Buttons in Jetpack Compose
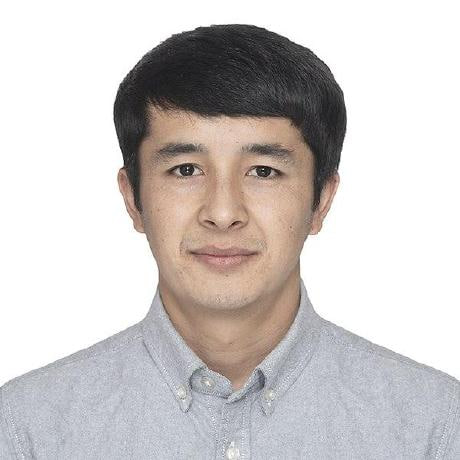
2 min read
In Jetpack Compose buttons, you need to give two arguments for buttons. The first argument as onClick callback and another one is your button text element. You can add a Text-Composable or any other Composable as child elements of the Button
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
Compose_BasicsTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
MainScreen()
}
}
}
}
}
@Composable
fun MainScreen(){
Column(
Modifier.padding(10.dp)
) {
SimpleButton()
ButtonWithColor()
ButtonWithTwoTextView()
ButtonWithIcon()
ButtonWithRectangleShape()
ButtonWithCutCornerShape()
ButtonWithBorder()
ButtonWithElevation()
}
}
@Composable
fun SimpleButton(){
Button(onClick = {
}) {
Text(text = "Simple Button")
}
}
@Composable
fun ButtonWithColor(){
Button(onClick = {
//your onclick code
},
colors = ButtonDefaults.buttonColors(
Color.DarkGray)) {
Text(text = "Button with gray background",
color = Color.White
)
}
}
@Composable
fun ButtonWithTwoTextView(){
Button(onClick = {
//your onClick code here
}) {
Text(text = "Click", color = Color.Magenta)
Text(text = "Here", color = Color.Green)
}
}
//Button With Icon
@Composable
fun ButtonWithIcon(){
Button(onClick = {}){
Image(
painterResource(id = R.drawable.img),
contentDescription = "Cart Button Icon",
modifier = Modifier.size(20.dp)
)
Text(text = "Add to cart",Modifier.padding(start = 10.dp))
}
}
@Composable
fun ButtonWithRectangleShape(){
Button(onClick = { /*TODO*/ },
shape = RoundedCornerShape(20.dp)
) {
Text(text = "Rectangle shape")
}
}
@Composable
fun ButtonWithCutCornerShape(){
//here we use Int,so it will take percentage
Button(onClick = { /*TODO*/ },
shape = CutCornerShape(10)
) {
Text(text = "Cut corner shape")
}
}
@Composable
fun ButtonWithBorder(){
Button(onClick = { /*TODO*/ },
border = BorderStroke(1.dp,Color.Red),
colors = ButtonDefaults.outlinedButtonColors(contentColor =
Color.Red
)
) {
Text(text = "Button with border",
color = Color.DarkGray
)
}
}
@Composable
fun ButtonWithElevation(){
Button(onClick = { /*TODO*/ },
elevation = ButtonDefaults.elevatedButtonElevation(
defaultElevation = 10.dp,
pressedElevation = 10.dp,
disabledElevation = 10.dp
)) {
Text(text = "Button with elevation")
}
}
@Preview(showBackground = true)
@Composable
fun GreetingPreview() {
Compose_BasicsTheme {
MainScreen()
}
}
0
Subscribe to my newsletter
Read articles from Oybek Kholiqov directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
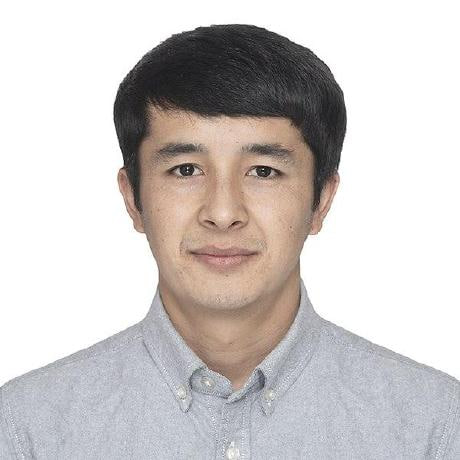
Oybek Kholiqov
Oybek Kholiqov
I am Android Developer from Uzbekistan