Understanding Controlled & Uncontrolled React Forms
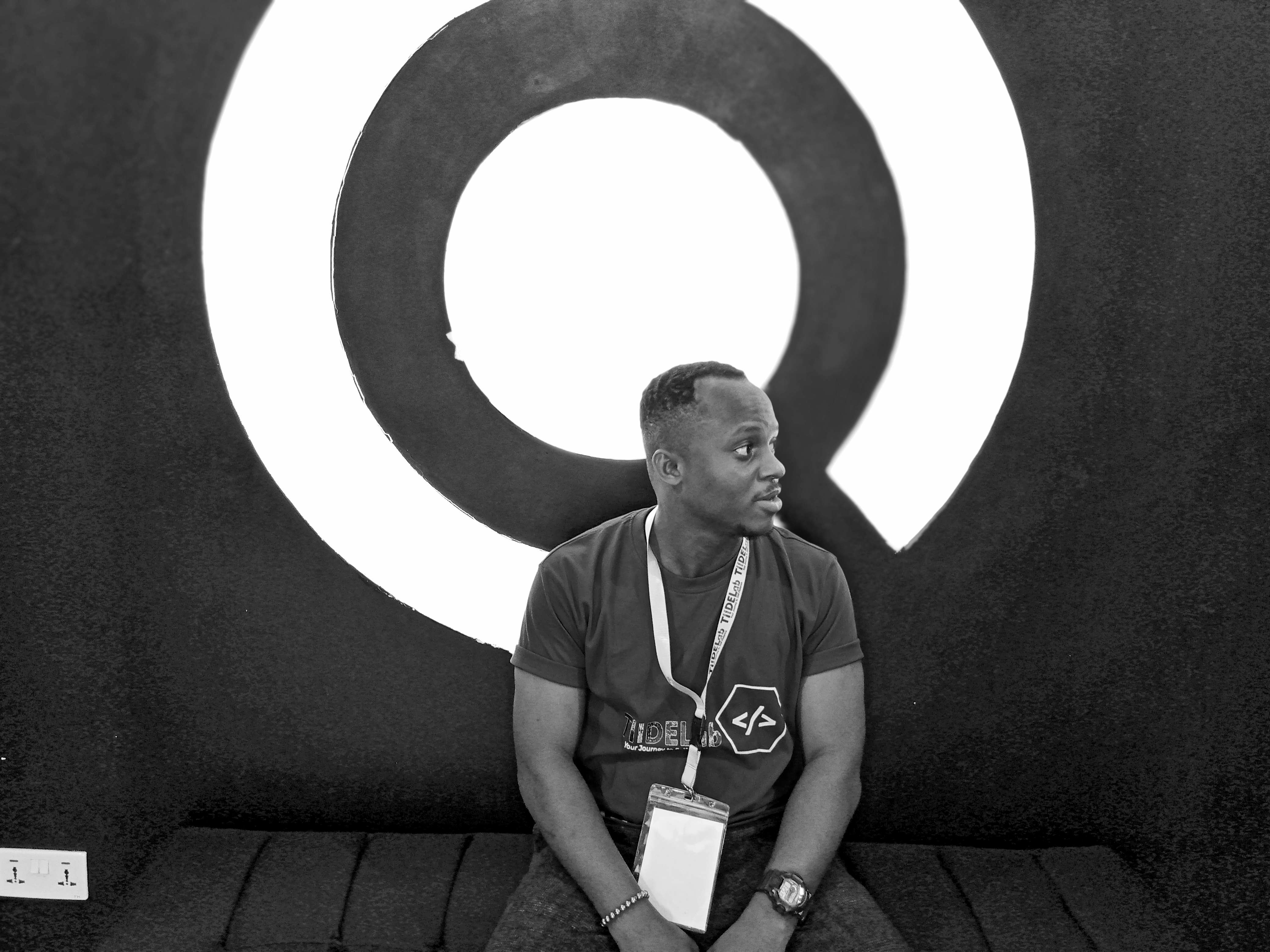
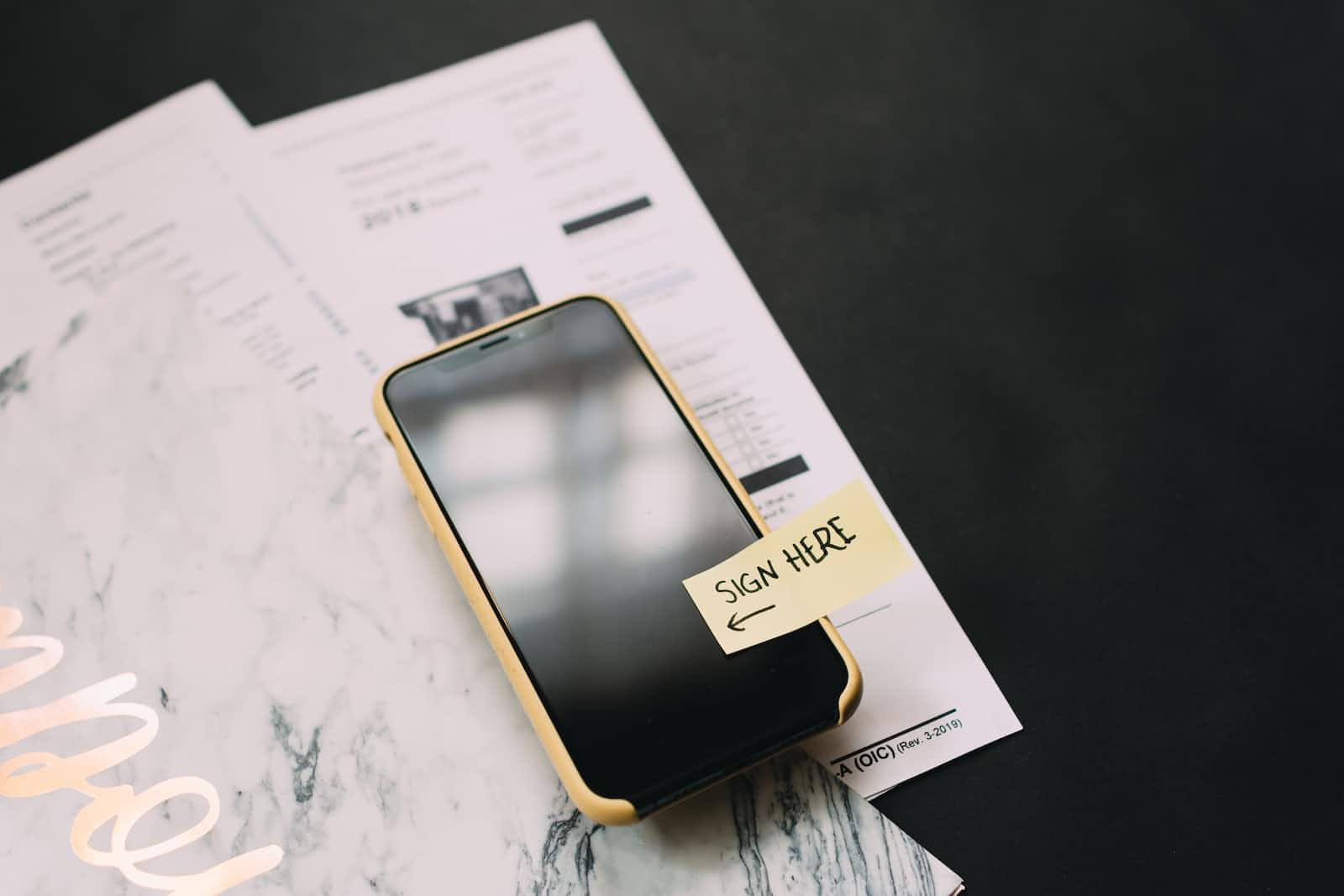
When it comes to working with forms in React, developers are faced with the choice between controlled components and uncontrolled components. Each approach has its own set of advantages and use cases. In this article, we will explore the differences between controlled and uncontrolled form components, their respective benefits, and when to choose one over the other.
Introduction
React, a popular JavaScript library for building user interfaces, provides two primary strategies for handling form components: controlled and uncontrolled. Controlled components follow a more structured approach by managing form input values through React state, while uncontrolled components allow the DOM to manage input values directly. Let's delve into each approach to understand their implications and applicability.
Uncontrolled Inputs
Uncontrolled inputs in React closely resemble standard HTML form inputs. They maintain their state directly within the DOM, and you can access their values using React refs. Here's a simple example of an uncontrolled input in a form:
const Form = () => {
const inputRef = useRef(null);
const handleSubmit = () => {
const inputValue = inputRef.current.value;
// Do something with the value
};
return (
<form onSubmit={handleSubmit}>
<input ref={inputRef} type="text" />
{/* ... */}
</form>
);
};
In this approach, the input's value isn't managed by React state. Instead, you retrieve the value from the DOM element using a ref when needed. Uncontrolled components are suitable for straightforward forms with minimal UI feedback requirements. They are quick to implement but lack some of the advanced features that controlled components offer.
Controlled Inputs
Controlled inputs, on the other hand, are managed through React state. The component that renders the input holds the input value in its state and updates it through event handlers. This approach enables tighter control over form behavior and facilitates real-time UI updates. Here's an example of a controlled input:
const Form = () => {
const [value, setValue] = useState("");
const handleChange = (e) => {
setValue(e.target.value);
};
return (
<form>
<input type="text" value={value} onChange={handleChange} />
{/* ... */}
</form>
);
};
With controlled inputs, every change in the input triggers a state update, keeping the data and UI in sync. This synchronization enables various features, including instant field validation, dynamic input handling, and conditional disabling of submit buttons based on input validity.
Choosing the Right Approach
The choice between controlled and uncontrolled components depends on the complexity of your form and the desired features. Here's a quick guide:
Uncontrolled Components: Choose uncontrolled inputs when your form is simple and requires minimal UI feedback. If you need basic input handling without real-time validation or advanced features, uncontrolled components with refs can suffice.
