To reverse an array or string - DSA Question 2
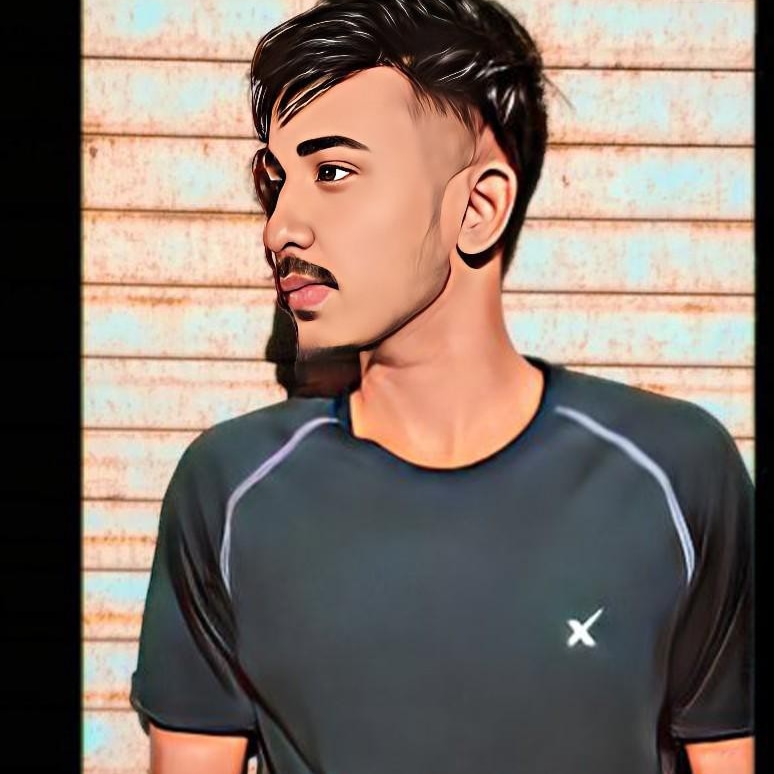
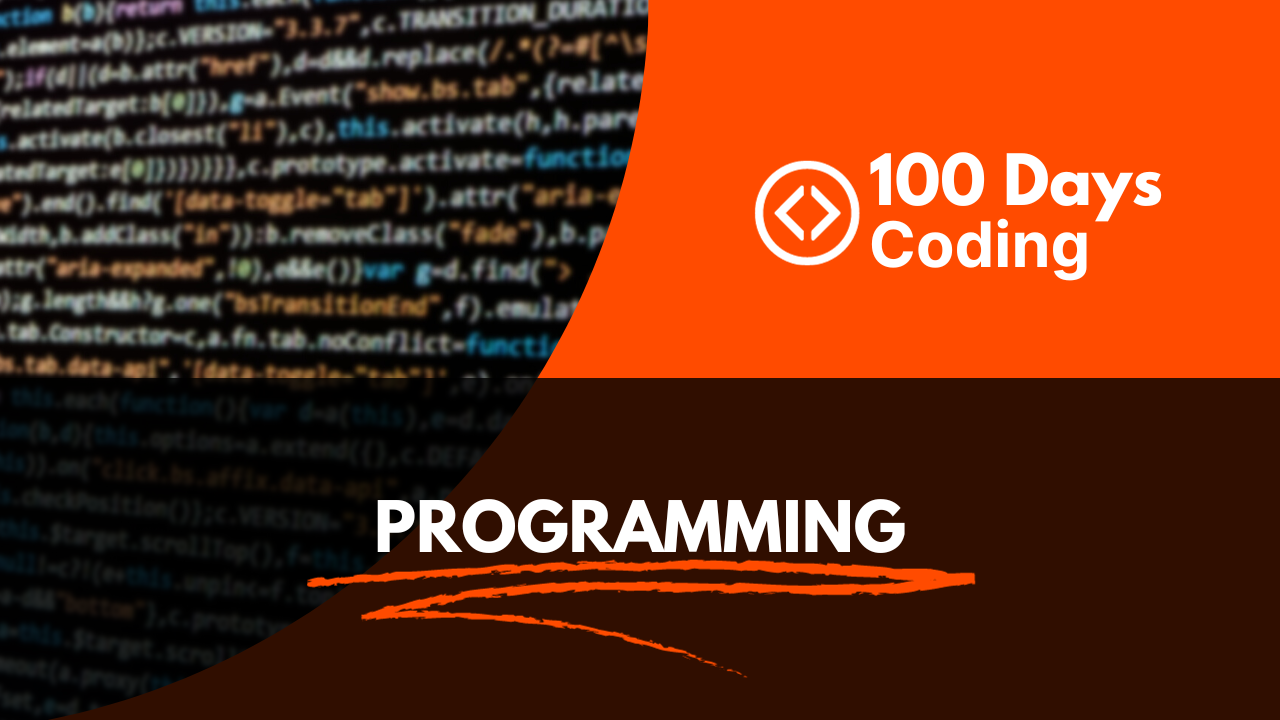
Title: "Java Program to Reverse an Array or String: A Step-by-Step Guide"
Introduction: Reversing an array or string is a common task in programming. In this tutorial, we will explore how to write a Java program to reverse an array or string efficiently. Whether you're a beginner or an experienced programmer, this guide will walk you through the process, providing clear explanations and examples.
