Enhancing Your Online Store with Cookies: Saving Data, Managing Cart, and Calculating Totals
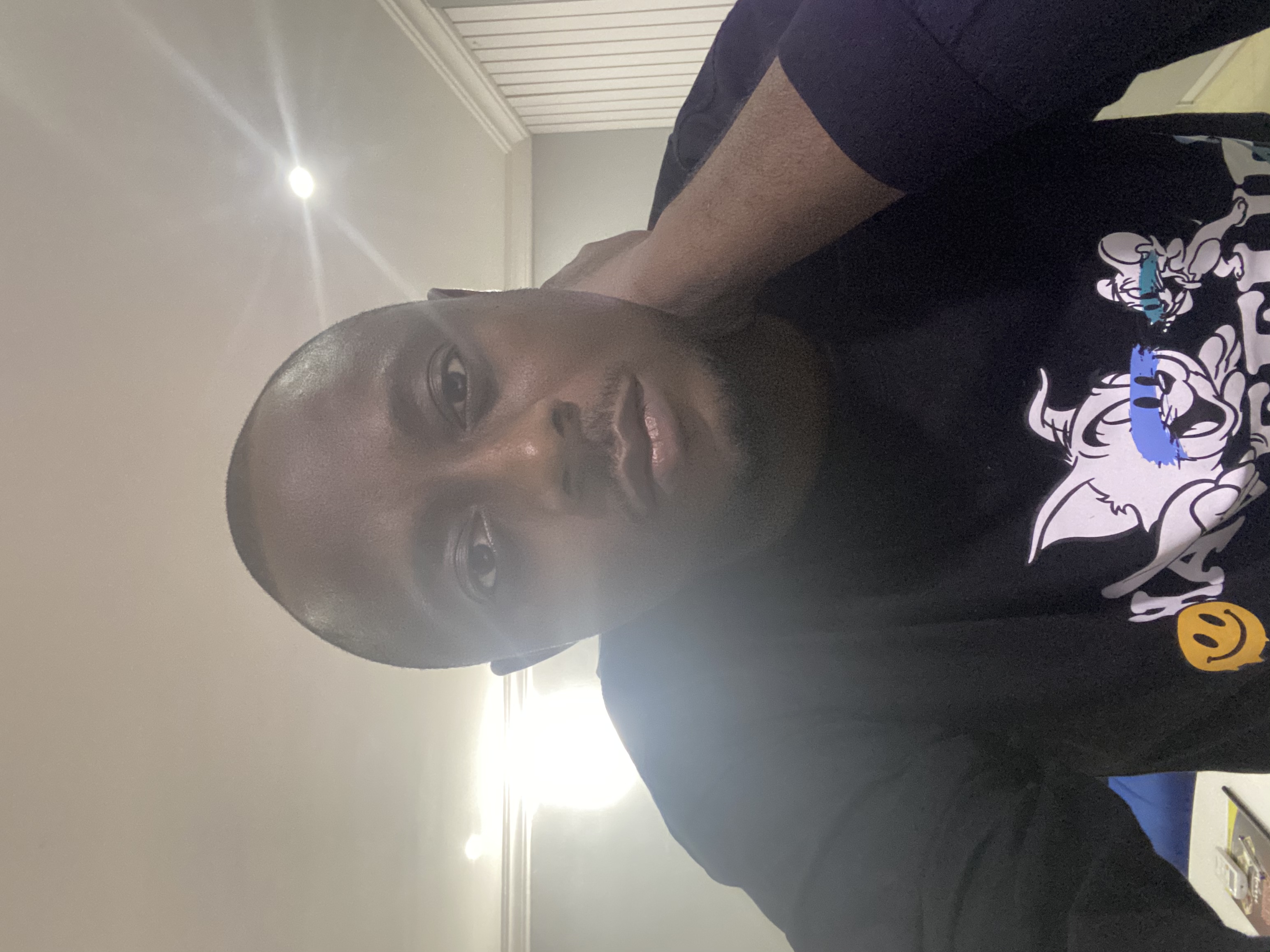
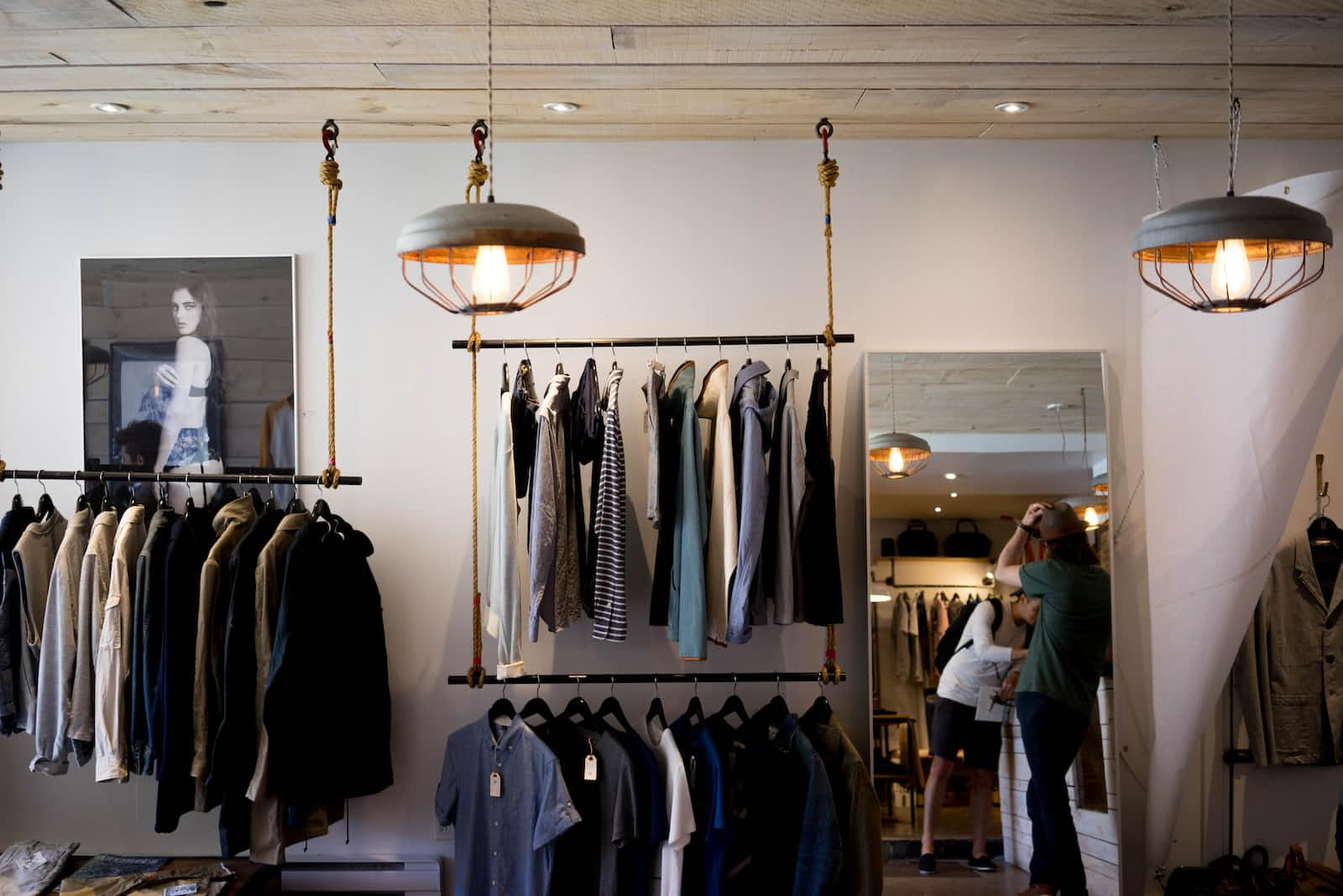
In an online store, it's crucial to offer a seamless and personalized shopping experience for your customers. One way to achieve this is by using cookies to save data, such as products added to the cart, and to calculate the total cost of items. In this blog post, we will explore how to use cookies to improve your online store. We'll provide code samples, including a few products, a cart management system, and the calculation of the total cost.
