JavaScript Data Types

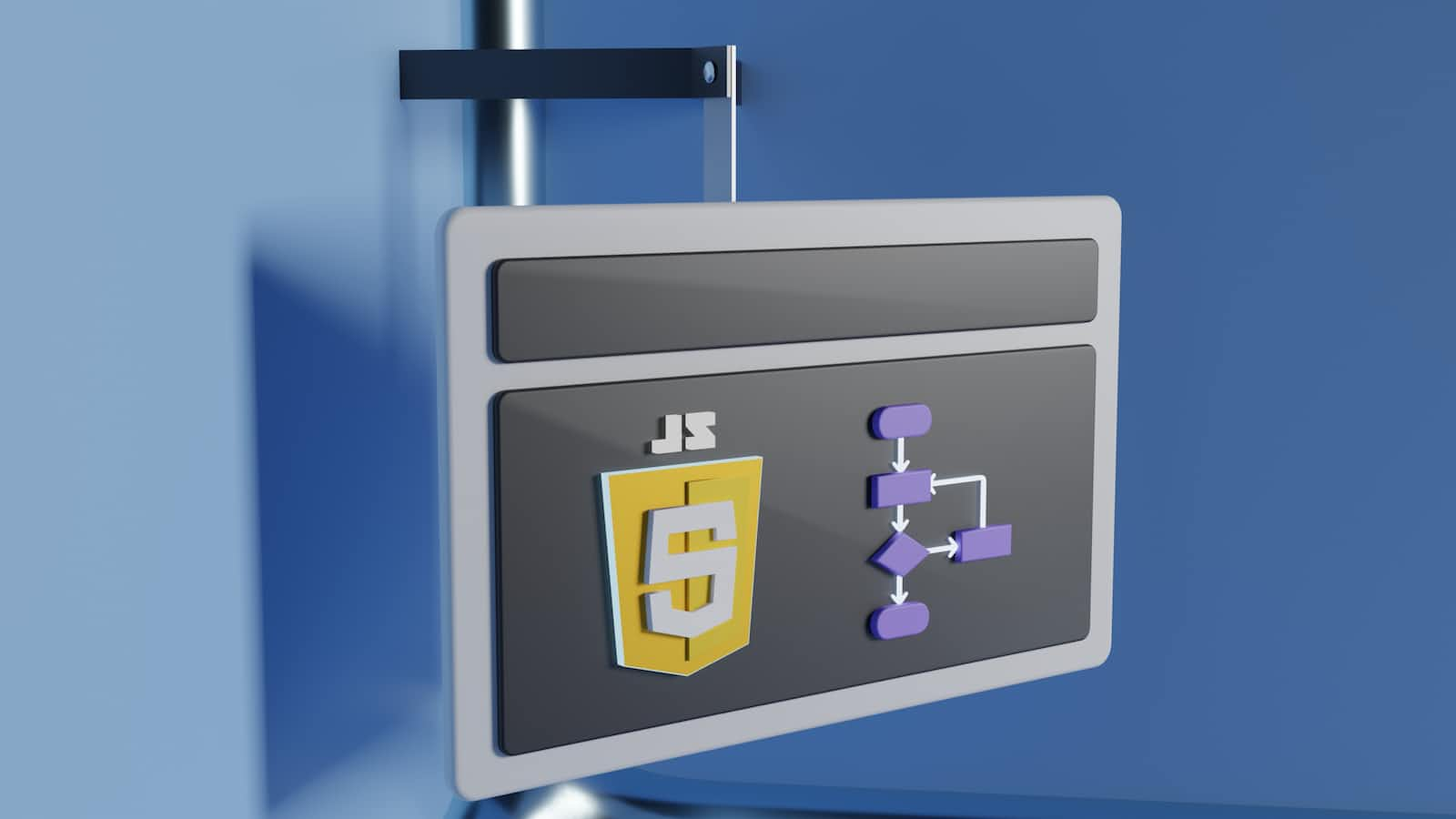
JavaScript is a high-level, dynamic, and interpreted programming language that supports a variety of data types. In this blog post, we'll take a look at the different data types supported by JavaScript and their characteristics.
