Jenkins Important interview Questions

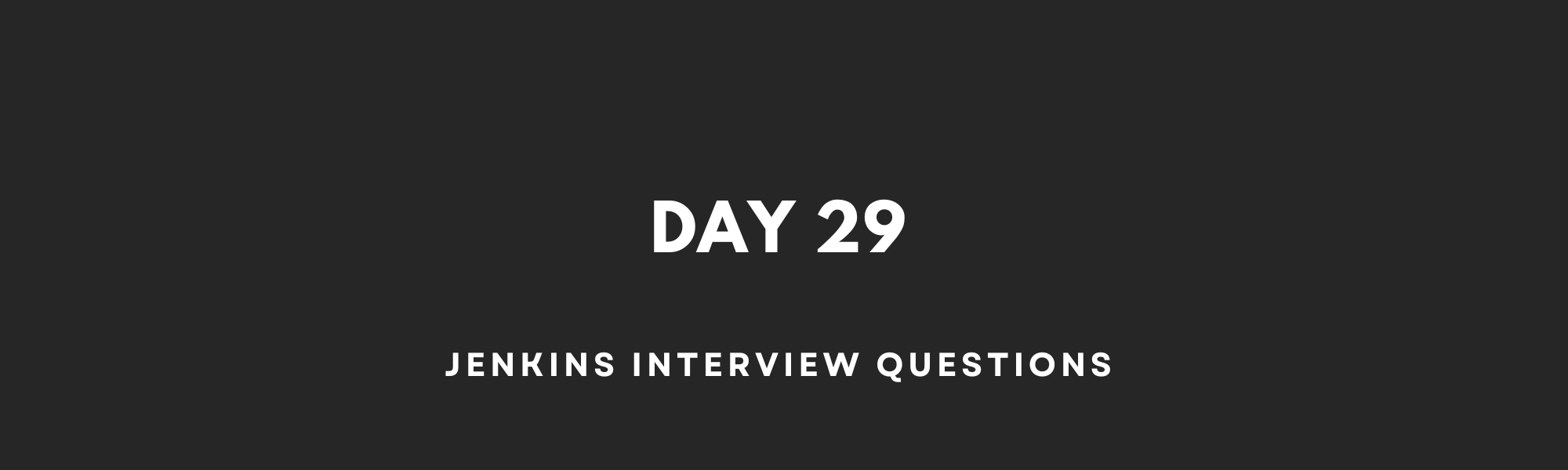
What’s the difference between continuous integration, continuous delivery, and continuous deployment?
CI/CD stands for Continous Integration and Continous Delivery/Deployment.
Continuous Integration (CI)
Continuous Integration (CI) is a development practice centered around the seamless merging and verification of code changes. When a developer commits or adds new code, CI involves assessing its functionality by confirming that it builds successfully, passes predefined test cases without issues, and operates without errors. If these criteria are met, the code is deemed successfully integrated.
CI serves as an early warning system, detecting potential issues promptly and allowing developers to rectify problems before they escalate. By automating the integration, testing, and assessment of code changes, CI enhances collaboration among developers, minimizes errors, and accelerates the development process.
Benefits:
Early detection of integration issues.
Faster feedback to developers.
Reduced integration risk.
Improved collaboration among team members.
Continuous Delivery (CD)
Continuous Delivery is a software development practice where code changes are automatically prepared, tested, and made ready for deployment to a production environment. In CD, every code change that passes automated tests is potentially deployable to production, but the actual deployment to the production environment is typically triggered manually by a team member or through an approval process.
Benefits:
Faster and more reliable releases.
Reduced manual intervention in the release process.
Increased confidence in deployments.
Continuous Deployment
Continuous Deployment takes the concept of Continuous Delivery a step further by automating the entire process of deploying code changes to production without manual intervention. In this approach, any code change that passes automated tests is automatically deployed to the production environment.
Benefits:
Rapid and continuous delivery of new features and bug fixes.
Minimal human error in deployments.
Immediate user feedback
Benefits of CI/CD?
Answer-: There are several benefits to implementing CI/CD in a software development workflow:
Faster development cycles.
Lower risk of integration issues.
Improved software quality.
Greater collaboration among teams.
Reduced manual intervention.
Faster time to market.
What is meant by CI-CD?
Answer-: CI/CD stands for Continuous Integration and Continuous Deployment (or Continuous Delivery), and it refers to a set of practices and principles in software development that aim to automate and streamline the process of building, testing, and deploying software changes. These practices help software development teams deliver code more frequently, reliably, and efficiently.
