Building an E-commerce System: A Comprehensive Code Breakdown - Part 4
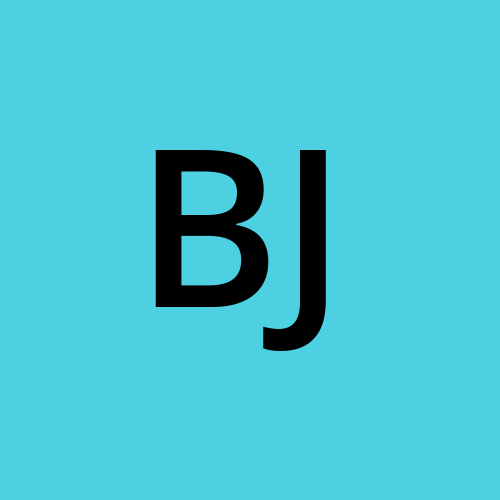
Welcome to the fourth installment of our exploration of the codebase for our e-commerce system. In this part, we'll delve into user management, user roles, and access control, which are crucial for maintaining a secure and organized e-commerce platform.
Part 4: User Management and Access Control
User management plays a pivotal role in an e-commerce system. It encompasses user registration, authentication, authorization, and defining user roles. Our codebase effectively handles these aspects, ensuring a seamless and secure user experience.
User Class and User Roles
In our codebase, the User
class represents a user in the system. Users can have different roles, such as CUSTOMER
or STAFF
, which determine their permissions and access levels within the platform.
enum UserAccessLevel {
CUSTOMER, STAFF
}
class User {
private String username;
private String password;
private String location;
private List<Order> orderHistory;
private UserAccessLevel accessLevel;
public User(String username, String password, String location) {
this.username = username;
this.password = password;
this.location = location;
this.orderHistory = new ArrayList<>();
this.accessLevel = UserAccessLevel.CUSTOMER;
}
// Getter methods and other functionality...
}
Explanation:
The
User
class stores user information, including their username, password, location, order history, and access level.UserAccessLevel
is an enum representing different user roles.
User Registration and Authentication
User registration is a fundamental step in our e-commerce system. Users can create accounts, providing essential information such as their username, password, and location. Authentication ensures that users are who they claim to be before granting access to specific features.
In our codebase, user registration and authentication are handled at the application level, often involving interactions with a database. These components are typically not shown in the provided code snippets but are essential for a complete system.
Authorization and Access Control
Authorization and access control define what users can and cannot do within the system based on their roles. In our codebase, access control is exemplified by the UserAccessLevel
enum. For instance, a user with the STAFF
role might have access to administrative functions that a regular customer does not.
if (user.getAccessLevel() == UserAccessLevel.STAFF) {
// Grant access to staff-specific functionality
} else {
// Restrict access
}
Explanation:
Authorization checks are performed based on the user's access level.
Different actions and functionality can be made available or restricted accordingly.
Order History
A crucial feature of our codebase is the ability to track a user's order history. This is managed within the User
class, where a list of orders is associated with each user.
public List<Order> getOrderHistory() {
return orderHistory;
}
Explanation:
- The
getOrderHistory
method allows users to retrieve their order history.
Key Takeaways:
User management involves registration, authentication, and user roles.
Authorization and access control ensure that users have appropriate permissions.
Order history tracking enhances the user experience.
In Part 4, we've explored the critical aspects of user management, roles, and access control within our e-commerce system. In Part 5, we'll wrap up our code breakdown series by discussing the final components and highlighting the importance of testing and maintenance.
We've now covered Part 4 of our comprehensive code breakdown series. If you have any questions or suggestions, please feel free to share them. In Part 5, we'll conclude our exploration of the e-commerce system codebase.
Subscribe to my newsletter
Read articles from Bryan Samuel James directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
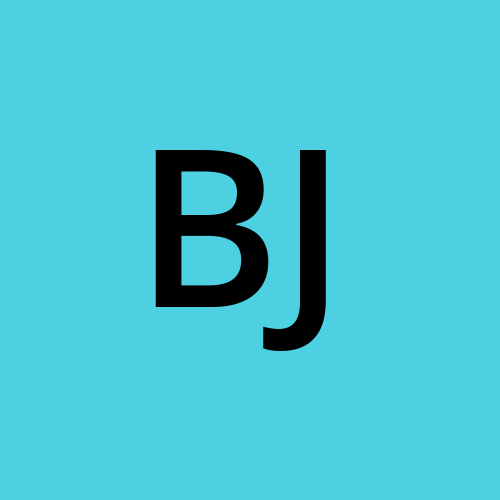