Understanding Short-Circuiting in JavaScript
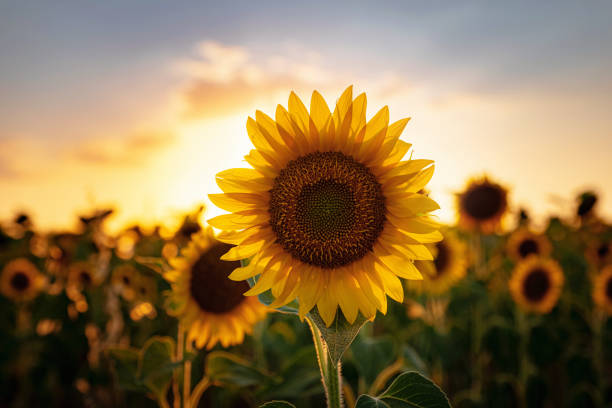
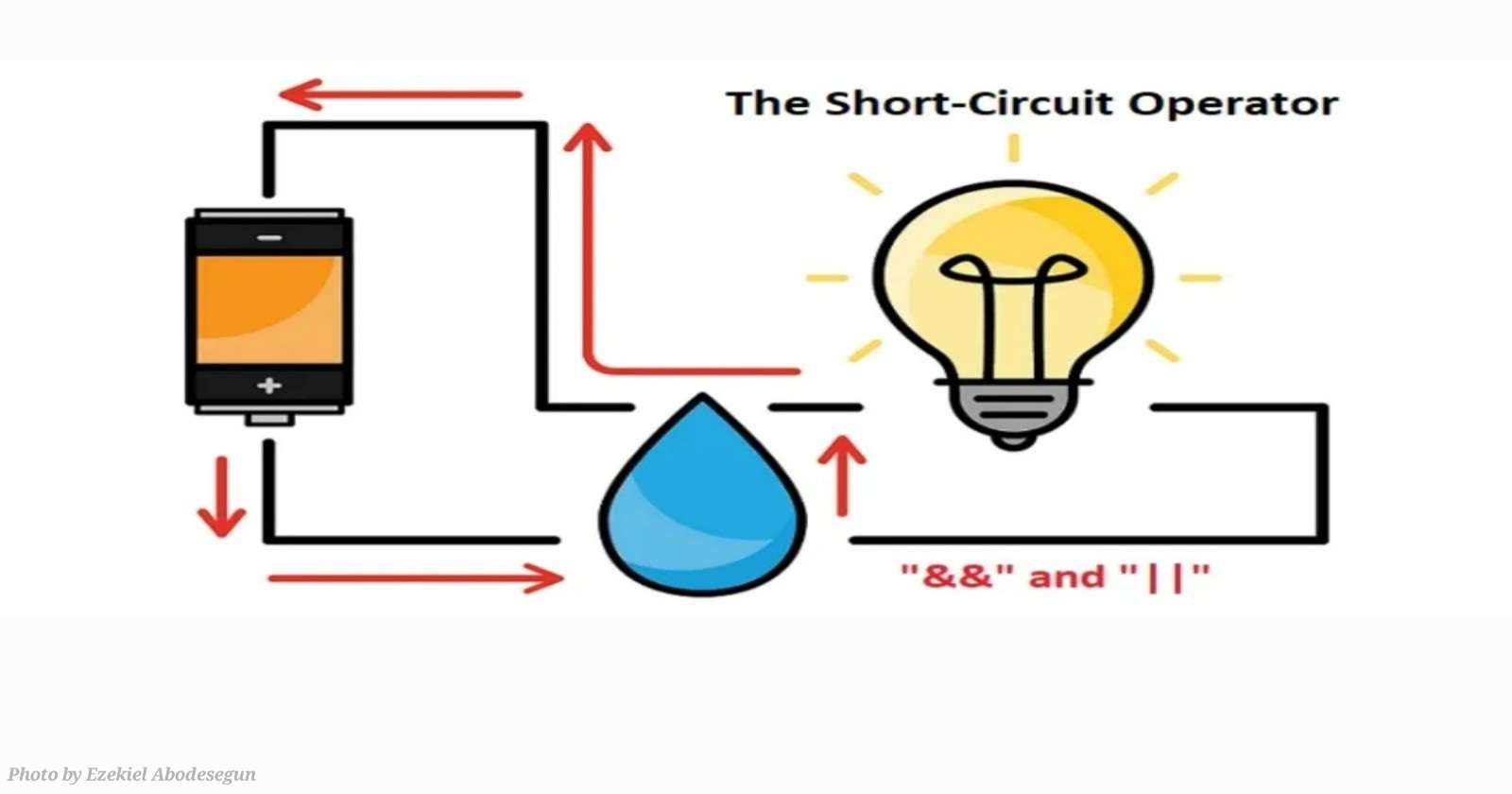
🔥What is Short-Circuiting?
