Building an E-commerce System: A Comprehensive Code Breakdown - Part 6
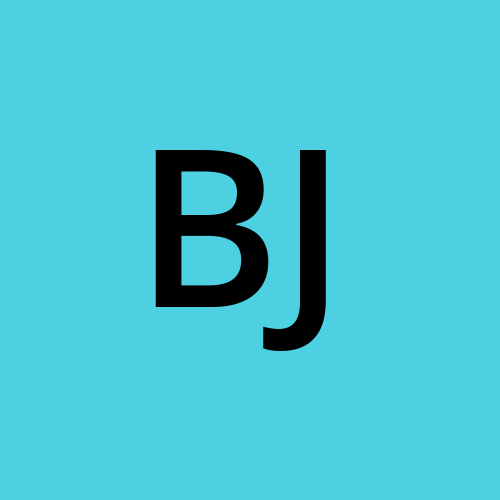
Part 6: Enhancing User Experience for Your E-commerce Application
In our ever-evolving digital world, an exceptional user experience can make or break an e-commerce platform. In Part 6 of our series on building a Simple E-commerce Application, we will delve into various ways to improve the user experience and keep your customers engaged.
User-Friendly Input Handling
User interaction lies at the heart of any e-commerce application. Ensuring that users can effortlessly navigate and interact with your platform is paramount. Let's explore how to handle user input gracefully:
try {
System.out.print("Enter your choice (1/2/3/4): ");
int paymentChoice = Integer.parseInt(scanner.nextLine());
if (paymentChoice < 1 || paymentChoice > 4) {
throw new IllegalArgumentException("Invalid choice.");
}
// Process payment choice
} catch (NumberFormatException | IllegalArgumentException e) {
System.out.println("Invalid input. Please enter a valid choice.");
}
In this code snippet, we've introduced robust input handling. By using try-catch blocks, we can detect invalid inputs such as non-numeric characters or choices outside the expected range. This not only prevents unexpected crashes but also provides clear feedback to users, enhancing their experience.
User Accounts and Personalization
Incorporating user accounts into your e-commerce platform opens up a world of possibilities. Users can create profiles, save their preferences, and enjoy personalized experiences. Here's how you can implement user accounts:
// User Account Creation
User newUser = new User("username", "password", "email@example.com");
userRepository.addUser(newUser);
// User Login
User loggedInUser = authenticateUser(userRepository.getUsers(), "username", "password");
if (loggedInUser != null) {
// User successfully logged in
} else {
System.out.println("Invalid credentials. Please try again.");
}
User accounts enable you to offer tailored recommendations, track order histories, and send personalized promotions. This level of personalization can significantly improve user engagement and loyalty.
Product Reviews and Ratings
One way to foster user engagement is by allowing customers to leave product reviews and ratings:
Product product = getProductById(productQuantityMap.keySet(), productId);
if (product != null) {
// Prompt the user to leave a review and rating
System.out.print("Leave a review: ");
String review = scanner.nextLine();
System.out.print("Rate the product (1-5): ");
int rating = Integer.parseInt(scanner.nextLine());
// Create a review object and associate it with the product
Review productReview = new Review(review, rating, currentUser);
product.addReview(productReview);
}
Product reviews and ratings help build trust among potential buyers. Customers can share their experiences, making it easier for others to make informed purchasing decisions. Additionally, providing a platform for customer feedback shows that you value their opinions.
Key Takeaways
Effective Input Handling: Implement robust input handling mechanisms to ensure that user interactions with your e-commerce application are smooth and error-resistant. Use try-catch blocks to catch and handle exceptions gracefully, providing clear feedback to users when they input invalid data.
User Accounts and Personalization: Introducing user accounts allows you to offer a personalized experience to your customers. Users can create profiles, save preferences, and enjoy customized recommendations. Personalization enhances user engagement and fosters loyalty.
Product Reviews and Ratings: Enabling customers to leave product reviews and ratings is a valuable feature. It builds trust among potential buyers, helps users make informed decisions, and demonstrates that you value customer feedback. Embrace this user-generated content to enhance your product offerings and reputation.
By focusing on these key areas, you can elevate the user experience of your e-commerce platform, ultimately attracting and retaining a satisfied customer base. Stay tuned for the next part of our series, where we'll explore the crucial topic of security in e-commerce applications.
Subscribe to my newsletter
Read articles from Bryan Samuel James directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
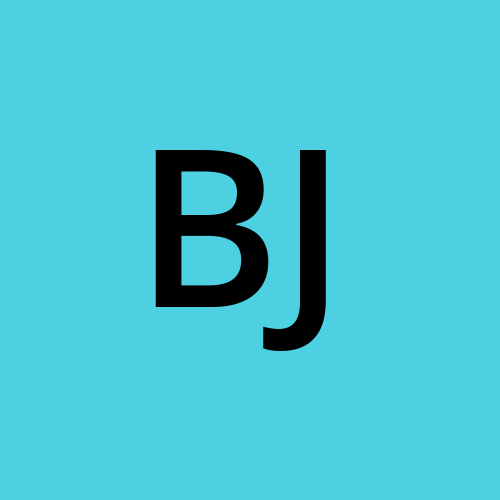