Project 2: Tic-Tac-Toe with Python
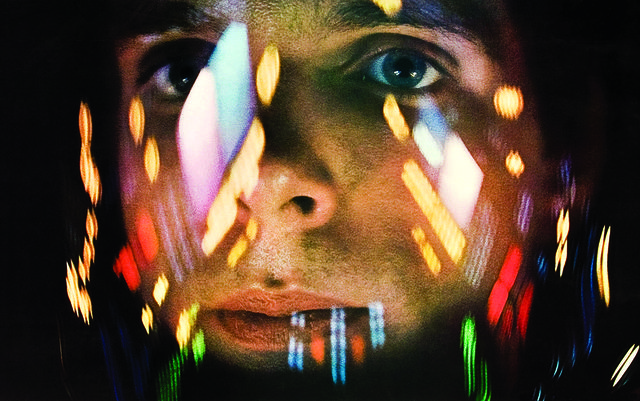
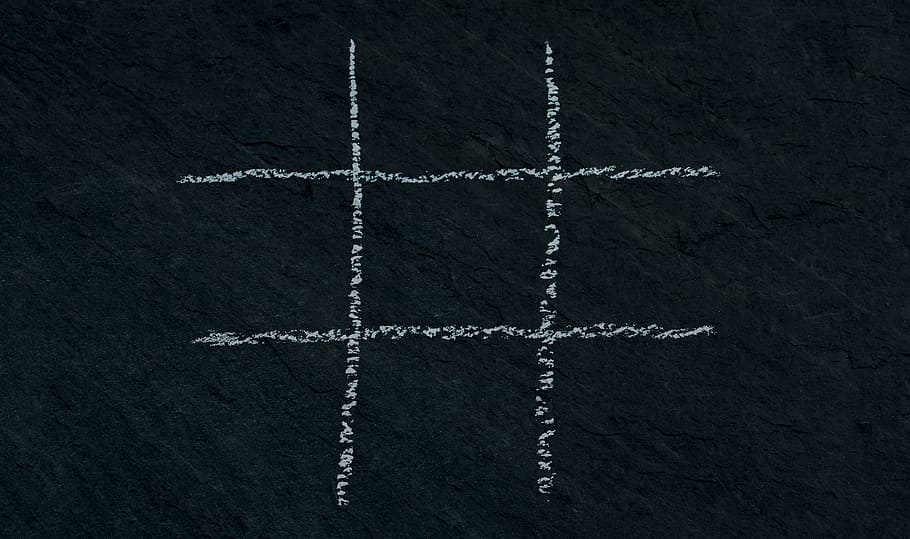
Let's make a tic-tac-toe game using Python. We won't be using any GUI or game engine to make this, to keep it as simple as possible, it will exist only in the console. Let's begin.
A normal tic-tac-toe game has 3 rows and 3 columns with 9 cells for the game. Considering this, we will make 9 variables representing each cell. "a1,a2,a3,b1,b2,b3,c1,c2,c3". For the game grid to appear as usual in the console, I used a series of dashes and spaces for each variable and these variables will be represented by either X's or O's when a move is played.
a1 = "_"
a2 = "_"
a3 = " "
b1 = "_"
b2 = "_"
b3 = " "
c1 = "_"
c2 = "_"
c3 = " "
To print it out we use this code.
print("a1|b1|c1")
print("a2|b2|c2")
print("a3|b3|c3")
It should appear like this in the console.
Great, we're cooking! ๐จโ๐ณ
We have our grid in the console. Now time to make the actual game. The game should wait for you to play a move before continuing, so we need a loop to make the game run until it is over or someone wins. We can do this with a boolean variable representing whether or not the user is still playing and then change the variable to false when the game is over. Let's also create the function that initiates the game. Let's also add instructions for the user, like positions or how they can play.
#Variables representing every single playable cell or position
a1 = "_"
a2 = "_"
a3 = " "
b1 = "_"
b2 = "_"
b3 = " "
c1 = "_"
c2 = "_"
c3 = " "
#Boolean representing whether or not the game is still going on
stillPlaying = True;
#All playable positions
positions = ["a1", "a2", "a3", "b1","b2","b3","c1","c2","c3"]
#The function that initialises the game
def begin_game():
global positions
global stillPlaying
print(a1+"|"+b1+"|"+c1)
print(a2+"|"+b2+"|"+c2)
print(a3+"|"+b3+"|"+c3)
print("pick a position to play: ")
print(positions)
#Loops the game until it is over
while stillPlaying:
begin_game()
Let's make a function that collects the input and replaces the variable with either X's or O's. We will call it "grid" since it replaces each cell in the grid. We use the stillPlaying variable, the cells variables and the positions list variable inside the function. We're using the positions list variable to check for available places you can still play. When the user plays in a position, the variable is removed from the list. We do this to know when a position is still available or not so the user can't play in an occupied position. We also want to check if the user is typing the right thing so we can make a function to check. We also make an input variable. Let's call it play.
