How to code and develop banking software using Truffle, Ethereum, and Ethers.js
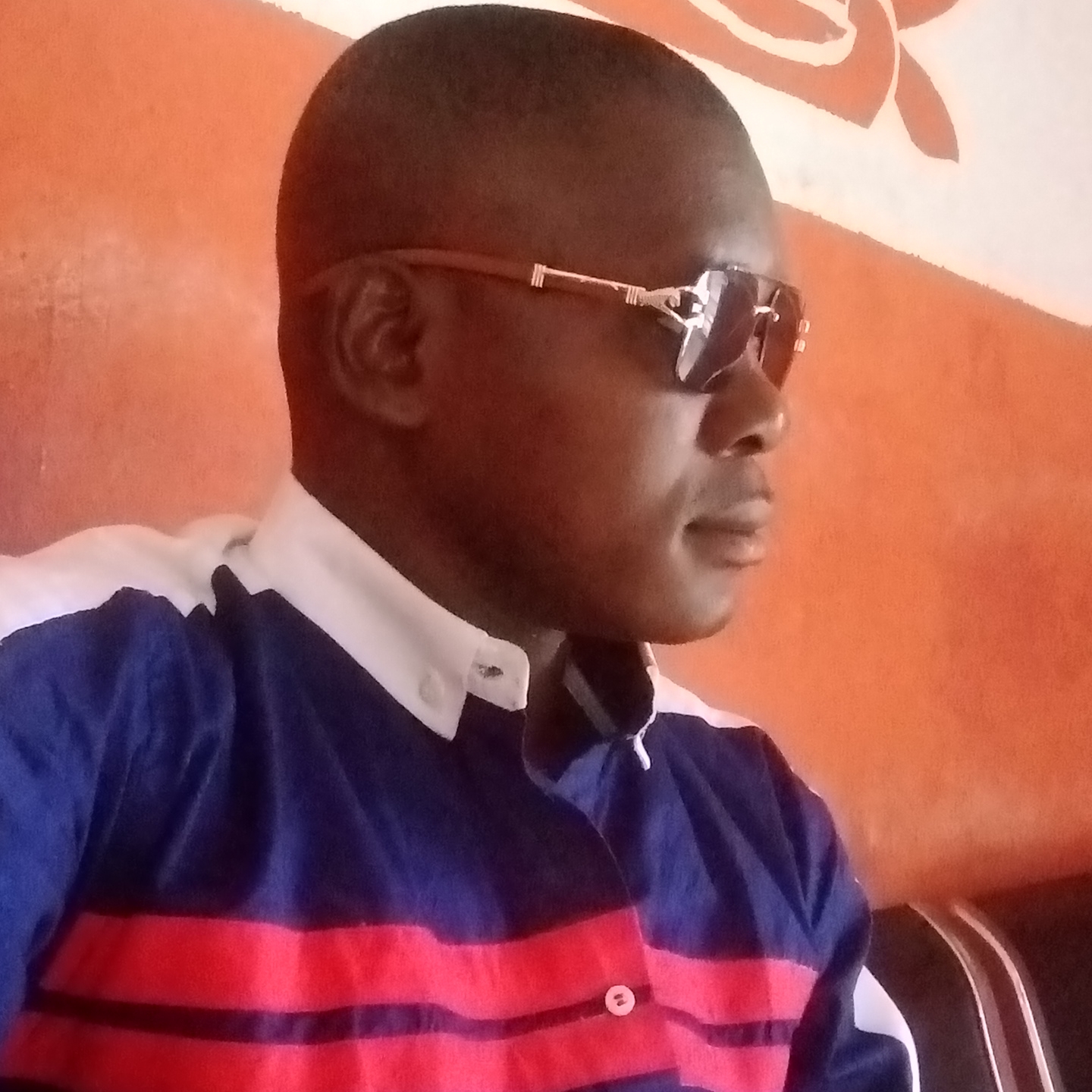
Truffle is a development framework for Ethereum that makes it easy to write, deploy, and test smart contracts. Ethers.js is a JavaScript library for interacting with Ethereum. In this article, I will show you how to code and develop banking software using Truffle, Ethereum, and Ethers.js.
Prerequisites
Node.js
Truffle
Ethers.js
MetaMask
Setting up your project
- Create a new directory for your project and initialize it with Truffle:
mkdir banking-software
cd banking-software
truffle init
- Install the Ethers.js package:
npm install ethers
Creating your smart contracts
- Create a new directory for your smart contracts:
mkdir contracts
- Create a new file called
Banking.sol
in thecontracts
directory. This file will contain the smart contract for your banking software.
pragma solidity ^0.8.0;
contract Banking {
mapping(address => uint256) public balances;
constructor() {}
function deposit() public payable {
balances[msg.sender] += msg.value;
}
function withdraw(uint256 amount) public {
require(balances[msg.sender] >= amount, "Insufficient balance");
balances[msg.sender] -= amount;
msg.sender.transfer(amount);
}
function getBalance() public view returns (uint256) {
return balances[msg.sender];
}
}
This smart contract has three functions:
deposit()
: Allows users to deposit ETH into their account.withdraw()
: Allows users to withdraw ETH from their account.getBalance()
: Returns the user's account balance.
Compiling your smart contracts
Once you have created your smart contracts, you need to compile them into bytecode. To do this, run the following command:
truffle compile
This will create a new directory called build
which contains the compiled bytecode for your smart contracts.