Primitive Data Types
String:
A sequence of characters. Strings are immutable, meaning that once created, they cannot be modified.
let x = "Hello World!";
console.log(x); // Output: Hello World!
Strings can also be written using back-ticks (``) syntax rather than quotes. For example:
let x = `Hello World!`;
Or you can use both back-ticks and quotes.
let answer = `They often call me "Abby"`;
Template literals provide an easy way to interpolate variables and expressions into strings.
The method is called string interpolation.
The syntax is:
${...}
let firstName = "Abby";
let lastName = "Much";
let text = `Welcome ${firstName}, ${lastName}!`;
//Output: Welcome Abby, Much!
Number:
A numeric value. Numbers can be integers or floating-point numbers.
let x = 3.14;
let y = 2;
console.log(x); // Output: 3.14
console.log(y); // Output: 2
Boolean:
A logical value that can be either true or false. It is commonly used for testing.
let x = true;
let y = false;
let isMarried = false;
console.log(x); // Output: true
console.log(y); // Output: false
console.log(isMarried) // Output : false
console.log(x == y); // Output : false
console.log(x != y); // Output : true
Null and Undefined:
These are special values that represent the absence of any object or property. Null is used to indicate that a variable or object has been intentionally set to nothing, while Undefined is used to indicate that a variable or object has not been initialized or is outside the scope of the current function.
let x = null;
console.log(x); // Output: null
let x;
console.log(x); // Output: undefined
Object Data Types:
Objects:
These are collections of key-value pairs. Objects can be created using the {} syntax, and keys must be strings.
const person = {
firstName: "Abigirl",
lastName: "Muchineripi",
age: 30,
eyeColor: "brown"
};
Properties can be accessed using dot notation or bracket notation. Here’s an example of accessing the lastName
property of the person
object using both notations:
person.lastName; // returns "Muchineripi"
person["lastName"]; // returns "muchineripi"
const person = {
firstName: "John",
lastName: "Doe",
age: 50,
eyeColor: "blue",
fullName: function() {
return this.firstName + " " + this.lastName;
}
};
person.fullName();// returns John Doe
Objects can have methods which are functions and can be stored properties.
Arrays:
These are ordered collections of values. Arrays are created using the [] syntax, and values can be of any data type.
let myArray = [1, 2, 3, 4, 5];
console.log(myArray); // Output: [1, 2, 3, 4, 5]
Date Object:
To get the current date you can write the below code snippet and run it using the browser. JavaScript will by default use your browser's day, time and time zone.
const date = new Date();
console.log(date); //Output:Mon Oct 02 2023 12:58:49 GMT+0200 (South Africa Standard Time)
Built-in Data Types:
Function:
A built-in data type that represents a function. Functions can be assigned to variables and called just like any other value.
function myFunction(x, y) {
return x + y;
}
console.log(myFunction(2, 3)); // Output: 5
Symbol:
A built-in data type that represents a unique identifier. Symbols are immutable and can be used to create unique keys for objects.
let mySymbol = Symbol("myUniqueIdentifier");
console.log(mySymbol); // Output: Symbol(myUniqueIdentifier)
BigInt:
All JavaScript numbers are stored in a 64-bit floating-point format.
JavaScript BigInt is a new datatype (ES2020) that can be used to store integer values that are too big to be represented by a normal JavaScript Number.
let y = BigInt("1234567890123456789012345678901234567890");
I hope this was super easy to grasp. Happy coding!
Subscribe to my newsletter
Read articles from Abigirl Muchineripi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Abigirl Muchineripi
Abigirl Muchineripi
I am embracing my nerdy side by starting to work on the foundations of web development: HTML, CSS, Figma, and JavaScript.