Graph PowerShell Authentication with Managed Identities

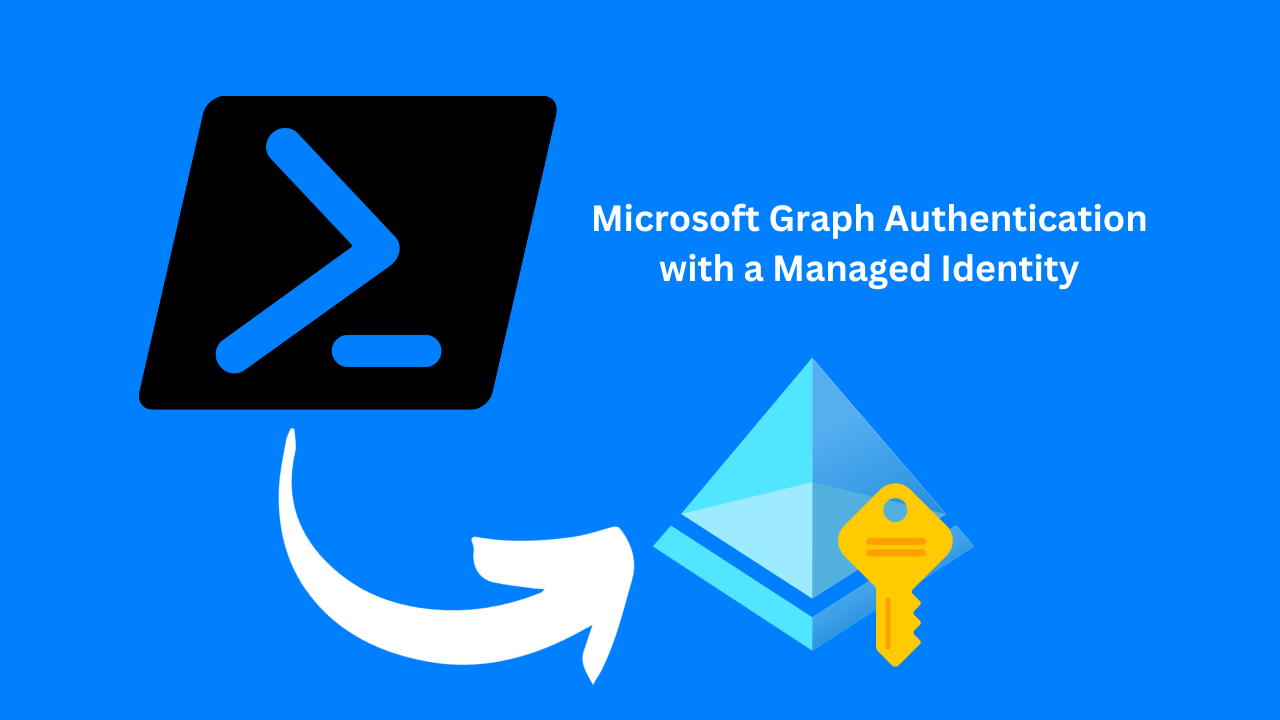
What is a Managed Identity?
Managed identities in Azure are a way for us to authenticate to selected Microsoft resources without having to manage credentials and secrets. A managed identity is associated with an Azure resource like an automation account and you can then use the identity to authenticate to resources from scripts within the automation account.
What is Microsoft Graph?
Microsoft graph is a bunch of API endpoints Microsoft provides to manage the Microsoft 365 platform. These API endpoints include the functionality to manage users and groups in Azure and much more.
The problem with Microsoft Graph and managed identity is that assigning permissions isn't that simple and has to be done using PowerShell. This is what we're going to cover in this post.
Create a new Automation Account
The below PowerShell code shows how we can create a new resource group with an Azure automation account and then enable a system-assigned managed identity.
$RGName = "rg-automation-prod"
$AAName = "aa-automation-prod"
# Connect to Azure PowerShell
Connect-AzAccount
# Create new Resource Group
$RG = New-AzResourceGroup -Location 'UkSouth' -Name $RGName
# Create new Automation Account
$AA = New-AzAutomationAccount -Location $RG.Location -ResourceGroupName $RG.ResourceGroupName -Name $AAName
# Enable Managed Identity
Set-AzAutomationAccount -ResourceGroupName $RG.ResourceGroupName -Name $AA.AutomationAccountName -AssignSystemIdentity
Assigning permissions to a Managed Identity
The below PowerShell snippet will connect to Microsoft Graph to get the managed identity service principal as well as the Microsoft Graph service principal. It will then loop through the roles you specify and assign them to the managed identity.
All you need to do is change out the array of $GraphRoles
to include the Microsoft Graph permissions you desire.
You'll need to ensure you either use the above PowerShell to create the automation account or fill out the $AAName
variable with the automation account name that has the managed identity assigned to.
$GraphRoles = @("Directory.Read.All")
# Connect to Graph
Connect-MgGraph -Scopes AppRoleAssignment.ReadWrite.All,Application.Read.All
# Get Service Principals
$MIID = (Get-MgServicePrincipal -Filter "DisplayName eq '$AAName'").Id
$ResourceId = Get-MgServicePrincipal -Filter "AppId eq '00000003-0000-0000-c000-000000000000'"
# Loop through required graph roles and assign them to Managed Identity
foreach($Role in $GraphRoles)
{
$AppRole = $ResourceID.approles | Where {$_.value -eq $Role -and $_.AllowedMemberTypes -contains "Application"}
if($AppRole)
{
New-MgServicePrincipalAppRoleAssignment -ServicePrincipalId $MIID -PrincipalId $MIID -AppRoleId $AppRole.Id -ResourceId $ResourceID.Id
}
else
{
Write-Output "$($Role) not found in Graph"
}
}
Installing Graph Modules
Within our Azure automation account, we need to install at least a couple of graph PowerShell modules to be able to test this and sign into graph PowerShell.
Within your automation account go to modules and click on 'add a module'
Choose 'Browse from gallery' and click on the link below that.
Search for Microsoft.Graph.Authentication and click on the module. On the next page click on 'Select'.
Choose your version of Powershell e.g. 5.1 and select import.
Once the module has successfully imported you will then need to do the same for another Microsoft.Graph module depending on what you're aiming to do with graph e.g. Microsoft.Graph.Users
Connecting to Microsoft Graph with Managed Identity
In your Automation account go to runbooks and select 'Create a runbook'
Enter a name for your runbook, select Powershell as the type and select your runtime version e.g. 5.1. Click on 'Create' to create the runbook.
To connect to Microsoft Graph using the managed identity you just need to use the 'Connect-MgGraph' cmdlet with the '-Identity' parameter as shown in the below example. This will intuitively use the managed identity to connect to Graph PowerShell.
try
{
Write-Output "Connect to Microsoft Graph"
Connect-MgGraph -ErrorAction Stop -Identity
Write-Output "Connect to Microsoft Graph: Success"
}
catch
{
Write-Output "Connect to Microsoft Graph: Failed"
Write-Output $Error[0].Exception.Message
throw
}
try
{
Write-Output "Get Users"
Get-MgUser -ErrorAction Stop
Write-Output "Get Users: Success"
}
catch
{
Write-Output "Get Users: Failed"
Write-Output $Error[0].Exception.Message
throw
}
When you run the runbook you'll then be able to see the output for your users.
Subscribe to my newsletter
Read articles from Jamie Lawson directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
