Demystifying Train-Test Split in Machine Learning
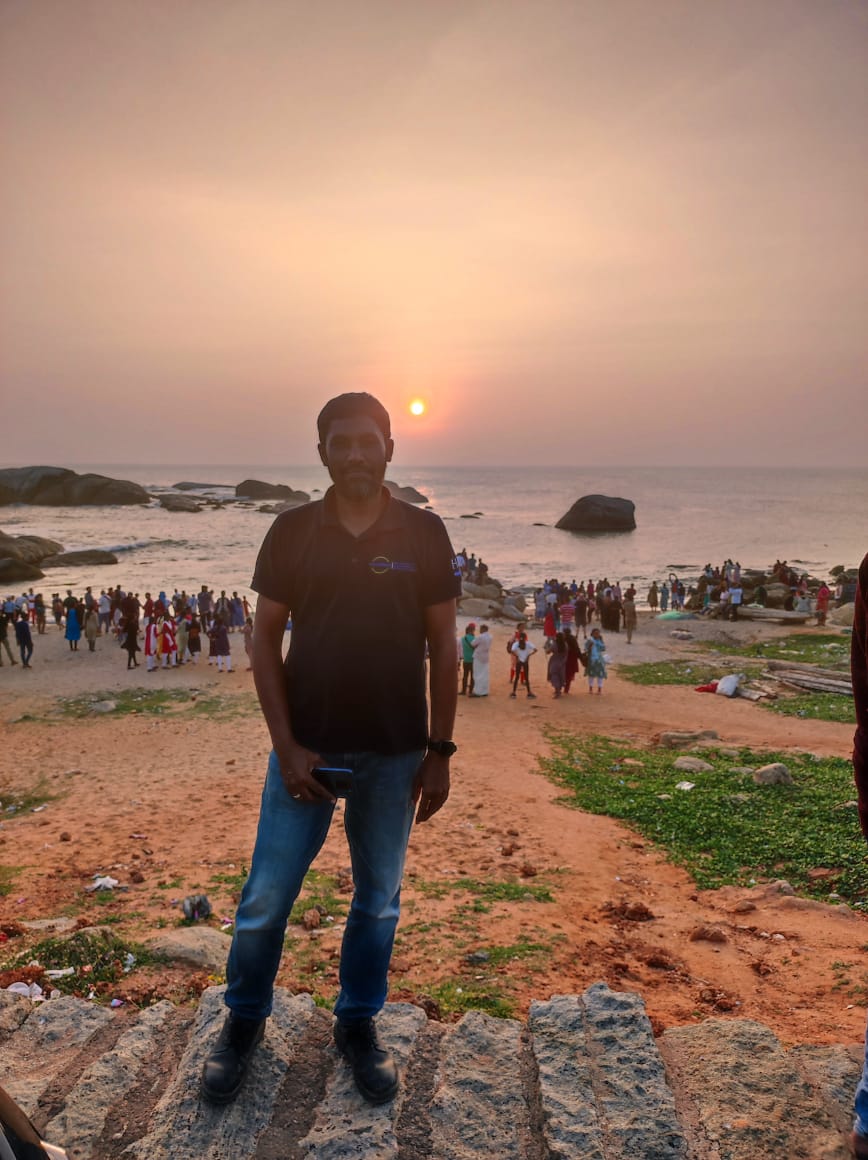
In the realm of machine learning, evaluating the performance of a model is crucial. The train_test_split
function from sklearn.model_selection
simplifies this process by dividing a dataset into training and testing sets, allowing robust model assessment. Let's delve into its significance with an example.
The purpose of train_test_split
is to partition a dataset into subsets for training and testing. This split is vital to assess how well a model generalizes to unseen data. It takes input data and labels, specifying the proportion of data allocated to the test set.
Consider a dataset with features X
and labels y
. To use train_test_split
, you'll typically perform the following:
from sklearn.model_selection import train_test_split
# Assuming X contains features and y contains labels
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
test_size
: Represents the proportion of the dataset to include in the test split. Here,test_size=0.2
indicates an 80-20 split.random_state
: Provides reproducibility. Setting it ensures the same split occurs every time you run the code.
Now, X_train
and y_train
holds the training data, while X_test
and y_test
contains the test data.
Significance:
Evaluation of Generalization: The model trains on the training set and is then assessed on the test set. This process mimics real-world scenarios where the model encounters new, unseen data.
Avoiding Overfitting: A model that performs exceedingly well on the training data but poorly on the test set may be overfitting.
train_test_split
helps detect such issues.Hyperparameter Tuning: It facilitates hyperparameter tuning by assessing how model performance varies with different parameter configurations.
Let's consider a practical example using the famous Iris dataset, where we'll use train_test_split
to split the data into training and testing sets. We'll then train a simple classifier and evaluate its performance.
