Demystifying JavaScript Variables: var, let, and const
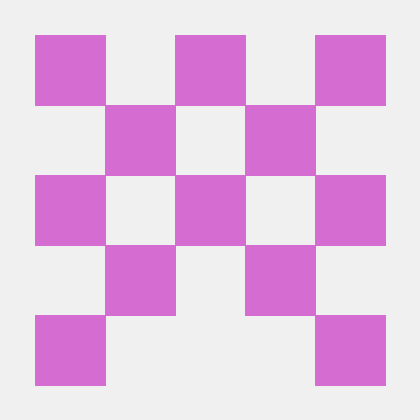
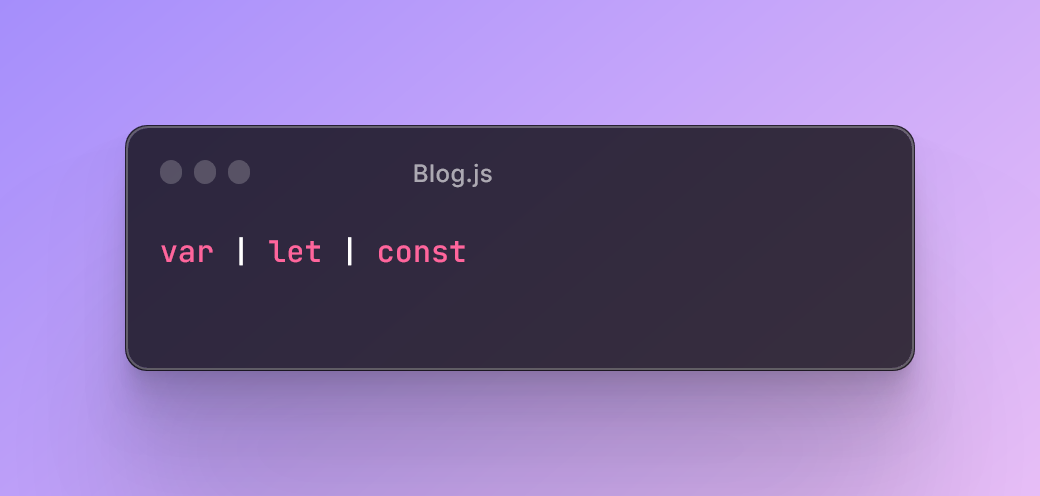
Today we will try to understand the various concepts related to var
, let
and const
we first need to understand variable scope in javascript. In the world of Javascript, variables have boundaries called 'scope'.Think of scope as the area where a variable is known and can be used in your code. It's like a spotlight that shines on your variable, but only in certain places.
Variable scope
A scope is a certain region of a program where a defined variable exists and can be recognized and beyond that it cannot be recognized.
There are two main types of scope in javascript global scope and local scope(block scope or functional scope). Let's try to understand this with an example code.
// Global Scope
let globalVar = "I'm global!";
function exampleFunction() {
// Local Scope
let localVar = "I'm local!";
console.log(globalVar); // Accessing the global variable from within the function
console.log(localVar); // Accessing the local variable from within the function
}
exampleFunction();
console.log(globalVar); // Accessing the global variable from outside the function
//console.log(localVar); // This would result in an error since localVar is not accessible outside the function
Variable shadowing occurs in JavaScript when a variable declared in a local scope has the same name as a variable declared in an outer scope. When this happens, the inner variable "shadows" or takes precedence over the outer variable within its scope. This can lead to unexpected behavior, as the inner variable temporarily hides the outer one.
Here's an example to illustrate variable shadowing:
let outerVar = "I'm outer!"; // This is in the outer/global scope
function shadowingExample() {
let outerVar = "I'm shadowed!"; // This is in the local scope of the function
console.log(outerVar); // This will access the inner variable
}
shadowingExample();
console.log(outerVar); // This will access the outer/global variable
In this example:
There are two variables with the name
outerVar
. One is in the global scope, and the other is in the local scope of theshadowingExample
function.Inside the
shadowingExample
function, when we useconsole.log(outerVar)
, it accesses the innerouterVar
variable within the local scope of the function. This is called variable shadowing because it temporarily "shadows" or hides the outer/globalouterVar
variable within that function's scope.Outside the function, when we use
console.log(outerVar)
, it accesses the outer/globalouterVar
variable because the inner variable is no longer in scope.
