8 Advanced Python Concepts

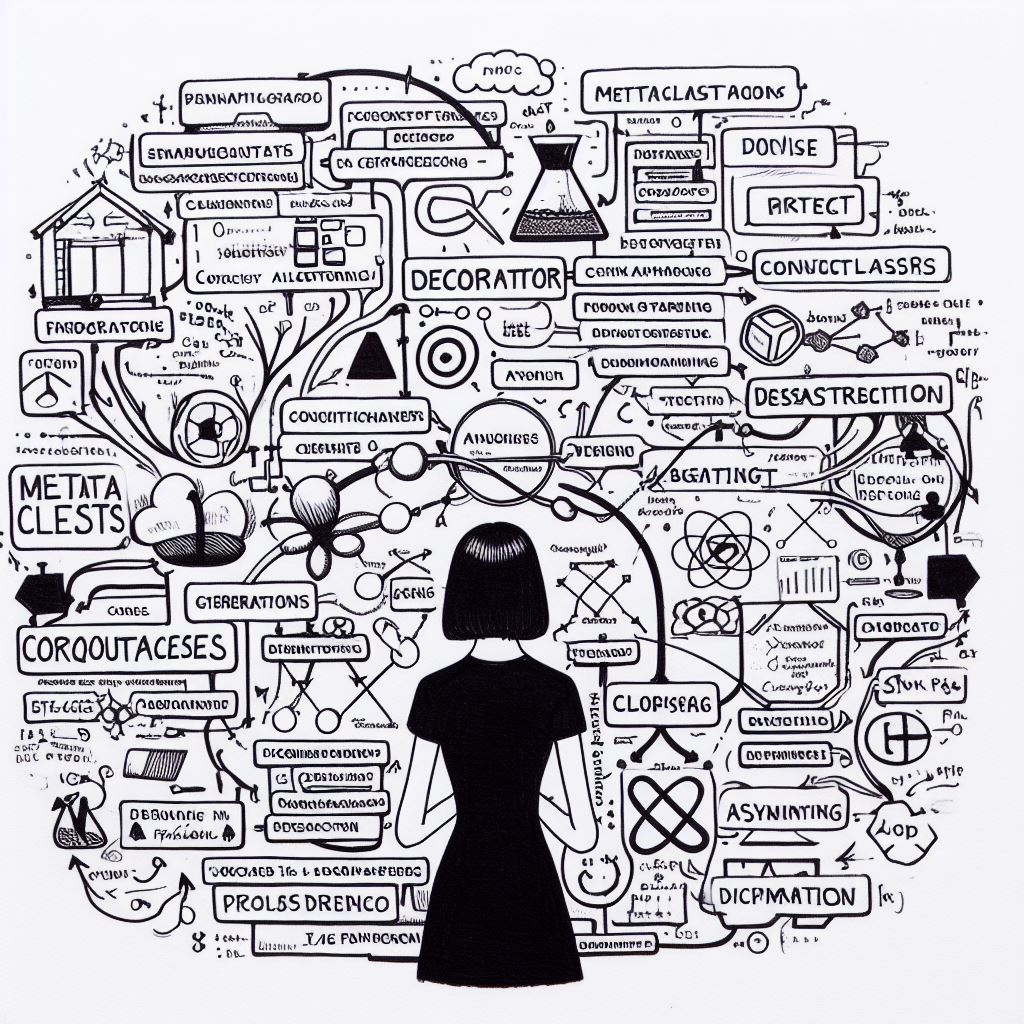
8.1 Generators and Iterators
In Python, generators and iterators are powerful concepts that allow you to work with large amounts of data efficiently and effectively. They provide a way to generate and iterate over a sequence of values without storing them all in memory at once. This can be particularly useful when dealing with large datasets or when you need to generate an infinite sequence of values.
Understanding Iterators
An iterator is an object that implements the iterator protocol, which consists of two methods: __iter__()
and __next__()
. The __iter__()
method returns the iterator object itself, and the __next__()
method returns the next value from the iterator. If there are no more items to return, it should raise the StopIteration
exception.
