Square Root Function Implementation in Assembly Language (MIPS32 ISA)
3 min read
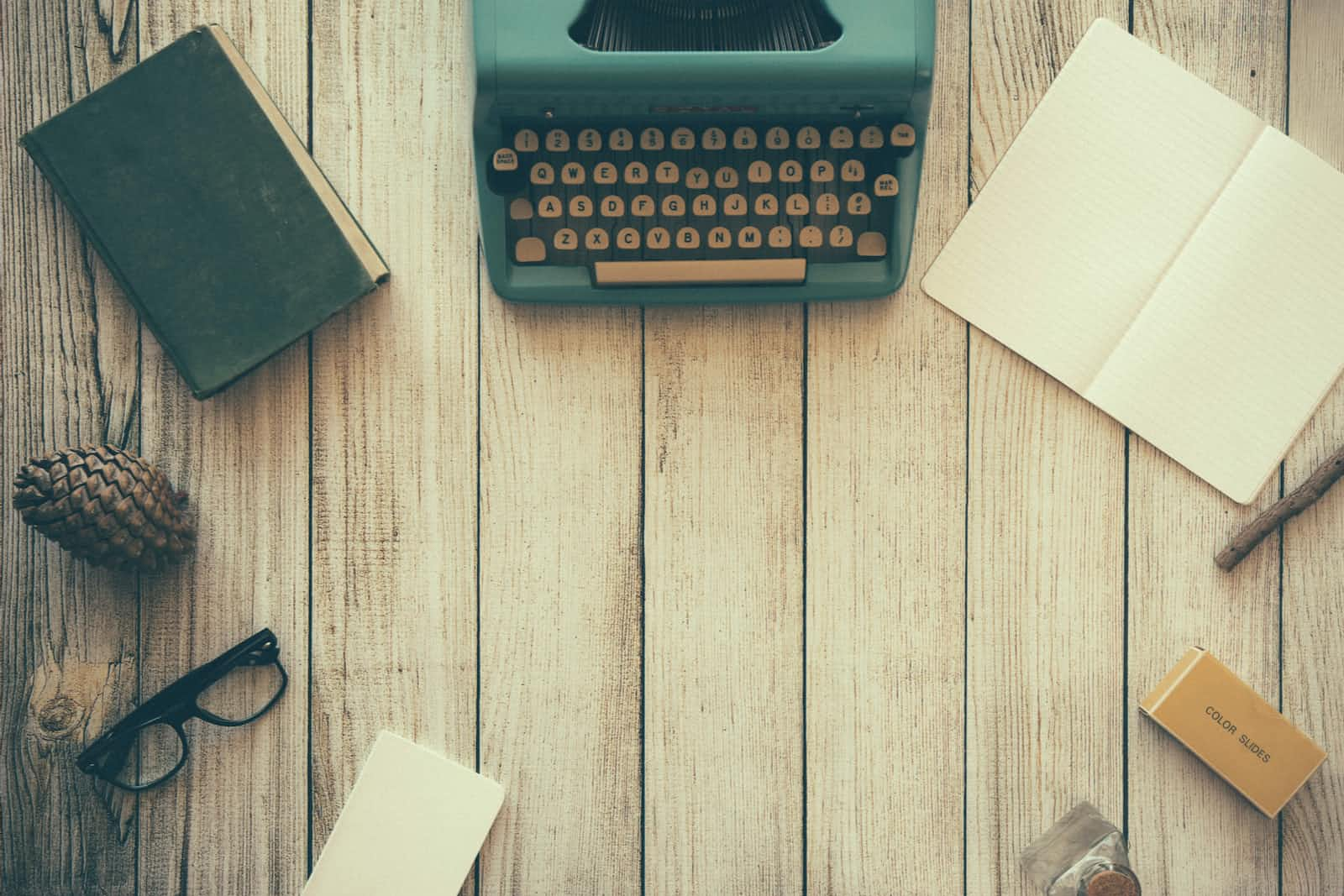
💡
You can use Cpulator (Online Simulator) to execute this code.
💡
Remember to set the stack pointer beforehand, otherwise, it might throw you an 'overflow' error. The stack pointer is typically initialized to the highest address in the data segment.
li $sp, 0x7ffffffc # Setting the stack to highest stack address
Algorithm for Square Root:
First, we'll attempt to include this C logic in the MIPS assembly program for the Square Root of a number (integer).
square_root(n){
int res = n;
for(int i=0;i<(n/res);i++){
res = (res + n/res) / 2;
}
return res;
}
MIPS32 Implementation:
This is a simple program that can be implemented in MIPS32.
💡
We are using procedures (Functions) for Square Root, Division and Multiplication
💡
Check the blog for the Division and Multiplication here:
Here is the code for Square Root (MIPS32 ISA)
SQUARE_ROOT:
# res = n
# while(i < n/2)
# res = (res + n / res) / 2;
addi $sp, $sp, -20
sw $ra, 16($sp) # Saving the return address into stack
sw $s3, 12($sp) # Saving other register values
sw $s2, 8($sp)
sw $s1, 4($sp)
sw $s0, 0($sp)
add $s3, $zero, $a0 # s3 = n
add $s0, $zero, $a0 # res = n
addi $a1, $zero, 2 # A = N, B = 2
jal DIVISON # Divison(n, 2)
add $s1, $zero, $v0 # s1 = n / 2
addi $s2, $zero, 0 # idx (s2) = 0
SQUARE_ROOT_LOOP:
beq $s1, $s2, EXIT_SQUARE_ROOT # if (idx == n/2) break
add $a0, $s3, $zero # A = n
add $a1, $s0, $zero # B = res
jal DIVISION # Divison(n, res)
add $s0, $s0, $v0 # res = res + (n/res)
add $a0, $zero, $s0 # A = res + (n/res)
addi $a1, $zero, 2 # B = 2
jal DIVISION # Divison((res + (n / res), 2)
add $s0, $zero, $v0 # res = res + (n / res) / 2
addi $s2, $s2, 1 # idx++
j SQUARE_ROOT_LOOP
EXIT_SQUARE_ROOT:
lw $s0, 0($sp) # Retrieving data stored in stack
lw $s1, 4($sp)
lw $s2, 8($sp)
lw $s3, 12($sp)
lw $ra, 16($sp) # Retrieving Return Address
addi $sp, $sp, 20 # Reseting stack pointer
jr $ra
DIVISION:
# While (A >= B) A -= B; result++
add $sp, $sp, -12 # Allocating Stack Memory
sw $s2, 8($sp) # Storing register values
sw $s1, 4($sp)
sw $s0, 0($sp)
addi $s0, $zero, 0 # result (s0) = 0
add $s1, $zero, $a0 # s1 = A
add $s2, $zero, $a1 # s2 = B
DIV_LOOP:
blt $s1, $s2, EXIT_DIV # If (A < B) break
sub $s1, $s1, $s2 # A -= B
addi $s0, $s0, 1 # result++
j DIV_LOOP
EXIT_DIV:
add $v0, $zero, $s0 # v0 = result
lw $s2, 8($sp) # restoring register values
lw $s1, 4($sp)
lw $s0, 0($sp)
addi $sp, $sp, 12 # restoring stack pointer
jr $ra
Conclusion:
In this article, you have learned to implement Square Root in Mips32 ISA
0
Subscribe to my newsletter
Read articles from Tanvir Ahmed Khan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Tanvir Ahmed Khan
Tanvir Ahmed Khan
Undergraduate Student from Bangladesh