3.5 File Handling

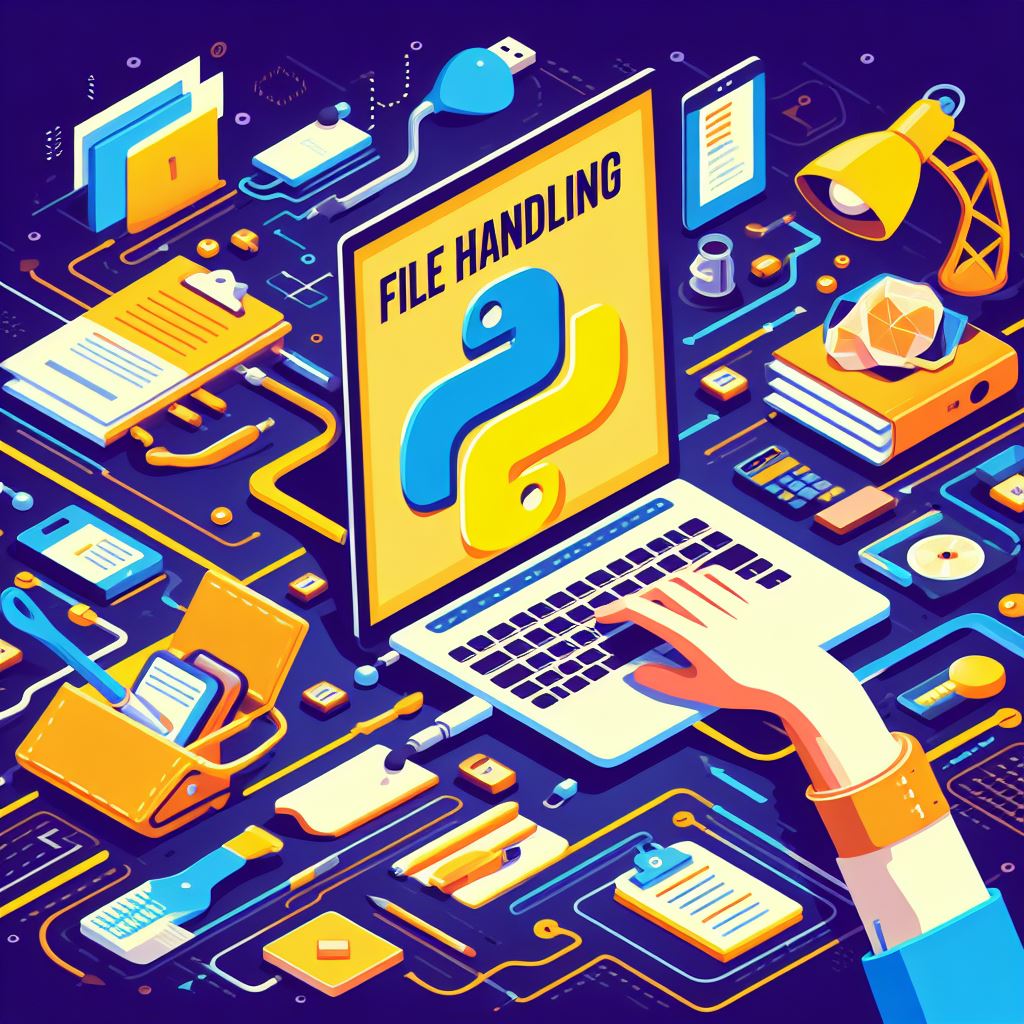
File handling is an essential aspect of programming, as it allows us to read and write data to and from files. In Python, file handling is made easy with built-in functions and methods that simplify the process. In this section, we will explore the various techniques and methods available for file handling in Python.
Opening and Closing Files
Before we can perform any operations on a file, we need to open it. Python provides the open()
function for this purpose. The open()
function takes two parameters: the file name and the mode in which the file should be opened. The mode can be "r" for reading, "w" for writing, or "a" for appending to an existing file. Additionally, we can specify whether the file should be opened in text mode or binary mode by adding "t" or "b" to the mode, respectively.
Once we have finished working with a file, it is important to close it to free up system resources. We can use the close()
method to close an open file. It is good practice to always close files after we are done with them.
# Opening a file in read mode
file = open("data.txt", "r")
# Opening a file in write mode
file = open("data.txt", "w")
# Opening a file in append mode
file = open("data.txt", "a")
# Closing a file
file.close()
Reading from Files
Python provides several methods for reading data from files. The most common method is the read()
method, which reads the entire contents of a file as a string. We can also use the readline()
method to read a single line from a file, or the readlines()
method to read all lines of a file into a list.
# Reading the entire file
file = open("data.txt", "r")
content = file.read()
print(content)
file.close()
# Reading a single line
file = open("data.txt", "r")
line = file.readline()
print(line)
file.close()
# Reading all lines into a list
file = open("data.txt", "r")
lines = file.readlines()
print(lines)
file.close()
Writing to Files
To write data to a file, we can use the write()
method. This method takes a string as a parameter and writes it to the file. If the file does not exist, it will be created. If it already exists, the existing content will be overwritten. To append data to an existing file, we can use the write()
method in conjunction with the "a" mode.
# Writing to a file
file = open("data.txt", "w")
file.write("Hello, World!")
file.close()
# Appending to a file
file = open("data.txt", "a")
file.write("This is a new line.")
file.close()
Handling Exceptions
When working with files, it is important to handle exceptions that may occur. For example, if a file does not exist or cannot be opened, an exception will be raised. To handle such exceptions, we can use the try-except
block.
try:
file = open("data.txt", "r")
content = file.read()
print(content)
file.close()
except FileNotFoundError:
print("File not found.")
except Exception as e:
print("An error occurred:", str(e))
Using the with
Statement
Python provides a convenient way to handle file operations using the with
statement. The with
statement automatically takes care of opening and closing the file, even if an exception occurs. This ensures that the file is always properly closed, regardless of the outcome of the operations.
with open("data.txt", "r") as file:
content = file.read()
print(content)
File Handling Best Practices
When working with files in Python, it is important to follow some best practices to ensure efficient and error-free code:
Always close files after you are done with them to free up system resources.
Use the
with
statement whenever possible to automatically handle file operations.Handle exceptions that may occur when opening, reading, or writing to files.
Use descriptive file names and file extensions to make your code more readable.
Avoid hardcoding file paths and use relative paths or command-line arguments instead.
By following these best practices, you can effectively handle files in Python and unlock the full potential of your programs.
Subscribe to my newsletter
Read articles from ESlavin1808 directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
