Day 29: Jenkins Important Interview Questions

Table of contents
- What’s the difference between continuous integration, continuous delivery, and continuous deployment?
- Continuous Integration (CI):
- Continuous Delivery (CD):
- Continuous Deployment (CD):
- Benefits of CI/CD
- What is Jenkins Pipeline?
- How do you configure the job in Jenkins?
- Where do you find errors in Jenkins?
- 1. Console Output:
- 2. Build History:
- 3. Build Status:
- In Jenkins how can you find log files?
- Jenkins workflow and write a script for this workflow?
- How to create continuous deployment in Jenkins?
- 1. Install Necessary Plugins:
- 2. Configure Source Code Management (SCM):
- 3. Set Up Build Triggers:
- 4. Define Build Steps:
- 5. Configure Deployment Steps:
- 6. Environment-Specific Configurations:
- 7. Post-Build Actions:
- 8. Testing and Rollback Strategies:
- 9. Secure Your Pipeline:
- 10. Save and Trigger Your Pipeline:
- How build job in Jenkins?
- Is Only Jenkins enough for automation?
- How will you handle secrets?
- Explain diff stages in CI-CD setup
- Name some of the plugins in Jenkins?
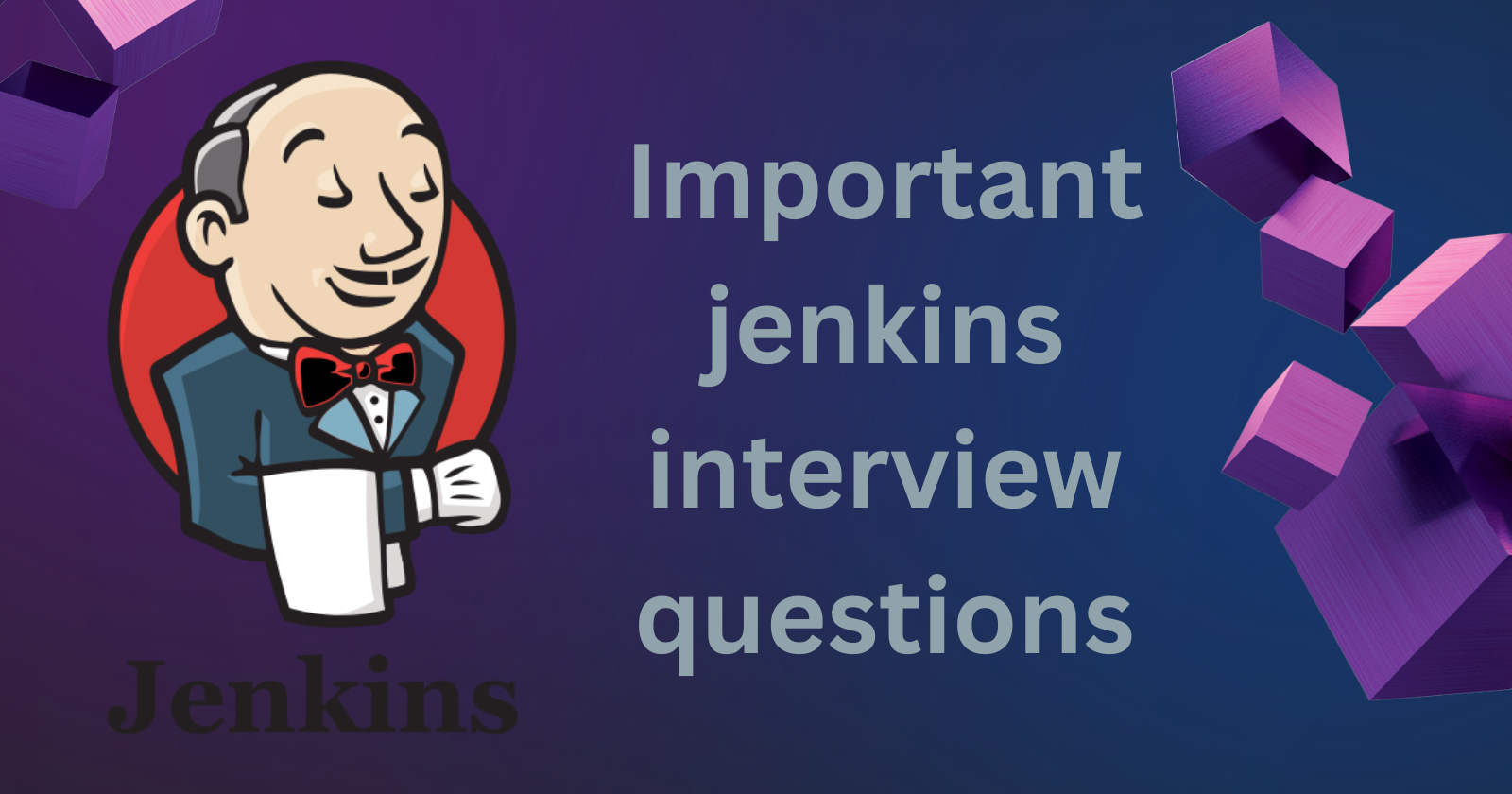
What’s the difference between continuous integration, continuous delivery, and continuous deployment?
