Unraveling the abrupt behaviour of JavaScript: Understanding the "Why?"

Table of contents
- JavaScript's Event-Driven, Single-Threaded Nature:
- Hoisting and Variable Scope:
- Type Coercion and Implicit Conversions:
- Lexical Scoping and Closure:
- Asynchronous JavaScript and Callbacks:
- JavaScript's Prototypal Inheritance:
- The "this" Keyword:
- Error Handling and Exception Handling:
- The Influence of Browser Differences:
- Conclusion:
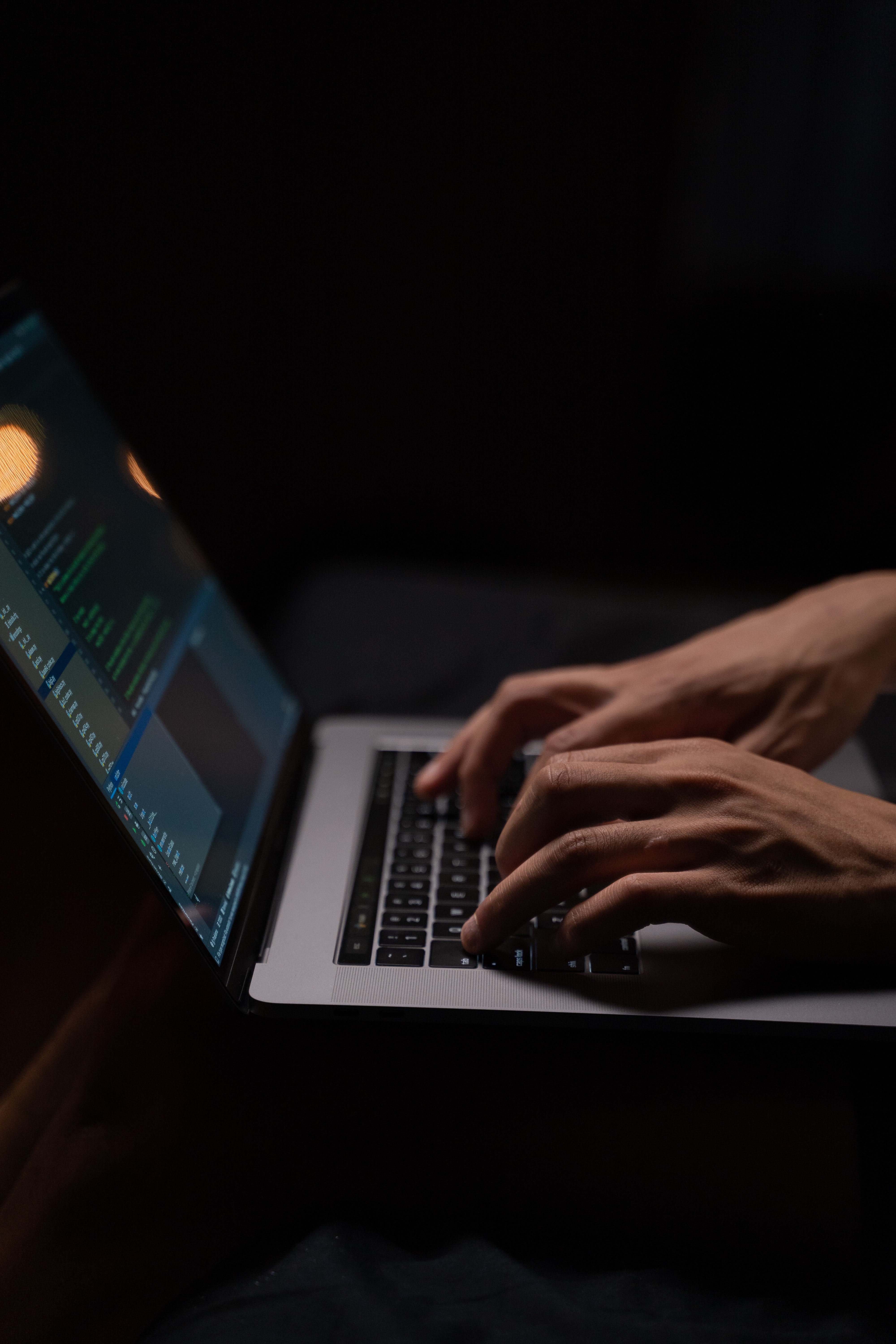
JavaScript is a dynamic and flexible programming language widely used for web development. While it offers many advantages, it is also notorious for its occasional abrupt behaviour, which can perplex developers. In this blog post, we will delve into the reasons behind the seemingly erratic behaviour of JavaScript and shed light on the underlying concepts that contribute to this behaviour. By understanding the "why" behind these behaviours, developers can navigate the language more effectively and write robust, reliable code.
