Unlocking the Power of the 'yield' Keyword in Python

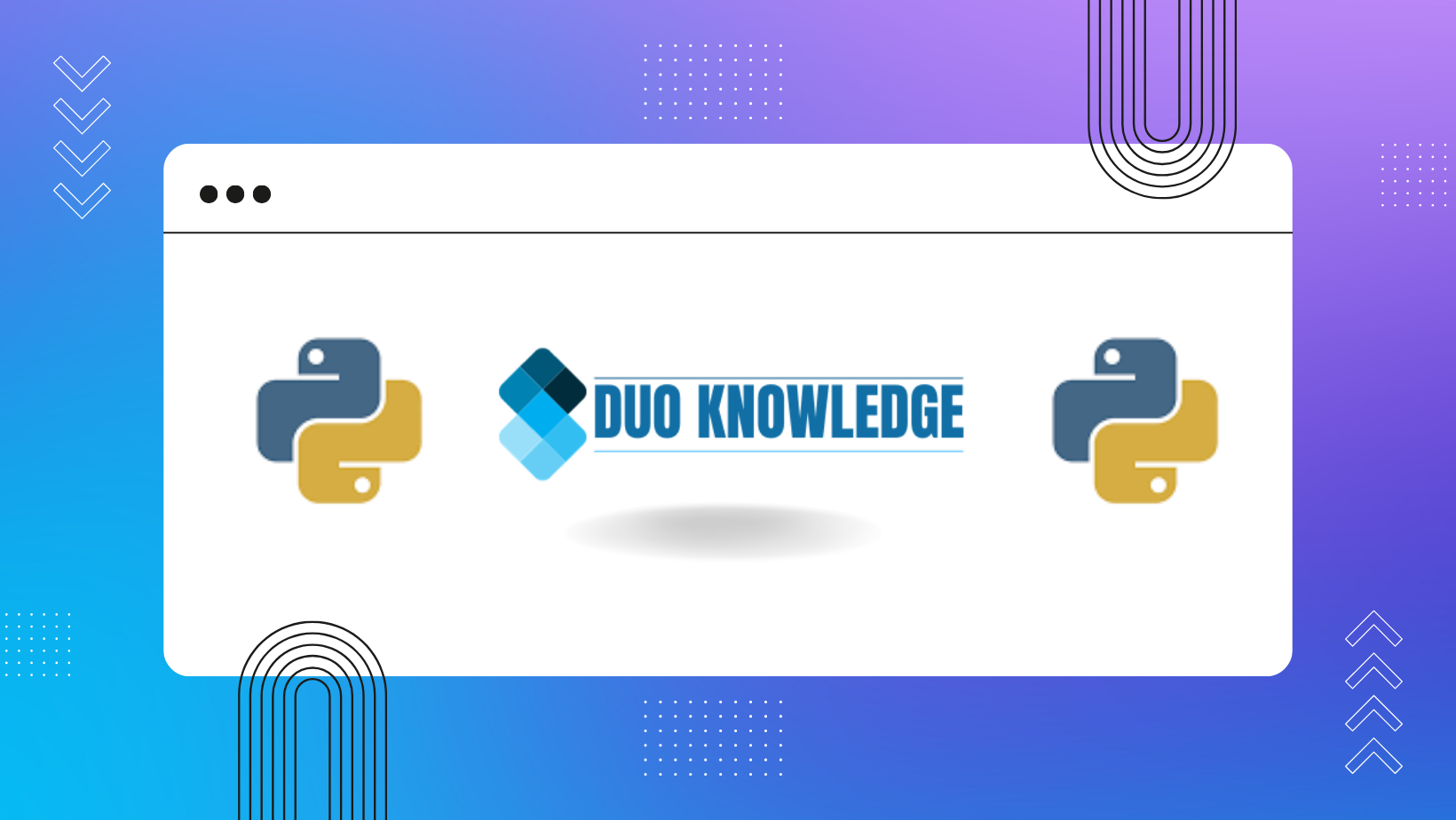
In Python, the yield
keyword is used in the context of generators and iterators. It allows you to create a generator function, which is a special type of function that can be iterated over, and it doesn't hold the entire sequence in memory. Instead, it generates values one at a time as needed. Here are some common use cases for yield
:
Lazy Evaluation: Generators are useful for generating values on the fly, which can be especially beneficial when working with large datasets. Instead of creating a list with all the values at once, you can generate and yield each value as it's needed, conserving memory.
def generate_numbers(n): for i in range(n): yield i for num in generate_numbers(5): print(num)
Stream Processing: You can use generators to process data as it's read from a file or received from a network stream, making it possible to handle large files or continuous data streams efficiently.
def process_large_file(file_path): with open(file_path, 'r') as file: for line in file: yield line.strip() for line in process_large_file('large_data.txt'): # Process each line one at a time print(line)
Infinite Sequences: Generators can be used to create infinite sequences, such as an infinite sequence of Fibonacci numbers or prime numbers.
def fibonacci(): a, b = 0, 1 while True: yield a a, b = b, a + b fib = fibonacci() for _ in range(10): print(next(fib))
Stateful Generators: You can use
yield
to maintain state across generator function calls. This is useful when you need to keep track of some information between iterations.def stateful_generator(): total = 0 while True: value = yield total if value is not None: total += value gen = stateful_generator() next(gen) # Start the generator print(gen.send(1)) # Send 1 and get the updated total print(gen.send(3)) # Send 3 and get the updated total
Custom Iterators: You can implement custom iterators by defining a generator function. This allows you to control the iteration logic for your objects.
class MyIterator: def __init__(self, data): self.data = data def __iter__(self): for item in self.data: yield item my_list = [1, 2, 3, 4, 5] custom_iterator = MyIterator(my_list) for item in custom_iterator: print(item)
These are just a few examples of how the
yield
keyword can be used in Python to create more memory-efficient, iterable sequences and solve various programming problems. It's a powerful tool for working with data in a memory-efficient and iterable manner.
Subscribe to my newsletter
Read articles from Divya Mohan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
