7.3 Debugging Techniques

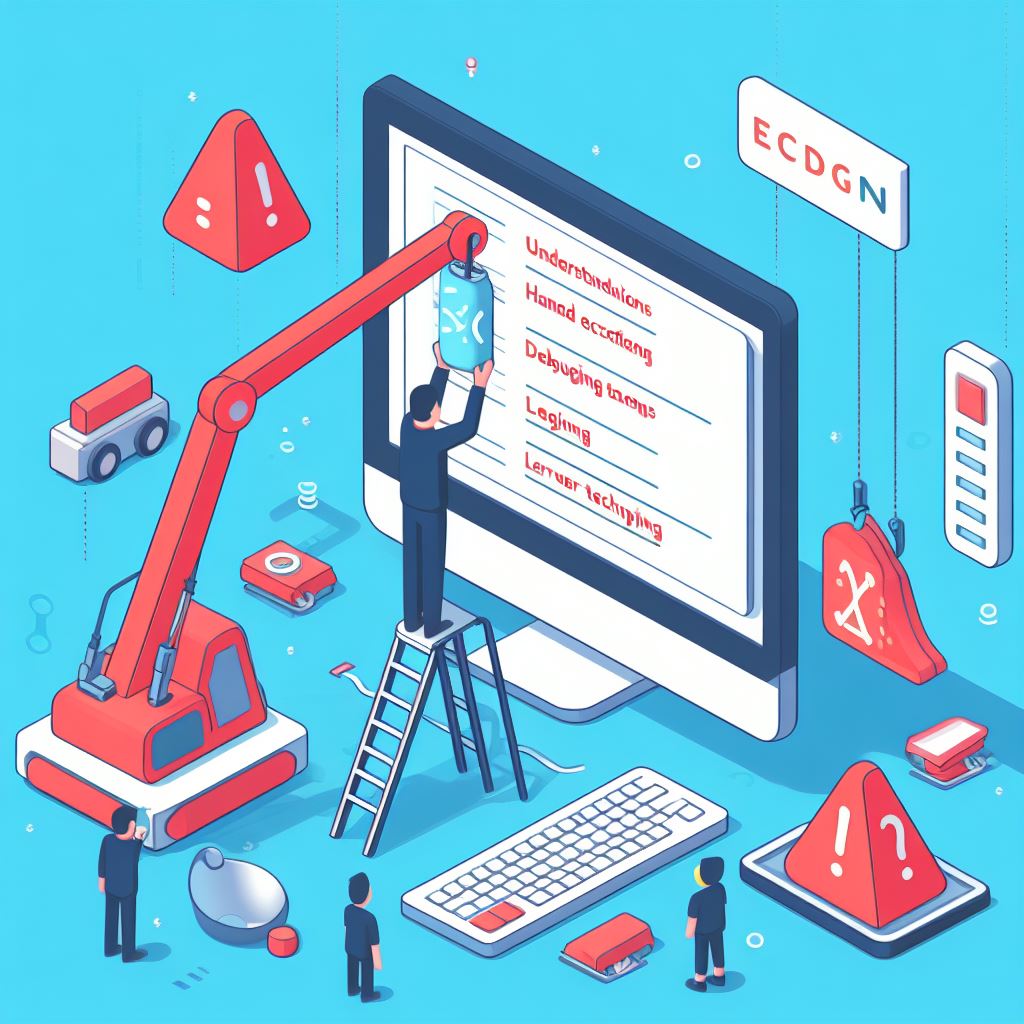
Debugging is an essential skill for any programmer. It involves identifying and fixing errors or bugs in your code. Python provides several tools and techniques to help you debug your programs effectively. In this section, we will explore some of the most commonly used debugging techniques in Python.
1. Print Statements
One of the simplest and most effective ways to debug your code is by using print statements. By strategically placing print statements at different points in your code, you can track the flow of execution and identify any unexpected behavior. Print statements allow you to display the values of variables, function outputs, or any other information that can help you understand what is happening in your program.
For example, if you are trying to debug a function that is not returning the expected output, you can insert print statements inside the function to display the intermediate values of variables and check if they match your expectations.
2. Using the Python Debugger (pdb)
Python comes with a built-in debugger called pdb (Python Debugger). The pdb module provides a set of commands that allow you to step through your code, set breakpoints, and inspect variables at runtime. To use the pdb debugger, you need to import the pdb module and insert the pdb.set_trace()
statement at the point where you want to start debugging.
Once the debugger is activated, you can use various commands to navigate through your code. Some of the commonly used pdb commands include:
n
(next): Execute the next line of code.s
(step): Step into a function call.c
(continue): Continue execution until the next breakpoint or the end of the program.p
(print): Print the value of a variable.q
(quit): Quit the debugger.
The pdb debugger is a powerful tool for debugging complex programs and understanding the flow of execution. It allows you to interactively explore your code and identify the source of errors.
3. Using Assertions
Assertions are statements that check if a given condition is true. They are often used to verify assumptions about the state of your program. By adding assertions to your code, you can catch potential errors early and ensure that your program is behaving as expected.
To use assertions for debugging, you can insert them at critical points in your code to validate the values of variables or the correctness of certain operations. If an assertion fails, Python will raise an AssertionError and provide information about the failed condition.
For example, if you have a function that calculates the square root of a number, you can add an assertion to check if the input is non-negative:
def square_root(x):
assert x >= 0, "Input must be non-negative"
# Calculate square root
...
If the input is negative, the assertion will fail, and an AssertionError will be raised, indicating that there is a problem with the input.
4. Using Logging
Logging is a technique that allows you to record information about the execution of your program. Python provides a built-in logging module that makes it easy to add logging statements to your code. Logging is particularly useful for debugging complex programs or for monitoring the behavior of a program in production.
To use logging, you need to import the logging module and configure the logging system. You can then insert logging statements at different points in your code to record relevant information. The logging module provides different levels of logging, such as DEBUG, INFO, WARNING, ERROR, and CRITICAL, allowing you to control the verbosity of your logs.
By using logging instead of print statements, you can have more control over the output and easily enable or disable logging statements depending on your needs. Additionally, logging allows you to write log messages to different destinations, such as a file or the console.
5. Using a Debugger GUI
In addition to the command-line debugger (pdb), there are also graphical user interface (GUI) debuggers available for Python. These debuggers provide a visual interface that allows you to step through your code, set breakpoints, and inspect variables.
Some popular Python GUI debuggers include PyCharm, Visual Studio Code, and PyDev. These IDEs (Integrated Development Environments) provide a comprehensive set of debugging tools, including variable inspection, call stack visualization, and interactive debugging.
Using a debugger GUI can be particularly helpful when dealing with large codebases or complex programs. The visual interface allows you to navigate through your code more easily and provides a more intuitive debugging experience.
In conclusion, debugging is an essential skill for any programmer, and Python provides several techniques and tools to help you debug your code effectively. By using print statements, the Python debugger (pdb), assertions, logging, or a debugger GUI, you can identify and fix errors in your code, ensuring that your programs run smoothly and as expected.
Subscribe to my newsletter
Read articles from ESlavin1808 directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
