8.2 Decorators

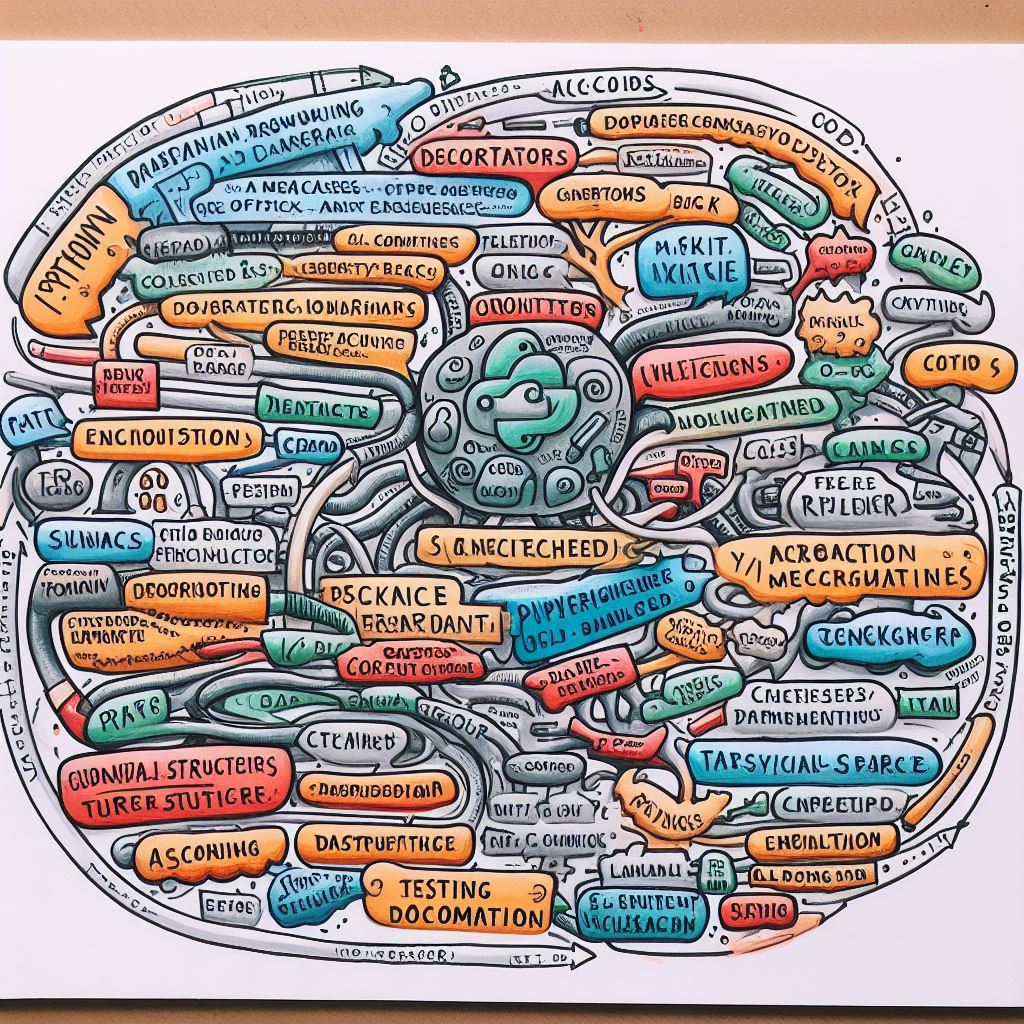
Decorators are a powerful feature in Python that allow you to modify the behavior of functions or classes without changing their source code. They provide a way to add functionality to existing code by wrapping it with additional code. Decorators are implemented using the concept of higher-order functions, which are functions that take other functions as arguments or return functions as results.
Understanding Decorators
In Python, a decorator is a special type of function that takes a function as input and returns a modified version of that function. The modified function can then be used in place of the original function. Decorators are typically used to add functionality such as logging, timing, or authentication to functions without modifying their code directly.
To define a decorator, you simply define a function that takes a function as an argument and returns a new function. The new function can then be used to replace the original function. Here's a simple example of a decorator that adds logging functionality to a function:
def log_decorator(func):
def wrapper(*args, **kwargs):
print(f"Calling function: {func.__name__}")
result = func(*args, **kwargs)
print(f"Function {func.__name__} finished execution")
return result
return wrapper
@log_decorator
def add_numbers(a, b):
return a + b
result = add_numbers(5, 10)
print(result)
In this example, the log_decorator
function takes a function func
as an argument and defines a new function wrapper
that wraps the original function. The wrapper
function adds logging statements before and after calling the original function. The @log_decorator
syntax is used to apply the decorator to the add_numbers
function.
When the add_numbers
function is called, the decorator is automatically applied, and the logging statements are executed before and after the function's execution. This allows you to easily add logging functionality to any function by simply applying the @log_decorator
decorator.
Common Use Cases for Decorators
Decorators can be used in a variety of scenarios to enhance the functionality of functions or classes. Here are some common use cases for decorators:
Logging
Decorators can be used to add logging functionality to functions or methods. By wrapping a function with a logging decorator, you can automatically log information about the function's execution, such as its arguments and return value. This can be useful for debugging or monitoring purposes.
Timing
Decorators can be used to measure the execution time of functions or methods. By wrapping a function with a timing decorator, you can automatically record the time it takes for the function to execute. This can be useful for performance optimization or profiling.
Authentication
Decorators can be used to enforce authentication or authorization checks on functions or methods. By wrapping a function with an authentication decorator, you can automatically check if the user has the necessary permissions to execute the function. This can be useful for securing sensitive operations in an application.
Caching
Decorators can be used to implement caching functionality for expensive or time-consuming operations. By wrapping a function with a caching decorator, you can automatically cache the results of the function and return the cached result if the same inputs are provided again. This can be useful for improving the performance of repetitive operations.
Creating Custom Decorators
In addition to using built-in decorators or third-party decorators, you can also create your own custom decorators in Python. Creating a custom decorator allows you to define your own functionality and apply it to functions or classes as needed.
To create a custom decorator, you follow the same pattern as defining a decorator function. You define a function that takes a function as an argument and returns a new function. Inside the new function, you can add your custom functionality before or after calling the original function.
Here's an example of a custom decorator that adds a prefix to the output of a function:
def prefix_decorator(prefix):
def decorator(func):
def wrapper(*args, **kwargs):
result = func(*args, **kwargs)
return f"{prefix} {result}"
return wrapper
return decorator
@prefix_decorator("Result:")
def add_numbers(a, b):
return a + b
result = add_numbers(5, 10)
print(result)
In this example, the prefix_decorator
function takes a prefix string as an argument and returns a decorator function. The decorator function takes a function func
as an argument and returns a wrapper function. The wrapper function adds the prefix to the result of calling the original function.
The @prefix_decorator("Result:")
syntax is used to apply the custom decorator to the add_numbers
function. When the add_numbers
function is called, the decorator is automatically applied, and the result is prefixed with the specified string.
Conclusion
Decorators are a powerful feature in Python that allow you to modify the behavior of functions or classes without changing their source code. They provide a way to add functionality to existing code by wrapping it with additional code. Decorators are implemented using the concept of higher-order functions and can be used in a variety of scenarios, such as logging, timing, authentication, and caching. You can use built-in decorators, third-party decorators, or create your own custom decorators to enhance the functionality of your Python code.
Subscribe to my newsletter
Read articles from ESlavin1808 directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
