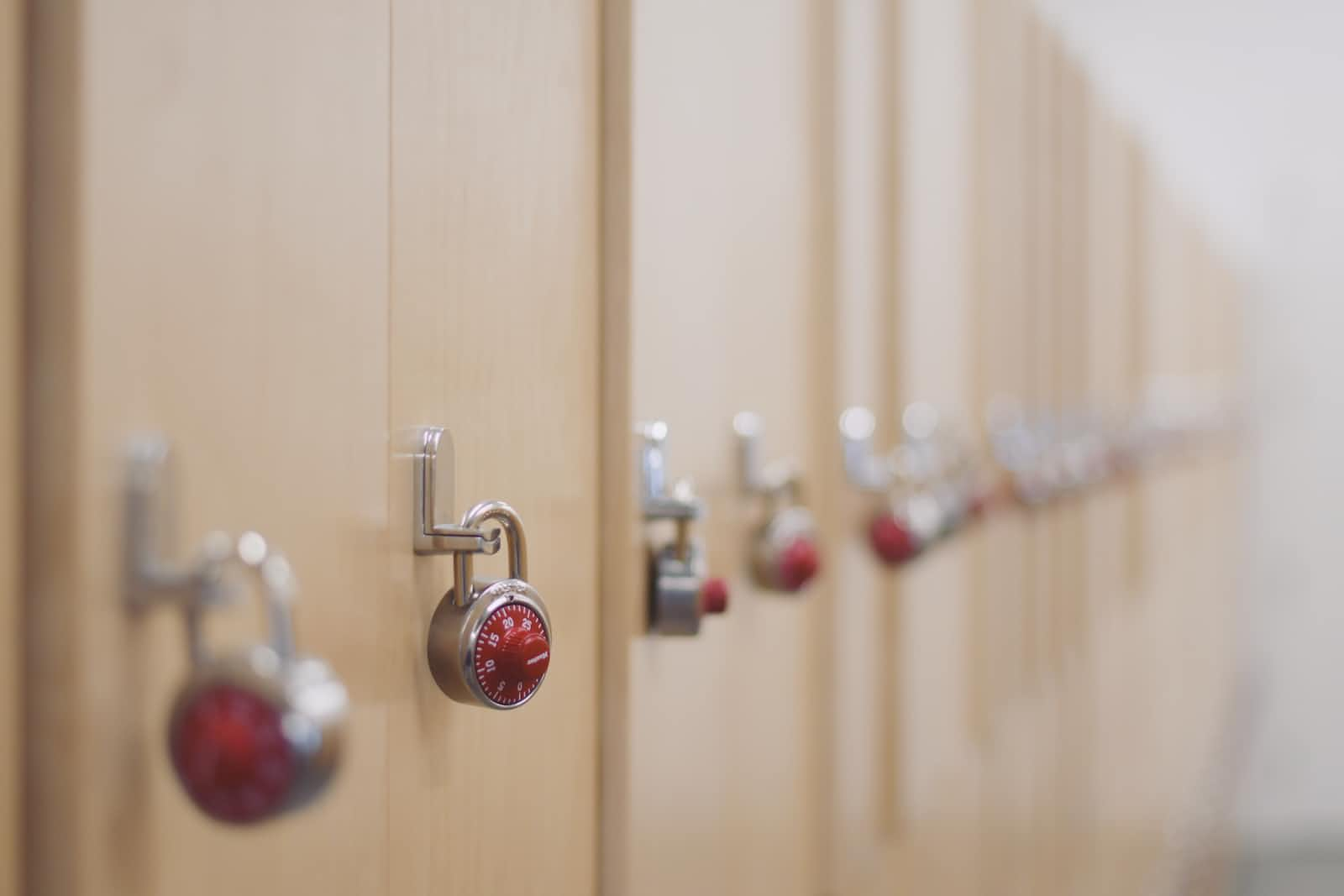
The Map interface is a fundamental part of the Java Collections Framework and is used to represent a collection of key-value pairs, where each unique key is associated with a value. It provides a way to store, retrieve, and manipulate data in a structured manner. In this introduction to the Map interface in Java, we'll explore its key features, common implementations, and basic usage.
Map interface does not extend the Collection framework.
Key Features of the Map Interface:
Key-Value Pairs: A Map stores data in the form of key-value pairs, where each key is unique, and it maps to a single value. This allows efficient retrieval of values based on their associated keys.
No Duplicate Keys: Map implementations do not allow duplicate keys. If you attempt to insert a duplicate key, it will overwrite the existing value associated with that key.
