Migrating from create-react-app to Vite with Jest and Browserslist
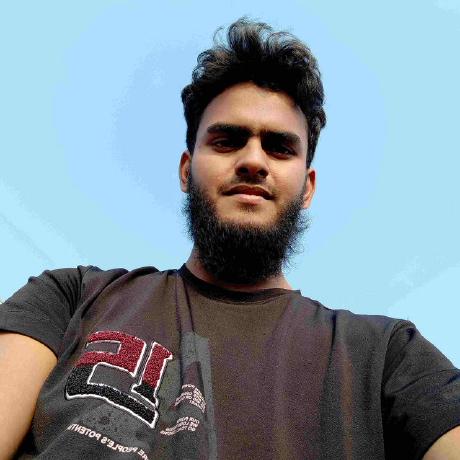
Table of contents
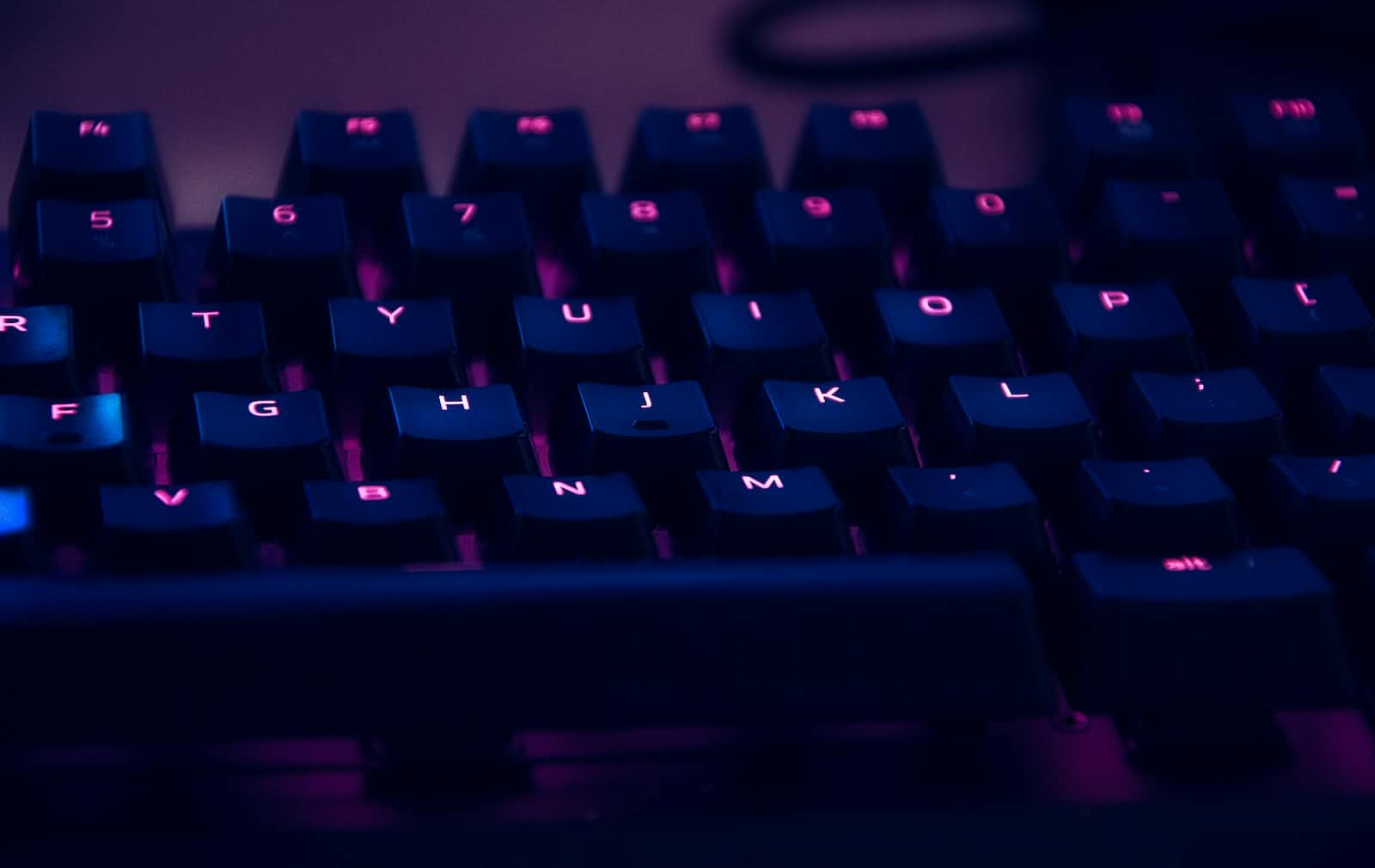
In the ever-evolving world of React development, staying updated with the latest tools and best practices is paramount. One significant change is the transition away from create-react-app (CRA) as the preferred bundler for new React applications. The React team, in collaboration with the community, recognizes that while CRA is an excellent starting point, it lacks the flexibility necessary for configuring and managing large and complex projects. Instead, the team now advocates for production-grade React frameworks like Next.js, Remix, Gatsby, or Expo. However, for those seeking a more customizable build process, Vite and Parcel stand out as top choices.
