8.1 Generators and Iterators

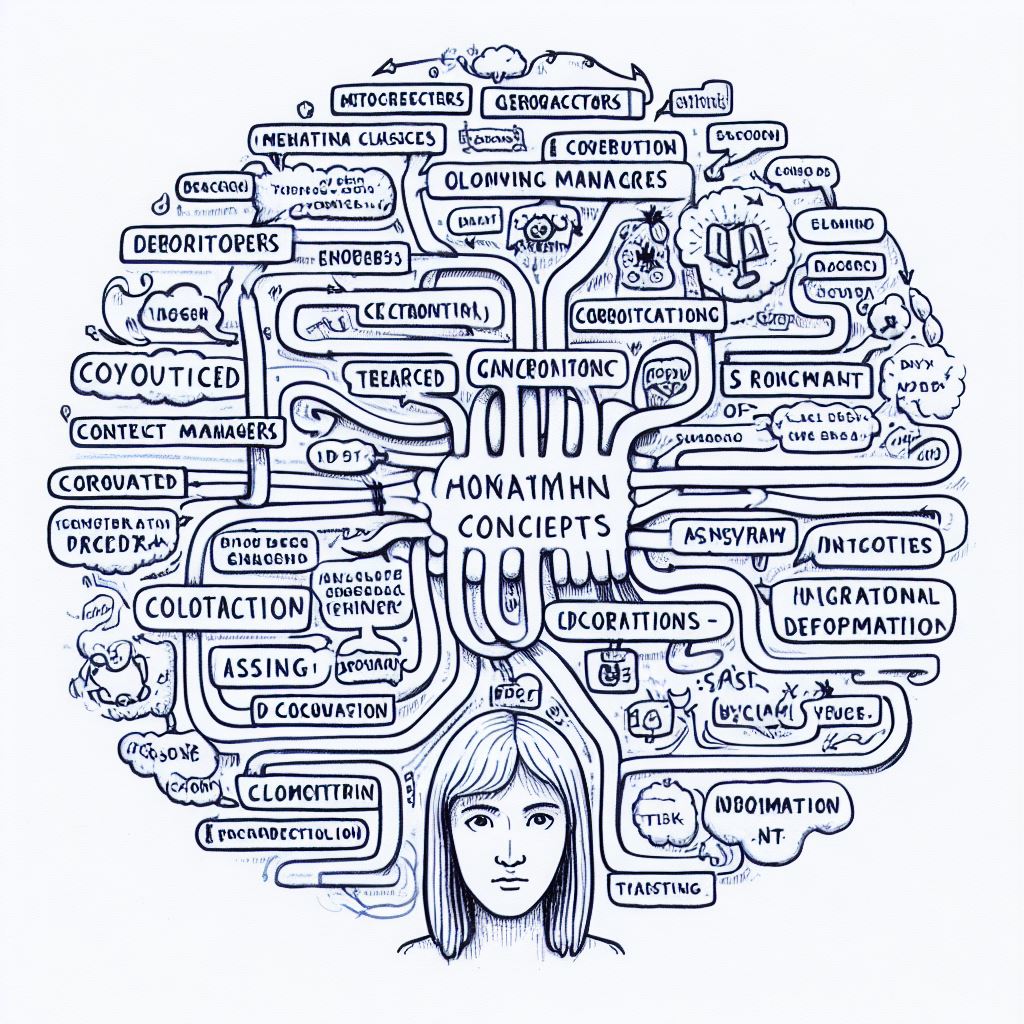
In Python, generators and iterators are powerful concepts that allow you to work with large amounts of data efficiently and effectively. They provide a way to generate and iterate over a sequence of values without storing them all in memory at once. This can be particularly useful when dealing with large datasets or when you need to generate an infinite sequence of values.
Understanding Iterators
An iterator is an object that implements the iterator protocol, which consists of two methods: __iter__()
and __next__()
. The __iter__()
method returns the iterator object itself, and the __next__()
method returns the next value from the iterator. If there are no more items to return, it should raise the StopIteration
exception.
Let's take a look at an example of an iterator in Python:
class MyIterator:
def __init__(self, limit):
self.limit = limit
self.current = 0
def __iter__(self):
return self
def __next__(self):
if self.current < self.limit:
value = self.current
self.current += 1
return value
else:
raise StopIteration
# Using the iterator
my_iterator = MyIterator(5)
for item in my_iterator:
print(item)
In this example, we define a custom iterator MyIterator
that generates values from 0 to the specified limit. The __iter__()
method returns the iterator object itself, and the __next__()
method generates the next value in the sequence. When there are no more values to generate, it raises the StopIteration
exception.
Introducing Generators
Generators are a special type of iterator that simplifies the process of creating iterators. They are defined using a special syntax that includes the yield
keyword. When a generator function is called, it returns a generator object that can be iterated over.
Let's see an example of a generator function in Python:
def my_generator(limit):
current = 0
while current < limit:
yield current
current += 1
# Using the generator
my_generator_obj = my_generator(5)
for item in my_generator_obj:
print(item)
In this example, we define a generator function my_generator
that generates values from 0 to the specified limit. Instead of using the __iter__()
and __next__()
methods, we use the yield
keyword to yield the next value in the sequence. The function is paused and resumed each time a value is yielded.
Advantages of Generators and Iterators
Generators and iterators offer several advantages over traditional approaches to working with sequences of values:
Memory Efficiency: Generators and iterators allow you to work with large datasets or infinite sequences without loading all the values into memory at once. This can significantly reduce memory usage and improve performance.
Lazy Evaluation: Generators and iterators use lazy evaluation, which means that values are generated or computed only when they are needed. This can be particularly useful when working with computationally expensive operations or when dealing with infinite sequences.
Code Simplicity: Generators and iterators simplify the code by separating the logic for generating values from the logic for consuming them. This can make the code more readable, maintainable, and modular.
Time Efficiency: Generators and iterators can save time by generating values on the fly, rather than precomputing and storing them. This can be especially beneficial when working with large datasets or when the values are expensive to compute.
Built-in Generator Functions and Iterators
Python provides several built-in generator functions and iterators that make it easier to work with sequences of values. Some of the most commonly used ones include:
range()
: Therange()
function is a built-in generator function that generates a sequence of numbers within a specified range.enumerate()
: Theenumerate()
function is a built-in generator function that generates pairs of values consisting of an index and an item from an iterable.zip()
: Thezip()
function is a built-in generator function that generates pairs of values by combining corresponding elements from multiple iterables.map()
: Themap()
function is a built-in generator function that applies a given function to each item of an iterable and generates the results.filter()
: Thefilter()
function is a built-in generator function that filters an iterable based on a given condition and generates the filtered values.
These built-in generator functions and iterators provide powerful tools for working with sequences of values in a concise and efficient manner.
Conclusion
Generators and iterators are powerful concepts in Python that allow you to work with sequences of values efficiently and effectively. They provide a way to generate and iterate over values without storing them all in memory at once. Generators simplify the process of creating iterators by using the yield
keyword, while iterators require the implementation of the __iter__()
and __next__()
methods. By using generators and iterators, you can improve memory efficiency, enable lazy evaluation, simplify code, and save time. Python also provides several built-in generator functions and iterators that make it easier to work with sequences of values.
Subscribe to my newsletter
Read articles from ESlavin1808 directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