Controlled Components: Opt for controlled inputs when you require dynamic UI updates, instant validation, and more control over form behavior. Controlled components are essential for implementing features like instant field validation, disabling submit buttons based on validity, and enforcing specific input formats.
Feature Comparison
Here's a summary of features supported by each approach:
Feature | Uncontrolled | Controlled |
One-time value retrieval (e.g., on submit) | Yes | Yes |
Validating on submit | Yes | Yes |
Instant field validation | No | Yes |
Conditionally disabling a submit button | No | Yes |
Enforcing a specific input format | No | Yes |
Several inputs for one piece of data | No | Yes |
Dynamic inputs | No | Yes |
Conclusion
Controlled and uncontrolled components offer different approaches to handling form inputs in React. Uncontrolled inputs are suitable for simple forms, while controlled inputs provide advanced features and real-time UI updates. Consider your form's complexity and feature requirements when choosing between the two approaches. By understanding the strengths of each method, you can make an informed decision and build effective, user-friendly forms in your React applications.
Subscribe to my newsletter
Read articles from George Udonte directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
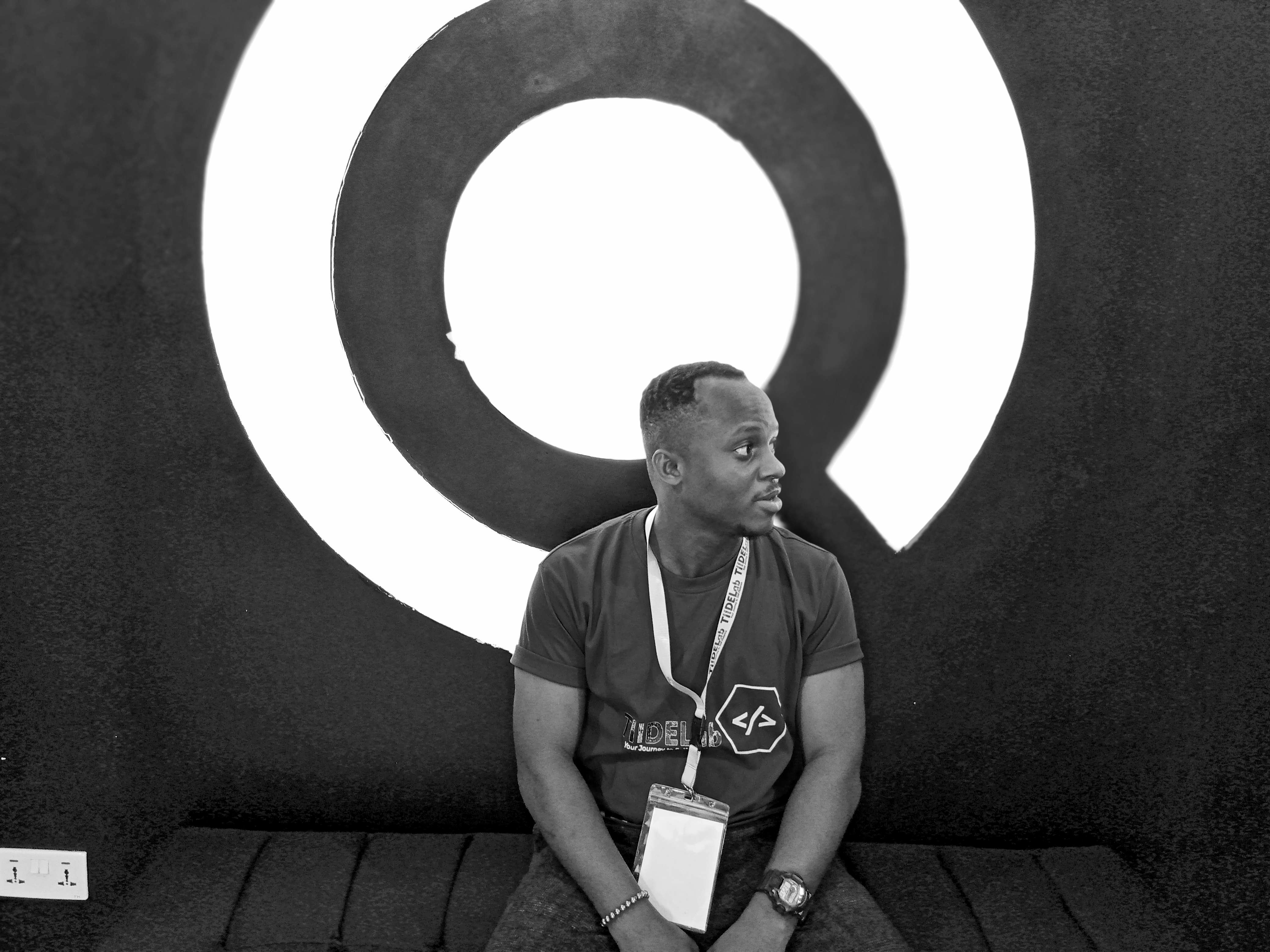