Let's take a look at an example of an iterator in Python:
class MyIterator:
def __init__(self, limit):
self.limit = limit
self.current = 0
def __iter__(self):
return self
def __next__(self):
if self.current < self.limit:
value = self.current
self.current += 1
return value
else:
raise StopIteration
# Using the iterator
my_iterator = MyIterator(5)
for item in my_iterator:
print(item)
In this example, we define a custom iterator MyIterator
that generates values from 0 to the specified limit. The __iter__()
method returns the iterator object itself, and the __next__()
method generates the next value in the sequence. When there are no more values to generate, it raises the StopIteration
exception.
Introducing Generators
Generators are a special type of iterator that simplifies the process of creating iterators. They are defined using a special syntax that includes the yield
keyword. When a generator function is called, it returns a generator object that can be iterated over.
Let's see an example of a generator function in Python:
def my_generator(limit):
current = 0
while current < limit:
yield current
current += 1
# Using the generator
my_generator_obj = my_generator(5)
for item in my_generator_obj:
print(item)
In this example, we define a generator function my_generator
that generates values from 0 to the specified limit. Instead of using the __iter__()
and __next__()
methods, we use the yield
keyword to yield the next value in the sequence. The function is paused and resumed each time a value is yielded.
Advantages of Generators and Iterators
Generators and iterators offer several advantages over traditional approaches to working with sequences of values:
Memory Efficiency: Generators and iterators allow you to work with large datasets or infinite sequences without loading all the values into memory at once. This can significantly reduce memory usage and improve performance.
Lazy Evaluation: Generators and iterators use lazy evaluation, which means that values are generated or computed only when they are needed. This can be particularly useful when working with computationally expensive operations or when dealing with infinite sequences.
Code Simplicity: Generators and iterators simplify the code by separating the logic for generating values from the logic for consuming them. This can make the code more readable, maintainable, and modular.
Time Efficiency: Generators and iterators can save time by generating values on the fly, rather than precomputing and storing them. This can be especially beneficial when working with large datasets or when the values are expensive to compute.
Built-in Generator Functions and Iterators
Python provides several built-in generator functions and iterators that make it easier to work with sequences of values. Some of the most commonly used ones include:
range()
: Therange()
function is a built-in generator function that generates a sequence of numbers within a specified range.enumerate()
: Theenumerate()
function is a built-in generator function that generates pairs of values consisting of an index and an item from an iterable.zip()
: Thezip()
function is a built-in generator function that generates pairs of values by combining corresponding elements from multiple iterables.map()
: Themap()
function is a built-in generator function that applies a given function to each item of an iterable and generates the results.filter()
: Thefilter()
function is a built-in generator function that filters an iterable based on a given condition and generates the filtered values.
These built-in generator functions and iterators provide powerful tools for working with sequences of values in a concise and efficient manner.
Conclusion
Generators and iterators are powerful concepts in Python that allow you to work with sequences of values efficiently and effectively. They provide a way to generate and iterate over values without storing them all in memory at once. Generators simplify the process of creating iterators by using the yield
keyword, while iterators require the implementation of the __iter__()
and __next__()
methods. By using generators and iterators, you can improve memory efficiency, enable lazy evaluation, simplify code, and save time. Python also provides several built-in generator functions and iterators that make it easier to work with sequences of values.
8.2 Decorators
Decorators are a powerful feature in Python that allow you to modify the behavior of functions or classes without changing their source code. They provide a way to add functionality to existing code by wrapping it with additional code. Decorators are implemented using the concept of higher-order functions, which are functions that take other functions as arguments or return functions as results.
Understanding Decorators
In Python, a decorator is a special type of function that takes a function as input and returns a modified version of that function. The modified function can then be used in place of the original function. Decorators are typically used to add functionality such as logging, timing, or authentication to functions without modifying their code directly.
