Discover Incredible Tricks with JavaScript
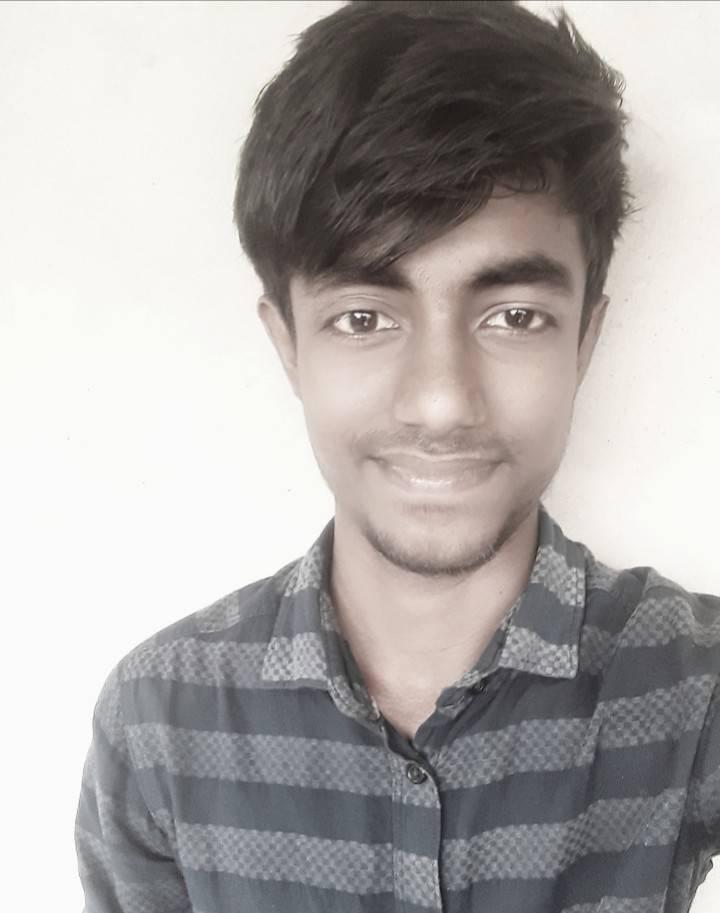
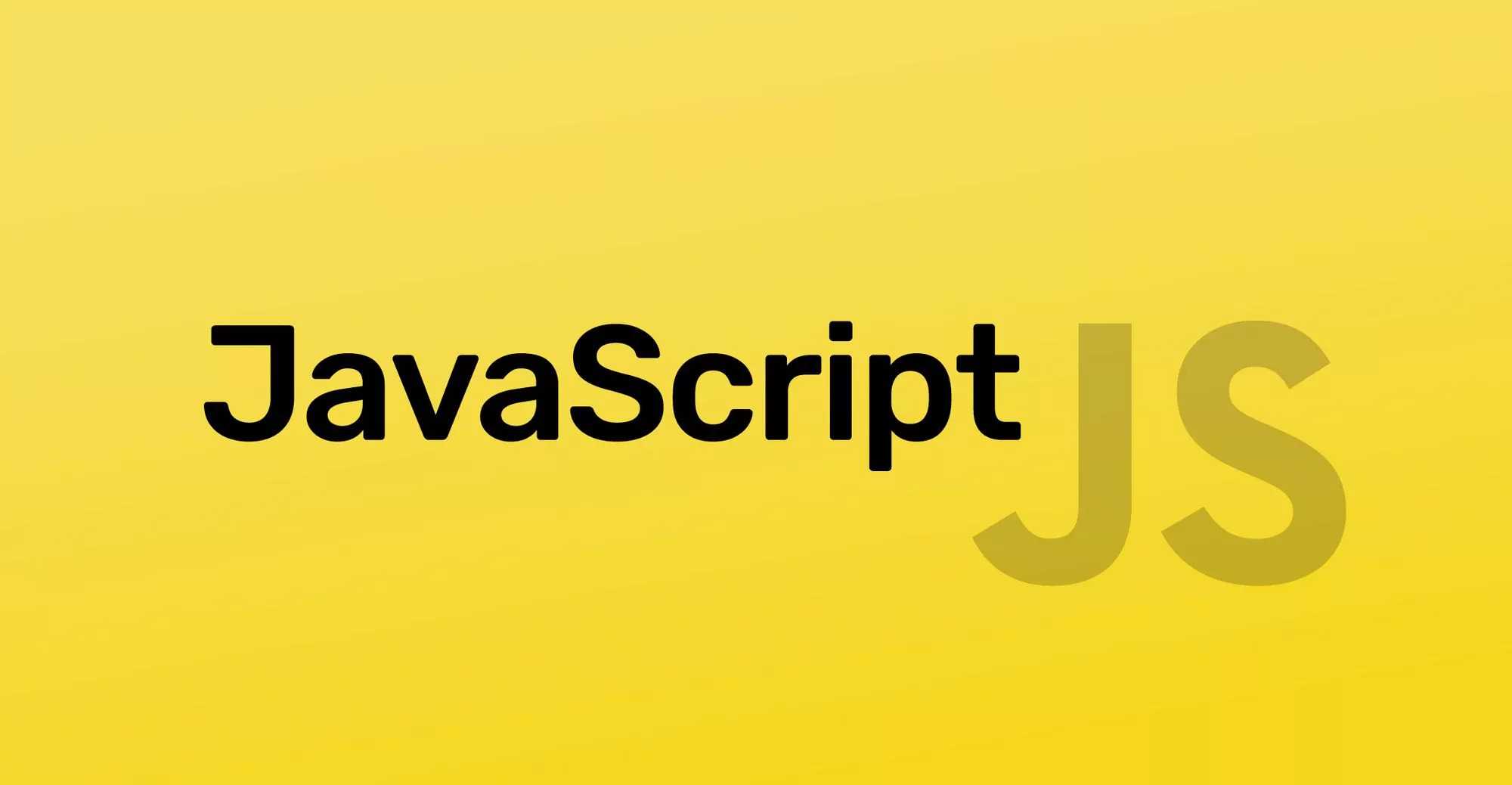
Within the scope of this article, you will be presented with some straightforward and impressive JavaScript techniques that will elevate the quality of your code.
Tip 1: Combining multiple objects together.
Consider you have three different objects:
// Jahid Hasan | www.jahid.me
const obj1 = {'a': 1, 'b': 2};
const obj2 = {'c': 3};
const obj3 = {'d': 4};
If we want to combine the properties of multiple objects, we can use the following code:
// Jahid Hasan | www.jahid.me
const objCombined = {...obj1, ...obj2, ...obj3};
Logging objCombined
will print the following value in the console:
The …
is the spread operator. You can read more about this in the MDN Web Docs
The object objCombined
is a newly created object. Updating any value of obj1
, obj2
, or obj3
will not affect the values of objCombined
.
When you have objects inside other objects, using spread syntax will duplicate the inner objects by reference, not create new ones. Spread syntax copies all the properties of the object, but it only creates a new object at the top level.
You can also combine objects using the Object.assign()
method.
Tip 2: How to insert values in between an array.
Imagine that you have an array of integers:
// Jahid Hasan | www.jahid.me
const arr = [0, 1, 2, 3, 5, 6, 7, 8];
How can we insert the integer 4 at index 4 of an array?
We can use the splice
function in Array's prototype. The syntax is:
// Jahid Hasan | www.jahid.me
arr.splice(index, itemsToDelete, item1ToAdd, item2ToAdd, ...);
To insert the integer 4 at index 4, we write the code:
// Jahid Hasan | www.jahid.me
arr.splice(4, 0, 4);
The array will be updated to:
We can also write code to insert multiple integers at an index, like this:
// Jahid Hasan | www.jahid.me
arr.splice(4, 0, 100, 101, 102, 103, 104, 105);
The original array will be updated to:
It's important to note that when using the splice
function, the original array will be modified instead of creating a new one.
Want to learn more about
splice
function? Read more about it in the following article:
Tip 3: Obtain the present time stamp.
To obtain the current timestamp in JavaScript, execute this code:
// Jahid Hasan | www.jahid.me
var date = new Date();
date.getTime();
This will give the timestamp for the date.