JavaScript's Event-Driven, Single-Threaded Nature:
JavaScript's event-driven, single-threaded nature sets the stage for its behaviour. The event loop is a crucial component that manages asynchronous events. However, JavaScript executes code in a single thread, leading to certain limitations and considerations when dealing with synchronous and asynchronous operations.
Example -
console.log("Start");
setTimeout(() => {
console.log("Timeout callback");
}, 0);
console.log("End");
/* output : Start
End
Timeout callback */
In this example, we have a setTimeout
function that schedules a callback to be executed after 0 milliseconds. However, the "Timeout callback" is not immediately printed on the console. Instead, "Start" and "End" are printed first. This is because JavaScript's event loop allows the main thread to continue executing while waiting for the callback to be triggered. This demonstrates how JavaScript handles asynchronous events and continues with other tasks without blocking the execution flow.
Hoisting and Variable Scope:
Hoisting is a JavaScript behaviour where variable and function declarations are moved to the top of their respective scopes during the compilation phase. It's important to understand the difference between variable declaration and initialization and how hoisting impacts them.
Example -
console.log(myVariable); // Output: undefined
var myVariable = 10;
console.log(myVariable); // Output: 10
In this example, we try to log the value of myVariable
before it is assigned a value. Despite the variable being declared afterwards, JavaScript's hoisting behaviour moves the variable declaration to the top of the scope, making it accessible throughout the scope. However, until the variable is explicitly assigned a value, it holds the value undefined
.
Type Coercion and Implicit Conversions:
JavaScript has a loose typing system, allowing implicit type conversions. Type coercion occurs when JavaScript automatically converts values from one type to another in certain situations. This behaviour can lead to unexpected results if not handled carefully.
Example -
console.log(5 + "5"); // Output: "55"
console.log(5 - "2"); // Output: 3
JavaScript performs implicit type conversions in certain operations. In the first line, the +
operator is used to concatenate a number and a string. JavaScript converts the number to a string and concatenates them, resulting in the string "55"
. In the second line, the -
operator is used between a number and a string. JavaScript converts the string to a number and performs subtraction, resulting in the number 3
.
Lexical Scoping and Closure:
Closures are a powerful feature of JavaScript that allows functions to retain access to variables from their outer scopes, even after those scopes have finished executing. While closures offer great flexibility and can be used to create private variables and functions, they can also lead to unexpected behaviour if not used carefully.
Example -
function outerFunction() {
var outerVariable = "I'm outer";
function innerFunction() {
console.log(outerVariable);
}
return innerFunction;
}
var closureFunction = outerFunction();
closureFunction(); // Output: "I'm outer"
In this example, we have an outer function outerFunction
that defines a variable outerVariable
. Inside outerFunction
, there's an inner function innerFunction
that can access the outerVariable
due to closure. The inner function captures and maintains a reference to the variable even after the outer function has finished executing. When closureFunction
is invoked, it logs the value of outerVariable
, demonstrating how closures work in JavaScript.
Asynchronous JavaScript and Callbacks:
Asynchronous operations are common in JavaScript, especially when interacting with servers or performing time-consuming tasks. Callbacks are a prevalent pattern for handling asynchronous code, but they can lead to callback hell and make code harder to read and maintain.