To define a decorator, you simply define a function that takes a function as an argument and returns a new function. The new function can then be used to replace the original function. Here's a simple example of a decorator that adds logging functionality to a function:
def log_decorator(func):
def wrapper(*args, **kwargs):
print(f"Calling function: {func.__name__}")
result = func(*args, **kwargs)
print(f"Function {func.__name__} finished execution")
return result
return wrapper
@log_decorator
def add_numbers(a, b):
return a + b
result = add_numbers(5, 10)
print(result)
In this example, the log_decorator
function takes a function func
as an argument and defines a new function wrapper
that wraps the original function. The wrapper
function adds logging statements before and after calling the original function. The @log_decorator
syntax is used to apply the decorator to the add_numbers
function.
When the add_numbers
function is called, the decorator is automatically applied, and the logging statements are executed before and after the function's execution. This allows you to easily add logging functionality to any function by simply applying the @log_decorator
decorator.
Common Use Cases for Decorators
Decorators can be used in a variety of scenarios to enhance the functionality of functions or classes. Here are some common use cases for decorators:
Logging
Decorators can be used to add logging functionality to functions or methods. By wrapping a function with a logging decorator, you can automatically log information about the function's execution, such as its arguments and return value. This can be useful for debugging or monitoring purposes.
Timing
Decorators can be used to measure the execution time of functions or methods. By wrapping a function with a timing decorator, you can automatically record the time it takes for the function to execute. This can be useful for performance optimization or profiling.
Authentication
Decorators can be used to enforce authentication or authorization checks on functions or methods. By wrapping a function with an authentication decorator, you can automatically check if the user has the necessary permissions to execute the function. This can be useful for securing sensitive operations in an application.
Caching
Decorators can be used to implement caching functionality for expensive or time-consuming operations. By wrapping a function with a caching decorator, you can automatically cache the results of the function and return the cached result if the same inputs are provided again. This can be useful for improving the performance of repetitive operations.
Creating Custom Decorators
In addition to using built-in decorators or third-party decorators, you can also create your own custom decorators in Python. Creating a custom decorator allows you to define your own functionality and apply it to functions or classes as needed.
To create a custom decorator, you follow the same pattern as defining a decorator function. You define a function that takes a function as an argument and returns a new function. Inside the new function, you can add your custom functionality before or after calling the original function.
Here's an example of a custom decorator that adds a prefix to the output of a function:
def prefix_decorator(prefix):
def decorator(func):
def wrapper(*args, **kwargs):
result = func(*args, **kwargs)
return f"{prefix} {result}"
return wrapper
return decorator
@prefix_decorator("Result:")
def add_numbers(a, b):
return a + b
result = add_numbers(5, 10)
print(result)
In this example, the prefix_decorator
function takes a prefix string as an argument and returns a decorator function. The decorator function takes a function func
as an argument and returns a wrapper function. The wrapper function adds the prefix to the result of calling the original function.
The @prefix_decorator("Result:")
syntax is used to apply the custom decorator to the add_numbers
function. When the add_numbers
function is called, the decorator is automatically applied, and the result is prefixed with the specified string.
Conclusion
Decorators are a powerful feature in Python that allow you to modify the behavior of functions or classes without changing their source code. They provide a way to add functionality to existing code by wrapping it with additional code. Decorators are implemented using the concept of higher-order functions and can be used in a variety of scenarios, such as logging, timing, authentication, and caching. You can use built-in decorators, third-party decorators, or create your own custom decorators to enhance the functionality of your Python code.
8.3 Concurrency and Multithreading
Concurrency and multithreading are powerful concepts in Python that allow you to execute multiple tasks simultaneously. In this section, we will explore how to leverage these concepts to improve the performance and efficiency of your Python programs.
Understanding Concurrency
Concurrency refers to the ability of a program to execute multiple tasks concurrently. It allows different parts of a program to make progress independently, without waiting for each other. In Python, concurrency can be achieved through various techniques such as multiprocessing, multithreading, and asynchronous programming.
Multithreading in Python
Multithreading is a technique that allows multiple threads to run concurrently within a single process. Each thread represents an independent flow of execution, and they share the same memory space. This means that threads can access and modify the same variables and data structures.
Python provides a built-in module called threading
that allows you to work with threads. You can create a new thread by subclassing the Thread
class and overriding the run()
method. Here's an example:
import threading
class MyThread(threading.Thread):
def run(self):
# Code to be executed in the thread
print("Hello from a thread!")