There is also a shortcut to get the current timestamp.
new Date()
or Date.now
()
Tip 4: Verify if an item is an array.
To determine if an object is an array, use the isArray()
method.
// Jahid Hasan | www.jahid.me
const obj = {data: 1};
const arr = [1, 2, 3];
To check for an array, use the following code snippet:
// Jahid Hasan | www.jahid.me
Array.isArray(obj); // false
Array.isArray(arr); // true
Tip 5: Utilizing Object Destructuring.
Imagine that you have the following JavaScript object:
const user = {
name: 'Jahid Hasan',
age: 20,
country: 'Bangladesh',
website: 'https://jahid.me',
};
To obtain the variables for an object's properties, use the following syntax directly:
const { name, website, country} = user;
console.log(name); // Jahid Hasan
console.log(country); // Bangladesh
console.log(website); // https://jahid.me
After executing the statement, 3 variables will contain the object's properties.
For this to work properly, the variable names on the left side must exactly match the key names of the object.
Object Destructuring is the name of the syntax.
Tip 6: Using Rest Parameter Syntax.
Did you know you can make a function that can take any number of arguments?
To use the rest parameter syntax, add three dots before the parameter name in the function's definition. This creates an array of arguments that can be easily modified within the function.
You can use the rest parameter syntax to create a function like that.
// Jahid Hasan | www.jahid.me
function sum(...values) {
console.log(values);
}
sum(1);
sum(1, 2);
sum(1, 2, 3);
sum(1, 2, 3, 4);
When you call the sum
function, it gathers the values
into an array from the parameters you passed to the function.
This will display the following result.
We can enhance the sum
function to calculate the total sum of all the parameters you pass to it.
// Jahid Hasan | www.jahid.me
function sum(...values) {
let sum = 0;
for (let i = 0; i < values.length; i++) {
sum += values[i];
}
return sum;
}
console.log(sum(1)); // 1
console.log(sum(1, 2)); // 3
console.log(sum(1, 2, 3)); // 6
console.log(sum(1, 2, 3, 4)); // 10
Tip 7: String Pad in JavaScript.
To pad a string, provide the desired string and length. Use padStart for beginning padding and padEnd for end padding.
You can easily pad strings to a desired length using these methods.
// Jahid Hasan | www.jahid.me
const str = '5';
console.log( str.padStart(2, '0') ); // '05'
console.log( str.padEnd(5, 'X') ); // '5XXXX'
We can also use it to display masked numbers.
// Jahid Hasan | www.jahid.me
const bankNumber = '2222 2222 2222 2222';
const last4Digits = bankNumber.slice(-4);
last4Digits.padStart(bankNumber.length, '*');
// ***************2222
Tip 8: Understanding JavaScript currying.
Currying means evaluating functions with multiple arguments and breaking them down into a sequence of functions with a single argument.
First, we are going to create a simple function that accepts three parameters.
// Jahid Hasan | www.jahid.me
const add =(a, b, c) => return a+b+c ;
console.log(add(2, 3, 5)); // 10
Let's test the curry function implementation by accepting one argument and returning a series of functions.
// Jahid Hasan | www.jahid.me
const addCurry = (a) => {
return (b) => {
return (c) => {
return a+b+c ;
}
}
}
This is the implementation of the curry function. If we execute it, the output will be 10
console.log( addCurry(2)(3)(5) ); // 10
In the first example, we created a function
add
that accepted three argumentsa
,b
, andc
, added their suma+b+c
, (2)+(3)+(5), and returned the output as10
In the second example, we used a curried function that takes one argument
a
and returns a function that takes another argumentb
. The final function takes another argumentc
and returns the sum of all three arguments, resulting in an output of10
.
What we have done here is a nested function, so each of these functions takes one argument that returns another argument and the function doesn’t complete until it receives all parameters.
Tip 9: Learn about the ternary operator in JavaScript.
In JavaScript, we have the option to use a ternary conditional operator to condense our if-else statements.