Continuous Integration (CI):
CI is a development practice that requires developers to integrate code into a shared repository several times a day. Each check-in is then verified by an automated build, allowing teams to detect problems early.
Continuous Delivery (CD):
CD is an extension of CI that ensures that code changes can be built, tested, and prepared for release reliably and in a sustainable way. While the code is automatically built, tested, and validated, it doesn't necessarily mean it's deployed to production.
Continuous Deployment (CD):
CD is an advanced step of CI/CD where every change that passes automated tests is automatically deployed to production without manual intervention. This approach is often used in web applications, where new features and bug fixes are continuously and automatically deployed to users.
Benefits of CI/CD
Continuous Integration (CI) and Continuous Deployment (CD) offer numerous benefits in the software development lifecycle. Here are some of the key advantages:
Faster Development Cycles
Early Issue Detection
Higher Code Quality
Reduced Manual Errors
Improved Collaboration
Faster Time to Market
Easier Rollbacks
Resource Efficiency
Continuous Feedback Loop
Compliance and Security
What is Jenkins Pipeline?
Jenkins Pipeline is a suite of plugins that supports implementing and integrating continuous delivery pipelines into Jenkins. A Jenkins Pipeline allows you to define an entire application lifecycle, which includes building, testing, and deploying your code. The pipeline is typically defined in a text file called a Jenkinsfile, which contains the entire pipeline script.
How do you configure the job in Jenkins?
Configuring a Freestyle Project Job:
Log In to Jenkins:
- Open your web browser and navigate to the Jenkins web interface. Log in with your credentials.
Create a New Job:
Click on "New Item" or "Create New Jobs" on the Jenkins dashboard.
Enter a name for your job and select "Freestyle project," then click on "OK."
General Settings:
Provide a description for your job (optional).
Specify the source code management system (e.g., Git, Subversion) and configure the repository details if applicable.
Build Triggers:
Choose how you want your job to be triggered:
Poll SCM: Jenkins checks your version control system at specified intervals and triggers the build if changes are detected.
Build after other projects are built: Trigger this job after specific jobs have been built.
Build Environment (Optional):
- Configure any build environment settings, such as providing environment variables, setting up timestamps, or configuring build discard options.
Build:
Specify the build steps. For example:
Execute Shell: If your job involves shell commands, scripts, or build commands.
Invoke Ant: If your project is built using Apache Ant.
Invoke Gradle script: If your project uses Gradle for building.
Windows Batch Command: If your job needs to run Windows batch commands.
Post-Build Actions:
Define actions to be performed after the build is completed:
Archive the artifacts: Specify files and directories to be archived for future reference.
Send build artifacts over SSH: Transfer build artifacts to a remote server using SSH.
Publish JUnit test result report: If your build produces JUnit test results, publish the results for analysis.
Save and Apply Changes:
- Click on "Save" or "Apply" to save your job configuration.
Build Job:
- You can manually trigger the job by clicking on "Build Now." Alternatively, if you've set up triggers, the job will automatically run based on the specified triggers.
Where do you find errors in Jenkins?
In Jenkins, errors and issues can occur at various stages of the build and deployment process. Here are common places where you can find errors and logs related to Jenkins jobs:
1. Console Output:
When a Jenkins job runs, you can view the real-time console output. This is where you'll find detailed information about each step of the build process, including any errors or warnings that occurred. To access the console output, go to the specific build of your job and click on "Console Output."
2. Build History:
The build history provides a list of all builds for a particular job. Each build entry shows whether the build was successful, unstable, or failed.
3. Build Status:
The main dashboard of Jenkins displays the status of all jobs. If a job fails, it is usually indicated with a red or yellow icon. Clicking on the job will take you to the build details, including the console output.
In Jenkins how can you find log files?
