6.1 File Operations

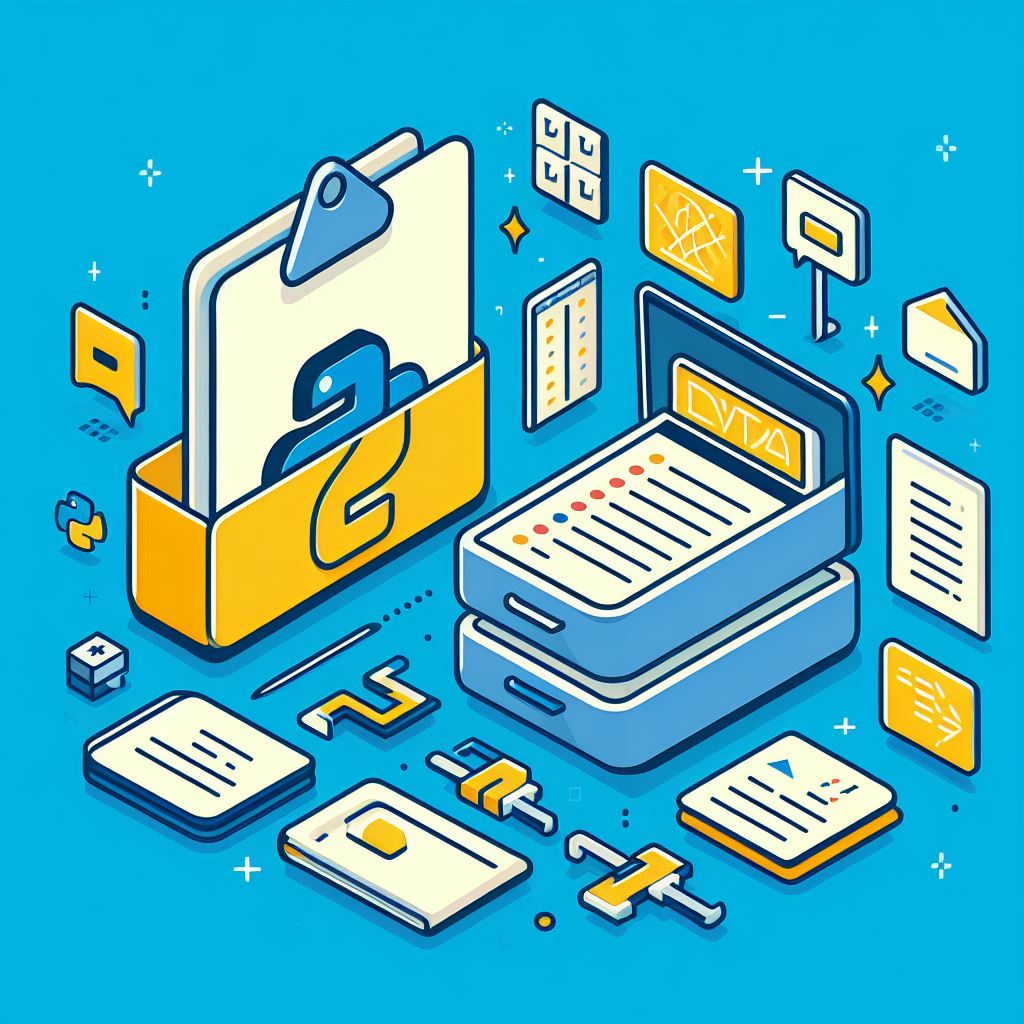
In Python, file operations are an essential part of working with data and managing information. Whether you need to read data from a file, write data to a file, or perform other file-related tasks, Python provides a variety of functions and methods to make these operations easy and efficient.
Opening and Closing Files
Before you can perform any file operations, you need to open the file. Python provides the open()
function for this purpose. The open()
function takes two parameters: the file name and the mode in which you want to open the file. The mode can be "r" for reading, "w" for writing, "a" for appending, or "x" for creating a new file.
Here's an example of opening a file in read mode:
file = open("data.txt", "r")
Once you have finished working with a file, it is important to close it. Closing a file ensures that any changes made to the file are saved and that system resources are freed up. To close a file, you can use the close()
method:
file.close()
Reading from Files
Python provides several methods for reading data from a file. The most common method is the read()
method, which reads the entire contents of a file as a string. Here's an example:
file = open("data.txt", "r")
content = file.read()
print(content)
file.close()
You can also read a file line by line using the readline()
method. This method reads a single line from the file and moves the file pointer to the next line. Here's an example:
file = open("data.txt", "r")
line1 = file.readline()
line2 = file.readline()
print(line1)
print(line2)
file.close()
If you want to read all the lines of a file into a list, you can use the readlines()
method. This method reads all the lines of a file and returns them as a list of strings. Here's an example:
file = open("data.txt", "r")
lines = file.readlines()
for line in lines:
print(line)
file.close()
Writing to Files
To write data to a file, you need to open the file in write mode ("w") or append mode ("a"). In write mode, if the file already exists, it will be overwritten. In append mode, new data will be added to the end of the file.
To write data to a file, you can use the write()
method. This method takes a string as an argument and writes it to the file. Here's an example:
file = open("data.txt", "w")
file.write("Hello, World!")
file.close()
If you want to write multiple lines to a file, you can use the writelines()
method. This method takes a list of strings as an argument and writes each string as a separate line in the file. Here's an example:
file = open("data.txt", "w")
lines = ["Line 1\n", "Line 2\n", "Line 3\n"]
file.writelines(lines)
file.close()
File Position and Seek
When you read or write data from a file, Python keeps track of the current position in the file. This position is called the file pointer. By default, the file pointer is at the beginning of the file.
You can use the seek()
method to change the position of the file pointer. The seek()
method takes two parameters: the offset and the reference point. The offset specifies the number of bytes to move, and the reference point determines the starting position for the offset. The reference point can be 0 (beginning of the file), 1 (current position), or 2 (end of the file).
Here's an example of using the seek()
method to move the file pointer to the beginning of the file:
file = open("data.txt", "r")
file.seek(0, 0)
content = file.read()
print(content)
file.close()
File Handling with Context Managers
Python provides a convenient way to handle files using context managers. A context manager automatically takes care of opening and closing the file, ensuring that the file is properly closed even if an exception occurs.
To use a context manager for file handling, you can use the with
statement. Here's an example:
with open("data.txt", "r") as file:
content = file.read()
print(content)
In this example, the file is automatically closed when the with
block is exited, whether by reaching the end of the block or by an exception being raised.
Using a context manager for file handling is considered a best practice in Python, as it helps prevent resource leaks and makes the code more readable.
Conclusion
File operations are an essential part of working with data in Python. In this section, you learned how to open and close files, read data from files, write data to files, and manipulate the file pointer. You also discovered the benefits of using context managers for file handling. With this knowledge, you can confidently work with files and manage information in your Python programs.
Subscribe to my newsletter
Read articles from ESlavin1808 directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