Part 1: Introduction to Cookies
Cookies are small pieces of data that a web server sends to a user's web browser. They are then stored on the user's device and can be accessed and manipulated by both the server and the client. In the context of an online store, cookies can be used to store information about the user's shopping cart and other preferences.
Part 2: Setting Up Your Online Store
To demonstrate the use of cookies in an online store, let's set up a simple store with a few products and a shopping cart.
Sample Product List (products.php)
<?php
$products = [
['id' => 1, 'name' => 'Product A', 'price' => 10.00],
['id' => 2, 'name' => 'Product B', 'price' => 15.00],
['id' => 3, 'name' => 'Product C', 'price' => 20.00],
];
?>
Part 3: Adding Products to the Cart
In your online store, you'll need to provide an interface for users to add products to their shopping cart. You can do this by using a "Add to Cart" button for each product. When the user clicks the button, you can use JavaScript to send a request to a PHP script that adds the product to the cart.
Sample "Add to Cart" Button (products.php)
<!-- Include this code for each product in your product list -->
<div class="product">
<h2>Product A</h2>
<p>Price: $10.00</p>
<button class="add-to-cart" data-product-id="1">Add to Cart</button>
</div>
JavaScript for Adding Products to Cart (script.js)
// Add an event listener to all "Add to Cart" buttons
document.querySelectorAll('.add-to-cart').forEach(button => {
button.addEventListener('click', function () {
const productId = this.getAttribute('data-product-id');
// Send a request to a PHP script to add the product to the cart using AJAX
// Handle the response from the server
});
});
Part 4: Managing the Shopping Cart with Cookies
In the PHP script that handles the "Add to Cart" request, you can use cookies to maintain the shopping cart's state. Here's a simplified example:
Adding a Product to the Cart (add-to-cart.php)
<?php
if (isset($_POST['product_id'])) {
$productId = $_POST['product_id'];
// Check if the cart cookie exists
if (isset($_COOKIE['cart'])) {
$cart = json_decode($_COOKIE['cart'], true);
} else {
$cart = [];
}
// Add the selected product to the cart
$cart[] = $productId;
// Save the updated cart in a cookie
setcookie('cart', json_encode($cart), time() + 3600, '/');
// Respond with a success message
echo "Product added to cart!";
}
?>
Part 5: Calculating the Total Cost
To calculate the total cost of the products in the cart, you can iterate through the cart items and fetch their prices from the product list. Here's a basic example:
Calculating the Total Cost (calculate-total.php)
<?php
if (isset($_COOKIE['cart'])) {
$cart = json_decode($_COOKIE['cart'], true);
$total = 0;
foreach ($cart as $productId) {
$product = $products[$productId - 1]; // Product ID starts at 1
$total += $product['price'];
}
echo "Total cost: $" . number_format($total, 2);
} else {
echo "Your cart is empty.";
}
?>
Conclusion
By using cookies to save data in your online store, you can provide users with a more interactive and personalized shopping experience. In this blog post, we've covered how to set up a simple online store, add products to the cart, and calculate the total cost of items using cookies. This is just the beginning, and you can further enhance your online store by incorporating features like user authentication, order processing, and more advanced cart management.
