Building an E-commerce System: A Comprehensive Code Breakdown - Part 1
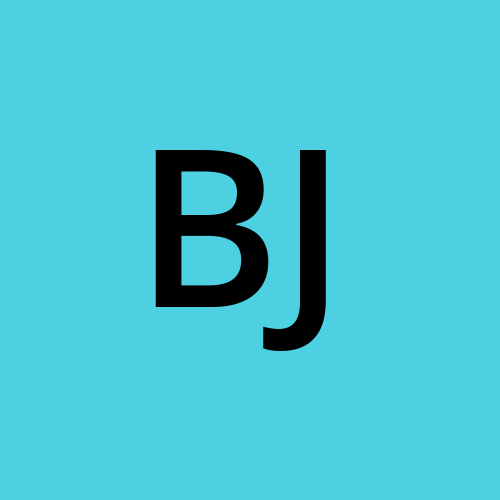
In this series, we'll take an in-depth journey through a multi-part codebase that forms the foundation of a robust e-commerce system. Each part of the code corresponds to a specific aspect of this system, ranging from product management to sales reporting. Our goal is not only to understand how the system works but also to appreciate the elegance and effectiveness of the code.
Part 1: Product Management
In this first part, we'll focus on the product management aspect of the code. An e-commerce platform relies heavily on efficient product handling, and this code snippet demonstrates how it's done. Let's delve into the code and understand how it manages products.
The Product
Class
class Product {
// ... (Constructor and other fields)
public void addItemToOrder(Product product, int quantity) {
// Check product availability and reduce quantity from inventory
if (product != null && product.getQuantity() >= quantity) {
OrderItem orderItem = new OrderItem(product, quantity);
orderItems.add(orderItem);
product.reduceQuantity(quantity); // Deduct quantity from inventory
product.updateSales(quantity); // Update product sales
}
}
// Other methods...
}
Explanation:
The
Product
class represents a product that can be sold on the e-commerce platform.The
addItemToOrder
method allows adding products to an order while considering product availability and updating inventory accordingly.When an item is added to an order, it creates an
OrderItem
, reduces the product's quantity in inventory, and updates the product's sales.
Key Takeaways:
Effective management of product availability in orders.
Real-time inventory updates to prevent overselling.
Seamless tracking of product sales.
The Product
class sets the foundation for efficient product management within the e-commerce system. It ensures that customers can order products, and the inventory stays accurate.
In the next part of this series, we'll explore the Order
class and its role in processing orders and managing the order lifecycle.
Stay tuned for Part 2, where we'll dive deeper into the order processing system of this e-commerce platform.
This completes Part 1 of our comprehensive code breakdown series.
Subscribe to my newsletter
Read articles from Bryan Samuel James directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
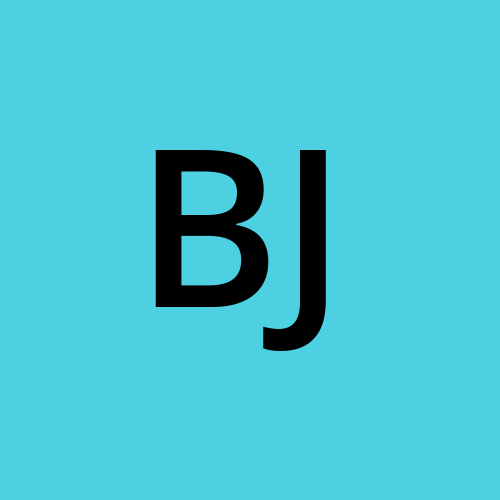