Building a React App from Scratch: Creating Your Own File Structure
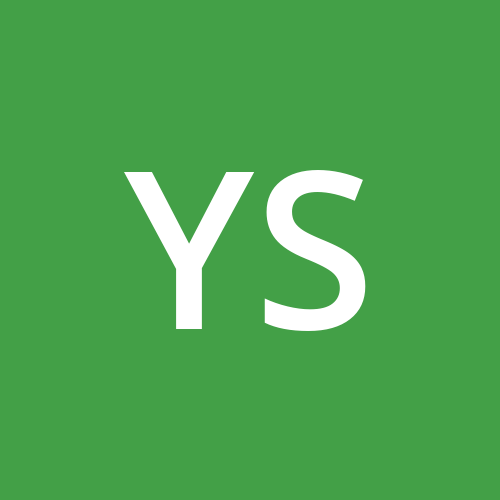
Table of contents
- Introduction
- Prerequisites:
- Step 1: Initialize a New React Project
- Step 2: Install React and ReactDOM
- Step 3: Set Up Your Project Structure
- Step 4: Create the Entry Point
- Step 5: Create Your First Component
- Step 6: Create the App Component
- Step 7: Create the HTML Template
- Step 8: Start Your Development Server
- Conclusion:
Introduction
Creating a React application is often simplified by using tools like "create-react-app." While this tool is fantastic for quick setups, understanding how to build a React app from scratch with your file structure can be immensely beneficial. In this blog post, we will guide you through the process of creating a React app without relying on "create-react-app," allowing you to gain a deeper understanding of the project's structure.
Prerequisites:
Before we begin, make sure you have Node.js and npm (Node Package Manager) installed on your system. You can download them from the official website: Node.js.
Step 1: Initialize a New React Project
To start a React project from scratch, create a new directory for your project and navigate to it in your terminal.
mkdir my-react-app
cd my-react-app
Now, initialize a new npm project by running:
npm init -y
This command will generate a package.json
file that contains metadata about your project and its dependencies.
Step 2: Install React and ReactDOM
Next, you need to install React and ReactDOM as project dependencies. Run the following command:
npm install react react-dom
Step 3: Set Up Your Project Structure
It's time to create the basic structure for your React app manually. You can structure your project in various ways, but here's a common and straightforward approach:
my-react-app/
├── src/
│ ├── index.js
│ ├── App.js
│ ├── components/
│ │ └── MyComponent.js
├── public/
│ ├── index.html
├── package.json
├── package-lock.json
└── .gitignore (if you're using Git)
src: This directory will contain your application's JavaScript files.
public: This directory will contain the HTML file and any other static assets.
Step 4: Create the Entry Point
Inside the src
directory, create an index.js
file. This will serve as the entry point of your application.
// src/index.js
import React from 'react';
import ReactDOM from 'react-dom';
import App from './App';
ReactDOM.render(
<React.StrictMode>
<App />
</React.StrictMode>,
document.getElementById('root')
);
Step 5: Create Your First Component
Now, let's create a simple React component inside the components
directory. This will be the main component of your application.
// src/components/MyComponent.js
import React from 'react';
function MyComponent() {
return (
<div>
<h1>Hello, React!</h1>
</div>
);
}
export default MyComponent;
Step 6: Create the App Component
Inside the src
directory, create an App.js
file. This will be the root component of your application, where you can import and use other components.
// src/App.js
import React from 'react';
import MyComponent from './components/MyComponent';
function App() {
return (
<div>
<h1>My React App</h1>
<MyComponent />
</div>
);
}
export default App;
Step 7: Create the HTML Template
In the public
directory, create an index.html
file. This will be the HTML template for your React app.
<!-- public/index.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>My React App</title>
</head>
<body>
<div id="root"></div>
</body>
</html>
Step 8: Start Your Development Server
To see your React app in action, you can use the webpack-dev-server
along with Babel to transpile your code. First, install the necessary dependencies:
npm install webpack webpack-cli webpack-dev-server babel-loader @babel/core @babel/preset-env @babel/preset-react --save-dev
Next, create a webpack configuration file named webpack.config.js
in the project root directory:
// webpack.config.js
const path = require('path');
module.exports = {
entry: './src/index.js',
output: {
path: path.resolve(__dirname, 'dist'),
filename: 'bundle.js',
},
module: {
rules: [
{
test: /\.(js|jsx)$/,
exclude: /node_modules/,
use: {
loader: 'babel-loader',
},
},
],
},
devServer: {
contentBase: path.join(__dirname, 'public'),
port: 3000,
},
resolve: {
extensions: ['.js', '.jsx'],
},
};
Now, you can start the development server by running:
npx webpack serve --mode development
Visit http://localhost:3000
in your web browser, and you should see your React app up and running!
Conclusion:
Building a React app from scratch provides you with a deeper understanding of how the framework works. While using tools like "create-react-app" is convenient for many projects, knowing how to set up your own file structure and development environment gives you more control and flexibility in your React applications. With this foundation, you can now start building more complex React applications and tailor your project structure to suit your specific needs. Happy coding!
Subscribe to my newsletter
Read articles from Yadvendra Shukla directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
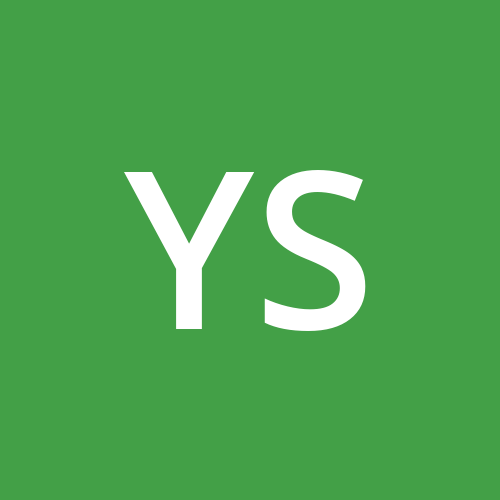
Yadvendra Shukla
Yadvendra Shukla
I am a learner, an explorer, curious about technology, ambitious. web-development and blockchain enthusiast.