5 Python Modules and Packages

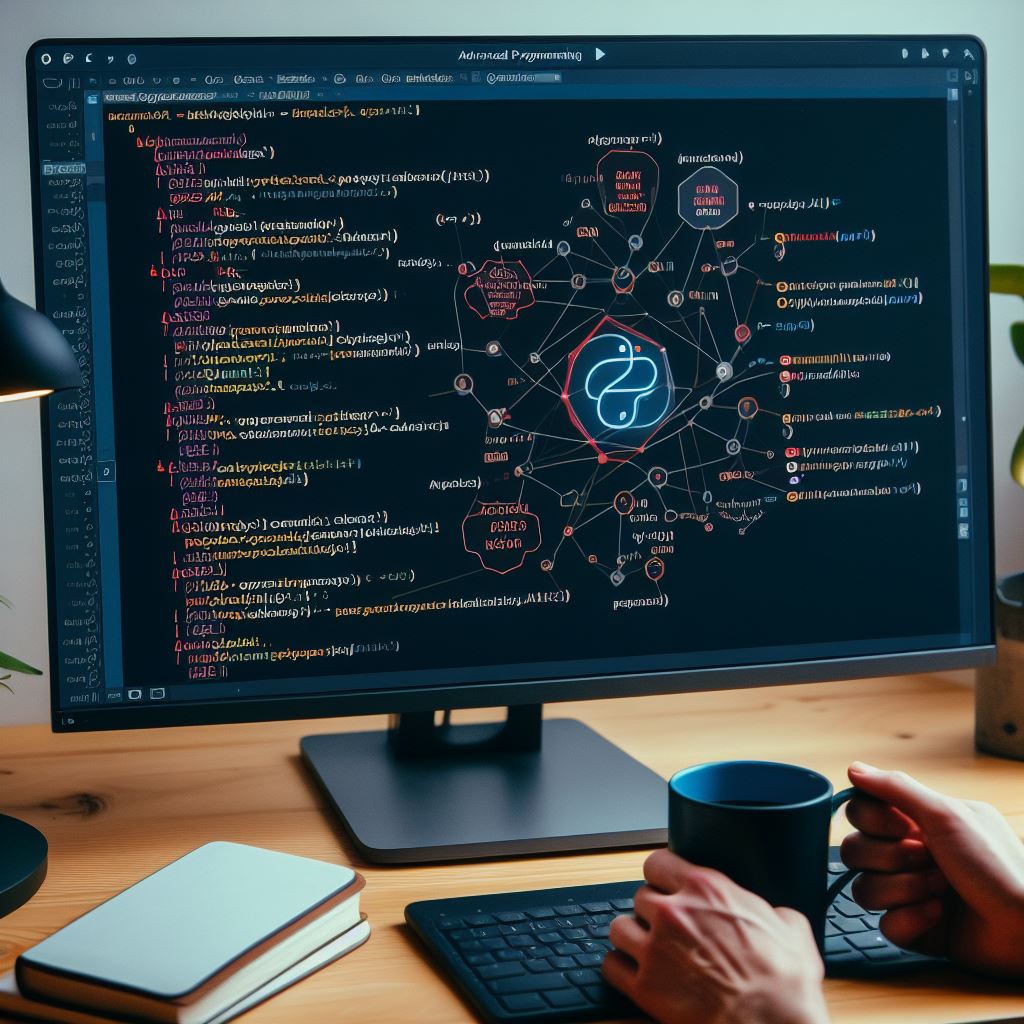
5.1 Importing Modules
In Python, modules are files that contain Python code, which can be used to define functions, classes, and variables. These modules can be imported into other Python programs to reuse code and access the functionality provided by the module. Importing modules is a fundamental concept in Python programming and allows developers to leverage the power of existing code libraries and packages.
