State Mangement in React
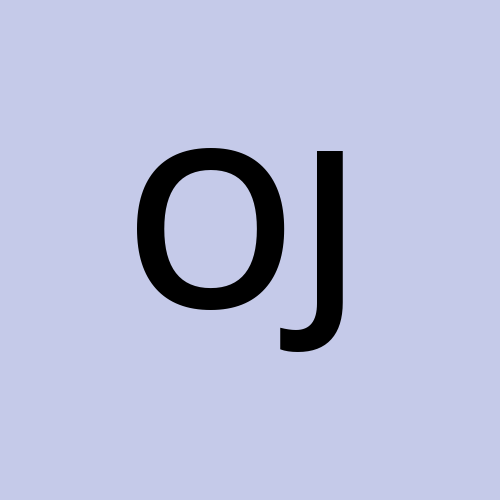
State Management Overview
Introduction:
React, a JavaScript library for building user interfaces, has gained immense popularity due to its declarative syntax and component-based architecture. One critical aspect of building robust React applications is efficient state management. In this article, i'll explore the importance of state, the default state in React components, and various strategies for advanced state management.
Understanding State in React:
State in React represents the current condition or data within a component. It is dynamic and can change over time, leading to updates in the user interface. State is crucial for handling user interactions, managing data, and ensuring a responsive application.
Default State in React Components: React components can have state through the useState hook or, in class components, through the setState method. The useState hook was introduced in React 16.8, providing a concise way to manage state in functional components. For example:
using the javascript code
import React, { useState } from 'react'; function Counter() { const [count, setCount] = useState(0); return ( <div> <p>Count: {count}</p> <button onClick={() => setCount(count + 1)}> Increment </button> </div> ); }
This simple example demonstrates how state is initialized using useState and updated using the setCount function.
Challenges with Local Component State: While local state works well for small to medium-sized applications, it can become challenging to manage as applications grow in complexity. Sharing state between components, handling global state, and ensuring consistency across the application become more critical.
Advanced State Management: Several libraries and patterns address the challenges of state management in larger React applications:
Context API: React's Context API enables the sharing of state between components without manually passing props through each level of the component tree. It acts as a global store that components can subscribe to and receive updates.
Redux: Redux is a popular state management library for React applications. It maintains the entire state of the application in a single store, making it predictable and easy to manage. Actions and reducers are used to modify the state in a controlled manner.
MobX: MobX is another state management library that provides a simple and scalable solution. It uses observable state and reacts automatically to changes, simplifying the development process.
React Query: Focused on managing remote and server state, React Query simplifies data fetching, caching, and synchronization with the server. It's particularly useful for applications heavily dependent on data from APIs.
Choosing the Right State Management Approach: The choice between local state, Context API, Redux, MobX, or other solutions depends on the specific needs of your application. Consider factors such as project size, complexity, team familiarity, and maintainability when making this decision.
Conclusion:
Effective state management is a crucial aspect of building scalable and maintainable React applications. Whether using the built-in useState hook for local component state or opting for more advanced solutions like Redux or MobX, understanding the principles of state management is key to successful React development. Choose the approach that aligns with your project's requirements and contributes to a smooth and efficient development process.
Subscribe to my newsletter
Read articles from Oluwafemi Josephine directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
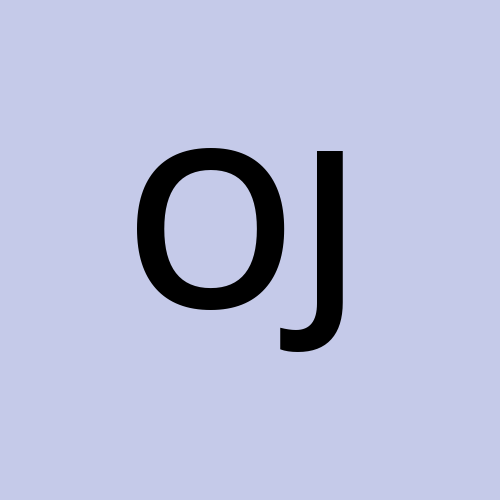
Oluwafemi Josephine
Oluwafemi Josephine
Hi, hi! I'm Josephine Oluwafemi, a passionate and dedicated MERN Stack Software Engineer with a strong background in full-stack web development. I specialize in leveraging the MERN (MongoDB, Express.js, React.js, Node.js) stack to create robust, scalable, and user-centric applications. My expertise lies in crafting efficient and intuitive user interfaces using React.js and Redux, building RESTful APIs with Node.js and Express.js, and designing scalable databases using MongoDB. Throughout my career, I have been involved in the entire software development lifecycle, from project conceptualization and architecture design to implementation, testing, and deployment. I thrive in dynamic and collaborative environments, working closely with cross-functional teams to deliver high-quality solutions that meet client requirements and exceed expectations. My skills extend beyond the MERN stack to encompass a wide range of front-end and back-end technologies, including HTML5, CSS3, JavaScript ES6+, Git, and SQL. I am constantly exploring new technologies and frameworks to stay up-to-date with the ever-evolving landscape of web development, ensuring that I can leverage the best tools to solve complex problems efficiently. If you're looking for a skilled MERN Stack Software Engineer who is passionate about building cutting-edge web applications and contributing to the success of your team, I would love to connect. Let's collaborate on creating exceptional digital experiences that drive user engagement and business growth. Feel free to reach out to me on LinkedIn or via email at Oluwafemijosephine4@gmail.com. I look forward to connecting with you and discussing how I can contribute to your organization's success. Key Skills: MERN Stack Development React.js, Redux Node.js, Express.js MongoDB, SQL HTML5, CSS3, JavaScript ES6+ Git, UI/UX Design Agile Methodologies Problem-solving and Analytical Thinking Excellent Communication and Collaboration Education: BSC, Lagos State University, 2023. Proficient in English Language.