# Create an instance of the custom thread class
my_thread = MyThread()
# Start the thread
my_thread.start()
# Wait for the thread to finish
my_thread.join()
In this example, we create a new thread by subclassing the Thread
class and overriding the run()
method. The run()
method contains the code that will be executed in the thread. We then create an instance of our custom thread class and start it using the start()
method. Finally, we use the join()
method to wait for the thread to finish its execution.
Thread Synchronization
When multiple threads access and modify shared data, it can lead to race conditions and data inconsistencies. To prevent this, Python provides synchronization primitives such as locks, semaphores, and condition variables.
A lock is a simple synchronization primitive that allows only one thread to access a shared resource at a time. You can use the Lock
class from the threading
module to create a lock. Here's an example:
import threading
# Create a lock
lock = threading.Lock()
# Acquire the lock
lock.acquire()
# Code to be executed while the lock is held
# Release the lock
lock.release()
In this example, we create a lock using the Lock
class and acquire it using the acquire()
method. The code between the acquire()
and release()
calls will be executed while the lock is held. Once the code is executed, we release the lock using the release()
method.
Global Interpreter Lock (GIL)
Python has a Global Interpreter Lock (GIL) that ensures only one thread executes Python bytecode at a time. This means that even though you can create multiple threads in Python, they won't run in parallel on multiple CPU cores. Instead, they will take turns executing on a single CPU core.
The GIL is a mechanism designed to simplify the implementation of the CPython interpreter (the reference implementation of Python). While the GIL can limit the performance of CPU-bound multithreaded programs, it doesn't affect I/O-bound programs as much. This is because the GIL is released when a thread performs I/O operations, allowing other threads to run.
Multiprocessing in Python
If you need to perform CPU-bound tasks in parallel, you can use the multiprocessing
module in Python. Unlike multithreading, multiprocessing allows you to bypass the GIL and take advantage of multiple CPU cores.
The multiprocessing
module provides a Process
class that allows you to create and manage processes. Each process has its own memory space, which means that they don't share variables and data structures by default. To share data between processes, you can use techniques such as shared memory and message passing.
Here's an example of using the multiprocessing
module to execute a function in parallel:
import multiprocessing
def square(x):
return x ** 2
# Create a pool of processes
pool = multiprocessing.Pool()
# Apply the function to a list of inputs
results = pool.map(square, [1, 2, 3, 4, 5])
# Print the results
print(results)
In this example, we define a function square()
that calculates the square of a number. We then create a pool of processes using the Pool
class from the multiprocessing
module. The map()
method applies the square()
function to a list of inputs in parallel, and the results are stored in the results
variable.
Asynchronous Programming
Asynchronous programming is another technique for achieving concurrency in Python. It allows you to write non-blocking code that can perform multiple tasks concurrently without waiting for each other.
Python provides the asyncio
module for asynchronous programming. It introduces the async
and await
keywords, which allow you to define asynchronous functions and await the completion of asynchronous tasks.
Here's an example of using the asyncio
module to perform asynchronous I/O operations:
import asyncio
async def fetch_data(url):
# Code to fetch data from a URL
...
async def main():
# Create a list of tasks
tasks = [
fetch_data("https://example.com"),
fetch_data("https://google.com"),
fetch_data("https://python.org")
]
# Wait for all tasks to complete
await asyncio.gather(*tasks)
# Run the main function
asyncio.run(main())
In this example, we define an asynchronous function fetch_data()
that fetches data from a URL. We then create a list of tasks, each representing a call to the fetch_data()
function with a different URL. The gather()
function waits for all tasks to complete before continuing.
Conclusion
Concurrency and multithreading are powerful concepts in Python that allow you to improve the performance and efficiency of your programs. Whether you choose to use multithreading, multiprocessing, or asynchronous programming depends on the nature of your tasks and the specific requirements of your application. By understanding and leveraging these concepts, you can unlock the full potential of Python and build high-performance applications.