The import
Statement
To import a module in Python, you use the import
statement followed by the name of the module. For example, to import the math
module, you would write:
import math
This statement makes all the functions and variables defined in the math
module available in your program. You can then use these functions and variables by referencing them with the module name followed by a dot. For example, to use the sqrt
function from the math
module, you would write:
result = math.sqrt(25)
Importing Specific Functions or Variables
Sometimes, you may only need to use a specific function or variable from a module, rather than importing the entire module. In such cases, you can use the from
keyword followed by the module name and the specific function or variable you want to import. For example, to import only the sqrt
function from the math
module, you would write:
from math import sqrt
This statement allows you to directly use the sqrt
function without referencing the module name. You can then use the function as follows:
result = sqrt(25)
You can also import multiple functions or variables from a module by separating them with commas. For example:
from math import sqrt, sin, cos
Importing Modules with Aliases
In some cases, you may want to import a module with a different name to avoid naming conflicts or to provide a shorter name for convenience. You can achieve this by using the as
keyword followed by the desired alias name. For example, to import the math
module with the alias m
, you would write:
import math as m
This statement allows you to use the functions and variables from the math
module by referencing them with the alias name. For example:
result = m.sqrt(25)
Importing All Functions and Variables
If you want to import all the functions and variables from a module without explicitly specifying each one, you can use the *
wildcard character. For example, to import all functions and variables from the math
module, you would write:
from math import *
However, it is generally recommended to avoid using the *
wildcard import, as it can make your code less readable and may lead to naming conflicts if multiple modules define functions or variables with the same name.
Importing Modules from Different Directories
By default, Python looks for modules in the current directory and the directories specified in the PYTHONPATH
environment variable. However, if the module you want to import is located in a different directory, you need to specify the path to that directory.
To import a module from a specific directory, you can use the sys
module and its path
attribute. The sys.path
attribute is a list that contains the directories Python searches for modules. You can add the desired directory to this list using the append()
method. For example, to import a module named my_module
located in the directory /path/to/module
, you would write:
import sys
sys.path.append('/path/to/module')
import my_module
This allows you to import the my_module
module from the specified directory.
Conclusion
Importing modules is a crucial aspect of Python programming. It allows you to leverage existing code libraries and packages, making your development process more efficient and productive. Whether you need to import specific functions or variables, use aliases, or import modules from different directories, Python provides flexible and powerful mechanisms to handle module imports. Understanding how to import modules effectively will greatly enhance your ability to write clean and modular Python code.
5.2 Creating Modules
In Python, a module is a file containing Python definitions and statements. It serves as a way to organize and reuse code. Modules can be imported into other Python programs, allowing you to use the functions, classes, and variables defined in the module.
Creating your own modules in Python is a straightforward process. You can start by creating a new file with a .py extension, which will serve as your module. Let's explore the steps involved in creating modules in Python.
Creating a Simple Module
To create a module, you need to define the functions, classes, or variables that you want to include in the module. Let's start by creating a simple module called "math_operations.py" that contains some basic mathematical operations.
# math_operations.py
def add(a, b):
return a + b
def subtract(a, b):
return a - b
def multiply(a, b):
return a * b
def divide(a, b):
return a / b
In the above example, we have defined four functions: add
, subtract
, multiply
, and divide
. These functions perform basic mathematical operations. Now, let's see how we can use this module in another Python program.
Using a Module
To use a module in Python, you need to import it into your program. You can import the entire module or specific functions, classes, or variables from the module.
To import the entire module, you can use the import
statement followed by the name of the module. For example, to import the math_operations
module we created earlier, you can use the following code:
import math_operations
result = math_operations.add(5, 3)
print(result) # Output: 8
In the above code, we import the math_operations
module and use the add
function from the module to add two numbers.
If you only need to use specific functions or variables from a module, you can import them individually using the from
keyword. For example, to import only the add
function from the math_operations
module, you can use the following code:
from math_operations import add
result = add(5, 3)
print(result) # Output: 8
In this case, you don't need to prefix the function name with the module name when using it.
Module Search Path
When you import a module, Python searches for it in a specific order. It first looks for built-in modules, then searches for modules in directories specified by the PYTHONPATH
environment variable, and finally searches in the default system path.
To see the list of directories where Python searches for modules, you can use the sys.path
variable. This variable is a list that contains the directories in the module search path.
import sys
print(sys.path)
The output will be a list of directories where Python searches for modules.
Creating Packages
In addition to creating individual modules, you can also organize related modules into packages. A package is simply a directory that contains multiple modules. It allows you to group related functionality together and provides a way to organize your code.
To create a package, you need to create a directory with a special file called __init__.py
. This file can be empty or can contain initialization code for the package.
