Building an E-commerce System: A Comprehensive Code Breakdown - Part 3
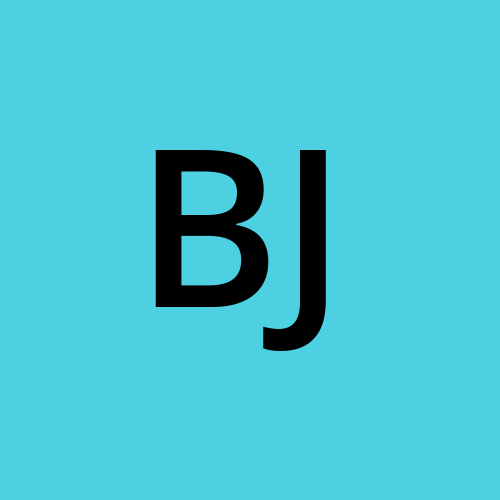
Welcome to the third installment of our exploration of the codebase for our e-commerce system. In this part, we'll delve into the crucial aspects of pricing, taxation, and generating order receipts.
Part 3: Pricing and Taxation
Pricing and taxation are fundamental components of any e-commerce system. Customers expect transparent and accurate pricing, while businesses must adhere to tax regulations. Our codebase handles these aspects with precision.
Pricing and Tax Calculation
In the e-commerce world, calculating the total cost of an order involves several components:
Subtotal: The sum of the prices of all items in the order.
Tax: Calculated based on the customer's location and the product type.
Total Cost: The final amount to be paid by the customer.
Let's take a closer look at how our code accomplishes this:
public class Order {
// ... (Constructor and other fields)
public double calculateSubtotal() {
double subtotal = 0.0;
for (OrderItem item : orderItems) {
subtotal += item.calculateItemCost();
}
return subtotal;
}
public double calculateTotalTax() {
double totalTax = 0.0;
for (OrderItem item : orderItems) {
totalTax += item.calculateItemTax();
}
return totalTax;
}
public double calculateTotalCost() {
double subtotal = calculateSubtotal();
double totalTax = calculateTotalTax();
double totalCost = subtotal + totalTax;
return totalCost;
}
// Other methods...
}
Explanation:
The
calculateSubtotal
method iterates through order items, summing up their costs to compute the subtotal.calculateTotalTax
calculates the total tax for the order by summing the taxes for each item.calculateTotalCost
brings it all together, adding the subtotal and total tax to determine the final cost.
Tax Calculation Details
Taxation varies by location and product type. Our codebase provides flexibility to handle different tax rates:
public double calculateTax(double subtotal, String customerLocation, String productType) {
// Retrieve the applicable tax rate based on the customer's location and product
double taxRate = getTaxRateByLocationAndProductType(customerLocation, productType);
// Calculate tax amount
double taxAmount = subtotal * (taxRate / 100.0);
// Store the tax amount in the Order class or return it
return taxAmount;
}
Explanation:
The
calculateTax
method takes the subtotal, customer location, and product type as inputs to calculate tax.It retrieves the applicable tax rate using
getTaxRateByLocationAndProductType
.The tax amount is calculated as a percentage of the subtotal and returned.
Generating Receipts
Once an order is complete, it's essential to provide customers with a clear and detailed receipt:
public void generateReceipt() {
// Create a receipt object or print the details directly (not shown here)
System.out.println("Receipt for Order ID: " + orderId);
// ... (Printing other details)
System.out.println("Total Cost: $" + totalCost);
System.out.println("Thank you for shopping with us!");
}
Explanation:
- The
generateReceipt
method generates a receipt, including order details, itemized costs, and a thank-you message.
Key Takeaways:
Transparent and accurate pricing for customers.
Taxation flexibility based on location and product type.
Detailed order receipts for a professional customer experience.
In Part 3, we've explored how our e-commerce system calculates pricing and handles taxation, ensuring a smooth and fair shopping experience. In Part 4, we'll dive into user management and access control, highlighting the importance of user roles in an e-commerce platform.
We've now covered Part 3 of our comprehensive code breakdown series. If you have any questions or suggestions, please feel free to share them. In Part 4, we'll explore user management and access control in e-commerce.
Subscribe to my newsletter
Read articles from Bryan Samuel James directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
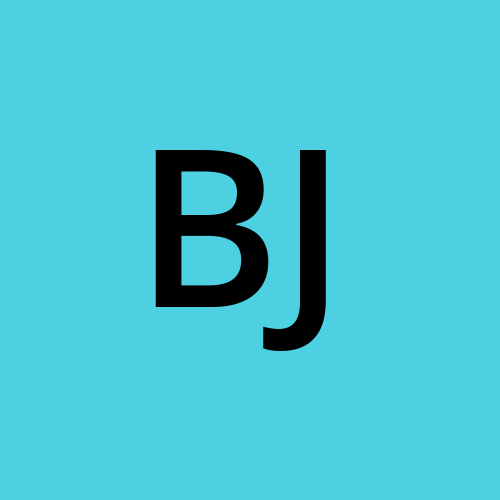