Lists and Keys in ReactJS.

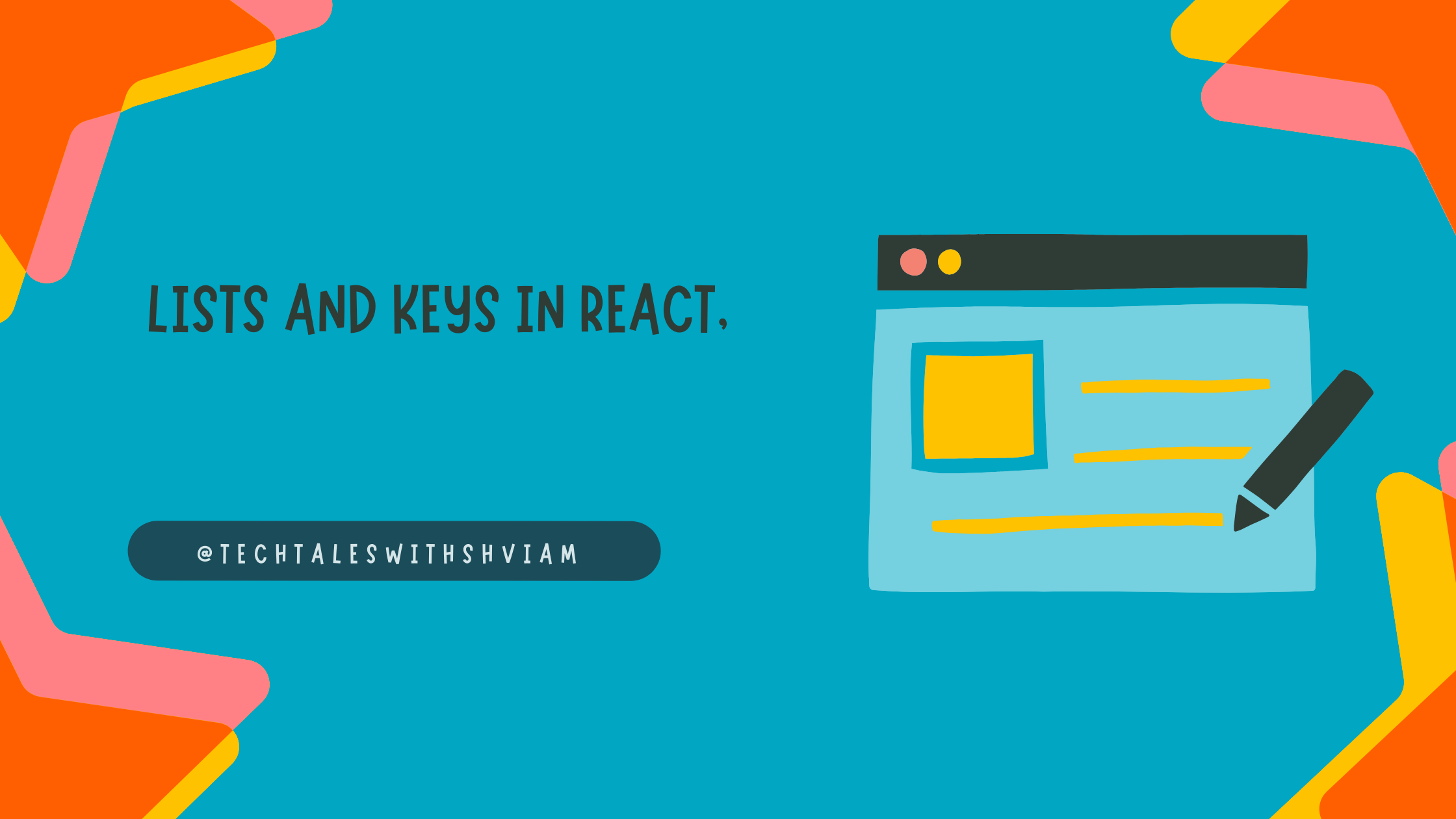
One of the most common tasks in web development is to render a list of data items on the screen. For example, you might want to display a list of products, a list of comments, a list of tasks, etc. In this blog post, we will learn how to use lists and keys in ReactJS to create dynamic and efficient user interfaces.
What are lists and keys in ReactJS?
Lists are collections of elements that can be rendered using JSX syntax. JSX allows us to write HTML-like code in JavaScript and use it as React components. For example, we can create a list of numbers using an array and JSX:
const numbers = [1, 2, 3, 4, 5];
const listItems = numbers.map((number) => <li>{number}</li>);
The map()
method is a JavaScript array function that takes a callback function and applies it to each element of the array, returning a new array with the results. In this case, we are using an arrow function that takes a number as an argument and returns a <li>
element with the number as its content.
We can then use the listItems
array as a child element of a <ul>
element to render an unordered list:
<ul>{listItems}</ul>
This code will produce the following output:
<ul> <li>1</li> <li>2</li> <li>3</li> <li>4</li> <li>5</li> </ul>
Keys are special string attributes that we need to include when creating lists of elements in React. Keys help React identify which items have changed, are added, or are removed. Keys should be unique among the siblings of a list, but they can be duplicated globally.
Keys are important for performance and stability reasons. When React renders a list of elements, it compares the new elements with the previous ones to determine which ones need to be updated, inserted, or deleted. If we don’t provide keys, React will use the index of the element as the default key, which can cause problems if the order of the elements changes or if some elements are added or removed.
