Getting Started with Flutter: A Guide for Beginners!

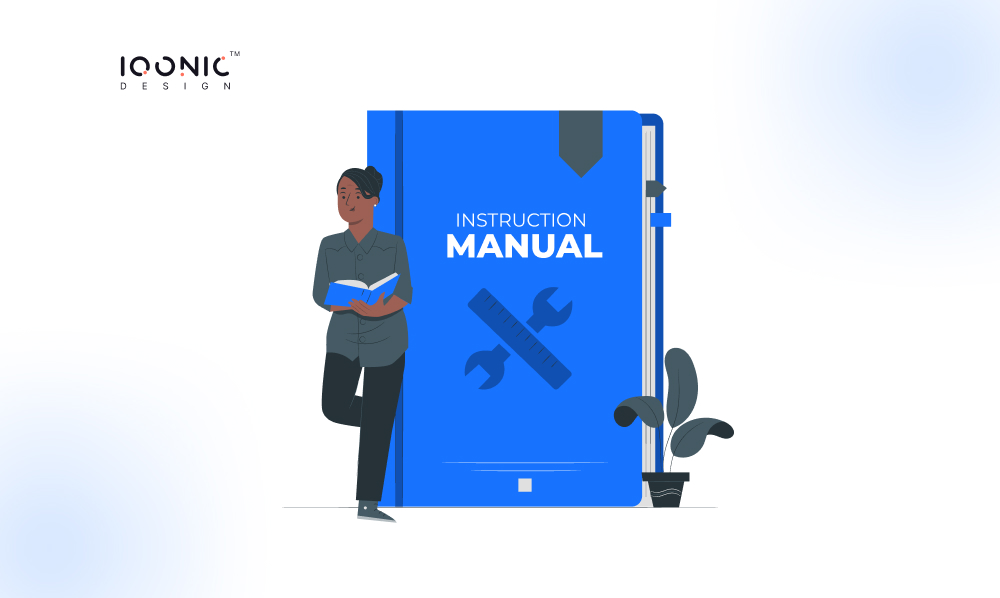
It is no secret that Flutter is rising to fame in app development. Flutter is an open-source UI framework that allows developers to create natively compiled applications for various platforms, including mobile, web, and desktop, all from a single code base.
"Why Flutter?" you might ask. Well, it has been gaining immense popularity for many reasons. Especially for beginners, it offers some notable advantages.
To begin with, Flutter simplifies the often complex world of app development. You'll only need to learn one programming language to run code on multiple platforms. You should know this if you're just starting.
Additionally, Flutter boasts a rich set of predesigned widgets, making creating stunning, custom user interfaces a breeze. Plus, its "hot reload" feature lets you see your changes instantly, speeding up the development process and making it less intimidating for newcomers.
Flutter could help you get into app development without overwhelming yourself. Our guide will help you learn the basics of Flutter to get started.
Why Choose Flutter?
So, why should beginners like you opt for Flutter when diving into app development? Let's break it down into three compelling reasons:
1. CrossPlatform Capabilities:
You can create apps for Android and iOS using Flutter. You don't have to write distinct code for each platform.
This cross-platform approach saves time and ensures your users have the same experience.
2. LightningFast Development:
Flutter's "hot reload" feature is a game-changer. It lets you instantly see the results of your code changes. There is no need to wait for the app to rebuild.
This rapid development cycle is perfect for beginners as it encourages experimentation and learning.
3. Expressive UI with Widgets:
Flutter offers a vast library of customizable widgets. These are like building blocks for your app's user interface.
Widgets in Flutter are not just visually pleasing but also highly functional. You can create intricate UIs without breaking a sweat.
In a nutshell, Flutter simplifies the development process for beginners. You can create powerful, cross-platform apps with a snappy development cycle. It's free and open source, and Google supports it. You'll also be able to keep up with updates and join a thriving community.
Setting Up Flutter
With a quick development cycle, you can create strong and cross-platform apps. It is free, open-source, and supported by Google. You'll also keep up with updates and join a thriving community.
Install Flutter on your system. To get started with Flutter on Windows, macOS, or Linux, follow these steps:
Prerequisites:
A computer running Windows, macOS, or Linux.
A stable internet connection for downloading Flutter and its dependencies.
A code editor like Visual Studio Code or Android Studio (optional but recommended).
Installation Steps:
1. Download Flutter:
Visit the official Flutter website and click "Get Started". Download the Flutter SDK for Windows, macOS, or Linux.