// Jahid Hasan | www.jahid.me
const age = 10;
let group;
// LONG VERSION
if (age >= 18) {
group = "Adult" ;
} else
{
group = "Child" ;
}
// SHORTHAND VERSION
group = age > 18 ? "Adult" : "Child" ;
console.log(group); // Child
Remember, this shorthand is meant to make the code cleaner. Use it only when it clearly makes the expression more understandable.
Tip 10: JavaScript async await defined
To start, let's get a working definition of the async and await JavaScript keywords:
async: Declares a function as one that will contain the await keyword within it.
await: Consumes an asynchronous function that returns a promise with synchronous syntax.
Let's try an example code.
// Jahid Hasan | www.jahid.me
async function getStarWarsData() {
try {
const response = await fetch('https://swapi.dev/api/people/1/');
const data = await response.json();
console.log(data);
} catch (error) {
console.error(error);
}
}
getStarWarsData();
// Returns: {name: 'Luke Skywalker', height: '172', mass: '77', hair_color: 'blond', skin_color: 'fair', …}
Notice that you can get hold of the promise returned by a method like fetch()
; in this case, the response object. response.json()
is also an asynchronous method returning a promise, so we again use await
.
Our getStarWarsData()
function must be prefixed with the async
keyword— otherwise, the browser will not allow await
in its body.
Need some JavaScript work to be done? Contact with me derect: Email
...................... Follow me on HashNode @Jahid Hasan ..................
Connect with me on LinkedIn: https://www.linkedin.com/in/jahidorjahid/
Subscribe to my newsletter
Read articles from Jahid Hasan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
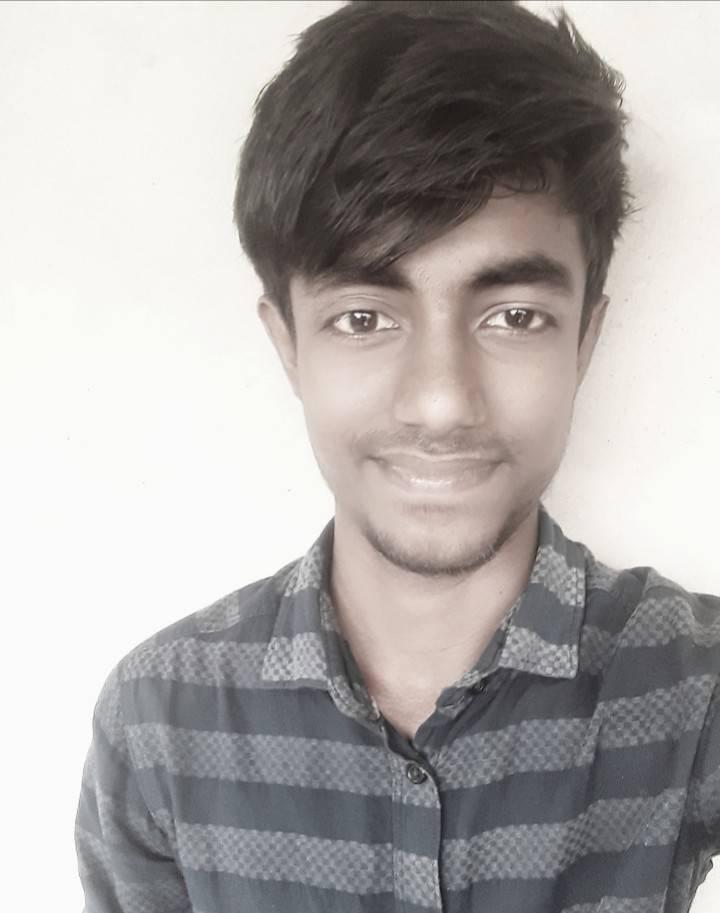
Jahid Hasan
Jahid Hasan
🚀 Crafting digital dreams with Node.js! 🌟 As a code magician, I turn caffeine into code and errors into experiences. 💻 Building robust backends, I make data dance and websites waltz. When I'm not coding, I'm exploring the latest tech trends or chasing sunsets on two wheels. Let's turn your ideas into reality, one line of code at a time. 🌐 #NodeNinja #CodeCreator #WebWizard"