Mongo Db
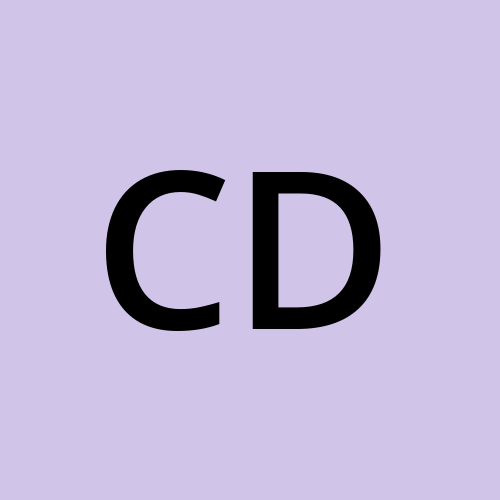
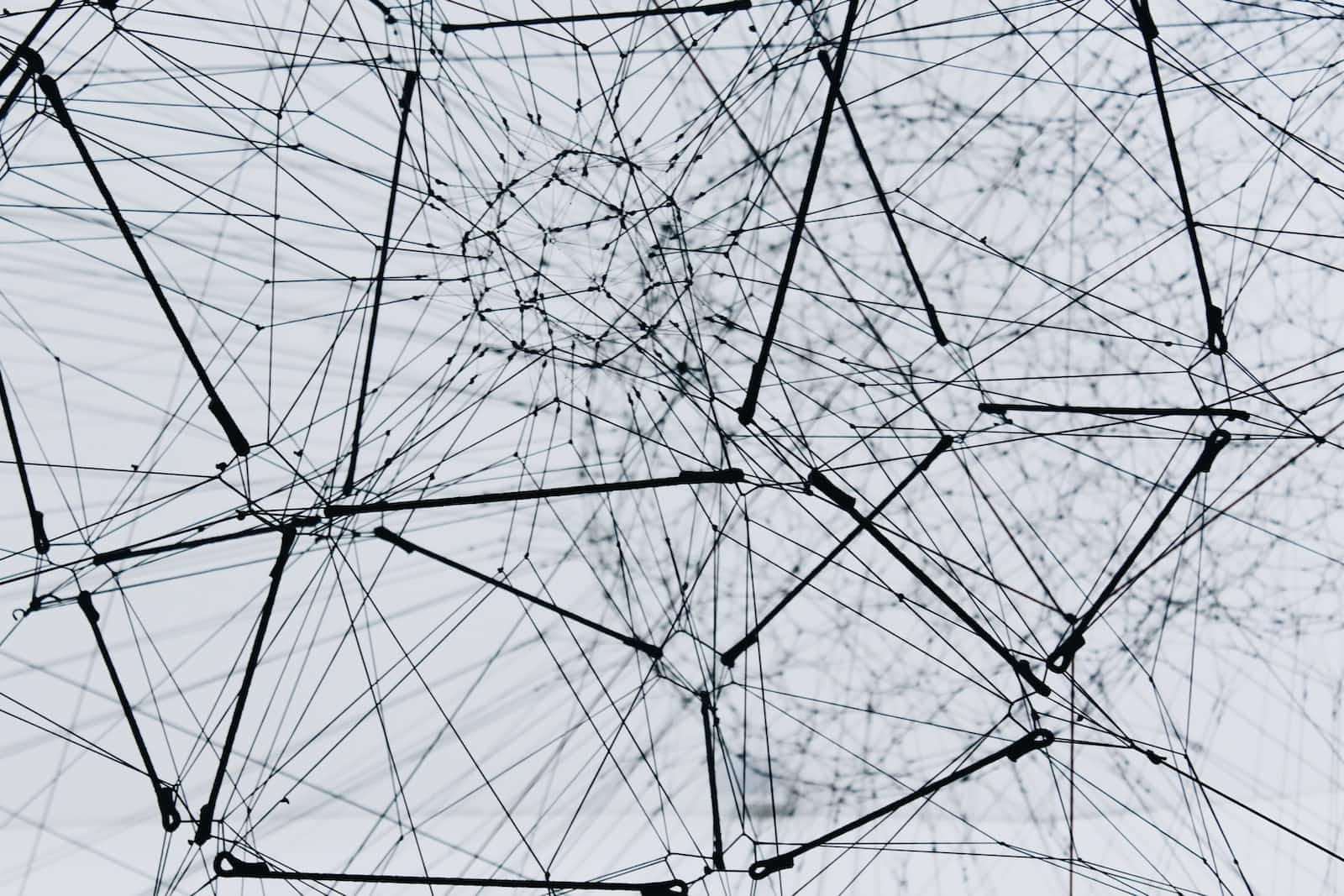
A database is an organized collection of data that can be easily accessed and managed
Mongo Db is a No SQL database
Mongo Db is a scalable, open-source, high-performance, document-oriented database
Differences
SQL | No SQL |
RDBMS - Relational Database Management System | Non-Relational or Distributed |
Data stored in tables | Data stored in Collections (like JSON) |
Vertically Scalable (Rows will be added vertically) | Horizontal Scalable (Data gets added horizontally) |
Setup
Mongod + Compass - link
Mongosh - link
Critical Note
After downloading and installing the Mongo DB. Do the following for windows
Create
data
folder in the C driveCreate
db
folder insidedata
Server and Client
mongod
and mongodb
refers to different components of the MongoDB database system:
mongod (server)
It is the primary daemon process for the MongoDB server. It is responsible for managing the core database system and handling client requests.