Associative: Maps are often used to represent associations between keys and values, making it easy to look up values based on their corresponding keys.
Iterability: Maps can be iterated over, allowing you to traverse and manipulate the key-value pairs within the map.
Null Values: Most Map implementations allow null values, but not null keys. This means you can have key-value pairs where the value is null.
Ordering: Some Map implementations, such as
LinkedHashMap
, maintain the order of insertion, while others likeHashMap
do not guarantee any specific order.
Common Implementations of the Map Interface:
Java provides several implementations of the Map interface, each with its own characteristics and use cases:
HashMap: HashMap is one of the most commonly used implementations of the Map interface in Java. It provides fast access to key-value pairs and is widely used in various applications. Here are the key points you need to know about HashMap:
Key-Value Pairs: HashMap stores data as key-value pairs. Each key is associated with a unique value, allowing efficient retrieval of values based on their keys.
No Duplicate Keys: HashMap does not allow duplicate keys. If you attempt to insert a key that already exists in the map, it will replace the existing value associated with that key.
Null Keys and Values: HashMap allows null values, and you can have key-value pairs where the value is null. However, it does not allow null keys. Attempting to insert a null key will result in a
NullPointerException
.Ordering: HashMap does not guarantee any specific order of key-value pairs. The order may change over time, especially when the map is resized.
Performance: HashMap provides constant-time (O(1)) average-case performance for basic operations like
get()
,put()
, andremove()
. However, in rare cases, when hash collisions occur frequently, the performance can degrade to O(n).Hashing: Internally, HashMap uses hashing to determine the storage location of key-value pairs. It computes a hash code for each key and uses that hash code to determine the index in the underlying array where the key-value pair should be stored. check below blog to understand Hashing.
Hash-based Search In Java using linked List
Load Factor: HashMap has a load factor that determines when the map should be resized. When the number of key-value pairs in the map exceeds the load factor multiplied by the current capacity, the map is resized to maintain performance. The default load factor is 0.75.
Capacity: HashMap has an initial capacity, which is the size of the underlying array used for storage. If you know the approximate number of elements you will store in the map, you can specify the initial capacity to optimize performance.
Thread-Safety: HashMap is not thread-safe by default. If you need thread safety, you should use
ConcurrentHashMap
or synchronize access to the HashMap using external synchronization mechanisms likesynchronized
blocks orCollections.synchronizedMap()
.Iterating: You can iterate over the key-value pairs in a HashMap using various methods, such as
entrySet()
,keySet()
, orvalues()
. For example, you can use afor-each
loop or an iterator to traverse the entries.Resizing: When the HashMap reaches its load factor, it automatically resizes itself by doubling its capacity and rehashing all key-value pairs. This ensures that the map remains efficient even as it grows.
Performance Trade-offs: While HashMap offers fast access and insertion, it may not be suitable for scenarios where you require ordering of keys or where you need sorted keys. In such cases, consider using
LinkedHashMap
orTreeMap
.Java Generics: You can specify the types of keys and values when creating a HashMap. For example,
HashMap<String, Integer>
would indicate a map with string keys and integer values.
Here's a basic example of using a HashMap in Java:
import java.util.HashMap;
import java.util.Map;
public class HashMapExample {
public static void main(String[] args) {
// Create a HashMap with String keys and Integer values
Map<String, Integer> scores = new HashMap<>();
// Insert key-value pairs
scores.put("Alice", 95);
scores.put("Bob", 88);
scores.put("Charlie", 92);
// Retrieve values based on keys
int aliceScore = scores.get("Alice");
System.out.println("Alice's score: " + aliceScore);
// Iterate over the map
for (Map.Entry<String, Integer> entry : scores.entrySet()) {
System.out.println(entry.getKey() + ": " + entry.getValue());
}
}
}
HashMap is a versatile and widely used data structure in Java, making it a valuable tool for various programming tasks involving key-value associations. Understanding its characteristics and proper usage is essential for efficient development.