Deploying your smart contracts
Once your smart contracts have been compiled, you can deploy them to the Ethereum network. To do this, run the following command:
truffle migrate
This will deploy your smart contracts to the Ethereum network that you are currently connected to.
Interacting with your smart contracts using Ethers.js
To interact with your smart contracts using Ethers.js, you need to create a new instance of the ethers.Provider
class. This will allow you to connect to the Ethereum network and interact with your smart contracts.
const provider = new ethers.providers.Web3Provider(window.ethereum);
Once you have created a new instance of the ethers.Provider
class, you can create a new instance of the ethers.Contract
class to interact with your smart contract.
const contract = new ethers.Contract("0x1234567890123456789012345678901234567890", Banking.abi, provider);
The first argument to the ethers.Contract
constructor is the address of your smart contract. The second argument is the ABI of your smart contract. The ABI is a JSON file that contains information about the functions and events of your smart contract. You can generate the ABI for your smart contract using the Truffle compiler.
Once you have created a new instance of the ethers.Contract
class, you can call the functions of your smart contract. For example, to deposit ETH into your account, you can call the deposit()
function.
contract.deposit({value: 1000000000000000000});
To withdraw ETH from your account, you can call the withdraw()
function.
contract.withdraw(1000000000000000000);
To get your account balance, you can call the getBalance()
function.
const balance = await contract.getBalance();
Conclusion
In this article, I showed you how to code and develop banking software using Truffle, Ethereum, and Ethers.js. We created a simple banking smart contract and showed you how to deploy it to the Ethereum
Subscribe to my newsletter
Read articles from Remote Crypto directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
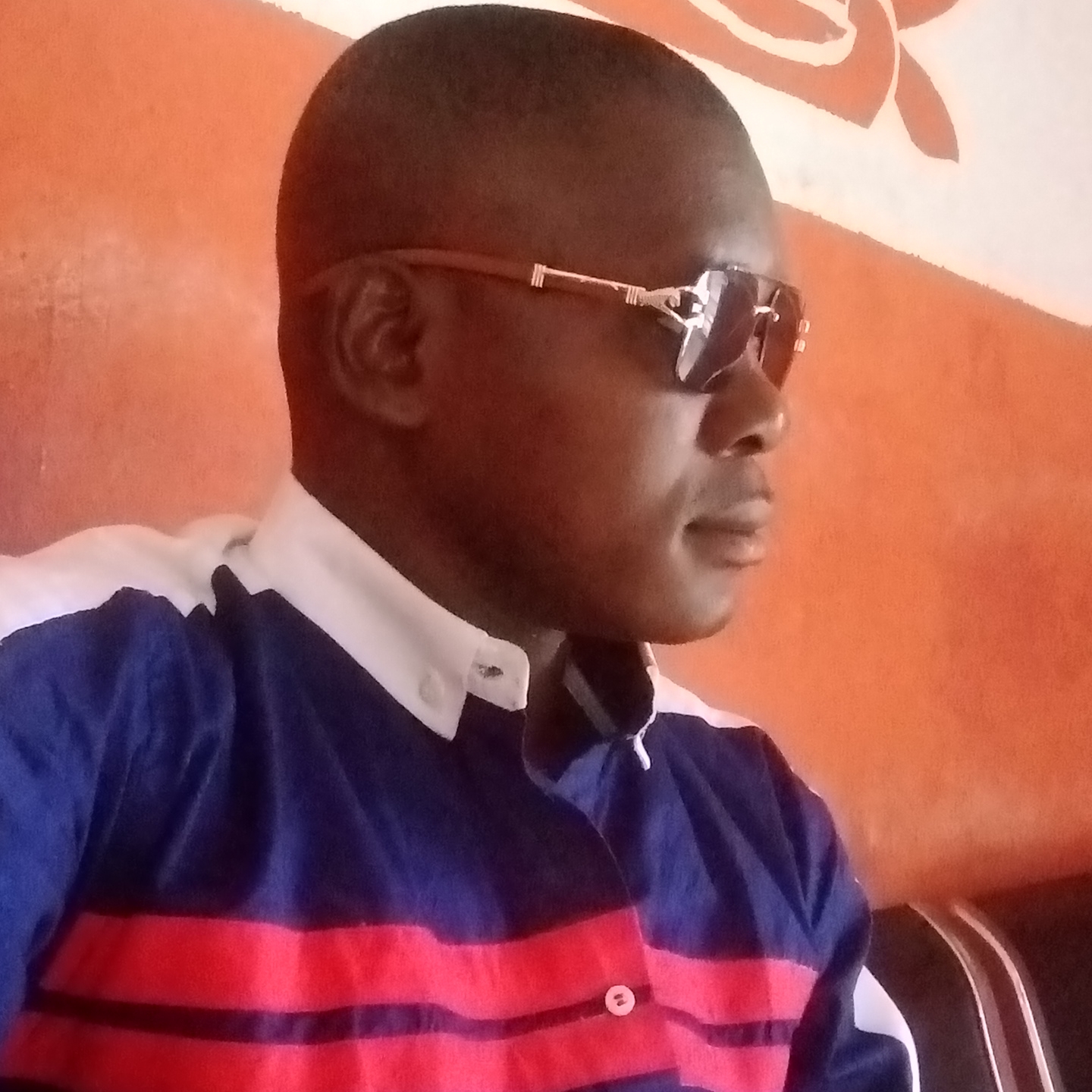
Remote Crypto
Remote Crypto
I am a technical writer, who write on varieties of topics on Cryptocurrency, Blockchain, Web3 and Project documentations.