#Function to check if the right input is gotten
def rightInput(pos):
if pos == "a1" or pos == "a2" or pos == "a3" or pos == "b1" or pos == "b2" or pos == "b3" or pos == "c1" or pos == "c2" or pos == "c3":
return True
else:
return False
#Function to get input from the user and replace it in the grid
played = 14
def grid():
global stillPlaying #This variable to continue running the game
global a1,a2,a3,b1,b2,b3,c1,c2,c3 #All positions
global positions #All available positions
global played #A variable to see who's turn it is, even for player, odd for computer
play = input() #input collection
if(len(positions) != 0): #if there are available free spaces continue
if rightInput(play) == True: #if the input is right
if(play in positions): #if the input is an available position
played = played - 1 #Make the variable odd by subtracting one, indicating the other player's turn
positions.remove(position) #Remove the played position from available positions
if play== "a1": #Replace the position with X
a1 = "X"
elif play== "a2":
a2 = "X"
elif play== "a3":
a3 = "X"
if play== "b1":
b1 = "X"
elif play== "b2":
b2 = "X"
elif play== "b3":
b3 = "X"
if play== "c1":
c1 = "X"
elif play== "c2":
c2 = "X"
elif play== "c3":
c3 = "X"
elif(play not in positions): #If input not in position, the it is occupied, request for input again
print("occupied, pick another")
begin_game()
Now let's make a function for the computer to play, call it comp_grid. Since I am only a beginner in python and can't create anything crazy like an AI to play with the user, we will let the computer pick random available positions lol.
def comp_grid():
global stillPlaying; #If the game is still going on
global a1, a2, a3, b1,b2,b3,c1,c2,c3 #all positions
global positions #all free positions you can play
global played #To see who's turn it is. Even for player, odd for Computer
if(len(positions) != 0): #if there are available positions
pick = random.choice(positions) #pick a random position
positions.remove(pick) #remove that choice from available positions
played = played - 1 #make it the user's turn
if pick == "a1": #replace the cell with O
a1 = "O"
elif pick == "a2":
a2 = "O"
elif pick == "a3":
a3 = "O"
if pick == "b1":
b1 = "O"
elif pick == "b2":
b2 = "O"
elif pick == "b3":
b3 = "O"
if pick == "c1":
c1 = "O"
elif pick == "c2":
c2 = "O"
elif pick == "c3":
c3 = "O"
else: #Stop the game if there are no more free positions
print("No more Free positions")
stillPlaying = False;
We're almost done, now we need the game to detect when someone wins. A user can win in 8 ways, 3 same rows, 3 same columns and 2 diagonals. We can do that with a function and a for loop.
def winner():
global a1, a2, a3, b1,b2,b3,c1,c2,c3 #all positions
winning_combos = [(a1,a2,a3), (a1,b2,c3), (a1,b1,c1), (b1,b2,b3),(c1,c2,c3), (c1,b2,a3), (a2,b2,c2), (a3,b3,c3)]
#winning-combos is a List of Sets with all the combinations you can win
for combo in winning_combos: #For each combo in the winning combos
if(all(cell == "X" for cell in combo)): #if each cell in the combo is X, then return 1, which means the user won.
return 1
elif(all(cell == "O" for cell in combo)): #if each cell in the combo is O, return 2, Computer wins
return 2
Now we can complete the begin_game function to include winning and the turns of each player.