It is the MongoDB server itself, and it runs as a background service or process on a server.
You typically interact with the MongoDB server through client applications, drivers, and shells.
mongosh (Client)
It is often used to refer to the MongoDB shell, which is a command-line interface (CLI) tool for interacting with the MongoDB server.
The MongoDB shell is a JavaScript-based interface that allows you to connect to a MongoDB server, execute database operations, run queries, and perform administrative tasks.
It is a client-side tool used to communicate with the mongod server.
In summary, mongod is the MongoDB server process responsible for database management and serving client requests, while Mongodb refers to the MongoDB shell, which is a client-side tool for interacting with the server. Both components are essential for working with MongoDB effectively, with mongod handling the server-side operations and mongodb providing a command-line interface for users to interact with the database.
Troubleshoot issues
- If you are facing issues in copying and pasting the commands in mongosh command line interface
steps:
1. Open the mongosh command line.
2. Right-click on the command line prompt (On main header Panel)
3. Select Properties.
4. Click on the Layout tab.
5. Check the Use Ctrl + Shift + C/V as Copy/Paste option.
6. Click on the OK button.
Mongo DB Commands
Show all the available dbs
show dbs
Create or switch to a new DB
use product
Create a collection in the current DB
db.createCollection('prod')
To know the current db
db
Show all the available collections in the current DB
show collections
Add documents (rows) to the collections. Note if there is a collection that doesn't exist earlier then it will create and insert documents.
db.users.insert({name:'Json', age:'20'}) // Depreciated db.users.insertOne({name:'Json', age:'20'}) db.users.insertMany([{name:'Json', age:'20'}, {name:'octa', age:'24'}])
Drop or delete a collection (books is a collection)
db.books.drop()
Drop or delete the current DB
db.dropDataBase()
To see all the documents in the collection
db.books.find()
To update a document
//Updates one document with given id. db.books.updateOne( ... { _id: ObjectId("6524317c389043329a67b677") }, ... {$set: { name: 'This is just testing book', pages: 400, price: 2000 }} ... ) //Updates all docs whose pages<600. Price will be incremented by 5 db.books.updateMany( ... {pages:{$lt:600}}, ... {$inc:{price:5}} ... )
Operators in mongodb (link)
- Renaming the field (column)
db.books.updateOne(
... {_id: ObjectId("6524317c389043329a67b677")},
... {$rename:{name:'bookName'}}
... )
- Updating the records with new field name which has pages 1000.
db.books.update( { pages: 1000 }, { $rename: { name: 'bookName' } } )
- Increment the value of the field
db.books.updateOne(
... {_id: ObjectId("65243269389043329a67b679")},
... {$inc:{price:5}}
... )
- Updating the records with less than a particular number
db.books.updateMany(
... {pages:{$lt:600}},
... {$inc:{price:5}}
... )
Deletion
db.books.deleteOne({ _id: ObjectId("65243269389043329a67b679") }) db.books.deleteMany({pages: 500})
Formatting the results
db.books.find().pretty()
Limit the results
db.books.find().limit(2)
Sorting the results
- Ascending order
db.books.find().sort({pages:1})
- Descending Order
db.books.find().sort({pages:-1})
Sample usage of selection
db.books.find().limit(2).sort({name:-1})
Query Operators (link)
//equals to 900 db.books.find({price:{$eq:900}}) //Greater than or equals to 900 db.books.find( { price: { $gte: 900 } } ) //Greater than 900 db.books.find( ... {price: {$gt:900}} ... ) //price between 300 and 900 db.books.find( ... {price: {$in:[300,900]}} ... )
Complex Query
//Find document which has pages 500 and price 105 db.books.find( ... {$and:[{pages:500},{price:105}]} ... ) //Price not greater than 55 db.books.find( ... {price: {$not: {$gt:55}}} ... )
Projections (Show only a few columns or fields) (1-include, 0-exclude)
//This shows the records with name field only with condition price=105 //This also prints _id field by default db.books.find( ... {price: 105}, ... {name:1} ... ) //Show only name field and ignore _id db.books.find( ... { price: 105 }, ... { name: 1, _id: 0 } ... ) //Don't show name and price fields. All other fields will be shown. db.books.find( ... {price:105}, ... {name:0,price:0} ... )
Subscribe to my newsletter
Read articles from Chetan Datta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
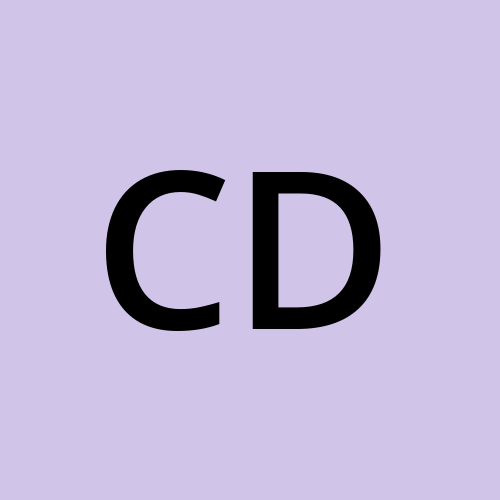
Chetan Datta
Chetan Datta
I'm someone deeply engrossed in the world of software developement, and I find joy in sharing my thoughts and insights on various topics. You can explore my exclusive content here, where I meticulously document all things tech-related that spark my curiosity. Stay connected for my latest discoveries and observations.