Let's say we want to create a package called "shapes" that contains modules for different geometric shapes. Here's how the directory structure would look:
shapes/
__init__.py
circle.py
rectangle.py
triangle.py
In this example, the shapes
directory is the package, and it contains three modules: circle.py
, rectangle.py
, and triangle.py
. The __init__.py
file indicates that this directory is a package.
To use modules from a package, you can import them using the package name followed by the module name. For example, to import the circle
module from the shapes
package, you can use the following code:
import shapes.circle
radius = 5
area = shapes.circle.calculate_area(radius)
print(area)
In the above code, we import the circle
module from the shapes
package and use the calculate_area
function from the module to calculate the area of a circle.
Alternatively, you can use the from
keyword to import specific functions or variables from a module within a package. For example:
from shapes.circle import calculate_area
radius = 5
area = calculate_area(radius)
print(area)
In this case, we import only the calculate_area
function from the circle
module.
Creating modules and packages in Python allows you to organize and reuse your code effectively. By encapsulating related functionality into modules and packages, you can create more maintainable and modular code.
5.3 Using Packages
In Python, a package is a way to organize related modules into a single directory hierarchy. It provides a convenient way to group related functionality together, making it easier to manage and reuse code. Packages are an essential part of Python's modular design and are widely used in the Python ecosystem.
What is a Package?
A package is simply a directory that contains Python modules. It can also contain sub-packages, which are themselves directories that contain modules. The main purpose of a package is to provide a hierarchical structure for organizing related modules.
Packages are identified by their directory structure. Each package must have a special file called __init__.py
in its directory. This file can be empty, but it is necessary to mark the directory as a package. The __init__.py
file can also contain initialization code that is executed when the package is imported.
Importing Packages
To use a package in your Python code, you need to import it. The import
statement is used to import packages and modules. When importing a package, you can either import the entire package or specific modules within the package.
To import the entire package, you can use the following syntax:
import package_name
For example, if you have a package called math
that contains mathematical functions, you can import it like this:
import math
Once the package is imported, you can access its modules and functions using dot notation. For example, to use the sqrt
function from the math
package, you would write:
result = math.sqrt(16)
If you only need to import specific modules from a package, you can use the from
keyword. This allows you to import specific modules directly into your code without having to use the package name as a prefix.
from package_name import module_name
For example, if you only need to import the sqrt
function from the math
package, you can write:
from math import sqrt
Now you can use the sqrt
function directly without having to use the package name as a prefix:
result = sqrt(16)
Creating Your Own Packages
Creating your own packages is a great way to organize and distribute your code. To create a package, you need to follow a specific directory structure and include the __init__.py
file in each package directory.
Here's an example of how you can create a package called my_package
:
Create a directory called
my_package
.Inside the
my_package
directory, create a file called__init__.py
. This file can be empty or contain initialization code.Inside the
my_package
directory, create one or more Python modules. These modules can contain functions, classes, or any other code you want to include in your package.
Once you have created your package, you can import it in your code using the import
statement, just like any other package.
Popular Python Packages
The Python ecosystem is rich with a wide variety of packages that extend the functionality of the language. These packages cover a wide range of domains, including web development, data analysis, machine learning, and more. Here are a few popular Python packages that you might find useful:
NumPy: NumPy is a powerful package for scientific computing in Python. It provides support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays.
Pandas: Pandas is a data manipulation and analysis library. It provides data structures like DataFrames and Series, which make it easy to work with structured data. Pandas also offers a wide range of functions for data cleaning, transformation, and analysis.
Matplotlib: Matplotlib is a plotting library that allows you to create a wide variety of static, animated, and interactive visualizations in Python. It provides a high-level interface for creating charts, plots, histograms, and more.
Django: Django is a high-level web framework that follows the model-view-controller (MVC) architectural pattern. It provides a robust set of tools and features for building web applications quickly and efficiently.
Flask: Flask is a lightweight web framework that is easy to learn and use. It provides a simple and flexible way to build web applications and APIs. Flask is often used for small to medium-sized projects or as a starting point for larger applications.
These are just a few examples of the many packages available in the Python ecosystem. Depending on your specific needs, you can explore and leverage the vast collection of packages to enhance your Python projects.