What is Jenkins Pipeline?
Answer-: Jenkins Pipeline is a powerful and flexible feature in the Jenkins automation server that allows you to define and automate your software delivery pipeline as code. It provides a way to script and manage your build, test, and deployment processes within Jenkins, making it easier to create, maintain, and version control your pipelines.
How do you configure the job in Jenkins?
Steps to configure a job in Jenkins:
Access Jenkins Dashboard port no 8080.
Click on the "New Item" or "Create New Job" link on the Jenkins dashboard.
Give your job a name and select the type of job you want to create.
In the job configuration page, specify all data like Specify a description for your job (optional), Choose the source code management system (e.g., Git, Subversion) and provide repository details, Define the build triggers (e.g., manual, scheduled, SCM changes), Configure Build Environment (Optional).
Build Steps define the steps that Jenkins should execute during the build process. For a Freestyle project, you can add build steps such as shell commands, Windows batch commands, or other build tools.
For a Pipeline job, you define your build and deployment process using Groovy script in either Declarative or Scripted syntax.
Once you've configured all the necessary settings for your job, click the "Save" or "Apply" button to save your job configuration.
You can manually trigger the job to run by clicking the "Build Now" or "Build" button on the job's dashboard page. Monitor the job's progress, logs, and build results in the Jenkins dashboard.
After the job has run, you can view its build history, console output, and any artefacts generated by the job.
Where do you find errors in Jenkins?
The error can be found at "Console Outputs" which can be seen by clicking on the build at the bottom left corner and then selecting "Console Output". It provides a detailed log of all the steps it performs during the build process.
Pipeline Stage Logs: If it is a pipeline you can also see the logs of a particular stage by clicking on that particular stage of the visually represented pipeline.
In Jenkins how can you find log files?
Jenkins logs are stored in the jenkins.log file. The location of this file hinges on the Jenkins installation and operating system.
Common destinations include /var/log/Jenkins/jenkins.log (Linux) or C:\Program Files (x86) \Jenkins\jenkins.log (Windows).
Jenkins workflow and write a script for this workflow?
A fundamental Jenkins workflow could encompass:
Retrieving code from version control.
Building the code.
Executing tests.
Packaging artifacts.
Deploying to a staging environment.
Here's a simplified Jenkins Pipeline script exemplifying this workflow:
pipeline { agent any stages { stage('Checkout') { steps { // Code checkout from version control } } stage('Build') { steps { // Code build activities } } stage('Test') { steps { // Running tests } } stage('Deploy to Staging') { steps { // Deploying to staging } } } }
How to create a continuous deployment in Jenkins?
a step-by-step guide on how to create a continuous deployment in Jenkins:
Set Up Jenkins in your local.
create a Jenkins pipeline using either a scripted pipeline or a declarative pipeline. example of a declarative pipeline that you can use as a starting point for continuous deployment:
COPY
COPY
# Customize the stages according to your project's requirements. pipeline { agent any stages { stage('Checkout') { steps { checkout scm } } stage('Build') { steps { // Build your application here (e.g., compile, package) } } stage('Test') { steps { // Run automated tests } } stage('Deploy to Production') { steps { // Deploy your application to production here } } } }
configure environment-specific variables, such as credentials and environment URLs, as Jenkins environment variables or in a secure credential store, depending on your security and infrastructure setup.
Ensure that proper access control mechanisms are in place, depending on your deployment strategy. The deployment to production should only proceed if all tests pass successfully.
Test the pipeline thoroughly to validate the automated deployment process. The deployment to production should only proceed if all tests pass successfully.
How to build a job in Jenkins?
To build a job in Jenkins, follow these steps:
Login to Jenkins: Access the Jenkins web interface using your web browser.
Create a New Job:
Click on "New Item" or "Create a new job" on the Jenkins dashboard.
Enter a name for your job.
Select the type of job you want to create (e.g., Freestyle project, Pipeline, Multi-configuration project).
General Configuration:
Configure basic settings such as the job description and parameters if needed.
Specify the source code management (SCM) system (e.g., Git, Subversion) and provide repository details.
Build Triggers:
Define when the job should be triggered. Options include:
Polling the SCM for changes.
Triggering the job manually.
Triggering the job remotely using a webhook.
Scheduling the job to run at specific times.
Build Environment (Optional):
- Configure the build environment if necessary. This may include setting environment variables, choosing build tools, or specifying custom workspace locations.
Build Steps:
Define the actions that the job should perform. This is where you specify the build, test, and deployment commands or scripts.
For Freestyle projects, you can use a wide range of build steps provided by Jenkins or install additional plugins to extend functionality.