LinkedHashMap: LinkedHashMap is another implementation of the
Map
interface in Java, just likeHashMap
. It extends HashMap and maintains the order of key-value pairs based on insertion. It's useful when you need to preserve the insertion order. However, it has some distinct characteristics and features that set it apart fromHashMap
. Here are the key points you need to know aboutLinkedHashMap
:Key-Value Pairs:
LinkedHashMap
stores data as key-value pairs, similar toHashMap
. Each key is associated with a unique value, allowing efficient retrieval of values based on their keys.No Duplicate Keys: Like
HashMap
,LinkedHashMap
does not allow duplicate keys. If you attempt to insert a key that already exists in the map, it will replace the existing value associated with that key.Null Keys and Values:
LinkedHashMap
allows null values, and you can have key-value pairs where the value is null. However, it does not allow null keys. Attempting to insert a null key will result in aNullPointerException
.Ordering: One of the key distinctions of
LinkedHashMap
is that it maintains the order of key-value pairs based on insertion. This means that when you iterate over the map, the order of elements will be the same as the order in which they were added.Performance:
LinkedHashMap
provides performance characteristics similar toHashMap
. Basic operations likeget()
,put()
, andremove()
have constant-time (O(1)) average-case performance. However, in rare cases when hash collisions occur frequently, performance can degrade to O(n).Hashing:
LinkedHashMap
uses hashing to determine the storage location of key-value pairs, just likeHashMap
. It computes a hash code for each key and uses that hash code to determine the index in the underlying array where the key-value pair should be stored.Load Factor: Similar to
HashMap
,LinkedHashMap
has a load factor that determines when the map should be resized. When the number of key-value pairs in the map exceeds the load factor multiplied by the current capacity, the map is resized to maintain performance. The default load factor is 0.75.Capacity:
LinkedHashMap
also has an initial capacity, which is the size of the underlying array used for storage. You can specify the initial capacity when creating aLinkedHashMap
if you know the approximate number of elements you will store in the map.Thread-Safety:
LinkedHashMap
is not thread-safe by default. If you need thread safety, you should useConcurrentHashMap
or synchronize access to theLinkedHashMap
using external synchronization mechanisms likesynchronized
blocks orCollections.synchronizedMap()
.Iterating: When you iterate over a
LinkedHashMap
, the order of elements will be the same as the insertion order. This can be useful when you need to maintain a specific order of elements, such as a chronological order of events.Access Order: In addition to insertion order,
LinkedHashMap
can be configured to maintain elements in access order. In access order mode, the most recently accessed elements move to the end of the iteration order. This feature is helpful for implementing LRU (Least Recently Used) caches.Java Generics: You can specify the types of keys and values when creating a
LinkedHashMap
. For example,LinkedHashMap<String, Integer>
would indicate a map with string keys and integer values.
Here's a basic example of using a LinkedHashMap
in Java:
import java.util.LinkedHashMap;
import java.util.Map;
public class LinkedHashMapExample {
public static void main(String[] args) {
// Create a LinkedHashMap with String keys and Integer values
Map<String, Integer> scores = new LinkedHashMap<>();
// Insert key-value pairs
scores.put("Alice", 95);
scores.put("Bob", 88);
scores.put("Charlie", 92);
// Retrieve values based on keys
int aliceScore = scores.get("Alice");
System.out.println("Alice's score: " + aliceScore);
// Iterate over the map (in insertion order)
for (Map.Entry<String, Integer> entry : scores.entrySet()) {
System.out.println(entry.getKey() + ": " + entry.getValue());
}
}
}
LinkedHashMap
is a useful choice when you need to maintain the order of key-value pairs based on insertion or access order, such as when implementing caching or maintaining a specific order of elements.
TreeMap: TreeMap is another implementation of the
Map
interface in Java, but it has distinct characteristics compared toHashMap
andLinkedHashMap
. TreeMap stores key-value pairs in a sorted order based on the keys. It's ideal for situations where you need keys to be sorted.Here are the key points you need to know about
TreeMap
:Key-Value Pairs:
TreeMap
stores data as key-value pairs, similar to otherMap
implementations. Each key is associated with a unique value, allowing efficient retrieval of values based on their keys.Sorted Keys: One of the primary distinctions of
TreeMap
is that it maintains the keys in a sorted order. The keys are automatically sorted according to their natural ordering (if they implement theComparable
interface) or by a customComparator
provided during TreeMap creation.No Duplicate Keys: Like other
Map
implementations,TreeMap
does not allow duplicate keys. If you attempt to insert a key that already exists in the map, it will replace the existing value associated with that key.Null Keys and Values:
TreeMap
does not allow null keys. If you attempt to insert a null key, it will result in aNullPointerException
. However, it does allow null values.Performance:
TreeMap
provides performance characteristics different fromHashMap
andLinkedHashMap
. Basic operations likeget()
,put()
, andremove()
have a time complexity of O(log n) due to the underlying Red-Black Tree structure. This makesTreeMap
suitable for scenarios where you require keys to be sorted.Red-Black Tree: Internally,
TreeMap
uses a Red-Black Tree data structure to maintain the sorted order of keys. The Red-Black Tree ensures balanced and efficient tree operations.Load Factor: Unlike
HashMap
andLinkedHashMap
,TreeMap
does not have a load factor because it doesn't rely on hashing for key placement. The tree structure maintains a balanced state.Thread-Safety:
TreeMap
is not thread-safe by default. If you need thread safety, you should useConcurrentSkipListMap
, which is a concurrent and thread-safe alternative.Iterating: When you iterate over a
TreeMap
, the elements are returned in sorted order, either natural or based on the customComparator
provided during TreeMap creation.Submaps:
TreeMap
provides methods to create submaps (views) based on a specified range of keys. This allows you to work with a subset of key-value pairs within the map efficiently.Navigable Map:
TreeMap
implements theNavigableMap
interface, which provides navigation and search operations for keys. You can find elements greater than, less than, or equal to a given key.Java Generics: You can specify the types of keys and values when creating a
TreeMap
. For example,TreeMap<String, Integer>
would indicate a map with string keys and integer values.
