How to Convert ChartModule to Spectator for Easy Testing
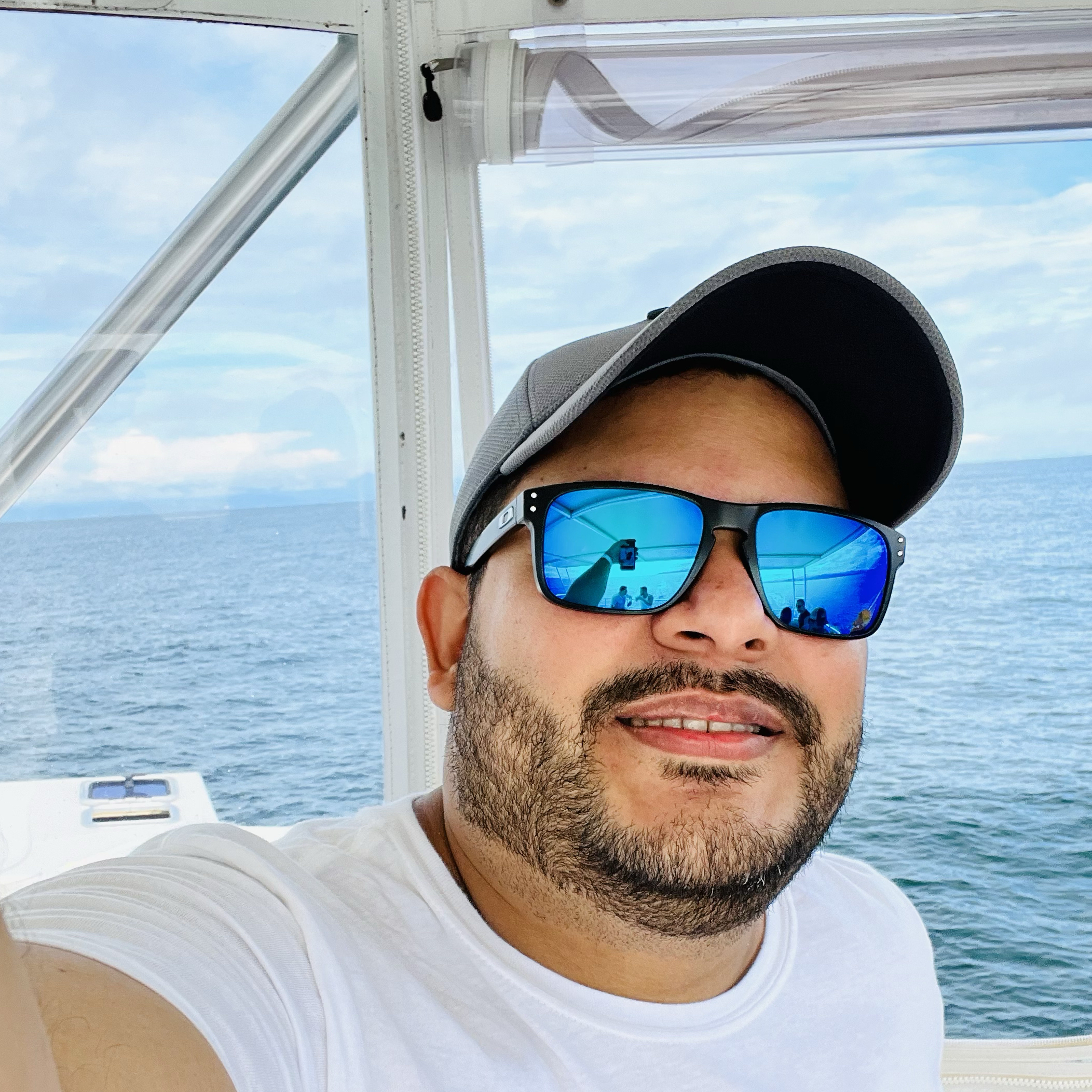
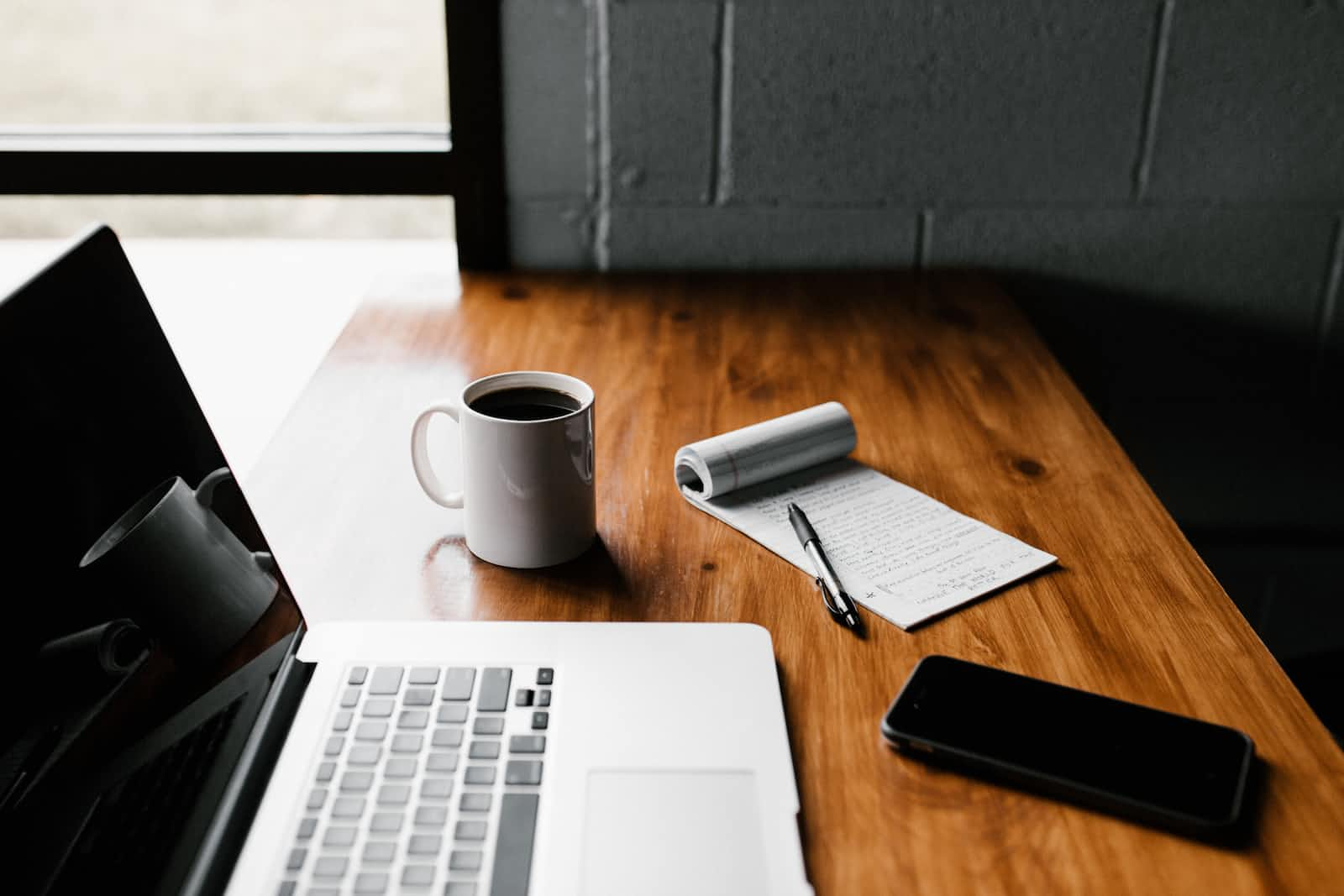
Recently, we migrated some libraries to Jest and encountered issues with the tests we had created for a component that used the PrimeNg ChartModule (which, is a wrapper for ChartJS).
At first glance, we identified that the issue stemmed from Jest's lack of support for Canvas
, MutationObserver
, and other browser APIs necessary to run the tests (Karma uses a real browser to run the test, but Jest does not).
Finally, we realized that we had two options: either mock the browser APIs or mock the ChartModule
. Since we were using Spectator for testing, we managed to do this easily (after much searching, test, and error).
Firstly, we created a mock to replace what we needed. In this case, since PrimeNG still uses modules, we created a component and a test module specifically for this purpose.
// Component containing chart.js in PrimeNG's ChartModule
@Component({
selector: 'p-chart',
template: 'PrimeNg Chart - mocked component'
})
class MockUIChart {
// Here we add all the inputs that we are using
@Input()
data: unknown;
@Input()
options: unknown;
@Input()
plugins: unknown;
}
// Module that imports and exports the component
@NgModule({
declarations: [MockUIChart],
exports: [MockUIChart]
})
export class MockChartModule {}
In our test, we do the following:
describe('ComponentStandalone', () => {
let spectator: Spectator<ComponentStandalone>;
const createComponent = createComponentFactory({
component: ComponentStandalone,
overrideComponents: [
[
ComponentStandalone,
{
remove: { imports: [ChartModule] },
add: { imports: [MockChartModule] }
}
]
]
});
});
With this, we prevent the p-chart
component from attempting to instantiate ChartJS in the ngAfterViewInit
, which was the root cause of all the Jest warnings.