Understanding the Problem:
The task is simple: given an array or string, the goal is to reverse its elements. For example, if the input is {1, 2, 3}
, the output should be {3, 2, 1}
. Similarly, for the input {4, 5, 1, 2}
, the output should be {2, 1, 5, 4}
.
Approach:
Initialize two pointers:
start
pointing to the first element (index 0) andend
pointing to the last element (indexarray.length - 1
).Swap the elements at the
start
andend
pointers.Move the
start
pointer to the right and theend
pointer to the left.Continue swapping and moving pointers until the
start
pointer is less than theend
pointer.
Java Program to Reverse an Array:
Here's a Java program to reverse an array:
public class ReverseArray {
public static void main(String[] args) {
int[] arr = {1, 2, 3, 4, 5};
int start = 0;
int end = arr.length - 1;
int temp;
// Reversing the array
while (start < end) {
// Swap elements at start and end
temp = arr[start];
arr[start] = arr[end];
arr[end] = temp;
// Move the pointers towards the center
start++;
end--;
}
// Printing the reversed array
System.out.print("Reversed Array: ");
for (int num : arr) {
System.out.print(num + " ");
}
}
}
Explanation:
The program initializes
start
at the beginning of the array andend
at the end of the array.Inside the
while
loop, it swaps the elements at thestart
andend
pointers, effectively reversing the array.The loop continues until the
start
pointer is less than theend
pointer.After the loop, the program prints the reversed array.
Step-by-Step Explanation:
Array Initialization:
#javaCopy code int[] arr = {1, 2, 3, 4, 5};
Here, we initialize an integer array with values
{1, 2, 3, 4, 5}
. You can replace these values with any integers of your choice.Pointer Initialization:
#javaCopy code int start = 0; int end = arr.length - 1;
We set up two pointers,
start
andend
, pointing to the beginning and end of the array, respectively. Theend
pointer is initialized toarr.length - 1
because array indices start from 0.Array Reversal:
#javaCopy code while (start < end) { // Swap elements at start and end temp = arr[start]; arr[start] = arr[end]; arr[end] = temp; // Move the pointers towards the center start++; end--; }
In the
while
loop, the elements at thestart
andend
pointers are swapped using a temporary variabletemp
.The
start
pointer moves towards the end of the array, and theend
pointer moves towards the beginning.The loop continues until the
start
pointer is less than theend
pointer.
Printing the Reversed Array:
#javaCopy code System.out.print("Reversed Array: "); for (int num : arr) { System.out.print(num + " "); }
Finally, the program prints the reversed array using a
for-each
loop. The output will be:Reversed Array: 5 4 3 2 1
.
This program efficiently reverses an array in Java by swapping elements from the start and end pointers until they meet at the center of the array. Understanding this basic algorithm is fundamental in programming and can be applied to various problem-solving scenarios. Feel free to experiment with different arrays and observe how the program reverses them.
Conclusion:
In this tutorial, we've learned how to reverse an array in Java efficiently. Understanding this fundamental programming concept is essential, as it forms the basis for solving more complex problems. Feel free to modify the program and experiment with different data types and structures.
Feel free to ask if you have any questions or need further clarifications. Happy coding! ๐
Keywords: Java program to reverse array, reverse an array in Java, reverse a string in Java, Java array reversal, programming tutorial, Java programming, coding examples.
Subscribe to my newsletter
Read articles from Harsh Raj directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
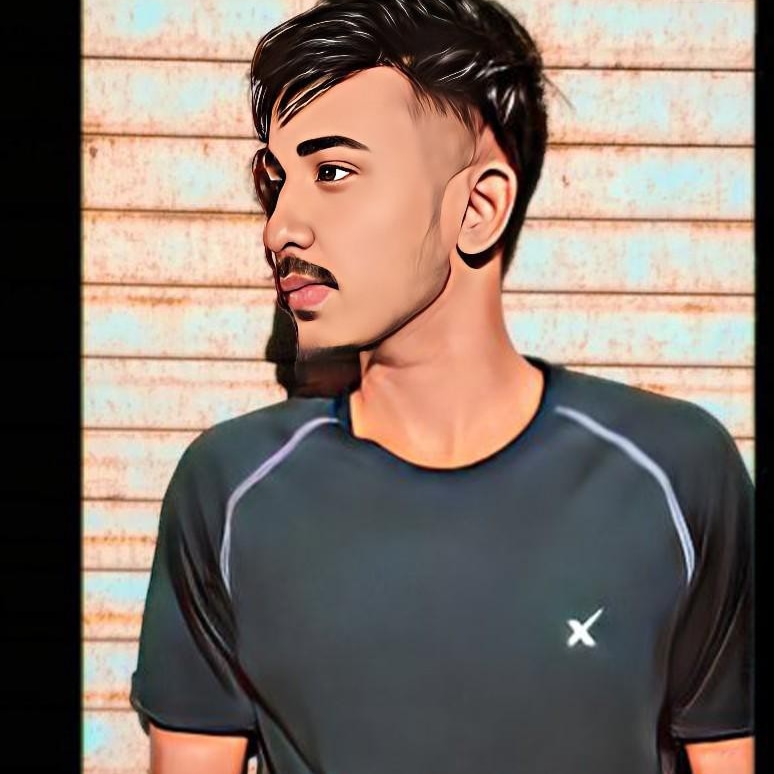