Day-3 : TWS BashBlaze Challenge
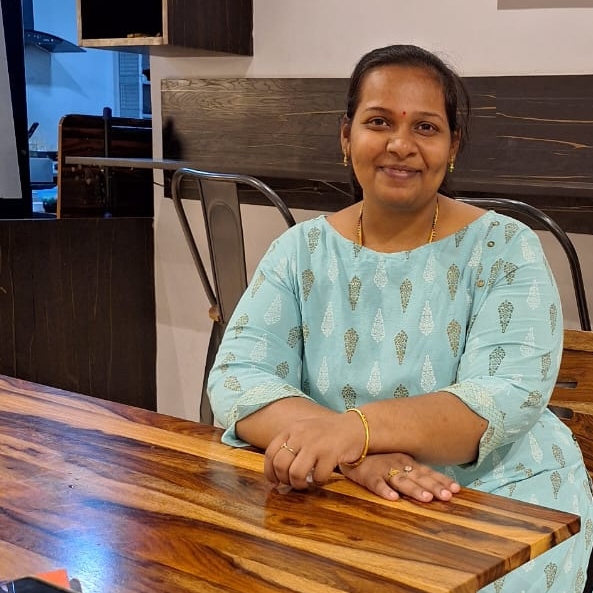
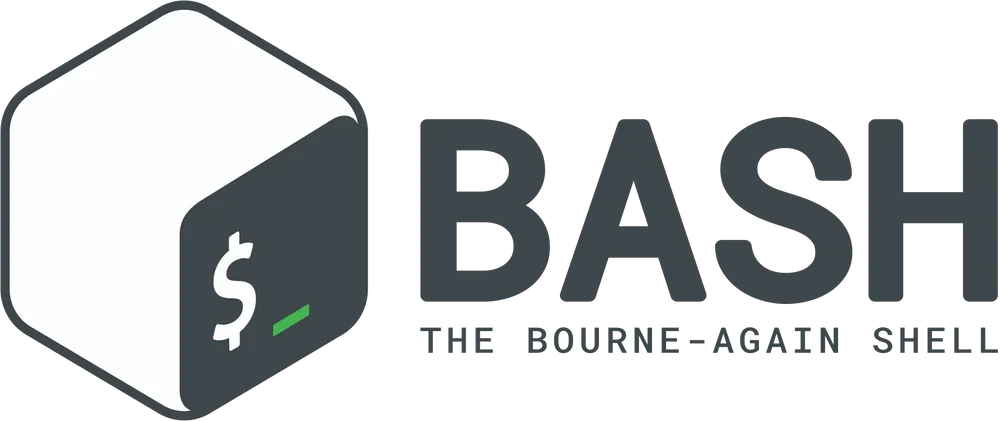
Welcome to Day 3 of our Bash Scripting Challenge! 😊 Today, we'll be using our awesome Bash scripting skills to handle user management. Together, we'll create a handy script that lets us add or remove users, reset passwords, list users, and even show helpful usage info for our script. Let's get started!
✔ Account Creation 🔎:
In this task, we need to write a bash script that creates a user account. Here, the script should prompt the user to enter a new username and password. The script should check if the username already exists. If the user exists then the script should pass a statement throwing an error else it should create a user for you. Let's script it!
# Create a new user account
if [ "$1" == "-c" ] || [ "$1" == "--create" ]
then
read -p "Enter a new username: " username
id "$username" &>/dev/null
if [ $? -eq 0 ]; then
echo "The username '$username' already exists. Please choose a different username."
else
sudo useradd -m "$username"
sudo passwd "$username"
echo "User account '$username' created successfully."
fi
❌Account Deletion 🚫:
Now, let's try to perform the deletion of a user account. the function initially verifies whether the specified user exists. If the user exists, it proceeds to delete the account; otherwise, it displays an error message.
delete_user(){
echo
read -p "Enter username : " username
check_user_exists "$username"
if (( $? == 0 ))
then
sudo userdel -r "$username"
if (( $? == 0 ))
then
echo User - "$username", deleted successfully
else
echo Error : unable to delete user
fi
else
echo Error : $username user doesnot exists
fi
echo
}
📌Password Reset 🔐:
Next, we'll implement the password reset feature. The script should ask for the username and the new password. If the username doesn't exist, the script should inform and exit.
function if_user_exists() {
if id "$1" &>/dev/null; then
return 0
else
return 1
fi
}
function reset_passwd() {
read -p "Enter the username to reset password: " username
if if_user_exists "$username"; then
read -s -p "Enter the password for $username: " password
echo "$username:$password" | sudo chpasswd
echo
echo "Password for user '$username' reset successfully."
else
echo "Error: The username '$username' does not exist. Please enter a valid username."
fi
}
☀List User Accounts 📝:
Now, let's try to implement a script that allows us to list all the user accounts along with their UIDs.
list_users(){
echo
echo "List of users on the system:"
sudo cat /etc/passwd | awk -F: '{print $1}'
echo
}
🍁Print script usage info✏:
Now finally we need to add a help option to display usage information and the available command-line options. So let's write a function for the same.
if [ "$1" == "-h" ] || [ "$1" == "--help" ]
then
echo "Usage: $0 [OPTIONS]"
echo "Options:"
echo " -c, --create Create a new user account."
echo " -d, --delete Delete an existing user account."
echo " -r, --reset Reset password for an existing user account."
