Day2/TWSBashBlazeChallenge-File exploration and backup using a bash script
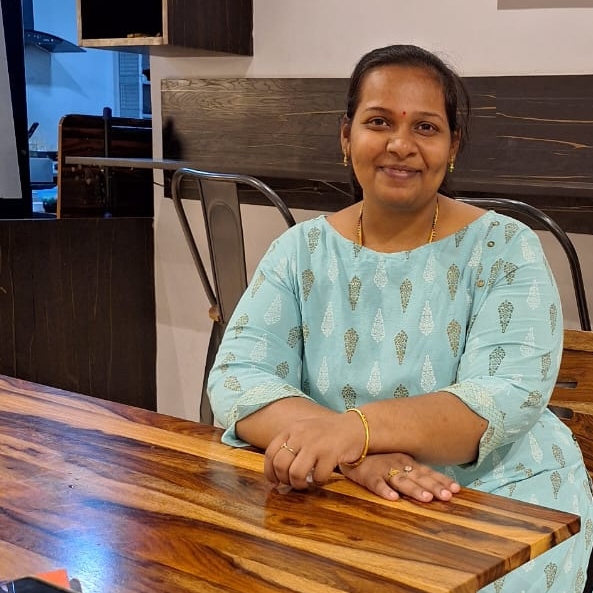
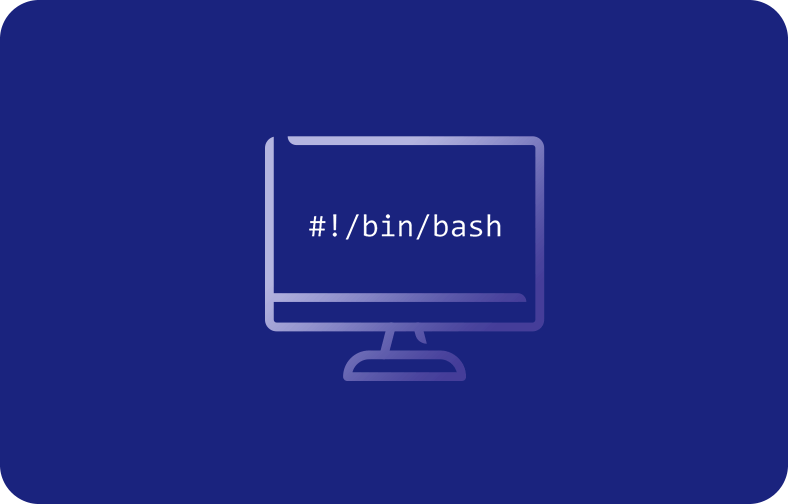
It's Day 2 ๐ of the #TWSBashBlazeChallenge, and we've got two amazing tasks lined up for today! First up, we have file and directory exploration, and then we'll back up our directories. Let's get started! ๐
Task 1: File and directory exploration
For the first task, our script needs to display a welcome message on the screen, followed by the contents of the current directory along with their sizes in a human-readable format. Let's take a look at the solution script to address the given problem statement.
#!/bin/bash
# Description: #TWSBashBlazeChallenge Day-2
# Task 1: Interactive File and Directory Explorer
# print a welcome message for the user
echo Welcome to the Interactive File and Directory Explorer!
# Format and display files and directories
# with their size in human readable format
echo " current path - $PWD"
echo "size of file/directory" :
ls -lh | sed 1d | awk '{print "- ",$NF,"\t","(", "$1" , $5,")"}'
# Infinite loop to continiously take user input
while true;
do
# prompt user to enter a text
echo -n "Enter a line of text (Press Enter without text to exit)"
read input
# use -z option to test for empty string
if [[ -z $input ]];
then
echo Goodbye!
exit
else
# bash built-in string length
input_length=${#input}
# print the character count on screen
echo "Character count $input_length"
fi
done
Task 2: Directory backup with rotation
For the second task, we need to create a Bash script that accepts a directory path as a command-line argument and performs a backup of the directory. The script should generate timestamped backup folders and copy all the files from the specified directory into the backup folder.
Additionally, the script should implement a rotation mechanism to maintain only the last three backups. This means that if there are more than three backup folders, the oldest backup folders should be removed to ensure only the most recent backups are retained.
#!/bin/bash
# Check if a directory path is provided as a command-line argument
# checks if the number of command-line arguments is not equal to 1
if [ $# -ne 1 ]
then
echo "Usage: $0 <directory_path>"
exit 1
fi
# Validate if the provided argument is a directory
backup_source="$1"
# The -d test operator checks if the given path exists and is a directory
if [ ! -d "$backup_source" ]
then
echo "Error: The provided path is not a valid directory."
exit 1
fi
# Create a timestamp for the backup folder
backup_time=$(date +"%Y-%m-%d_%H-%M-%S")
backup_folder="${backup_source}/backup_${backup_time}"
# Perform the backup
# rsync is a powerful file copying tool
rsync -Rr "$backup_source" "$backup_folder" || {echo "Error: Backup failed." exit 1}
# Perform rotation to keep only the last 3 backups
# here we list the folders in reverse chronological order and remove the older backups
ls -dt "${backup_source}/backup_"* | tail -n +4 | xargs rm -rf
# Provide feedback to the user
echo "Backup created: $backup_folder."
Happy Learning!!!๐โจ
Subscribe to my newsletter
Read articles from Siri Chandana directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
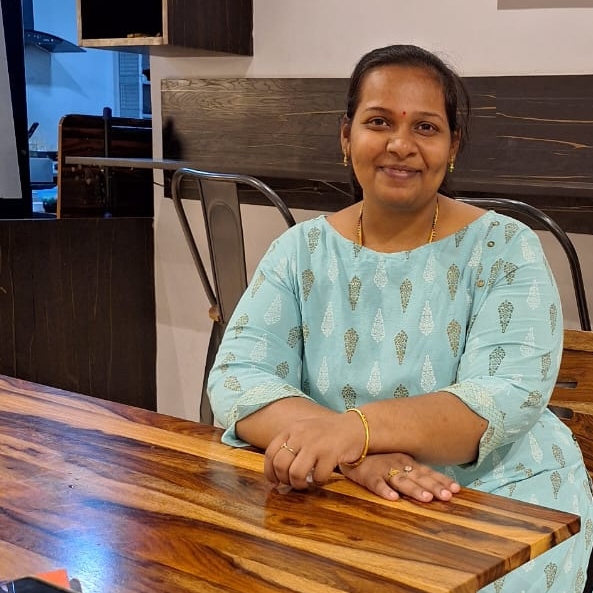