8.4 Regular Expressions
Regular expressions are a powerful tool for pattern matching and text manipulation in Python. They allow you to search, extract, and manipulate text based on specific patterns. Regular expressions are widely used in various domains, including web development, data processing, and text mining. In this section, we will explore the basics of regular expressions and how to use them effectively in Python.
What are Regular Expressions?
A regular expression, also known as regex, is a sequence of characters that defines a search pattern. It consists of a combination of literal characters and special characters called metacharacters. Metacharacters have special meanings and are used to define the rules for pattern matching.
Regular expressions provide a flexible and concise way to search for specific patterns in text. They can be used to match strings that follow a certain format, such as email addresses, phone numbers, or URLs. Regular expressions can also be used to extract specific parts of a string or replace certain patterns with new text.
Creating Regular Expressions in Python
In Python, regular expressions are supported through the re
module. Before using regular expressions, you need to import the re
module into your Python script or interactive session. You can do this by using the following import statement:
import re
Once the re
module is imported, you can start using regular expressions in your code.
Basic Regular Expression Patterns
Regular expressions consist of various metacharacters and special sequences that define the search pattern. Here are some of the basic metacharacters and special sequences commonly used in regular expressions:
.
(dot): Matches any character except a newline.^
(caret): Matches the start of a string.$
(dollar): Matches the end of a string.*
(asterisk): Matches zero or more occurrences of the preceding character or group.+
(plus): Matches one or more occurrences of the preceding character or group.?
(question mark): Matches zero or one occurrence of the preceding character or group.\
(backslash): Escapes special characters or indicates special sequences.[]
(square brackets): Matches any single character within the brackets.()
(parentheses): Groups multiple characters or expressions together.
These are just a few examples of the metacharacters and special sequences available in regular expressions. The re
module provides many more options and functionalities for pattern matching.
Using Regular Expressions in Python
To use regular expressions in Python, you need to compile the pattern using the re.compile()
function. This function takes the regular expression pattern as a string and returns a pattern object that can be used for matching.
Here's an example of how to compile a regular expression pattern:
import re
pattern = re.compile(r'abc')
In this example, the pattern object is created to match the string 'abc'. The r
before the string indicates a raw string, which is used to avoid any unwanted escape characters.
Once the pattern object is created, you can use various methods provided by the re
module to perform pattern matching operations. Some of the commonly used methods include:
match()
: Determines if the pattern matches at the beginning of the string.search()
: Searches the string for a match to the pattern.findall()
: Returns all non-overlapping matches of the pattern in the string.finditer()
: Returns an iterator yielding match objects for all matches of the pattern in the string.
Here's an example of how to use the search()
method to find a pattern in a string:
import re
pattern = re.compile(r'world')
text = 'Hello, world!'
match = pattern.search(text)
if match:
print('Pattern found!')
else:
print('Pattern not found.')
In this example, the search()
method is used to search for the pattern 'world' in the string 'Hello, world!'. If a match is found, the program prints 'Pattern found!'; otherwise, it prints 'Pattern not found.'.
Advanced Regular Expression Techniques
Regular expressions offer a wide range of advanced techniques for more complex pattern matching. Some of these techniques include:
Quantifiers: Allow you to specify the number of occurrences of a character or group.
Character classes: Define a set of characters to match.
Anchors: Specify the position of a match within a string.
Grouping and capturing: Group parts of a pattern and capture the matched text.
Lookahead and lookbehind: Specify conditions for a match without including the matched text in the result.
These advanced techniques provide more control and flexibility in pattern matching. They can be used to handle complex scenarios and extract specific information from text.
Conclusion
Regular expressions are a powerful tool for pattern matching and text manipulation in Python. They allow you to search, extract, and manipulate text based on specific patterns. In this section, we explored the basics of regular expressions and how to use them effectively in Python. We learned about the different metacharacters and special sequences used in regular expressions, as well as the methods provided by the re
module for pattern matching. We also briefly touched on some advanced techniques for more complex pattern matching. Regular expressions are a valuable skill to have in your Python toolkit, and mastering them will greatly enhance your ability to work with text data.
Subscribe to my newsletter
Read articles from ESlavin1808 directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