Subscribe to my newsletter
Read articles from Ogunuyo Ogheneruemu B directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
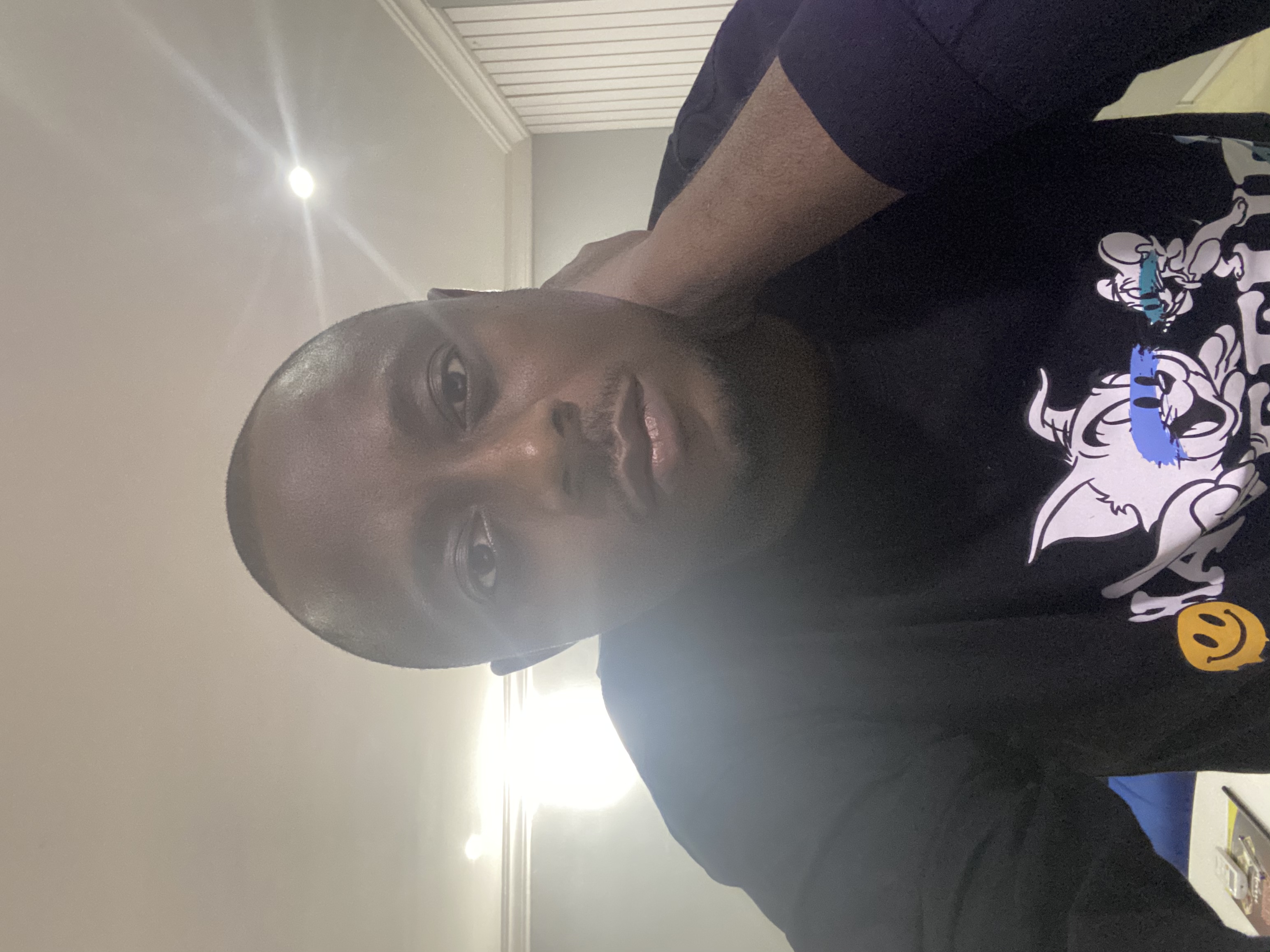
Ogunuyo Ogheneruemu B
Ogunuyo Ogheneruemu B
I'm Ogunuyo Ogheneruemu Brown, a senior software developer. I specialize in DApp apps, fintech solutions, nursing web apps, fitness platforms, and e-commerce systems. Throughout my career, I've delivered successful projects, showcasing strong technical skills and problem-solving abilities. I create secure and user-friendly fintech innovations. Outside work, I enjoy coding, swimming, and playing football. I'm an avid reader and fitness enthusiast. Music inspires me. I'm committed to continuous growth and creating impactful software solutions. Let's connect and collaborate to make a lasting impact in software development.