Do you have any experience with Jest
, PrimeNG, or testing tools that you'd like to share? Leave your comments below!
Subscribe to my newsletter
Read articles from Arcadio Quintero directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
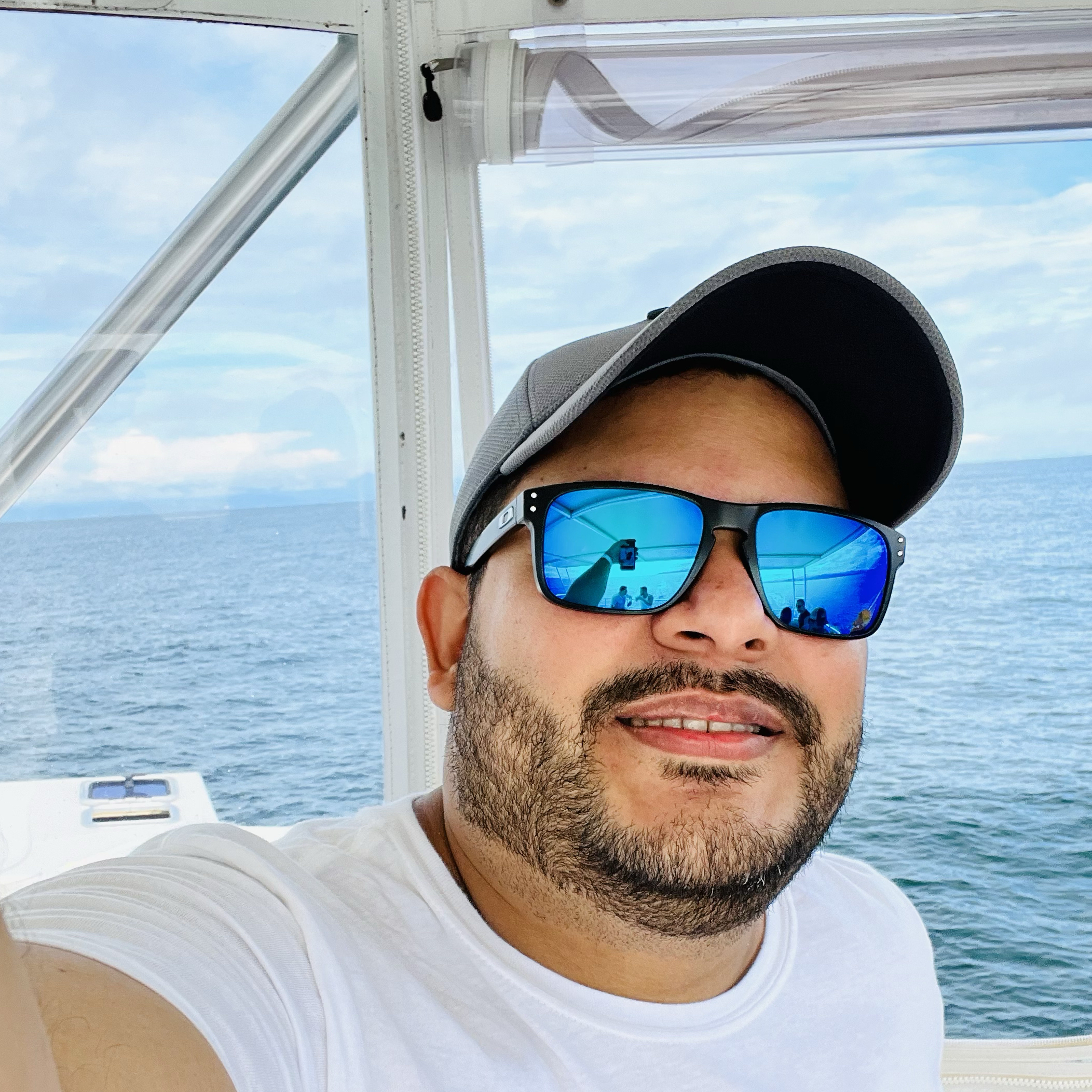
Arcadio Quintero
Arcadio Quintero
Frontend developer at @dotCMS โข Angular Developer โข System Engineer โข ๐ Passionate software engineer from Panama ๐ต๐ฆ located in Ontario, Canada ๐จ๐ฆ , with over 16 years of experience in web development. ๐ป I've worked extensively with various programming languages such as PHP, Typescript, and JavaScript. I have deep knowledge of frameworks like Angular, NestJS, and CakePHP, leveraging their power to create robust and scalable applications. ๐ I'm passionate about continuous learning and staying updated with the latest trends in the ever-evolving field of software development.