In this comprehensive guide, we'll walk you through the process of migrating a production-ready application from CRA to Vite. We'll delve into the reasoning behind each step, discuss how to retain Jest for testing, and address the peculiarities of Browserslist when working with Vite.
Step 1: Install Vite and Essential Plugins:
yarn add vite @vitejs/plugin-react vite-tsconfig-paths
In addition to Vite, we're adding two crucial plugins: @vitejs/plugin-react for fast React development and vite-tsconfig-paths for TypeScript path mapping support.
Step 2: Create a Vite Configuration:
Create a vite.config.ts
file at the root of your project:
import { defineConfig } from 'vite'
import react from '@vitejs/plugin-react'
import viteTsconfigPaths from 'vite-tsconfig-paths'
export default defineConfig({
base: '',
plugins: [react(), viteTsconfigPaths()],
server: {
open: true,
port: 3000,
},
})
This file serves as your Vite configuration, allowing you to customize the build process.
Step 3: Create a Vite Types File Reference:
Create a vite-env.d.ts
file to reference type declarations:
/// <reference types="vite/client" />
This reference file enhances type checks and IntelliSense support when using Vite.
Step 4: Move the index.html File:
Move your index.html
file from the public directory to the root of your project.
Step 5: Update the index.html File:
Remove %PUBLIC_URL%
placeholders from your index.html
file as Vite automatically resolves URLs. Also, add a module script to the bottom of the body tag:
<link rel="icon" type="image/svg+xml" href="/favicon.svg" />
<script type="module" src="/src/index.tsx"></script>
Step 6: Replace CRA with Vite:
- Remove CRA by running:
yarn remove react-scripts
- Update your
package.json
scripts:
{
"scripts": {
"start": "vite",
"build": "tsc && vite build",
"preview": "vite preview"
}
}
- Update your
tsconfig.json
:
{
"compilerOptions": {
"lib": ["dom", "dom.iterable", "esnext"],
"target": "ESNext",
"types": ["vite/client"],
"isolatedModules": true
}
}
- Optionally, update your environment variables if you're using them.
Step 7: Run your Application:
yarn start
With these steps, you've successfully migrated from CRA to Vite, enjoying the benefits of a more flexible and performant development environment.
Step 8: Set Up Jest for Testing (Optional):
- Install Jest and related dependencies:
yarn add -D jest @types/jest ts-jest jest-environment-jsdom
Update your Jest configuration in
package.json
.Create a
__mocks__
directory in the project root.Update your package.json scripts for testing.
Step 9: Update Browserslist Configuration:
- Install
browserslist-to-esbuild
:
yarn add browserslist-to-esbuild
- Configure your Browserslist in the Vite config file.
These steps will resolve compatibility issues between Browserslist and Vite.
Step 10: Add Mocks Directory:
At the root of your project, create a folder named __mocks__
.
Inside the created __mocks__
folder, add a file named styleMock.js
and add the following content:
module.exports = {}
Inside the created __mocks__
folder, add a file named fileMock.js
with the following content:
module.exports = 'test-file-stub'
Step 11: Update the package.json Scripts for Testing:
Replace react-scripts
tests with jest
in your package.json
scripts.
Step 12: Run your Tests:
yarn test
If you face issues related to import.meta
, you can resolve them by moving all your environment
Subscribe to my newsletter
Read articles from Rubel Mehmed directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
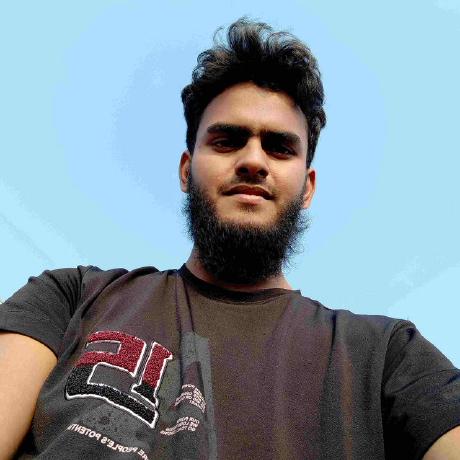
Rubel Mehmed
Rubel Mehmed
I am a Frontend Engineer from Bangladesh