Here's a basic example of using a TreeMap
in Java:
import java.util.TreeMap;
import java.util.Map;
public class TreeMapExample {
public static void main(String[] args) {
// Create a TreeMap with String keys and Integer values
TreeMap<String, Integer> scores = new TreeMap<>();
// Insert key-value pairs
scores.put("Alice", 95);
scores.put("Bob", 88);
scores.put("Charlie", 92);
// Retrieve values based on keys (sorted order)
int aliceScore = scores.get("Alice");
System.out.println("Alice's score: " + aliceScore);
// Iterate over the map (in sorted order)
for (Map.Entry<String, Integer> entry : scores.entrySet()) {
System.out.println(entry.getKey() + ": " + entry.getValue());
}
}
}
TreeMap
is a useful choice when you need to maintain keys in a sorted order, such as when you need to implement a dictionary, maintain sorted collections, or perform range-based searches on keys.
Hashtable: Hashtable is a legacy Map implementation that is synchronized, making it thread-safe but potentially slower in multi-threaded environments. It does not allow null keys or values.It has some characteristics and features that distinguish it from other
Map
implementations likeHashMap
andTreeMap
. Here are the key points you need to know aboutHashtable
:Key-Value Pairs:
Hashtable
stores data as key-value pairs, similar to otherMap
implementations. Each key is associated with a unique value, allowing efficient retrieval of values based on their keys.No Duplicate Keys: Like other
Map
implementations,Hashtable
does not allow duplicate keys. If you attempt to insert a key that already exists in the map, it will replace the existing value associated with that key.Null Keys and Values:
Hashtable
does not allow null keys or null values. Attempting to insert a null key or value will result in aNullPointerException
.Synchronization: One of the primary distinctions of
Hashtable
is that it is synchronized, which means it is thread-safe by default. Multiple threads can safely access and modify aHashtable
concurrently without the need for external synchronization.Performance: Due to its synchronization,
Hashtable
can be slower than non-synchronized alternatives likeHashMap
. Basic operations likeget()
,put()
, andremove()
still have a time complexity of O(1) on average, but the synchronization overhead can impact performance in high-concurrency scenarios.Hashing: Internally,
Hashtable
uses hashing to determine the storage location of key-value pairs. It computes a hash code for each key and uses that hash code to determine the index in the underlying array where the key-value pair should be stored.Load Factor:
Hashtable
has a load factor that determines when the map should be resized. When the number of key-value pairs in the map exceeds the load factor multiplied by the current capacity, the map is resized to maintain performance. The default load factor is 0.75.Enumeration:
Hashtable
provides anEnumeration
interface that allows you to iterate over its keys and values. This is an older iteration mechanism compared to the enhanced for loop and iterators provided by modernMap
interfaces.Legacy: While
Hashtable
is still available in Java, it is considered a legacy class. In modern Java programming,HashMap
and other non-synchronized alternatives are preferred for most use cases due to better performance.Java Generics: You can specify the types of keys and values when creating a
Hashtable
. For example,Hashtable<String, Integer>
would indicate a map with string keys and integer values.
Here's a basic example of using a Hashtable
in Java:
import java.util.Hashtable;
import java.util.Map;
public class HashtableExample {
public static void main(String[] args) {
// Create a Hashtable with String keys and Integer values
Hashtable<String, Integer> scores = new Hashtable<>();
// Insert key-value pairs
scores.put("Alice", 95);
scores.put("Bob", 88);
scores.put("Charlie", 92);
// Retrieve values based on keys
int aliceScore = scores.get("Alice");
System.out.println("Alice's score: " + aliceScore);
// Iterate over the map using Enumeration
Enumeration<String> keys = scores.keys();
while (keys.hasMoreElements()) {
String key = keys.nextElement();
int value = scores.get(key);
System.out.println(key + ": " + value);
}
}
}
Hashtable
is primarily used in scenarios where thread safety is a top priority, but in most cases, ConcurrentHashMap
or other modern thread-safe alternatives are preferred due to better performance and more features.