Variable shadowing
In javascript after the introduction of let and cost in ES6 along block scoping allows shadowing. So let's try to understand shadowing with an example.
// Global Scope
let globalVar = "I'm global!";
function exampleFunction() {
// Local Scope
let localVar = "I'm local!";
console.log(globalVar); // Accessing the global variable from within the function
console.log(localVar); // Accessing the local variable from within the function
}
exampleFunction();
console.log(globalVar); // Accessing the global variable from outside the function
//console.log(localVar); // This would result in an error since localVar is not accessible outside the function
Variable shadowing occurs in JavaScript when a variable declared in a local scope has the same name as a variable declared in an outer scope. When this happens, the inner variable "shadows" or takes precedence over the outer variable within its scope. This can lead to unexpected behavior, as the inner variable temporarily hides the outer one.
While shadowing a variable it should not cross the boundary of scope i.e. you can shadow var
variable using let but you cannot do the opposite.
Hoisting
Now let's look at Hoisting. Hoisting is a JavaScript behavior where variable and function declarations are moved to the top of their containing scope during the compilation phase. This means that you can use variables and functions before they are declared in your code but with some caveats. However, only the declarations are hoisted, not the initializations.
Here's an example to illustrate hoisting with variables:
console.log(x); // Output: undefine
var x = 10;
console.log(x); // Output: 10
In this example var x
is hoisted to the top of the current scope during compilation, so console.log(x)
is allowed before the actual declaration. However, it's assigned the value undefined until the actual assignment of x = 10
is encountered. When you later assign x = 10
, it updates the value of x
, and the second console.log(x)
prints 10.
Temporal Deadzone(TDZ)
The Temporal Dead Zone is a specific behavior that occurs when using let
and const
declarations. In this zone, variables are considered declared but cannot be accessed until their actual declaration statement is encountered in the code. Attempting to access a variable in the TDZ results in a ReferenceError.
Here's an example to illustrate the Temporal Dead Zone:
console.log(x); // Throws a ReferenceError
let x = 10;
console.log(x); // Output: 10
In this example:
The first
console.log(x)
attempts to accessx
before its declaration. This triggers a ReferenceError becausex
is in the Temporal Dead Zone.After the declaration
let x = 10;
,x
is no longer in the TDZ, and it can be accessed and used normally.
To summarize, hoisting moves variable and function declarations to the top of their scope, allowing you to use them before their actual declaration but with initial values of undefined
. The Temporal Dead Zone is a concept specific to let
and const
declarations, where variables are considered declared but inaccessible until their declaration statement is encountered, helping catch certain types of errors in your code.
Mastering JavaScript variables, scope, hoisting, temporal dead zones, and variable shadowing empowers you to write clean, bug-free code. With a solid grasp of these fundamentals, you're well-equipped to navigate the complexities of JavaScript and build efficient, error-free applications. Keep coding and exploring, and you'll continue to enhance your JavaScript skills!
Thanks for reading my blog, I hope it was helpful. Do share it if you find this useful. That's it in this, blog hope to see you in the next blog ๐.
Subscribe to my newsletter
Read articles from Sohan Shetty directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
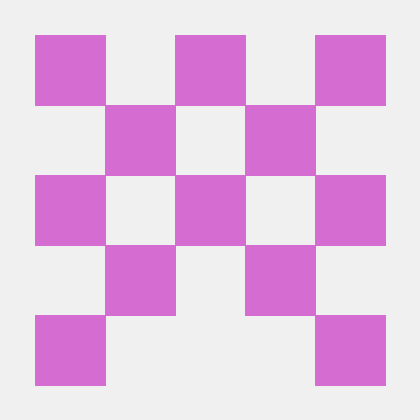