For Pipeline projects, you define your build steps using Groovy scripts within a Jenkinsfile.
Post-Build Actions (Optional):
Configure actions to be taken after the build is complete. Common post-build actions include:
Archiving artifacts for later reference.
Sending email notifications.
Publishing test results and code coverage reports.
Triggering downstream jobs.
Source Code Management (SCM):
- Configure how Jenkins should retrieve the source code from your version control system (e.g., Git, SVN). Provide the repository URL, credentials, and branch to build.
Save and Build:
Save your job configuration by clicking "Save" or "Apply."
You can initiate a build manually by selecting "Build Now" if your job is not configured to trigger automatically.
View Build History:
- Monitor the build progress and access build logs and reports. Jenkins will keep a history of all builds for the job.
Why do we use a pipeline in Jenkins?
We use pipelines in Jenkins for the following key reasons:
Automation: Pipelines automate the entire software delivery process, from building and testing to deployment. This reduces manual intervention and human error.
Consistency: Pipelines ensure that the same set of steps is followed every time code is built and deployed, leading to consistent and reliable results.
Visibility: Pipelines provide a clear visual representation of the entire workflow, making it easier to monitor, track, and troubleshoot the process.
Reproducibility: Pipelines define the entire workflow as code (Jenkinsfile), which can be version-controlled and reused across projects, ensuring reproducibility.
Scalability: Pipelines scale with the development team and project complexity, making it suitable for both small and large-scale projects.
Flexibility: Jenkins pipelines can be tailored to specific technology stacks and development processes, offering flexibility and customization.
Efficiency: By automating repetitive tasks, pipelines streamline the software delivery cycle, leading to faster releases and bug fixes.
Is Only Jenkins enough for automation?
No, Jenkins alone is not always enough for automation. While Jenkins is a powerful and widely used automation server for building, testing, and deploying software, it is just one component of a larger automation ecosystem. The choice of tools and technologies for automation depends on the specific needs and complexities of your software development and deployment processes.
How will you handle secrets?
Handling secrets in Jenkins can be done using several methods:
Jenkins Credentials Plugin: Jenkins has a built-in Credentials Plugin that allows you to securely store and manage secrets, such as usernames and passwords, API tokens, SSH keys, and more. You can then reference these credentials in your Jenkins jobs and pipelines.
Secrets Management Tools: Use dedicated secrets management tools like HashiCorp Vault or AWS Secrets Manager to store and retrieve sensitive data securely. Jenkins can integrate with these tools to fetch secrets when needed.
Environment Variables: Store secrets as environment variables in Jenkins, but ensure that access to these variables is restricted. This method is less secure than using dedicated secrets management.
Third-Party Plugins: Jenkins offers various third-party plugins that enhance secrets management, such as the "HashiCorp Vault Plugin" for integrating with HashiCorp Vault.
Cloud Provider Services: If you're running Jenkins on a cloud platform like AWS or Azure, consider using their native secrets management services for secure storage and retrieval of secrets.
Explain different stages in CI-CD setup.
Certainly, here are the key stages in a CI/CD (Continuous Integration/Continuous Deployment) setup:Code Integration: Developers frequently merge their code into a shared repository.
Automated Build: The CI server automatically compiles and builds the code.
Automated Testing: Automated tests are run to ensure code quality.
Artifact Creation: Build artifacts, like executables or binaries, are created.
Deployment to Staging: Code is deployed to a staging environment for further testing.
Manual Testing: Human testers perform additional checks in the staging environment.
Approval: If all tests pass, the code is approved for deployment to production.
Deployment to Production: The code is automatically or manually deployed to the production environment.
Monitoring and Feedback: Continuous monitoring provides feedback for future improvements.
Name some of the plugins in Jenkin.
Certainly, here are some commonly used plugins in Jenkins:
Git Plugin: Integrates Jenkins with Git version control.
GitHub Plugin: Provides integration with GitHub repositories.
Docker Plugin: Allows Jenkins to build and publish Docker images.
Pipeline Plugin: Enables the creation of pipelines using Jenkinsfile.
Artifactory Plugin: Integrates with JFrog Artifactory for artifact management.
JUnit Plugin: Parses JUnit test results for reporting.
Email Extension Plugin: Sends customizable email notifications.
Credentials Plugin: Manages credentials securely.
Matrix Authorization Strategy Plugin: Provides advanced access control.
Slack Notification Plugin: Sends build notifications to Slack.
Subscribe to my newsletter
Read articles from Ajay Gite directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
