Pre-DSA Essentials: Pass By Value , Pass By Reference and Arrays in C++
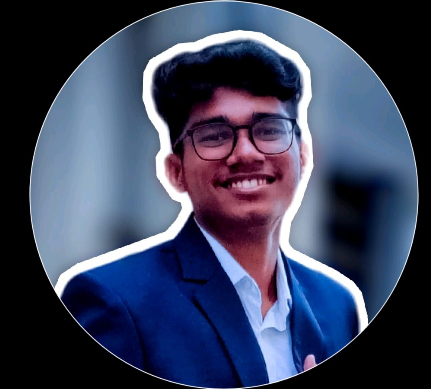
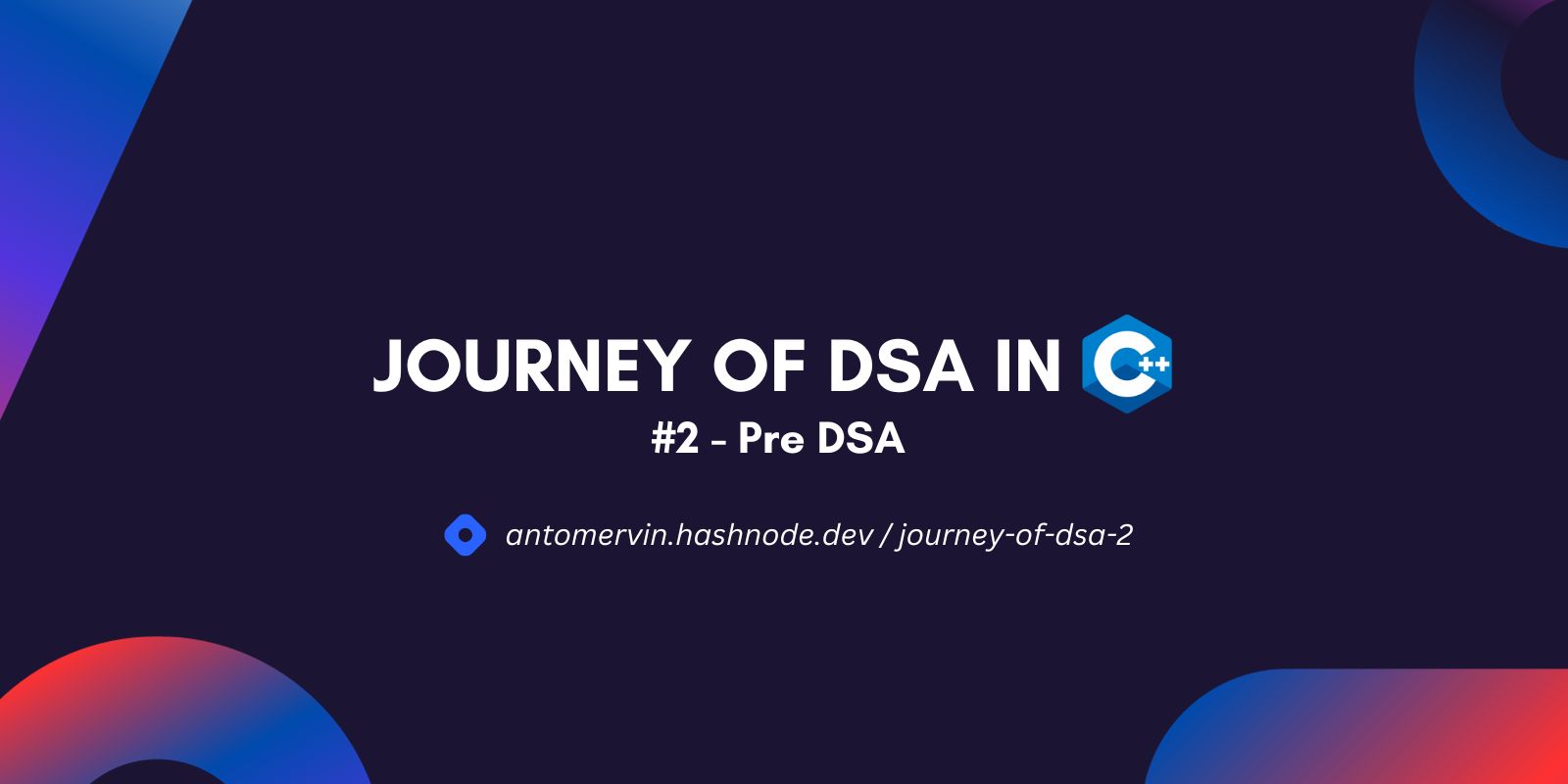
Hello ๐, Welcome back to my exciting journey of learning Data Structures and Algorithms (DSA) using C++. In today's session, which I like to call "Pre-DSA Part 2," we'll be exploring some more fundamental concepts that will lay a solid foundation for my DSA adventure.
