3.4 Sets

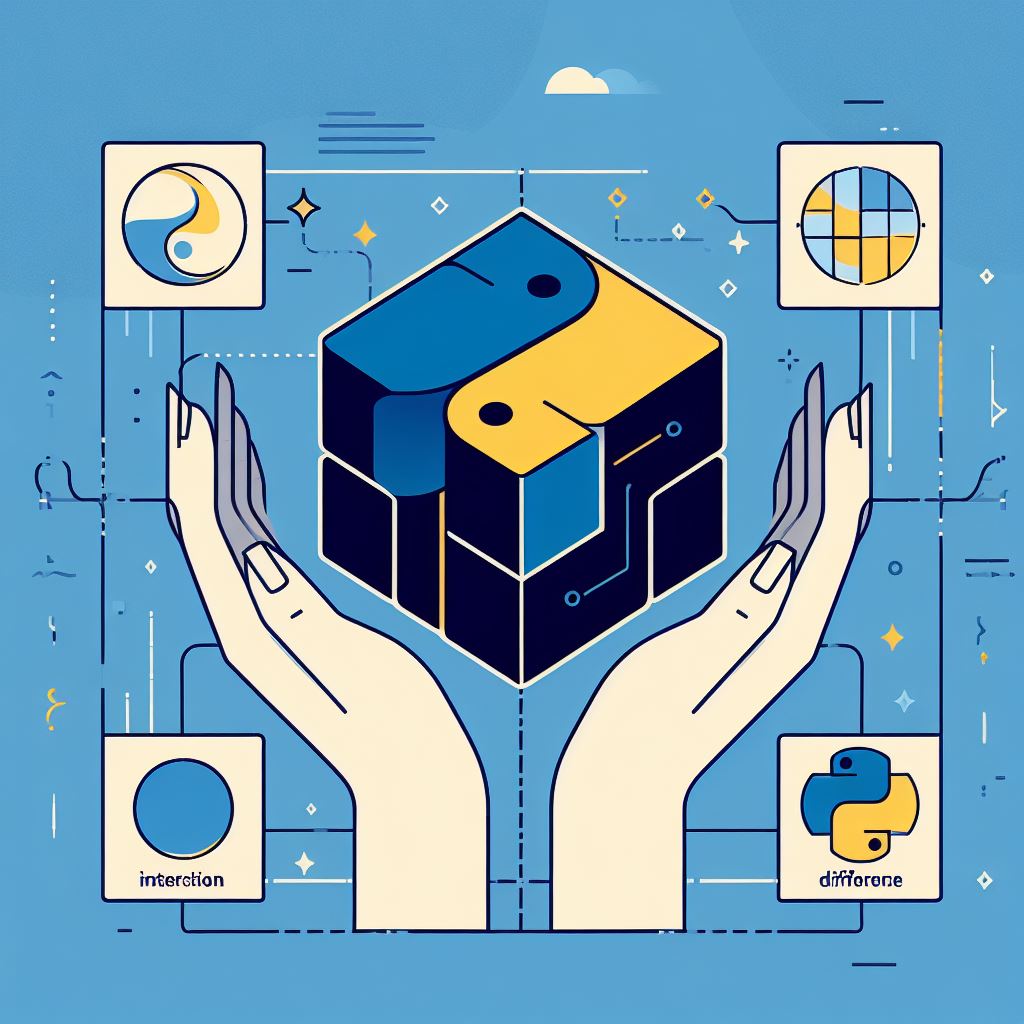
In Python, a set is an unordered collection of unique elements. It is a powerful data structure that allows you to perform various operations such as union, intersection, difference, and symmetric difference. Sets are mutable, which means you can add or remove elements from them. In this section, we will explore the concept of sets in Python and learn how to use them effectively.
Creating a Set
To create a set in Python, you can use curly braces {}
or the set()
function. Let's see some examples:
# Creating an empty set
empty_set = set()
print(empty_set) # Output: set()
# Creating a set with elements
fruits = {'apple', 'banana', 'orange'}
print(fruits) # Output: {'apple', 'banana', 'orange'}
As you can see, the elements in a set are enclosed in curly braces and separated by commas. Sets can contain elements of different data types, such as strings, numbers, and even other sets.
