Everything You Need To Know About useState

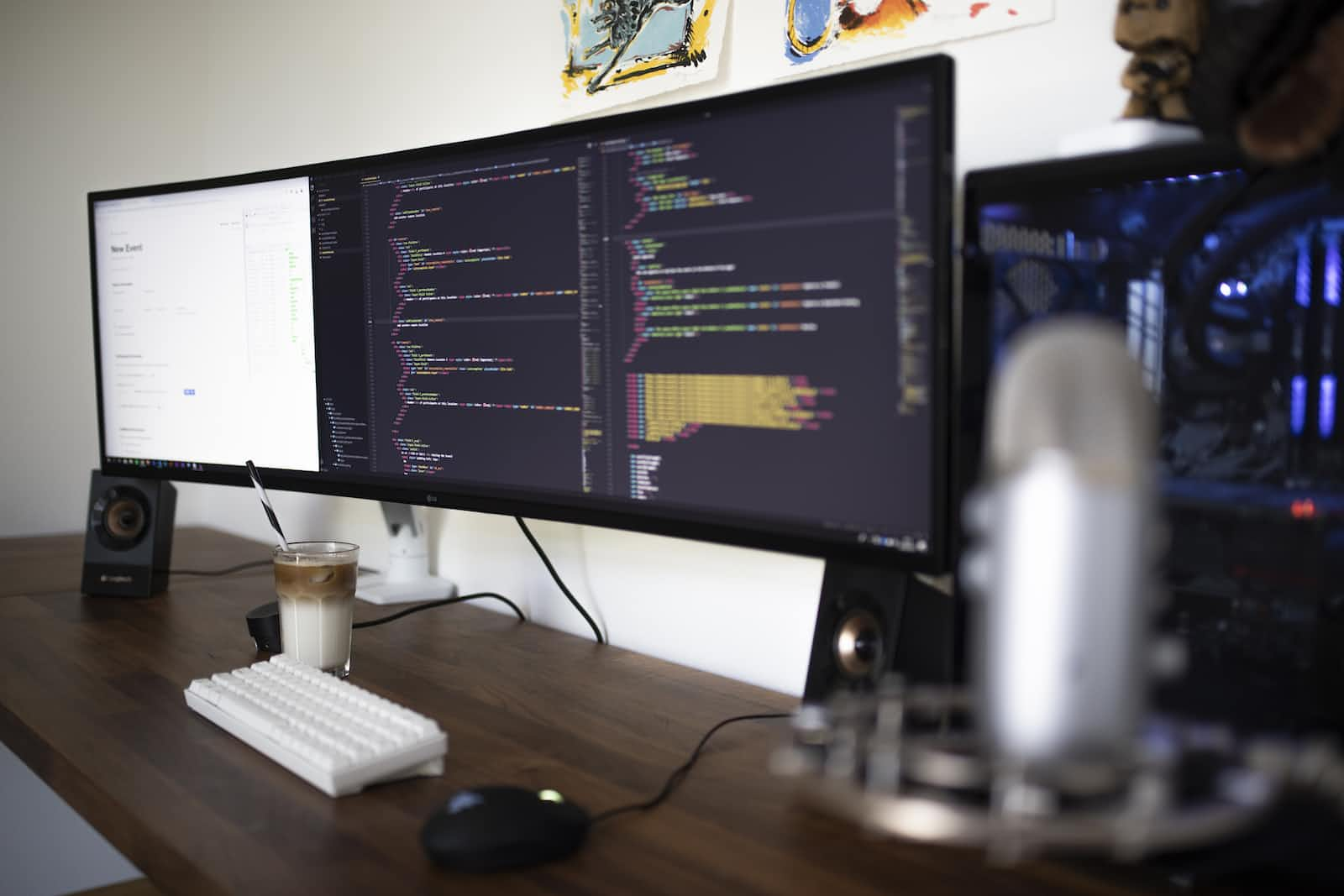
When developing React applications, managing state is a crucial aspect of creating dynamic and interactive user interfaces. Prior to the introduction of hooks, state management was primarily done within class components using the this.state
object. However, with the advent of functional components and hooks, handling state has become more streamlined and expressive.