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.neighbors import KNeighborsClassifier
from sklearn.metrics import accuracy_score
# Load Iris dataset
iris = load_iris()
X = iris.data
y = iris.target
# Split the dataset into training (80%) and testing (20%) sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Initialize the K-Nearest Neighbors classifier
knn_classifier = KNeighborsClassifier(n_neighbors=3)
# Train the classifier on the training set
knn_classifier.fit(X_train, y_train)
# Make predictions on the test set
y_pred = knn_classifier.predict(X_test)
# Evaluate the accuracy of the classifier
accuracy = accuracy_score(y_test, y_pred)
print(f'Accuracy of the classifier: {accuracy:.2f}')
Output
Accuracy of the classifier: 1.00
An accuracy of 1.00 (or 100%) suggests that the K-Nearest Neighbors classifier performed perfectly on the test set in this particular run. While achieving high accuracy is desirable, it's important to interpret it in context and consider potential factors:
Dataset Size: In the case of small datasets, achieving perfect accuracy is more feasible. The Iris dataset is relatively small, and the random split may lead to a test set that is well-representative of the overall data.
Model Complexity: The simplicity of the K-Nearest Neighbors model might be suitable for the Iris dataset, which is known for its distinct classes. For more complex datasets, achieving perfect accuracy might be a sign of overfitting.
Random State: The
random_state
parameter is set to 42, ensuring reproducibility. However, changing this seed may result in different splits and, consequently, different accuracy values.Noisy Data: If the dataset contains noise or outliers, it might affect the performance of the model. In this case, it's worth exploring other evaluation metrics and potentially preprocessing the data
train_test_split
is a fundamental tool for model evaluation and selection. Its simplicity and effectiveness make it a cornerstone in the development of robust and generalizable machine-learning models. Integrating this function into your workflow enhances your ability to build models that perform well on unseen data, a crucial aspect of successful machine learning applications.
Subscribe to my newsletter
Read articles from K Ahamed directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
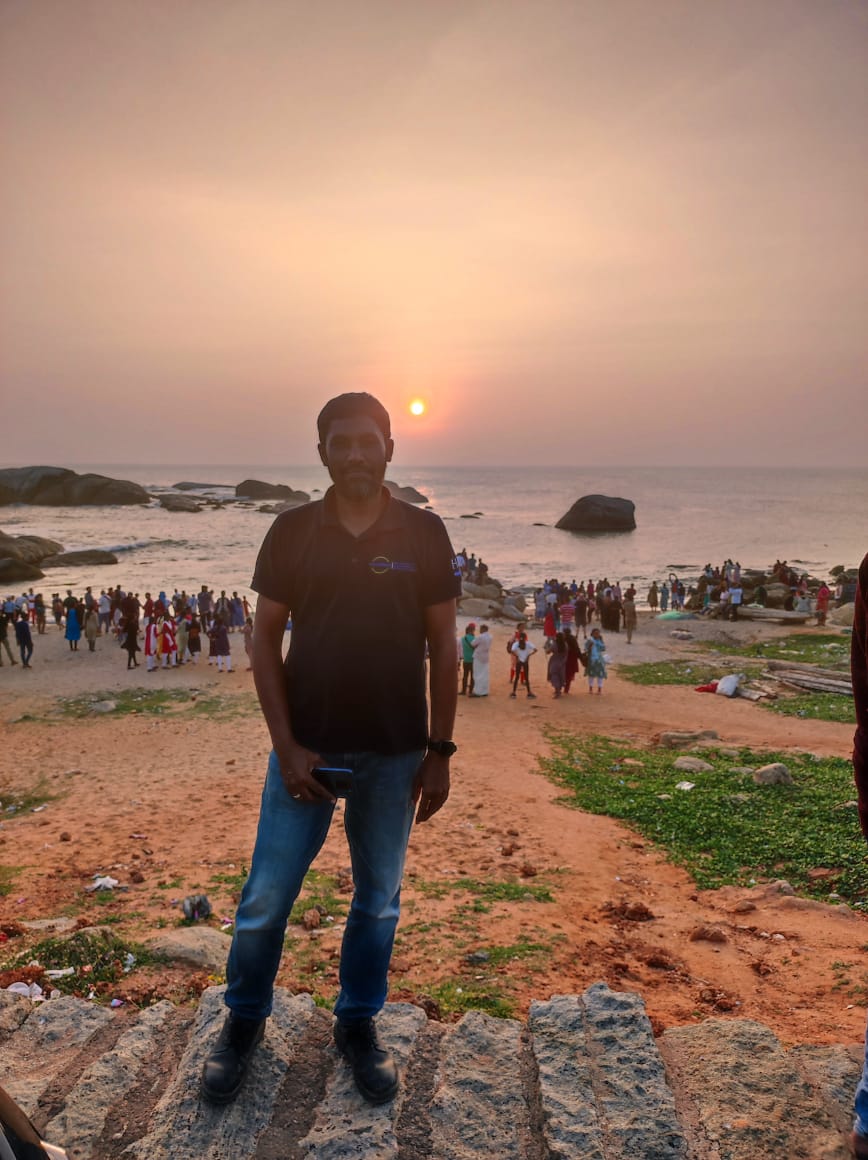
K Ahamed
K Ahamed
A skilled construction professional specializing in MEP projects. Armed with a Master's degree in Data Science, seamlessly combines hands-on expertise in construction with a passion for Python, NLP, Deep Learning, and Data Visualization. While currently at a basic level, dedicated to enhancing data skills, envisioning a future where insights derived from data reshape the landscape of construction practices. With a forward-thinking mindset, building structures but also shaping the future at the intersection of construction and data.