2. Extract the SDK:
Extract the Flutter SDK to a location of your choice after downloading.
3. Add Flutter to PATH (Linux/macOS):
Navigate to the directory where you extracted Flutter. Use the command export PATH="$PATH:
<yourflutterdirectory>`/flutter/bin"` to add Flutter to your system's PATH.
4. Run flutter doctor
:
Open a terminal window and enter the command flutter doctor.
This command will check for any missing dependencies or issues and guide you on resolving them.
5. Install Flutter Plugins (Optional):
If you're using Visual Studio Code or Android Studio, consider installing the Flutter and Dart plugins for a more streamlined development experience.
6. Configure an Editor (Optional):
If you're using an IDE like Visual Studio Code, configure it to recognize Flutter. This step usually involves installing extensions or plugins specific to your code editor.
You're all set to begin your Flutter adventure. With Flutter successfully installed and configured, you're one step closer to creating your first Flutter app.
Creating Your First Flutter App
Ready to embark on your Flutter journey by creating your very first app? We'll start with:
Step 1: Install Flutter (if not already done)
Follow the installation instructions.
Step 2: Create a new Flutter project.
Start your project in the directory you want. Run the command flutter create my_first_flutter_app.
Replace my_first_flutter_app
with your desired project name. Flutter will set up the basic project structure.
Step 3: Explore the project structure.
You'll find a lib
directory containing the main Dart code for your app in a file called main.dart
. The project directory also contains configuration files, assets, and other resources.
Step 4: Write Your First Code
Open main.dart
in your code editor.
A default "Hello World" app code will be displayed.
Modify the code to create your app. For example, change the text inside Text('Hello, World!')
to something like Text('My First Flutter App').
Step 5: Running Your App
Go to your project's directory using the terminal.
Run the command flutter run
.
Flutter will compile your app and launch it on an emulator or connected device (ensure you have one set up or running).
Step 6: See Your App in Action
Your app will now be running on the emulator/device.
Any changes you make to the code will be reflected instantly, thanks to Flutter's "hot reload" feature.
Experiment with different widgets and designs to customize your app.
Congratulations! You have just created and run your first Flutter app. This is just the beginning of your Flutter development journey.
Understanding the Flutter Architecture
The Flutter Architecture comprises key components that work seamlessly together to create apps. Here's a quick overview:
1. Widgets:
Flutter apps are built using widgets. Almost everything you see on the screen is a widget, from buttons to text to entire screens. Complex user interfaces can be created by combining widgets.
2. MaterialApp:
MaterialApp is the root widget for your app. It sets up essential services like navigation, accessibility, and localization. It also defines the overall theme and design of your Flutter app.
3. Scaffold:
A scaffold is vital to the app's structure. It provides the basic layout structure for your app, including your app bar, floating action button, and body.
The components of this system work seamlessly together MaterialApp wraps your entire app and sets the theme, while Scaffold provides the structure for individual screens or pages within your app. Widgets are used to populate these screens with interactive elements.
Flutter's Widget Hierarchy
Flutter's widget-based approach revolutionizes UI development. Let's take a look at how it all fits together:
1. Widget Tree:
Under your MaterialApp, you create a hierarchy of widgets to define your app's structure.
2. Composition:
Widgets are hierarchical, with parent widgets containing child widgets. For instance, a Column widget may contain multiple child widgets arranged vertically, while a Row widget arranges them horizontally.
3. Reusability:
Widgets are highly reusable. You can define custom widgets to encapsulate specific pieces of UI or functionality. This promotes clean, maintainable code.
4. Hot Reload:
Flutter's "hot reload" feature allows you to instantly change your widget hierarchy and see the results, speeding up development and experimentation.
Flutter's UI consists of widgets arranged in a tree structure. Thanks to widgets, each rendering part of the user interface, it is easy to create complex and interactive interfaces. Understanding this widget hierarchy is essential for mastering Flutter app development.
Building the User Interface
Creating a user-friendly interface in Flutter is as easy as assembling building blocks. Let's dive into building a simple UI layout:
1. Text Widget:
The Text
widget displays text. For example:
```dart
Text('Hello, Flutter!')