echo " -l, --list List all user accounts on the system."
echo " -h, --help Show this help message"
echo " -i, --info Display the user information"
echo " -m, --modify Modify user properties"
exit 0
fi
🔅Putting it all together📂:
In this example, a case statement is used to check user input from the command line and execute the corresponding function.:
#!/bin/bash
# Check for help option
if [ "$1" == "-h" ] || [ "$1" == "--help" ]
then
echo "Usage: $0 [OPTIONS]"
echo "Options:"
echo " -c, --create Create a new user account."
echo " -d, --delete Delete an existing user account."
echo " -r, --reset Reset password for an existing user account."
echo " -l, --list List all user accounts on the system."
echo " -h, --help Show this help message"
echo " -i, --info Display the user information"
echo " -m, --modify Modify user properties"
exit 0
fi
# Create a new user account
if [ "$1" == "-c" ] || [ "$1" == "--create" ]
then
read -p "Enter a new username: " username
id "$username" &>/dev/null
if [ $? -eq 0 ]; then
echo "The username '$username' already exists. Please choose a different username."
else
sudo useradd -m "$username"
sudo passwd "$username"
echo "User account '$username' created successfully."
fi
# Delete an existing user account
elif [ "$1" == "-d" ] || [ "$1" == "--delete" ]
then
read -p "Enter the username to delete: " username
id "$username" &>/dev/null
if [ $? -eq 0 ]; then
sudo userdel -r "$username"
echo "Done! Account '$username' deleted successfully."
else
echo "Sorry, The username '$username' doesn't exist.Please enter a valid username"
fi
# Reset password for a user account
elif [ "$1" == "-r" ] || [ "$1" == "--reset" ]
then
read -p "Enter the username to reset password: " username
id "$username" &>/dev/null
if [ $? -eq 0 ]; then
sudo passwd "$username"
echo "Password for '$username' reset successfully."
else
echo "Uh-oh! '$username' doesn't exist."
fi
# List all the user accounts
elif [ "$1" == "-l" ] || [ "$1" == "--list" ]
then
echo "User accounts on the system :"
echo "Username UID"
echo "----------------"
awk -F: '{print $1 " " $3}' /etc/passwd
# Display detailed information about a user account
elif [ "$1" == "-i" ] || [ "$1" == "--info" ]
then
read -p "Enter username to display information: " info_username
if id "$info_username" &>/dev/null; then
finger "$info_username"
else
echo "Error: Username '$info_username' does not exist."
fi
# Modify user accoun properties
elif [ "$1" == "-m" ] || [ "$1" == "--modify" ]
then
read -p "Enter username to modify properties: " modify_username
if id "$modify_username" &>/dev/null; then
read -p "Enter new username (leave empty to keep current): " new_username
read -p "Enter new UID (leave empty to keep current): " new_uid
if [[ -n "$new_username" ]]; then
sudo usermod -l "$new_username" "$modify_username"
echo "Username for user '$modify_username' changed to '$new_username'."
fi
if [[ -n "$new_uid" ]]; then
sudo usermod -u "$new_uid" "$modify_username"
echo "UID for user '$modify_username' changed to '$new_uid'."
fi
else
echo "Error: Username '$modify_username' does not exist."
fi
# Handle invalid options
else
echo "Oops! Invalid option: $1"
echo "Use '$0 -h' to see available options."
exit 1
fi
Instructions for running the script:
Write the script and save it as
user_management.sh
.Make the script executable using chmod:
chmod +x user_management.sh
.Execute the script for the required options as follows:
Create a new user:
./user_management.sh -c
or./user_management.sh --create
Delete an existing user:
./user_management.sh -d
or./user_management.sh --delete
Reset user password:
./user_management.sh -r
or./user_management.sh --reset
List all user accounts:
./user_management.sh -l
or./user_management.sh --list
Display help:
./user_management.sh -h
or./user_management.sh --help
Now, you have a powerful Bash script to manage user accounts on your system, this script will save you time and effort, ensuring smooth user account management.
Thank you for reading. please do share🔄 and click the like👍 to show your support.
Keep learning and happy scripting! 🤗
Subscribe to my newsletter
Read articles from Siri Chandana directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
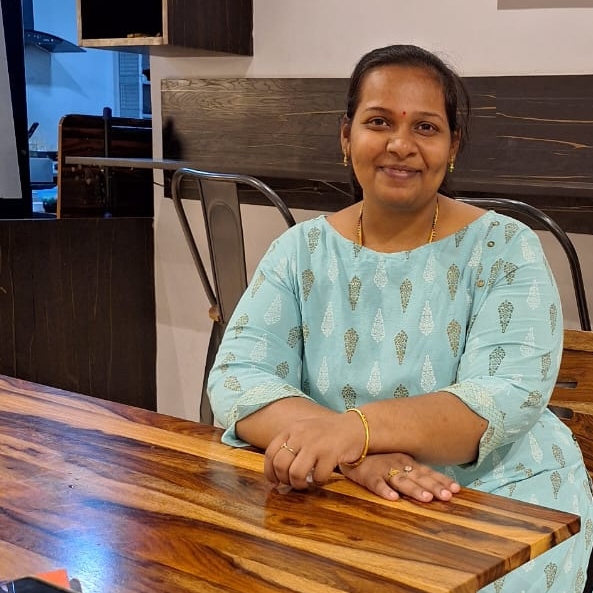