Adding and Removing Elements
You can add elements to a set using the add()
method. If the element already exists in the set, it will not be added again. To remove elements, you can use the remove()
or discard()
methods. The difference between these two methods is that remove()
will raise an error if the element does not exist in the set, while discard()
will not.
fruits = {'apple', 'banana', 'orange'}
# Adding an element
fruits.add('grape')
print(fruits) # Output: {'apple', 'banana', 'orange', 'grape'}
# Removing an element
fruits.remove('banana')
print(fruits) # Output: {'apple', 'orange', 'grape'}
# Removing a non-existent element
fruits.discard('watermelon')
print(fruits) # Output: {'apple', 'orange', 'grape'}
Set Operations
Sets in Python support various operations that can be performed on them. Let's explore some of the commonly used set operations:
- Union: The union of two sets contains all the unique elements from both sets. You can use the
union()
method or the|
operator to perform the union operation.
set1 = {1, 2, 3}
set2 = {3, 4, 5}
# Union using union() method
union_set = set1.union(set2)
print(union_set) # Output: {1, 2, 3, 4, 5}
# Union using | operator
union_set = set1 | set2
print(union_set) # Output: {1, 2, 3, 4, 5}
- Intersection: The intersection of two sets contains the common elements between them. You can use the
intersection()
method or the&
operator to perform the intersection operation.
set1 = {1, 2, 3}
set2 = {3, 4, 5}
# Intersection using intersection() method
intersection_set = set1.intersection(set2)
print(intersection_set) # Output: {3}
# Intersection using & operator
intersection_set = set1 & set2
print(intersection_set) # Output: {3}
- Difference: The difference between two sets contains the elements that are present in the first set but not in the second set. You can use the
difference()
method or the-
operator to perform the difference operation.
set1 = {1, 2, 3}
set2 = {3, 4, 5}
# Difference using difference() method
difference_set = set1.difference(set2)
print(difference_set) # Output: {1, 2}
# Difference using - operator
difference_set = set1 - set2
print(difference_set) # Output: {1, 2}
- Symmetric Difference: The symmetric difference between two sets contains the elements that are present in either of the sets, but not in both. You can use the
symmetric_difference()
method or the^
operator to perform the symmetric difference operation.
set1 = {1, 2, 3}
set2 = {3, 4, 5}
# Symmetric difference using symmetric_difference() method
symmetric_difference_set = set1.symmetric_difference(set2)
print(symmetric_difference_set) # Output: {1, 2, 4, 5}
# Symmetric difference using ^ operator
symmetric_difference_set = set1 ^ set2
print(symmetric_difference_set) # Output: {1, 2, 4, 5}
Set Methods
Sets in Python come with a variety of useful methods that allow you to manipulate and perform operations on sets. Here are some commonly used set methods:
add(element)
: Adds an element to the set.remove(element)
: Removes an element from the set. Raises an error if the element does not exist.discard(element)
: Removes an element from the set. Does not raise an error if the element does not exist.clear()
: Removes all elements from the set.copy()
: Returns a shallow copy of the set.pop()
: Removes and returns an arbitrary element from the set.update(iterable)
: Updates the set by adding elements from an iterable.intersection_update(iterable)
: Updates the set by keeping only the common elements with an iterable.difference_update(iterable)
: Updates the set by removing the elements that are present in an iterable.symmetric_difference_update(iterable)
: Updates the set by keeping only the elements that are present in either the set or an iterable, but not both.
fruits = {'apple', 'banana', 'orange'}
# Adding an element
fruits.add('grape')
print(fruits) # Output: {'apple', 'banana', 'orange', 'grape'}
# Removing an element
fruits.remove('banana')
print(fruits) # Output: {'apple', 'orange', 'grape'}
# Clearing the set
fruits.clear()
print(fruits) # Output: set()
# Updating the set
fruits.update(['apple', 'banana', 'orange'])
print(fruits) # Output: {'apple', 'banana', 'orange'}
# Intersection update
fruits.intersection_update(['banana', 'orange'])
print(fruits) # Output: {'banana', 'orange'}
Sets are a valuable tool in Python when you need to work with unique elements and perform operations such as unions, intersections, differences, and symmetric differences. They provide a convenient and efficient way to handle data without worrying about duplicates. By understanding the concepts and operations of sets, you can unlock the full potential of Python in your programming journey.
Subscribe to my newsletter
Read articles from ESlavin1808 directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