Conclusion
Packages are a fundamental part of Python's modular design and provide a way to organize and distribute code. They allow you to group related functionality together, making it easier to manage and reuse code. By understanding how to import and create packages, you can take advantage of the vast collection of packages available in the Python ecosystem and enhance your Python projects with additional functionality.
5.4 Exploring Popular Python Packages
Python is a versatile programming language that offers a wide range of packages and libraries to enhance its functionality. These packages are created by the Python community and are available for developers to use in their projects. In this section, we will explore some of the popular Python packages that can be used to solve various problems and simplify development tasks.
NumPy
NumPy is a fundamental package for scientific computing in Python. It provides support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays efficiently. NumPy is widely used in fields such as physics, mathematics, engineering, and data science. It offers a powerful N-dimensional array object, which allows for efficient manipulation of large datasets. With NumPy, you can perform complex mathematical operations, such as linear algebra, Fourier transforms, and random number generation, with ease.
Pandas
Pandas is a powerful data manipulation and analysis library for Python. It provides data structures and functions to efficiently handle and analyze structured data, such as tabular data and time series. Pandas is built on top of NumPy and provides additional functionality for data cleaning, transformation, and exploration. With Pandas, you can easily load data from various sources, such as CSV files, Excel spreadsheets, and SQL databases, and perform operations like filtering, grouping, and merging. It also offers powerful tools for data visualization, making it a popular choice among data scientists and analysts.
Matplotlib
Matplotlib is a plotting library for Python that provides a flexible and comprehensive set of tools for creating static, animated, and interactive visualizations. It is widely used for generating high-quality plots, charts, and graphs in various formats, such as PNG, PDF, and SVG. Matplotlib allows you to create a wide range of plots, including line plots, scatter plots, bar plots, histograms, and more. It provides fine-grained control over every aspect of the plot, allowing you to customize the appearance and style to suit your needs. With Matplotlib, you can effectively communicate your data and insights through visually appealing visualizations.
TensorFlow
TensorFlow is an open-source machine learning framework developed by Google. It provides a comprehensive ecosystem of tools, libraries, and resources for building and deploying machine learning models. TensorFlow is designed to be flexible and scalable, allowing you to develop models for a wide range of applications, from image recognition to natural language processing. It provides a high-level API called Keras, which simplifies the process of building and training deep learning models. TensorFlow also supports distributed computing, allowing you to train models on multiple machines or GPUs for faster performance.
Scikit-learn
Scikit-learn is a machine learning library for Python that provides a wide range of algorithms and tools for data mining and analysis. It is built on top of NumPy, SciPy, and Matplotlib, and integrates well with the Python ecosystem. Scikit-learn offers a unified interface for various machine learning tasks, such as classification, regression, clustering, and dimensionality reduction. It provides a rich set of pre-processing techniques, model evaluation metrics, and model selection tools to help you build and evaluate machine learning models effectively. Scikit-learn is widely used in academia and industry for its simplicity, performance, and extensive documentation.
Django
Django is a high-level web framework for Python that follows the model-view-controller (MVC) architectural pattern. It provides a robust set of tools and features for building web applications quickly and efficiently. Django handles many common web development tasks, such as URL routing, form handling, database management, and user authentication, out of the box. It also includes a powerful object-relational mapper (ORM) that allows you to interact with the database using Python code instead of SQL queries. Django follows the principle of "Don't Repeat Yourself" (DRY), promoting code reusability and maintainability. It is widely used by developers to build scalable and secure web applications.
Flask
Flask is a lightweight web framework for Python that is designed to be simple and easy to use. It provides a minimalistic set of tools and features for building web applications, allowing developers to have more control over the application structure and design. Flask follows the principle of "microservices," where each component of the application is kept small and focused. It provides a flexible routing system, template engine, and support for various extensions to add additional functionality. Flask is often used for building small to medium-sized web applications or APIs, where simplicity and flexibility are key requirements.
These are just a few examples of the popular Python packages available for developers. Python's extensive package ecosystem makes it a powerful language for a wide range of applications, from scientific computing to web development and machine learning. By leveraging these packages, developers can save time and effort in building complex functionalities and focus on solving the core problems at hand.
Subscribe to my newsletter
Read articles from ESlavin1808 directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
