Understanding the Bag-of-Words (BoW) Model in Natural Language Processing (NLP) and Text Analysis
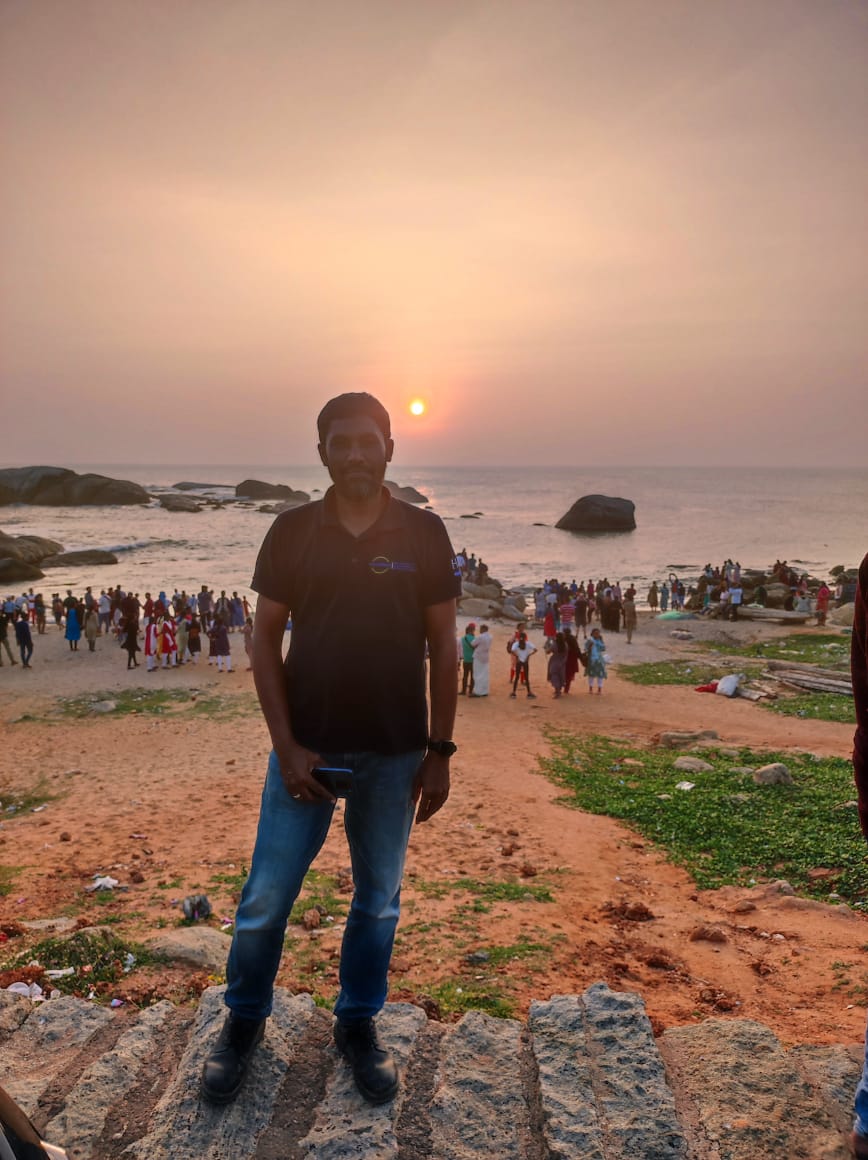
The Bag-of-Words (BoW) model is a fundamental technique in natural language processing (NLP) for converting text data into a numerical format. It represents a document as an unordered set of words, disregarding grammar and word order while emphasizing word frequency. The process begins with tokenization, breaking the text into individual words. A vocabulary is then constructed from all unique words across the dataset. Each document is transformed into a vector, with each element corresponding to the count of a word from the vocabulary. This results in a high-dimensional, sparse representation where the order of words is ignored. While BoW simplifies complex textual data and allows for efficient computation, it does not capture semantic relationships or context.
Here's a step-by-step explanation of how the BoW model works:
Tokenization: The first step is to break down the text into individual words or tokens. This process is known as tokenization. For example, the sentence "The cat is on the mat" would be tokenized into the set of words: {"The", "cat", "is", "on", "the", "mat"}.
Vocabulary Construction: Next, a vocabulary is created by compiling a list of all unique words (tokens) across all documents in the dataset. This vocabulary represents the features or dimensions of the BoW model. For our example sentence, the vocabulary might be: {"The", "cat", "is", "on", "the", "mat"}.
Word Frequency Representation: Each document is then represented as a vector based on the word frequencies in the vocabulary. The length of the vector is equal to the size of the vocabulary, and each element represents the count of the corresponding word in the document.
Document
"The cat is on the mat"
BoW Vector
[1, 1, 1, 1, 2, 1]
In this example, the BoW vector for the sentence "The cat is on the mat" indicates that the word "The" appears once, "cat" appears once, "is" appears once, "on" appears once, "the" appears twice, and "mat" appears once.