What is the useState
Hook?
The useState
hook is one of the built-in hooks provided by React. It enables functional components to manage and update state, replacing the need for class components and the this.state
object. This hook allows you to declare state variables and handle their changes in a concise and straightforward manner.
Basic Syntax
To use the useState
hook, you need to import it from the 'react' package. The basic syntax for using useState
is as follows:
import React, { useState } from 'react';
function MyComponent() {
const [stateVariable, setStateVariable] = useState(initialValue);
// Your component logic here
return (
// JSX to render
);
}
- stateVariable
: This is the state variable you want to create or manage. It holds the current state value.
- setStateVariable
: This function is used to update the state. You can call it with a new value to trigger a re-render of your component.
- initialValue
: This is the initial value for your state variable.
Using useState
Let's explore some common use cases for the useState
hook and understand how it simplifies state management in React applications:
1. Managing a Basic State Variable
Consider a simple counter component:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
In this example, we initialize a count
state variable with an initial value of 0. The setCount
function allows us to update the count
state whenever the "Increment" button is clicked.
2. Managing Complex State
The useState
hook is not limited to simple numeric values; it can also handle more complex state, such as objects or arrays:
import React, { useState } from 'react';
function UserProfile() {
const [user, setUser] = useState({
name: '',
email: ''
});
const handleNameChange = (e) => {
setUser({ ...user, name: e.target.value });
};
const handleEmailChange = (e) => {
setUser({ ...user, email: e.target.value });
};
return (
<div>
<input type="text" placeholder="Name" value={user.name} onChange={handleNameChange} />
<input type="email" placeholder="Email" value={user.email} onChange={handleEmailChange} />
</div>
);
}
Here, we manage a user
object with name
and email
properties. We use the spread operator to update only the relevant part of the state when input fields change.
Common Patterns
Here are some common patterns and best practices when using the useState
hook:
1. Multiple State Variables
It's common to use multiple useState
calls for different pieces of state within a component. This helps keep your code organized and focused:
const [count, setCount] = useState(0);
const [text, setText] = useState('');
const Counter = () => {
const [count, setCount] = useState(0);
const [text, setText] = useState('');
return (
<div>
<p>Count: {count}</p>
<p>Text: {text}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
<input type="text" value={text} onChange={(e) => setText(e.target.value)} />
</div>
);
};
2. Functional Updates
When updating state based on the previous state, use a functional update to ensure accuracy, especially when dealing with asynchronous updates:
setCount((prevCount) => prevCount + 1);
const Counter = () => {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount((prevCount) => prevCount + 1)}>Increment</button>
</div>
);
};
3. Object and Array Updates
When updating objects or arrays, make sure to create a new object or array with the changes rather than mutating the original:
// For objects
setUser({ ...user, name: 'New Name' });
// For arrays
setItems([...items, newItem]);
setUser({ ...user, name: 'New Name' });
const UserForm = () => {
const [user, setUser] = useState({ name: '', email: '' });
return (
<form>
<input type="text" placeholder="Name" value={user.name} onChange={(e) => setUser({ ...user, name: e.target.value })} />
<input type="email" placeholder="Email" value={user.email} onChange={(e) => setUser({ ...user, email: e.target.value })} />
<button type="submit">Submit</button>
</form>
);
};
4. Destructuring State
Destructure state variables for cleaner code:
const { name, email } = user;
Conclusion
The useState
hook is a powerful tool for managing state in your React components. By following the syntax, patterns, and best practices outlined in this comprehensive guide, you'll be well-equipped to handle state in a clear and efficient manner, leading to more maintainable and error-free React applications. Experiment with useState
in your projects to become proficient in using this essential hook for building dynamic web applications. Happy coding! ๐๐ฉโ๐ป๐จโ๐ป
Subscribe to my newsletter
Read articles from Opini Isaac directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
