Understanding Variables in Python: A Beginner's Guide

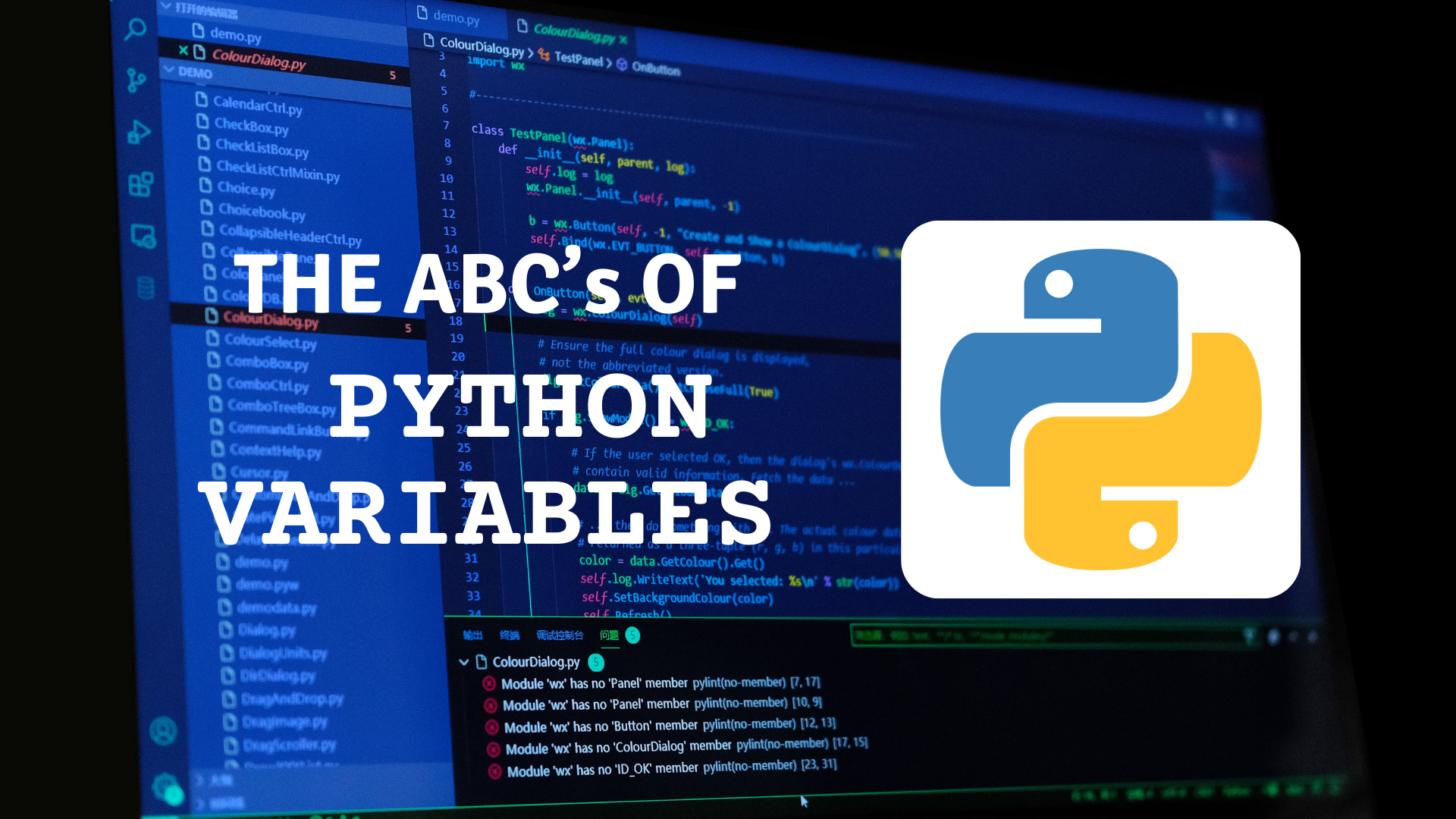
Introduction
Python is more than just a programming language; it's the gateway to a world of endless possibilities. In this comprehensive guide, we'll embark on a journey to demystify one of Python's fundamental building blocks: variables.
What Are Variables?
Variables in Python are like magic placeholders that can store all sorts of information. Imagine them as labelled jars in your programming pantry, each holding a unique ingredient.
Take, for instance, the variable age
. It can hold the age of a person, like 25
. Or the variable name
—it can store a name like "John"
. Variables are flexible, adapting to the type of data they contain.
Declaring Variables:
To declare a variable in Python, it's as easy as giving a name to your magic jar and putting something in it. Here's how it looks in code:
age = 25
name = "John"
In this small snippet, we've created two variables: age
and name
, which contain data about a person's age and name.
Variable Names:
Choosing names for your variables is crucial for clear communication in your code. Imagine you're writing a recipe; you wouldn't want to mix up the sugar and salt jars! Here are some rules for variable names:
Variable names can include letters, numbers, and underscores.
They should start with a letter or an underscore.
Variable names are sensitive to capitalization.
So, myVar
, myvar
, and my_var
are all different variables.
Data Types:
Python is not fussy about data types; it's like a chameleon that adapts to its surroundings. It figures out the data type automatically based on what you put in the variable. For instance:
If you put a number in a variable, it becomes an
int
(e.g.,age = 25
).If it's a decimal number, it's a
float
(e.g.,price = 19.99
).For text, it's a
str
(e.g.,name = "John"
).
Using Variables: Variables are not just placeholders; they are tools to bring your code to life. Want to greet someone? Use the print
function with a variable:
print("Hello, " + name + "!")
In this example, we've combined the "Hello, "
string with the name
variable to create a personalized greeting.
Changing Variable Values:
Variables aren't static; they can change over time, just like your age or your mood. For example, if you celebrate a birthday, you can update the age
variable:
age = 30
Now, age
reflects your new age, 30!
Conclusion:
Python's variables are like magic jars that hold the ingredients of your code recipes. You've taken your first steps in understanding how to create, use, and manipulate variables in Python. As you continue on your Python journey, you'll discover that variables are your trusty companions, ready to store and transform data for countless programming adventures.
Subscribe to my newsletter
Read articles from Riddhi Shinde directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Riddhi Shinde
Riddhi Shinde
🌱 Each day is an opportunity to learn something new, and I'm committed to soaking up knowledge like a sponge. Join me as I take my first steps into the world of coding, problem-solving, and all things tech-related. Whether you're a seasoned pro or a fellow newbie, let's embark on this learning journey together.