Short-circuiting is a concept related to how JavaScript evaluates logical expressions involving the &&
(logical AND) and ||
(logical OR) operators. These operators are used to combine conditions and make decisions based on them.
When JavaScript encounters a logical expression involving &&
or ||
, it evaluates the expression from left to right. However, it doesn't necessarily evaluate both sides of the expression before determining the result. Instead, it stops as soon as it can determine the outcome based on the values of the operands. Short circuit evaluation avoids unnecessary work, leads to efficient processing, and is often used to write concise and expressive code.
🔥Short-Circuiting with ||
(Logical OR):
The ||
operator returns the first "truthy" value it encounters, or the last value if all operands are "falsy." If the first operand is "truthy," JavaScript does not need to evaluate the second operand because the overall result is already known to be true
.
In JavaScript true || expression
always returns true, it simply doesn’t matter what the value of the expression is, as JavaScript will never even read that side of the Logical OR. But the false || expression
always returns the expression.
Note : In JavaScript, we have 6 falsy values: false, 0 (zero), " ", ' ' (empty string), null, undefined, NaN.
Let's explore with examples:
console.log( 22 || 'Suzan' ) // 22
console.log( '' || 'Zahra' ) // Zahra
console.log( true || 0 ) // true
console.log( undefined || null ) // null
console.log( undefined || 0 || '' || 'Hello' || 23 || null ) // Hello
Use Cases:
Short-circuiting has practical applications that can significantly improve your code's clarity and efficiency. Let's explore some common use cases:
We can provide default values for variables using the ||
operator. If the first operand is falsy, the second operand (default value) is used.
const userName = getUsername() || "Guest";
In this example, if getUsername()
returns a falsy value (e.g., null
or undefined
), the variable userName
will be set to "Guest."
const personInfo = {
name: 'Suzan',
age: 32
}
console.log(personInfo.job || 'unemployed'); // 'unemployed'
Here we want to check if the object personInfo
contains the job key. Since personInfo.job
doesn’t exist, we get undefined
. Since undefined is a falsy value, JavaScript will instead go to the other side of the ||
and accept whatever value is there, (in this example the default string of unemployed
).
🔥Short-Circuiting with &&
(Logical AND):
The &&
operator returns the first "falsy" value it encounters or the last value if all operands are "truthy." If the first operand is "falsy," JavaScript does not need to evaluate the second operand because the overall result is already known to be false
. Let's look at some examples:
console.log(0 && 'Zahra'); // 0
console.log(null && 'Yousra'); // null
console.log(true && undefined ); // undefined
console.log('Apple' && 'Orange'); // Orange
console.log( 'Hello' && true && 11 && false && 23 && null ) // false
Another example, we want to check if an object personInfo
key age
value is higher than 18. And if true, we state that this person is allowed to drive.
const personInfo = {
name: 'Zahra',
age: 15
}
console.log(personInfo.age > 18 && 'Allowed to drive');
Since age
doesn't satisfy the condition, the next condition will not be checked (it Short-Circuits).
If the age satisfies the condition (>18), the next condition will be checked, and the last truthy value will be printed (Allowed to drive).
🔥Conclusion
Understanding short-circuiting in JavaScript can lead to more efficient code and avoid unnecessary evaluations. This feature is a powerful tool in your JavaScript toolkit, allowing you to write code that not only works but also performs efficiently.
Subscribe to my newsletter
Read articles from Yousra Kamal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
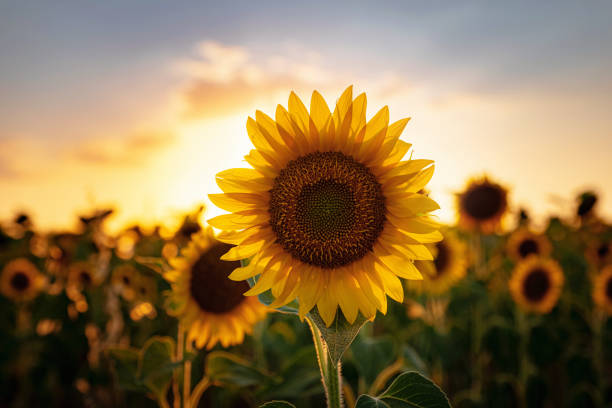
Yousra Kamal
Yousra Kamal
Hi I'm Yousra, a clinical pharmacist and a software developer in the making. I'm self-taught, exploring development world with heavy focus on frontend applications.