Example -
function fetchData(callback) {
setTimeout(() => {
const data = { name: "zaid", age: 22 };
callback(data);
}, 2000);
}
function processData(data) {
console.log("Processing data:", data);
}
fetchData(processData);
// output : Processing data: { name: 'zaid', age: 22 }
In this example, the fetchData
function simulates fetching data asynchronously using setTimeout
. It accepts a callback function as an argument. After a delay of 2000 milliseconds, the callback function (processData
) is invoked with the fetched data. This demonstrates the callback pattern in JavaScript, where functions are passed as arguments and executed once an asynchronous operation is complete.
JavaScript's Prototypal Inheritance:
JavaScript employs a unique approach to object-oriented programming through prototypal inheritance. Objects can inherit properties and methods from other objects, forming a prototype chain.
Example -
function Person(name) {
this.name = name;
}
Person.prototype.greet = function () {
console.log("Hello, my name is " + this.name);
};
var zaid = new Person("zaid");
zaid.greet(); // Output: "Hello, my name is zaid"
In this example, we define a constructor function Person
that sets the name
property. We then add a zaid
method to the Person
prototype, which can be shared across all instances of Person
. The zaid
object is created using the new
keyword, and it inherits the greet
method from the prototype. Invoking zaid.greet()
logs a greeting message with the name "zaid", showcasing JavaScript's prototypal inheritance.
The "this" Keyword:
The behaviour of the "this" keyword in JavaScript can be perplexing, as its value depends on how a function is invoked. Understanding the different contexts in which "this" can be used and how it is determined is crucial. Employing techniques like explicit binding or using arrow functions can help manage "this" effectively.
Example -
const person = {
name: "zaid",
sayHello: function () {
console.log("Hello, my name is " + this.name);
},
};
person.sayHello(); // Output: "Hello, my name is zaid"
const helloFunction = person.sayHello;
helloFunction(); // Output: "Hello, my name is undefined"
In this example, we have an object person
with a sayHello
method that references this.name
. When person.sayHello()
is invoked, this
refers to the person
object, so it logs the appropriate greeting message. However, when the method is assigned to helloFunction
and invoked separately, this
no longer points to the person
object, resulting in undefined
for this.name
.
Error Handling and Exception Handling:
Errors and exceptions are an inherent part of JavaScript. JavaScript provides the try-catch statement for handling errors and preventing program crashes. Proper error handling is essential for debugging and maintaining code quality.
Example -
try {
// Code that may throw an error
const result = 10 / 0;
console.log(result); // This line is never executed
} catch (error) {
console.log("An error occurred:", error);
}
In this example, we have code that attempts to divide a number by zero, which throws a TypeError
and crashes the program. However, by wrapping the code in a try
block, we can catch the error using the catch
block. The program continues executing after the error, allowing us to handle it gracefully and log an appropriate error message.
The Influence of Browser Differences:
JavaScript is executed within different web browsers, and each browser may have its quirks and inconsistencies in implementing JavaScript features. Cross-browser compatibility is crucial for ensuring that web applications work correctly across different environments.
Example -
var element = document.getElementById("myElement");
element.style.color = "red";
In this example, we try to access an element with the id
"myElement" using document.getElementById
. We then attempt to change the color of the element by setting the style.color
property to "red". However, browser implementations may have different behaviours or variations in how they handle certain DOM operations. Cross-browser compatibility testing and using appropriate workarounds or libraries can help mitigate these inconsistencies and ensure consistent behaviour across different browsers.
Conclusion:
JavaScript's abrupt behaviour can be attributed to its unique design choices and the challenges inherent in its event-driven, single-threaded nature. By understanding the underlying concepts and factors that contribute to these behaviours, developers can anticipate and mitigate potential issues. Armed with this knowledge, developers can write more robust and predictable JavaScript code, ultimately improving the quality and reliability of their web applications.
About Me:
'' I inhibit a passion to learn about new technologies with a belief to write about my favorite topics''
Subscribe to my newsletter
Read articles from Mohd Zaid Multani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