Jenkins logs are stored in the jenkins.log file. The location of this file hinges on the Jenkins installation and operating system.
Common destinations include /var/log/Jenkins/jenkins.log (Linux)
Jenkins workflow and write a script for this workflow?
pipeline {
agent any
stages {
stage('Build') {
steps {
//
}
}
stage('Test') {
steps {
//
}
}
stage('Deploy') {
steps {
//
}
}
}
}
How to create continuous deployment in Jenkins?
1. Install Necessary Plugins:
Install plugins required for your deployment process. For example, if you're deploying to a web server, you might need plugins for SSH, SCP, or container orchestration tools like Docker and Kubernetes.
2. Configure Source Code Management (SCM):
In your Jenkins job configuration, specify the URL of your version control repository (e.g., Git). Configure credentials if needed. Jenkins will pull the latest code from this repository.
3. Set Up Build Triggers:
Configure build triggers. For continuous deployment, you might want to use triggers like "Poll SCM" or webhooks (for repositories that support them). This ensures that Jenkins automatically starts the deployment process whenever there are new code changes.
4. Define Build Steps:
Define the build steps to prepare your application for deployment. These steps can include compiling code, running tests, and creating artifacts. Use build tools like Maven, Gradle, or npm as needed.
5. Configure Deployment Steps:
Add deployment steps to your Jenkins job. The configuration of these steps depends on your deployment target (e.g., web server, container orchestrator, etc.):
SSH Deployment: If deploying to a server via SSH, use SSH plugins to copy files and execute commands remotely.
Docker Deployment: If your application is packaged in containers, use Docker plugins to build and push images, and orchestration tools like Kubernetes for container deployment.
Other Deployment Tools: If you're using specific deployment tools (e.g., Ansible, AWS Elastic Beanstalk), there might be Jenkins plugins available to integrate them into your pipeline.
6. Environment-Specific Configurations:
- If your application requires environment-specific configurations (e.g., database connection strings, API endpoints), manage these configurations separately for each environment. Jenkins allows you to parameterize builds to handle different environment variables dynamically.
7. Post-Build Actions:
- Define post-build actions, such as sending notifications, updating dashboards, or triggering additional processes in response to successful deployment.
8. Testing and Rollback Strategies:
- Implement testing steps in your deployment pipeline. This can include automated tests, manual QA, or even canary releases to a subset of users. Additionally, plan for rollback strategies in case the deployment fails or introduces critical issues.
9. Secure Your Pipeline:
- Ensure that your Jenkins pipeline and deployment process are secure. Use credentials securely, limit access to Jenkins jobs, and follow security best practices for your deployment targets.
10. Save and Trigger Your Pipeline:
- Save your Jenkins job configuration and manually trigger the build to test your continuous deployment pipeline. Verify that the code is pulled, built, and deployed successfully.
How build job in Jenkins?
To build a job in Jenkins, follow these general steps:
1. Log in to Jenkins and navigate to the job that you want to build.
2. Click on “Build Now” to start a new build of the job.
3. Monitor the build progress in the build queue and in the build log, which shows the output of the build commands.
4. Once the build is complete, review the build results, which include information about the status of the build, any test results, and any artefacts that were generated.
Is Only Jenkins enough for automation?
Jenkins is a popular tool for automation, but it may not be enough for all automation needs, depending on the complexity of your software applications and your organization’s specific requirements. Other tools, such as Ansible and Puppet, may be needed to automate additional aspects of the software development lifecycle.
How will you handle secrets?
To handle secrets in Jenkins, you can use the Jenkins Credentials Plugin to securely store and manage sensitive information, such as passwords and API keys. It stores confidential data.
Explain diff stages in CI-CD setup
The stages in a typical CI-CD setup could include:
Code commit
Build
Test
Deploy to staging
Test in staging
Deploy to production
Name some of the plugins in Jenkins?
Github plugin- The Git Plugin integrates Jenkins with Git version control systems.
Blueocean plugin - It provides a visual representation of pipelines, making it easier to create, visualize, and understand complex CI/CD workflows.
Artifactory plugin- This plugin integrates Jenkins with JFrog Artifactory, a binary repository manager.
Docker Plugin- The Docker Plugin enables Jenkins to build, package, and distribute Docker containers.
Sonarqube plugin - The SonarQube Scanner plugin integrates Jenkins with SonarQube, a static code analysis tool.
These are some Jenkins questions that will help in cracking the interview. Hope these questions you will find useful and worthy.
Thankyou for reading this article!
Subscribe to my newsletter
Read articles from Moiz Asif directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