2. Image Widget:
Include images using the Image
widget. For example:
```dart
Image.asset('assets/logo.png')
```
3. Container Widget:
Customize the layout with the Container
widget. It can define width, height, color, and more. For instance:
```dart
Container(
width: 100,
height: 100,
color: Colors.blue,
)
```
4. RaisedButton Widget:
Add interactive buttons with the RaisedButton
widget. Example:
```dart
RaisedButton(
onPressed: () {
// Add action here
},
child: Text('Click Me'),
)
```
Adding Interactivity
Flutter makes your app come alive with interactivity. Let's start by adding a button that responds to user taps:
1. GestureDetector Widget:
Wrap your widget with GestureDetector
to make it tapable. Example:
```dart
GestureDetector(
onTap: () {
// Add action here
},
child: Container(
width: 100,
height: 50,
color: Colors.green,
child: Center(child: Text('Tap Me')),
),
)
```
2. Handling User Input:
inside the onTap
function, you can define what happens when the user taps the widget. It could be navigating to another screen, updating data, or displaying a message.
You can create engaging user interfaces in Flutter by combining widgets and adding interactivity. Don't forget the "hot reload" feature, which lets you instantly see the effects of your code changes, making UI development a breeze.
Running Your App on Emulators/Devices
Now that you've built your Flutter app, it's time to see it in action on emulators or real devices:
1. Emulators:
To test on emulators, open Android Studio or Xcode, create a virtual device, and run your app from your code editor using flutter run
.
2. Physical Devices:
Your Android or iOS device should be connected.
Enable Developer Mode on Android or use Xcode on macOS for iOS.
Run
flutter run
from your terminal, and your app will install and run on your device.
3. Flutter DevTools:
For debugging, use Flutter DevTools. It's a powerful suite of tools that helps you inspect UI, track performance, and diagnose issues in your app. Access it by running flutter pub global activate dev tools and then
flutter pub global run dev tools.
Conclusion and Next Steps
You've taken your first steps into the exciting world of Flutter! To recap:
Flutter simplifies app development with its cross-platform capabilities and "hot reload."
You learned about core Flutter components like widgets, MaterialApp, and Scaffold.
Building a user interface and adding interactivity is a breeze with Flutter's widgets.
Running your app on emulators/devices is easy, and you can use Flutter DevTools for debugging.
As a beginner, your journey has just begun. Explore further by diving into the official Flutter documentation, taking online courses, and joining Flutter forums. With Flutter's vibrant community and Google's support, you're on the right path to becoming a proficient app developer. Keep coding, keep experimenting, and keep learning! Your next Flutter masterpiece awaits.
Discover Your Ideal Flutter App Development Partner
You've just taken your first steps into the exciting world of Flutter app development and experienced firsthand how this framework can transform your app ideas into reality. Imagine having a dedicated team of Flutter experts ready to turn your visions into high-performing, user-centric mobile applications.
Who We Are
We are more than just developers; we are your partners in success. With a track record of delivering results in over 30,000 digital projects, we have honed our skills to perfection. We are a result-oriented team of Flutter experts, and we're here to serve you.
Who We Serve
Startups:
If you have an innovative app idea, we can make it. Our specialty is creating user-centric mobile apps that stand out, and we understand startups' unique challenges.
App Development Agencies:
Are you an agency looking to expand your capabilities in Flutter app development? We're the perfect partners to collaborate with. We provide fair and affordable solutions without compromising quality.
SMEs (Small and Medium-sized Enterprises):
We step in as a reliable partner for SMEs looking to create high-quality mobile app projects without disrupting their core operations. We recognize the significance of both efficiency and excellence.
What We Excel At
App Design (UI/UX):
Our designs marry aesthetics with functionality, ensuring your app engages and delights users.
App Development in Flutter:
We specialize in building cross-platform mobile apps that are visually stunning, high-performing, and scalable.
API / Backend Development:
Behind every successful app lies a robust backend. We excel in developing powerful APIs and backend systems.
Landing Pages for Your Apps:
A compelling landing page is your app's digital front door. We create landing pages that effectively communicate your app's value proposition.
Cloud Hosting and App Uploads:
Leave the technical details to us. We handle cloud hosting and the intricacies of app uploads, making your app accessible to users across platforms.
Support & Maintenance:
We understand that every mobile app needs ongoing updates. You can rely on us to ensure your app remains in sync with the latest technology platforms.
Why Choose Us
No, we're not "Yet Another" outsourcing partner. We're your team of experts, ready to step in whenever your project needs it. We don't just build apps; we craft digital experiences that make an impact.
Subscribe to my newsletter
Read articles from Iqonic Social directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