def begin_game():
global positions
global stillPlaying
print(a1+"|"+b1+"|"+c1)
print(a2+"|"+b2+"|"+c2)
print(a3+"|"+b3+"|"+c3)
print("pick a position to play: ")
print(positions)
#if the number is even, then you played or it's the user's turn
if(played%2==0):
print("You Played:")
grid()
#If it is odd, then it is the computer's turn
elif(played%2 !=0):
print("Computer Played:")
comp_grid()
#Check who's the winner using the winner function
if(winner() == 1):
stillPlaying = False
print("You Win!\n")
print(a1+"|"+b1+"|"+c1)
print(a2+"|"+b2+"|"+c2)
print(a3+"|"+b3+"|"+c3)
elif(winner() == 2):
stillPlaying = False
print("Computer Wins\n")
print(a1+"|"+b1+"|"+c1)
print(a2+"|"+b2+"|"+c2)
print(a3+"|"+b3+"|"+c3)
elif(winner() == False):
stillPlaying = False
print("Nobody Wins\n")
print(a1+"|"+b1+"|"+c1)
print(a2+"|"+b2+"|"+c2)
print(a3+"|"+b3+"|"+c3)
And that's it, a very basic ๐ tic-tac-toe game in Python. I will improve on it in the future to include things like AI and online capabilities. Insha'Allah. Thanks ๐
Full Code
import random
a1 = "_"
a2 = "_"
a3 = " "
b1 = "_"
b2 = "_"
b3 = " "
c1 = "_"
c2 = "_"
c3 = " "
stillPlaying = True;
positions = ["a1", "a2", "a3", "b1","b2","b3","c1","c2","c3"]
played = 14
print("Enter the position you want to enter with a1 to c3, these are the positions \n")
print("a1|b1|c1")
print("a2|b2|c2")
print("a3|b3|c3")
print("\nThe game begins, You are 'X', I am 'O'")
def rightInput(pos):
if pos == "a1" or pos == "a2" or pos == "a3" or pos == "b1" or pos == "b2" or pos == "b3" or pos == "c1" or pos == "c2" or pos == "c3":
return True
else:
return False
def grid():
global stillPlaying;
global a1, a2, a3, b1,b2,b3,c1,c2,c3
global positions
global played
position = input()
if(len(positions) != 0):
if rightInput(position) == True:
if(position in positions):
played = played - 1
positions.remove(position)
if position == "a1":
a1 = "X"
elif position == "a2":
a2 = "X"
elif position == "a3":
a3 = "X"
if position == "b1":
b1 = "X"
elif position == "b2":
b2 = "X"
elif position == "b3":
b3 = "X"
if position == "c1":
c1 = "X"
elif position == "c2":
c2 = "X"
elif position == "c3":
c3 = "X"
elif(position not in positions):
print("occupied, pick another")
begin_game()
def comp_grid():
global stillPlaying;
global a1, a2, a3, b1,b2,b3,c1,c2,c3
global positions
global played
if(len(positions) != 0):
pick = random.choice(positions)
positions.remove(pick)
played = played - 1
if pick == "a1":
a1 = "O"
elif pick == "a2":
a2 = "O"
elif pick == "a3":
a3 = "O"
if pick == "b1":
b1 = "O"
elif pick == "b2":
b2 = "O"
elif pick == "b3":
b3 = "O"
if pick == "c1":
c1 = "O"
elif pick == "c2":
c2 = "O"
elif pick == "c3":
c3 = "O"
else:
print("No more Free positions")
stillPlaying = False;
def winner():
global a1, a2, a3, b1,b2,b3,c1,c2,c3
winning_combos = [(a1,a2,a3), (a1,b2,c3), (a1,b1,c1), (b1,b2,b3),(c1,c2,c3), (c1,b2,a3), (a2,b2,c2), (a3,b3,c3)]
for combo in winning_combos:
if(all(cell == "X" for cell in combo)):
return 1
elif(all(cell == "O" for cell in combo)):
return 2
def begin_game():
global positions
global stillPlaying
print(a1+"|"+b1+"|"+c1)
print(a2+"|"+b2+"|"+c2)
print(a3+"|"+b3+"|"+c3)
print("pick a position to play: ")
print(positions)
if(played%2==0):
print("You Played:")
grid()
elif(played%2 !=0):
print("Computer Played:")
comp_grid()
if(winner() == 1):
stillPlaying = False
print("You Win!\n")
print(a1+"|"+b1+"|"+c1)
print(a2+"|"+b2+"|"+c2)
print(a3+"|"+b3+"|"+c3)
elif(winner() == 2):
stillPlaying = False
print("Computer Wins\n")
print(a1+"|"+b1+"|"+c1)
print(a2+"|"+b2+"|"+c2)
print(a3+"|"+b3+"|"+c3)
elif(winner() == False):
stillPlaying = False
print("Nobody Wins\n")
print(a1+"|"+b1+"|"+c1)
print(a2+"|"+b2+"|"+c2)
print(a3+"|"+b3+"|"+c3)
#print(a1+"|"+b1+"|"+c1)
#print(a2+"|"+b2+"|"+c2)
#print(a3+"|"+b3+"|"+c3)
print("\n")
while stillPlaying:
begin_game()
Subscribe to my newsletter
Read articles from Ja'afar Zakariya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
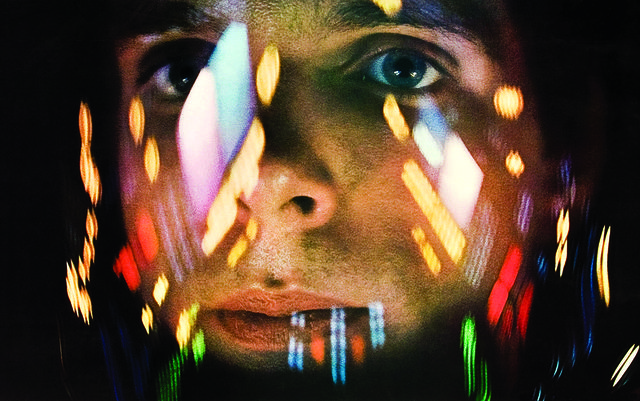
Ja'afar Zakariya
Ja'afar Zakariya
I like movies.