Mastering Solidity: A Beginner's Guide to Boolean Logic in Ethereum Smart Contracts
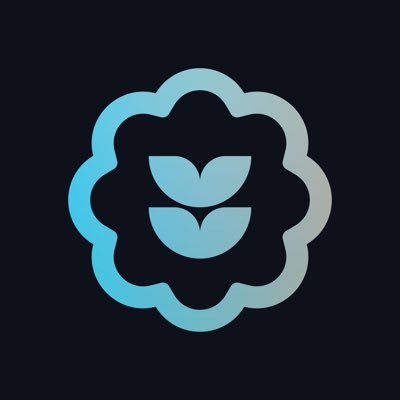
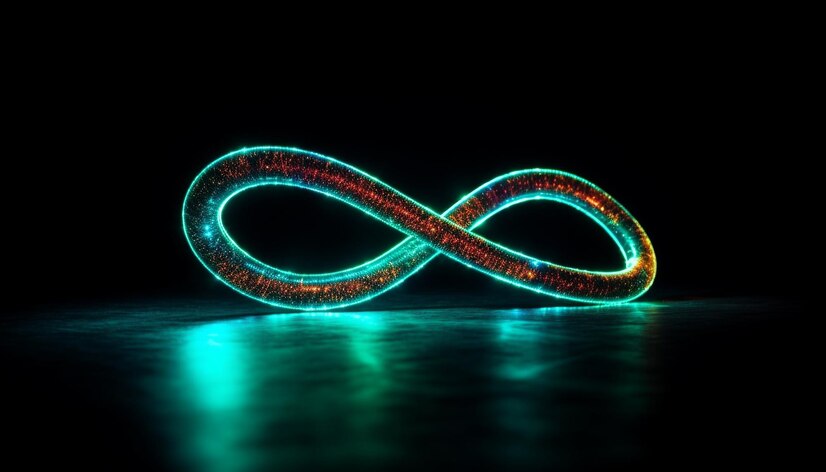
Hey there, fellow tech enthusiasts! Today, we're diving into the world of boolean values in Solidity, the language that powers Ethereum smart contracts. Now, you might wonder, what are these boolean thingamajigs? Well, they're essentially the digital version of a light switch, capable of being either ON (true) or OFF (false).
Meet the Bool: A True or False Affair
In the realm of Solidity, booleans are your go-to buddies for decision-making. Imagine you're programming a vending machine. A boolean would be the friend who tells you whether the machine is ready to serve (true) or needs a little break (false).
So, how do we use these boolean fellas? It's simple! We declare them using the bool
keyword, like this:
bool public isActive = true;
In this example, isActive
is a boolean variable that's set to true
. It's like saying, "Hey, vending machine, you're good to go!"
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.18;
contract BooleanOperations {
bool public isTrue = true;
bool public isFalse = false;
function andOperation() public view returns(bool) {
return isTrue && isFalse; // returns false
}
function orOperation() public view returns(bool) {
return isTrue || isFalse; // returns true
}
function notOperation() public view returns(bool) {
return !isTrue; // returns false
}
}
Boolean Logic: AND, OR, NOT
Now, here’s where it gets interesting. Booleans love to team up and perform logical gymnastics. They're great at AND, OR, and NOT operations.
Imagine you have two boolean buddies: one is isTrue
(true) and the other is isFalse
(false). Let's play with them:
AND Operation (&&): This is like asking, "Are both of you ready?" If
isTrue
andisFalse
are both true, the AND operation returns true. But if any one of them is false, it's a no-go, and the result is false.OR Operation (||): Think of this as asking, "Is at least one of you ready?" If
isTrue
orisFalse
is true, the OR operation returns true. It’s a party as long as someone’s ready!NOT Operation (!): This is the digital version of saying, “Whatever
isTrue
says, I’ll say the opposite.” IfisTrue
is true, NOTisTrue
becomes false, and vice versa.
In the world of Solidity, booleans are like the backstage crew, making sure the show (your smart contract) runs smoothly. They help you make decisions, control the flow of your code, and ensure everything works just as you intend.
Subscribe to my newsletter
Read articles from darkdepauli7.nft directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
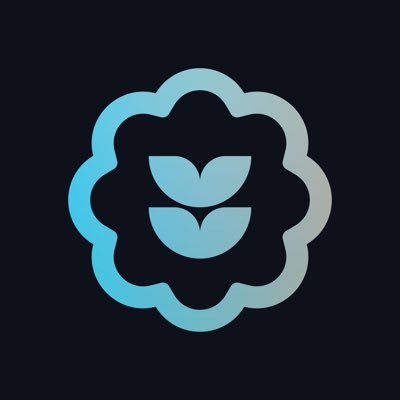
darkdepauli7.nft
darkdepauli7.nft
Web3 Technical Content Creator