Here's an example of implementing Bag-of-Words (BoW) using Python, specifically using the CountVectorizer
from scikit-learn:
from sklearn.feature_extraction.text import CountVectorizer
# Your input text
text = "The cat is on the mat"
# List of one document (your text)
documents = [text]
# Initialize the CountVectorizer
count_vectorizer = CountVectorizer()
# Fit and transform the documents
bow_matrix = count_vectorizer.fit_transform(documents)
# Get feature names (terms)
feature_names = count_vectorizer.get_feature_names_out()
# Convert the BoW matrix to a dense array for easier inspection
dense_array = bow_matrix.toarray()
# Create a DataFrame for better visualization
import pandas as pd
df_bow = pd.DataFrame(data=dense_array, columns=feature_names)
print(df_bow)
The BoW model has some advantages and limitations:
Advantages:
Simplicity: BoW is simple to understand and implement.
Independence: BoW treats each word independently, making it computationally efficient.
Limitations:
Loss of Word Order: BoW ignores the order of words in a document, which means it may lose important information about the structure and context of the text.
High Dimensionality: The dimensionality of the BoW representation can be large, especially for a large vocabulary.
Sparse Representation: The resulting vectors are often sparse, leading to increased memory requirements.
Despite its limitations, BoW is widely used for tasks such as text classification, sentiment analysis, and information retrieval, serving as a foundational method in the analysis of large text corpora.
Subscribe to my newsletter
Read articles from K Ahamed directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
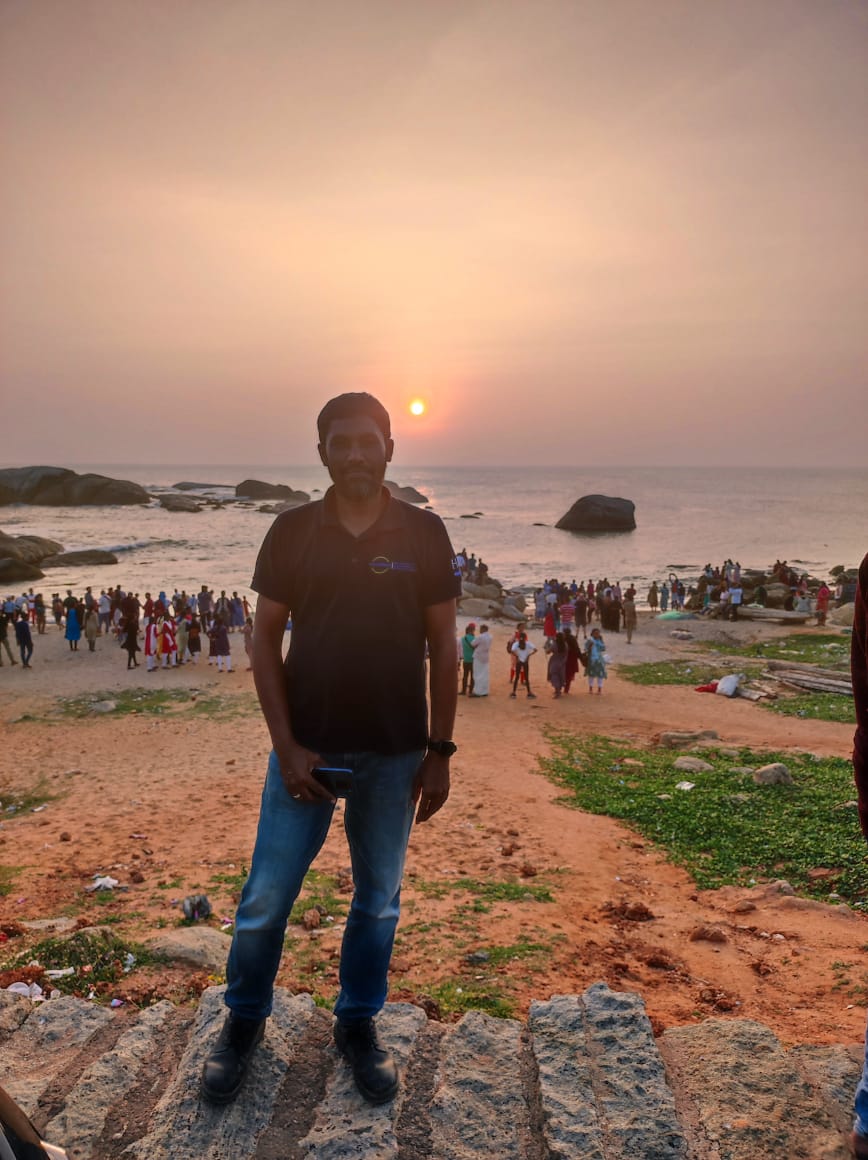
K Ahamed
K Ahamed
A skilled construction professional specializing in MEP projects. Armed with a Master's degree in Data Science, seamlessly combines hands-on expertise in construction with a passion for Python, NLP, Deep Learning, and Data Visualization. While currently at a basic level, dedicated to enhancing data skills, envisioning a future where insights derived from data reshape the landscape of construction practices. With a forward-thinking mindset, building structures but also shaping the future at the intersection of construction and data.