Cross-Origin Resource Sharing with Amazon S3
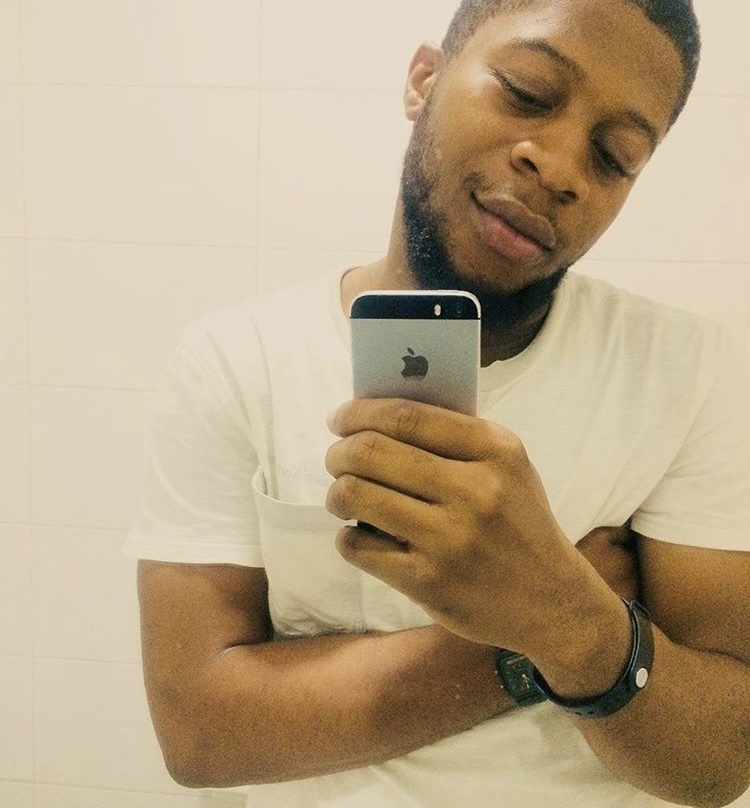
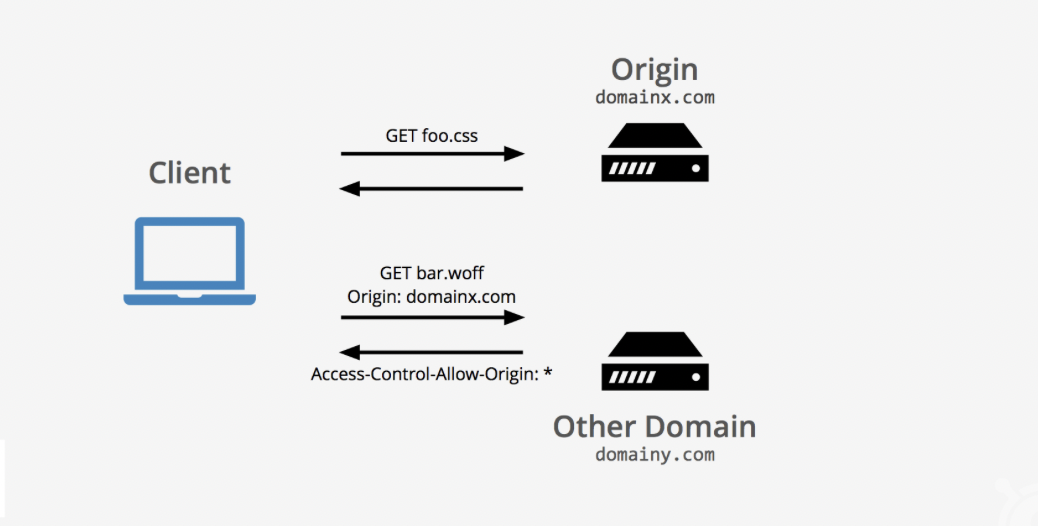
CORS stands for Cross-Origin Resource Sharing, which is a mechanism implemented by web browsers to protect users from malicious web applications. It allows web servers to specify which domains are allowed to access their resources, and which HTTP methods are allowed for those resources. CORS helps prevent unauthorized access to resources on a website and promotes secure communication between different domains. It is commonly used by web developers to enable communication between a client-side application and a server-side API hosted on a different domain.
Now let’s implement CORS with Amazon S3.
To implement CORS with Amazon S3, we will need to follow these steps:
Sign in to the AWS Management Console and navigate to the S3 service.
Then we create two S3 buckets with names ‘origin-bucket-demos3’ and ‘cross-origin-bucket-demos3’.
We create two html files, index.html and extra-page.html to demonstrate CORS with S3 buckets.
Note: S3 bucket names are meant to be unique so you will run into an error if you use these ones. Therefore, give unique names to your S3 buckets.
Next, we make sure to enable the right permissions so objects in these S3 buckets are publicly accessible. Then we will enable static website hosting on both buckets.
I have published an article that outlines the above in more granular steps. click link below to access article.
Demo — Hosting a static website on Amazon
The cloud has so many offerings that help take care of the heavy lifting involved in deploying infrastructure and…
i. Create a file called index.html, copy and paste the html code below in it. Do not forget to replace the url in the index.html file with the full path of the object, extra-page.html. It will typically be in the format <bucket website endpoint>/extra.html.