ConcurrentHashMap: This implementation is designed for high-concurrency scenarios. It offers thread-safety without the need for external synchronization.
ConcurrentHashMap
is a highly concurrent and thread-safe implementation of theMap
interface in Java. It is designed for efficient concurrent access by multiple threads, making it suitable for high-concurrency scenarios. Here are the key points you need to know aboutConcurrentHashMap
:Key-Value Pairs:
ConcurrentHashMap
stores data as key-value pairs, similar to otherMap
implementations. Each key is associated with a unique value, allowing efficient retrieval of values based on their keys.No Duplicate Keys: Like other
Map
implementations,ConcurrentHashMap
does not allow duplicate keys. If you attempt to insert a key that already exists in the map, it will replace the existing value associated with that key.Null Keys and Values:
ConcurrentHashMap
does not allow null keys or null values. Attempting to insert a null key or value will result in aNullPointerException
.Concurrency:
ConcurrentHashMap
is designed for high concurrency. It allows multiple threads to read and modify the map concurrently without the need for external synchronization. It uses fine-grained locking and other techniques to achieve this.Performance:
ConcurrentHashMap
provides good performance in multi-threaded scenarios. Basic operations likeget()
,put()
, andremove()
have good scalability and typically perform well under high concurrency.Load Factor: Similar to
HashMap
,ConcurrentHashMap
has a load factor that determines when the map should be resized. When the number of key-value pairs in the map exceeds the load factor multiplied by the current capacity, the map is resized to maintain performance. The default load factor is 0.75.Segmentation:
ConcurrentHashMap
is divided into segments or partitions, each of which can be locked independently. This allows multiple threads to access different segments concurrently, reducing contention and improving performance.Iterating: You can iterate over a
ConcurrentHashMap
, but it does not provide a strong consistency guarantee. Iterating over aConcurrentHashMap
may not reflect the most recent changes made by other threads.Java Generics: You can specify the types of keys and values when creating a
ConcurrentHashMap
. For example,ConcurrentHashMap<String, Integer>
would indicate a map with string keys and integer values.Memory Overhead:
ConcurrentHashMap
may have a slightly higher memory overhead compared to non-concurrent map implementations likeHashMap
due to the additional data structures required for concurrency control.Atomic Operations:
ConcurrentHashMap
supports atomic operations, such asputIfAbsent()
,remove(key, value)
, andreplace(key, oldValue, newValue)
, which can be useful in multi-step operations.
Here's a basic example of using a ConcurrentHashMap
in Java:
import java.util.concurrent.ConcurrentHashMap;
import java.util.Map;
public class ConcurrentHashMapExample {
public static void main(String[] args) {
// Create a ConcurrentHashMap with String keys and Integer values
ConcurrentHashMap<String, Integer> scores = new ConcurrentHashMap<>();
// Insert key-value pairs
scores.put("Alice", 95);
scores.put("Bob", 88);
scores.put("Charlie", 92);
// Retrieve values based on keys (concurrently safe)
int aliceScore = scores.get("Alice");
System.out.println("Alice's score: " + aliceScore);
// Iterate over the map (may not provide strong consistency)
scores.forEach((key, value) -> {
System.out.println(key + ": " + value);
});
}
}
ConcurrentHashMap
is particularly useful in multi-threaded applications where you need a thread-safe map with good performance under high concurrency. It is a preferred choice over Hashtable
in modern Java programming due to better performance and more advanced concurrency control mechanisms.
This is all about Map Interface, developers can choose the most suitable map type based on specific requirements.
My Other Blogs :
Subscribe to my newsletter
Read articles from Hemant Besra directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
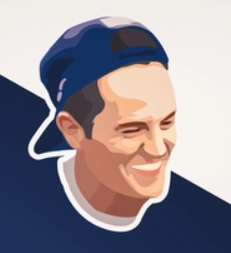
Hemant Besra
Hemant Besra
Experienced Full Stack Java developer. Have Strong Experience in JSP/Servlet, JSF, Jasper Report, Spring Framework, hibernate, Angular 5+, Microservices. Experienced in Front-end technologies such as HTML, CSS, JavaScript, angular 6+, AJAX, JSON, and XML. Strong Hands-on experience on working with Reactive Forms to build form-based application in Angular 6+.