Building your first Android widget with Glance
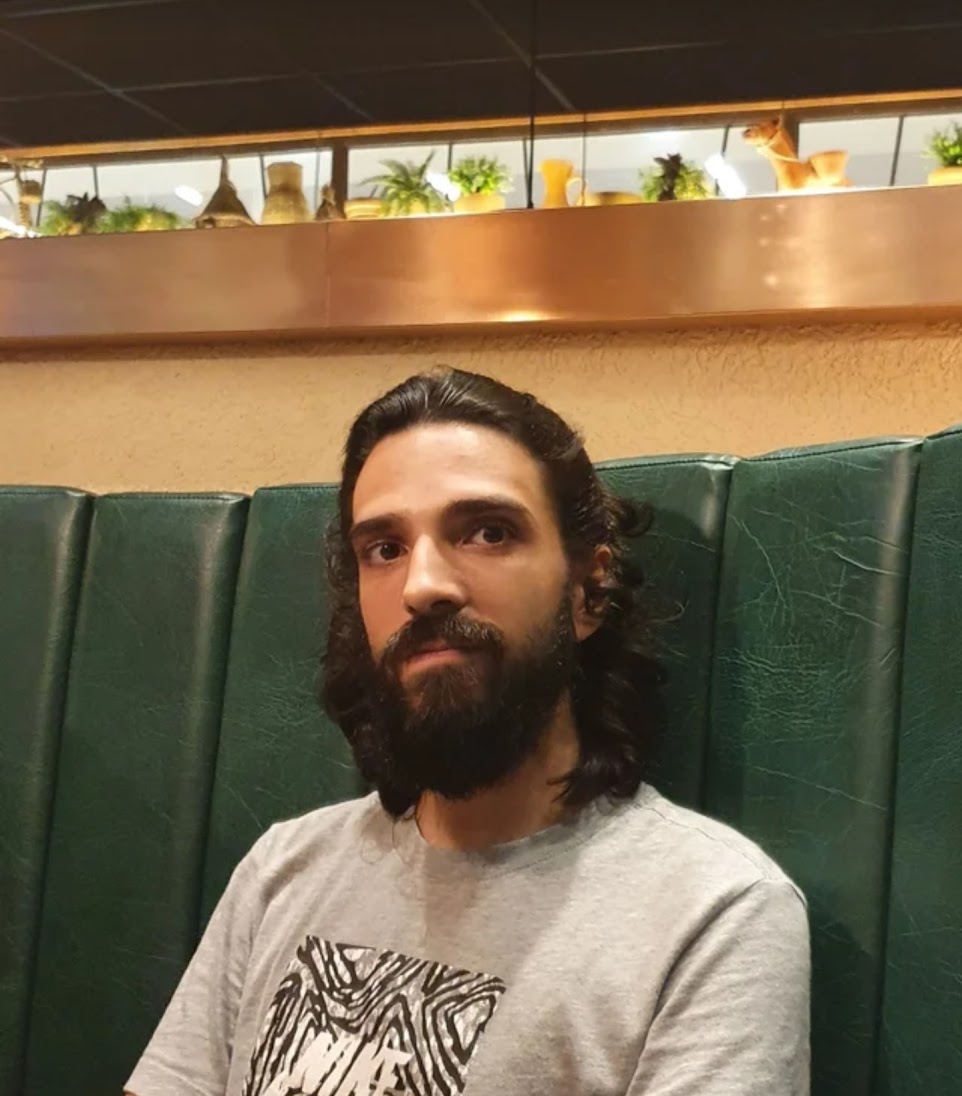
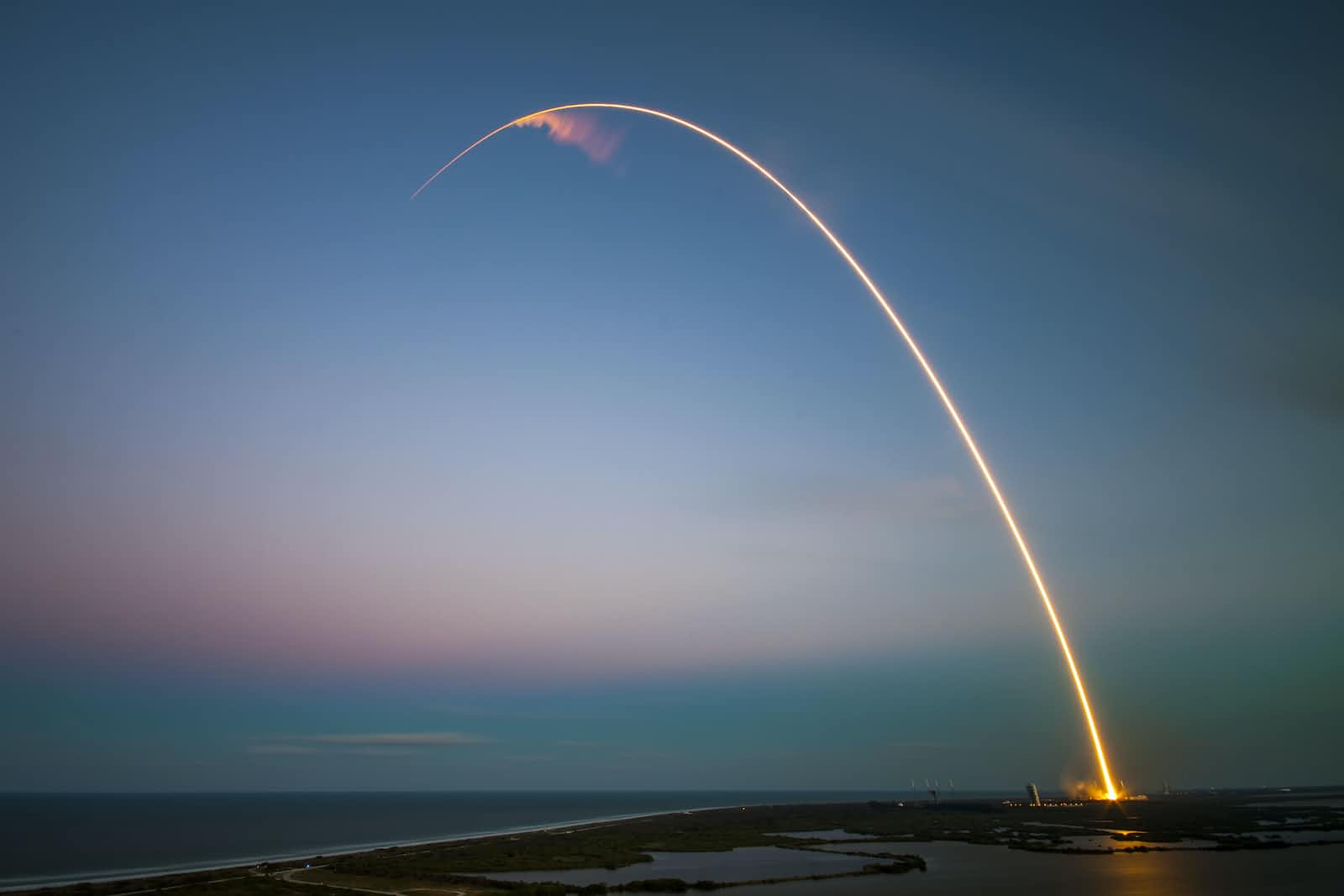
Disclaimer: This post was originally published in 2022 on my previous page that no longer exists.
When Google launched Android Snow Cone 12 one of the main focuses of the OS's version was widgets. As Google defines it widgets are "at-a-glance views of an app's most important data and functionality that are accessible right on the user's home screen". To help us develop widgets Google created Glance a library built on top of Jetpack Compose. So join me as I guide you through your first steps with Glance's. Let's start!
1 - Dependencies
implementation("androidx.glance:glance:1.0.0")
implementation("androidx.glance:glance-appwidget:1.0.0")
2 - Create Widget Provider (xml)
<?xml version="1.0" encoding="utf-8"?>
<appwidget-provider xmlns:android="http://schemas.android.com/apk/res/android"
android:minWidth="150dp"
android:minHeight="150dp"/>
Create this file named app_widget
under res/xml
.
For other properties check the official docs here.
3 - Create Widget Provider (Kotlin)
class MyFirstWidget : GlanceAppWidget() {
override suspend fun provideGlance(context: Context, id: GlanceId) {
provideContent {
MaterialTheme {
Box(
modifier = GlanceModifier
.background(Color.White)
.padding(24.dp)
.clickable(actionStartActivity<MainActivity>())
) {
Text(text = stringResource(id = R.string.widget_action_launch))
}
}
}
}
}
Make sure you use composable views from Glance instead of Compose. It should be androidx.glance.text.Text
instead of androidx.compose.material3.Text
the same is also valid for Box.
This class defines the appearance of our widget and also creates an action of type actionStartActivity
. It does exactly what it says, our widget launches our MainActivity.
