Building an E-commerce System: A Comprehensive Code Breakdown - Part 2
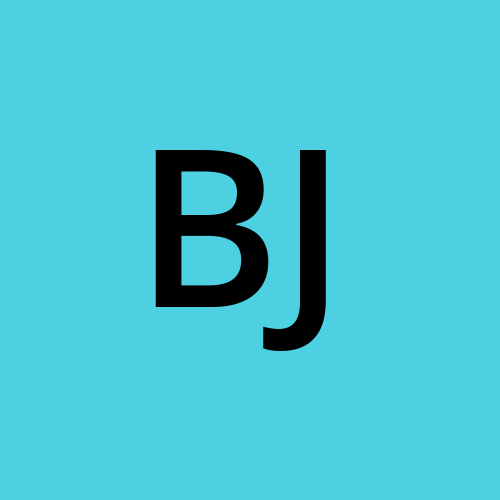
Welcome back to the second part of our journey through the codebase of an e-commerce system. In this installment, we'll explore the Order
class, which plays a pivotal role in managing the order lifecycle and ensuring a seamless shopping experience for customers.
Part 2: Order Processing and Fulfillment
In an e-commerce system, handling customer orders efficiently is paramount. The Order
class is at the heart of this process, facilitating everything from order creation to payment processing and generating receipts.
The Order
Class
class Order {
// ... (Constructor and other fields)
public void addItemToOrder(Product product, int quantity) {
// Check product availability and reduce quantity from inventory
if (product != null && product.getQuantity() >= quantity) {
OrderItem orderItem = new OrderItem(product, quantity);
orderItems.add(new OrderItem(product, quantity));
product.reduceQuantity(quantity);
product.updateSales(quantity);
}
}
public void processPayment(boolean paymentSuccessful) {
if (paymentSuccessful) {
updateOrderStatus(OrderStatus.PAID);
} else {
updateOrderStatus(OrderStatus.CANCELLED); // Update the status to CANCELLED on payment failure
}
}
// Other methods...
}
Explanation:
The
Order
class represents a customer's order, containing details like order items, customer information, and order status.The
addItemToOrder
method allows customers to add products to their order while checking availability and updating the order's content and product inventory.The
processPayment
method handles payment processing, updating the order status based on payment success or failure.
Key Takeaways:
Streamlined order creation and management.
Real-time inventory updates based on order contents.
Robust payment processing and order status handling.
The Order
class ensures that customers can create orders, and the platform efficiently processes payments and manages the order status.
In the next part of our series, we'll explore how the system calculates order totals, including subtotals, taxes, and the final cost. This is crucial for providing customers with transparent and accurate pricing.
We've now covered Part 2 of our comprehensive code breakdown series. If you have any questions or suggestions, please feel free to share them. In Part 3, we'll explore the world of pricing and taxation in e-commerce.
Subscribe to my newsletter
Read articles from Bryan Samuel James directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
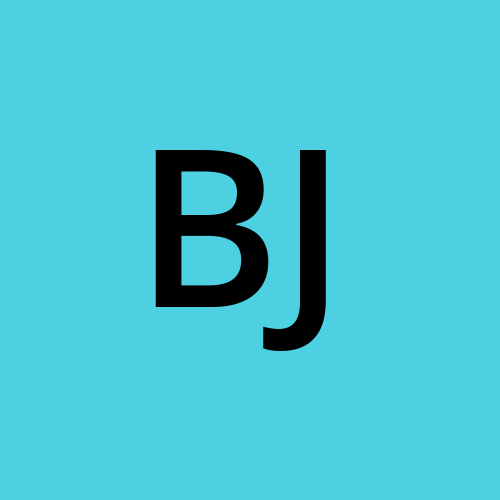