<html>
<head>
<title>Holiday Spot</title>
</head>
<body>
<h1>I love maldives</h1>
</body>
<img src="maldives.jpeg" width=500/>
<!-- CORS demo -->
<div id="tofetch"/>
<script>
var tofetch = document.getElementById("tofetch");
fetch('http://cross-origin-bucket-demos3.s3-website-us-east-1.amazonaws.com/extra-page.html')
.then((response) => {
return response.text();
})
.then((html) => {
tofetch.innerHTML = html
});
</script>
</html>
ii. Then create a file named extra-page.html, copy and paste the html code in it. In the extra-page.html, replace the image url with the full path, <bucket website endpoint>/cake.jpeg
<p> I love Cake </p>
<p><strong>The image below is from a different Amazon S3 bucket </strong> </p>
<img src="http://cross-origin-bucket-demos3.s3-website-us-east-1.amazonaws.com/cake.jpeg" width=500/>
Note: Download the pictures below as they are referenced in the html files we have created. Make sure to save them with the same name as the specified caption below so they will be rendered by the browser.
maldives.jpeg
cake.jpeg
4. Then we upload the files to the S3 buckets.
i. We upload the index.html and the maldives.jpeg files to the first S3 bucket, origin-bucket-demos3.
origin-bucket-demos3 S3 bucket
ii. Then upload the extra-page.html and the cake.jpeg files to the second s3 bucket, cross-origin-bucket-demos3.
cross-origin-bucket-demo3 S3 bucket
5. Next step is to select the S3 bucket for which we want to configure CORS. In this demo, we select the cross-origin-bucket-demos3 because it contains our extra resource we want to access.
Click on the “Permissions” tab and then click on “CORS configuration”. Click on edit, paste the code below in and save changes.
[
{
"AllowedHeaders": [
"Authorization"
],
"AllowedMethods": [
"GET"
],
"AllowedOrigins": [
"http://origin-bucket-demos3.s3-website-us-east-1.amazonaws.com"
],
"ExposeHeaders": [],
"MaxAgeSeconds": 3000
}
]
This configuration allows any origin to access the S3 bucket using the GET method, with an authorization header, and sets a maximum age of 3000 seconds (50 minutes) for the preflight response.
Screenshot of the website
In summary, this article explains Cross-Origin Resource Sharing (CORS), a mechanism used by web servers to specify which domains are allowed to access their resources and HTTP methods allowed for those resources. It helps prevent unauthorized access to resources and promotes secure communication between different domains. The article then goes on to explain how to implement CORS with Amazon S3 by following a few simple steps. These steps include creating S3 buckets, enabling permissions and static website hosting, creating HTML files, uploading files to S3 buckets, and configuring the CORS settings. A sample configuration for the CORS is also provided.
Subscribe to my newsletter
Read articles from Kelechi Daniels directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
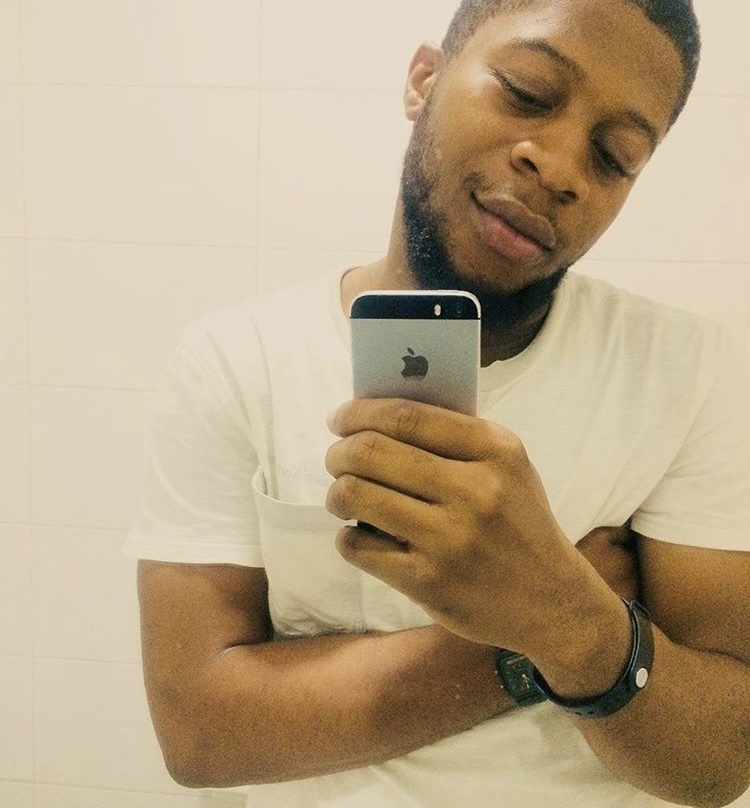
Kelechi Daniels
Kelechi Daniels
Experienced Data guy with software engineering and DevOps expertise, Kelechi is a versatile professional who excels in creating data-driven solutions, automating processes, and driving innovation