Other types of actions include:
actionStartService
actionSendBroadcast
actionRunCallback
You can check the docs for more details here.
4 - Create Widget Receiver
class MyFirstWidgetReceiver : GlanceAppWidgetReceiver() {
override val glanceAppWidget: GlanceAppWidget = MyFirstWidget()
}
5 - Add the widget to Manifest between the <application> tag
<receiver
android:name=".MyFirstWidgetReceiver"
android:exported="true">
<intent-filter>
<action android:name="android.appwidget.action.APPWIDGET_UPDATE" />
</intent-filter>
<meta-data
android:name="android.appwidget.provider"
android:resource="@xml/app_widget" />
</receiver>
It's also important to set your activity's launchMode
to singleTask
to prevent multiple instances of your application.
6 - Run the app and add the widget to the home screen
Once you run the app and add the widget to the device's home screen you'll be able to interact and test it. Clicking it will launch your app's desired activity.
Final thoughts
And that's it! You've now created your first Android widget with Glance.
Subscribe to my newsletter
Read articles from Eduardo Munstein directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
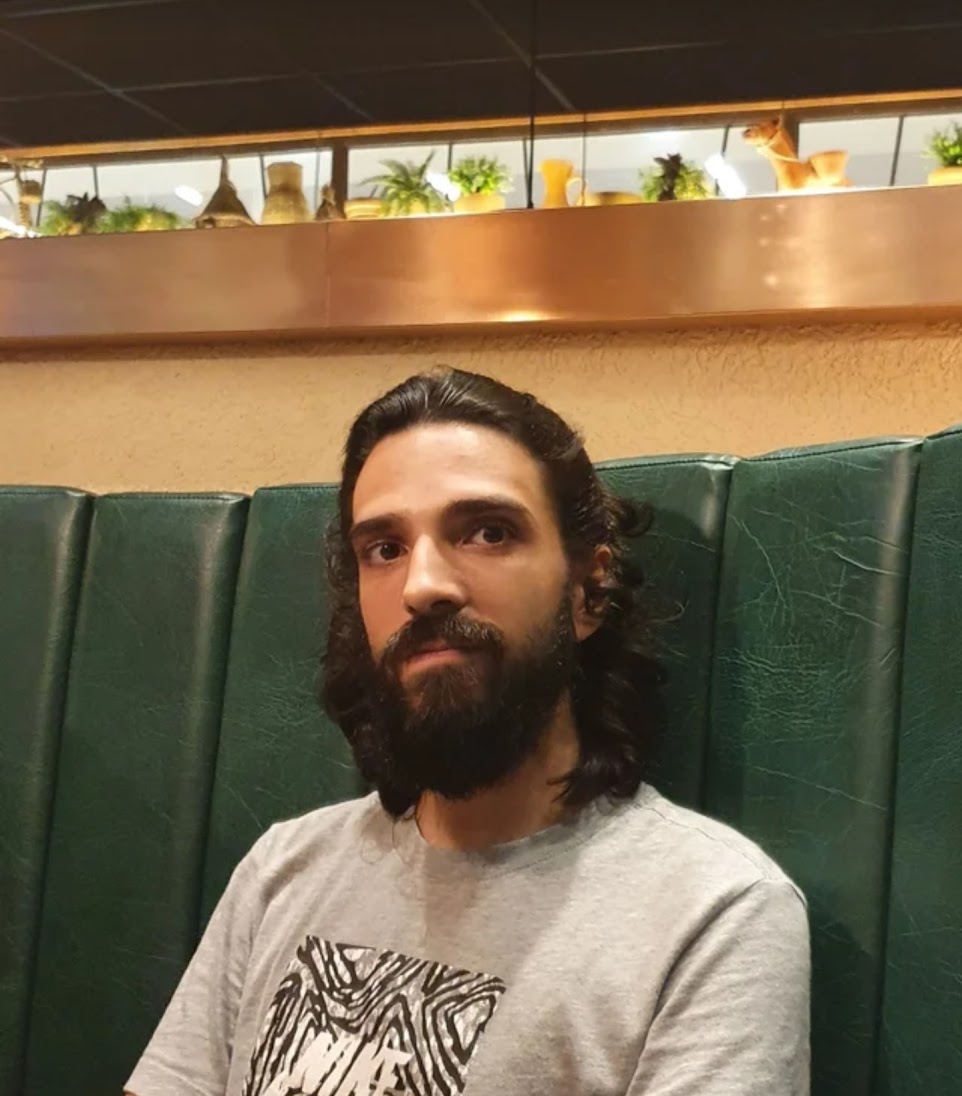