To avoid these issues, we should always provide keys that reflect the identity of each element. The best way to choose a key is to use a string that uniquely identifies the element among its siblings. For example, if we have a list of products, we can use their IDs as keys:
const products = [
{ id: 1, name: "Apple", price: 1 },
{ id: 2, name: "Banana", price: 1.3 },
{ id: 3, name: "Carrot", price: 2 },
];
const productItems = products.map((product) => (
<li key={product.id}>
{product.name} - ${product.price}
</li>
));
We can then use the productItems
array as a child element of a <ul>
element to render an unordered list:
<ul>{productItems}</ul>
This code will produce the following output:
<ul> <li>Apple - $1</li> <li>Banana - $1.3</li> <li>Carrot - $2</li> </ul>
How to use lists and keys in ReactJS?
There are different ways to use lists and keys in ReactJS depending on our needs and preferences. Here are some common scenarios and examples:
Rendering lists inside components
Usually, we will render lists inside components that accept an array of data items as props. This way, we can reuse the component for different data sources and make it more modular and maintainable.
For example, we can create a component called NumberList
that takes an array of numbers as props and renders a list of <li>
elements with those numbers:
function NumberList(props) {
const numbers = props.numbers;
const listItems = numbers.map((number) => <li key={number}>{number}</li>);
return <ul>{listItems}</ul>;
}
We can then use the NumberList
component with different arrays of numbers:
const oddNumbers = [1, 3, 5, 7, 9];
const evenNumbers = [2, 4, 6, 8, 10];
<NumberList numbers={oddNumbers} />
<NumberList numbers={evenNumbers} />
This code will produce the following output:
<ul> <li>1</li> <li>3</li> <li>5</li> <li>7</li> <li>9</li> </ul>
<ul> <li>2</li> <li>4</li> <li>6</li> <li>8</li> <li>10</li> </ul>
Extracting components with keys~
Sometimes, we might want to extract a component for each element of a list to make the code more readable and reusable. For example, we can create a component called Product
that takes a product object as props and renders a <li>
element with the product name and price:
function Product(props) {
const product = props.product;
return (
<li>
{product.name} - ${product.price}
</li>
);
}
We can then use the Product
component inside the map()
method to create a list of products:
const products = [
{ id: 1, name: "Apple", price: 0.99 },
{ id: 2, name: "Banana", price: 0.79 },
{ id: 3, name: "Carrot", price: 0.49 },
];
const productItems = products.map((product) => (
<Product key={product.id} product={product} />
));
We can then use the productItems
array as a child element of a <ul>
element to render an unordered list:
<ul>{productItems}</ul>
This code will produce the same output as before, but with a more modular and reusable code structure.
Note that we still need to provide keys for each element of the list, even if we extract them into separate components. The key should be specified on the <Product>
element, not on the <li>
element inside the Product
component. This is because the key is used by React to identify the element in the list, not the element in the DOM.
Using keys with arrays ~
Sometimes, we might want to use keys with arrays that are not lists of elements. For example, we might want to render multiple components for each item in an array. In this case, we need to provide keys for each component in the array, not for the array itself.
For example, we can create a component called BlogPost
that takes a blog post object as props and renders a <div>
element with the post title and content:
function BlogPost(props) {
const post = props.post;
return (
<div>
<h3>{post.title}</h3>
<p>{post.content}</p>
</div>
);
}
We can then use the BlogPost
component inside the map()
method to create an array of blog posts:
const posts = [
{ id: 1, title: "Hello World", content: "This is my first blog post." },
{ id: 2, title: "React Rocks", content: "React is awesome for building user interfaces." },
{ id: 3, title: "Lists and Keys", content: "Learn how to use lists and keys in ReactJS." },
];
const postElements = posts.map((post) => (
<BlogPost key={post.id} post={post} />
));
We can then use the postElements
array as a child element of a <div>
element to render a blog:
<div>{postElements}</div>
This code will produce the following output:
<div> <h3>Hello World</h3> <p>This is my first blog post.</p> </div>
<div> <h3>React Rocks</h3> <p>React is awesome for building user interfaces.</p> </div>
<div> <h3>Lists and Keys</h3> <p>Learn how to use lists and keys in ReactJS.</p> </div>
Note that we still need to provide keys for each element of the array, even if they are not lists of elements. The key should be specified on the <BlogPost>
element, not on the <div>
element inside the BlogPost
component. This is because the key is used by React to identify the element in the array, not the element in the DOM.
Conclusion~
In this blog post, we have learned what are lists and keys in ReactJS and how to use them in different scenarios. Lists and keys are essential concepts for creating dynamic and efficient user interfaces with React. We should always provide keys that reflect the identity of each element in a list and avoid using indexes or random values as keys. We should also use keys for each component in an array that is not a list of elements.
I hope you have enjoyed this blog post and learned something new. If you have any questions or feedback, please leave a comment below or contact me at [shivamkumar87148@gmail.com]. Thank you for reading! 😊
Subscribe to my newsletter
Read articles from Shivam Jha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Shivam Jha
Shivam Jha
LFX'24 @Kyverno | Web Dev | DevOps | OpenSource | Exploring Cloud Native Technologies.