Pass by Value Using Pointers: Simple and Straightforward
Hey not more technical! Passing by value using pointers is just what it sounds like - we're passing a copy of the value to a function. Let's break it down.
void modifyValue(int x) {
x = x * 2;
}
int main() {
int num = 5;
modifyValue(num);
// num remains 5
return 0;
}
In this example, num
remains 5 because the function modifyValue
receives a copy of num
, and any changes inside the function don't affect the original variable.
Pass by Reference Using Pointers: The Power to Change
Now, let's kick it up a notch! Pass by reference using pointers allows us to pass the memory address of a variable to a function, giving that function the power to modify the original variable.
void modifyValue(int* ptr) {
*ptr = *ptr * 2;
}
int main() {
int num = 5;
modifyValue(&num);
// num is now 10
return 0;
}
In this example, num
becomes 10 because the function modifyValue
receives a pointer to num
, and any changes to the memory location pointed to by ptr
directly affect num
.
Arrays: Managing Variables Like a Pro
Imagine you have to manage the scores of 100 students in your class. Each student has a unique score, and you need a way to keep track of all these numbers efficiently. Without the right tool, this could be a daunting task.
But fear not, for we have a powerful tool at our disposal: Arrays!
Arrays in C++ are like magical containers that allow us to store and manage multiple values of the same type under a single variable name. They bring order to chaos, making it possible to handle a vast amount of data with ease. Let's grasp the basics.
int scores[100]; // Declare an integer array with space for 5 elements
scores[0] = 95; // Assign values to array elements
scores[1] = 87;
scores[2] = 72;
scores[3] = 68;
scores[4] = 91;
.
.
.
scores[100] = 78;
Arrays are zero-indexed in C++, meaning the first element is at index 0, the second at 1, and so on. To access array elements, you use square brackets.
int secondScore = scores[4]; // Access the second element (91)
Arrays are essential for many data structures, so mastering them is a key step in our journey toward DSA expertise!
Conclusion
In this session, we've covered more ground in our Pre-DSA exploration. We've learned the difference between pass by value and pass by reference using pointers, giving us the tools to control variable modification within functions. Additionally, we've touched on arrays, which are the fundamental data structure for storing collections of data.
Our journey is just beginning, and there's so much more to learn about data structures and algorithms using C++. Stay tuned for our next session, where we'll delve deeper into this fascinating world. Happy coding, and keep the tech spirit alive! ๐โจ
Subscribe to my newsletter
Read articles from Anto Mervin directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
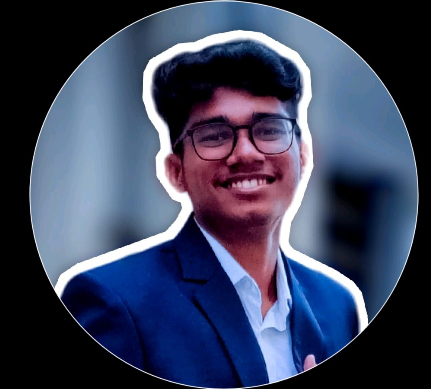
Anto Mervin
Anto Mervin
Passionate React.js Developer with 2 years of hands-on experience in building responsive web applications. Proficient in creating efficient, reusable, and scalable components using React.js. Skilled in front-end technologies such as HTML5, CSS3, and JavaScript. Experienced in integrating RESTful APIs and working with state management libraries like Redux. Strong understanding of modern web development practices and tools. Committed to delivering high-quality code and exceptional user experiences. Open to new challenges and eager to contribute to innovative projects. Let's connect and discuss how we can collaborate on exciting opportunities! #ReactJS #FrontEndDeveloper #WebDevelopment