My Problem-Solving Blog - 1
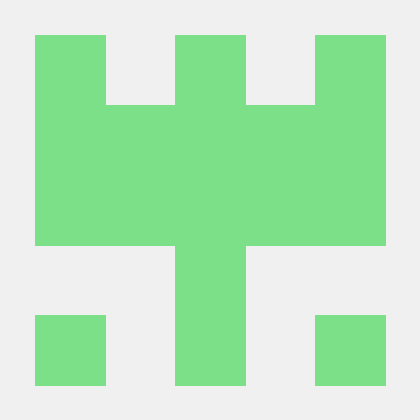
Encoded Strings
Today's problem was a HackerEarth daily problem, which I thought would be simple and complete in max. 5-6 min. But instead, it took almost 20 min. the reason behind this was my negligence and overconfidence.
the problem statement can be accessed from the link at the top of the blog.
Initially, I thought, that it was quite a simple problem, as I just needed to convert every character to its equivalent numerical order in the English alphabet. Finally, the number I will get later should be checked if it's divisible by 6 or not. Print yes and no accordingly. Sounds simple? It was a bit tricky for me when I read the question first. I took it too lightly, swiftly converted the given string to an equivalent number, and tried to print yes or no if divisible by 6. But I missed the constraint. The string can have at most 10^5 characters. That means my number should be max. Of 10^5 digits. Which is not possible as it surpasses the storage limit of int or long long.
Initial Approach ( partially accepted )
#include <bits/stdc++.h>
using namespace std;
int main() {
string s;
cin>>s;
int num = 0;
for(int i = 0;i<s.length();i++){
int temp = (int)s[i] - (int)('a');
num = num*10 + (temp+1);
}
num%6==0?cout<<"YES"<<endl:cout<<"NO"<<endl;
}
When I noticed the constraint, I suddenly realized my mistake and switched my approach. I decided to check the divisibility of the large number by 2 and 3 as if it satisfies the condition, it will ultimately be divisible by 6 as well.
Hence, I stored the sum of the number in a separate variable and checked its divisibility by 3. As for divisibility by 2, I just had to check if the last digit is even or odd.
Final Solution ( all test cases passed )
#include <bits/stdc++.h>
using namespace std;
int main() {
string s;
cin>>s;
long long sum = 0;
for(int i = 0;i<s.length();i++){
int temp = (int)s[i] - (int)('a')+1;
sum += temp;
}
int last = (int)s[s.length()-1] - (int)('a')+1;
sum%3==0 && last%2 == 0 ? cout<<"YES":cout<<"NO";
}
Conclusion
I solved the problem successfully, but I learned a lesson, that no matter how simple the problem is, don't let your overconfidence rule you. because a minor negligence may cost you resources. Reviews and optimal solutions will be much appreciated.
Subscribe to my newsletter
Read articles from Randip Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
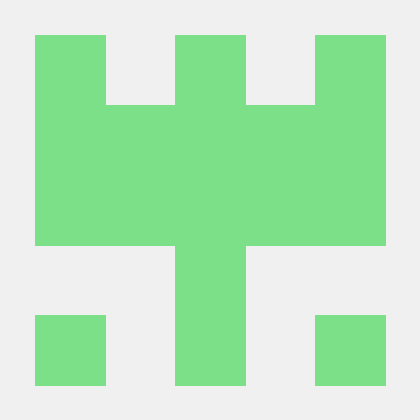