Mastering the Power of TypeScript with React Query: An In-Depth Guide
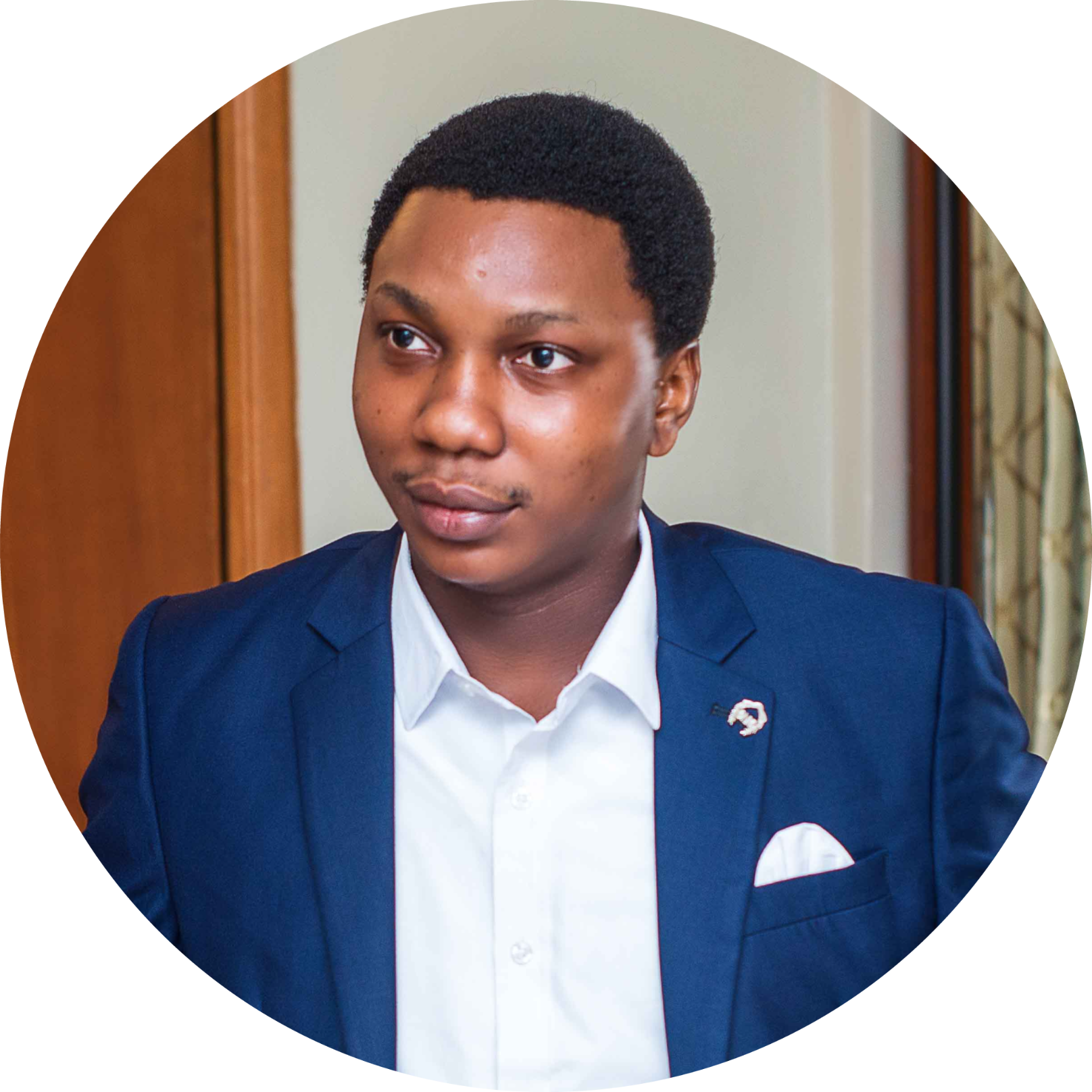
Table of contents
- Setting up React Query with TypeScript: A Step-by-step Guide
- Typing Query and Mutation Hooks
- Typing Query Function
- TypeScript and React Query's Infinite Queries
- Handling Error and Loading States with TypeScript
- TypeScript with React Query's Devtools
- Optimistic Updates with TypeScript
- Using TypeScript with React Query's useQueryClient Hook
- Conclusion
Have you ever faced challenges in managing server states in your React applications? You're not alone. In the ever-evolving world of web development, developers continuously seek efficient, robust, and type-safe methods for handling server states in React applications.
React Query and TypeScript—a dynamic duo that can considerably streamline this process. In this section, we'll delve deep into how you can fuse the prowess of these technologies to establish a type-safe server state synchronization for your React applications.
React Query is a beacon in data synchronization, tailored explicitly for React, ensuring optimal server state management. Conversely, TypeScript is a statically typed superset of JavaScript, adding muscle to the JavaScript ecosystem with its rigorous type-checking and object-oriented programming capabilities.
Paired with React Query, TypeScript promises an elevated development experience—enhancing auto-completion, making the code speak for itself, and drastically reducing runtime errors.
So, what's in store for you? We'll be navigating the step-by-step process of setting up a project with React Query and TypeScript. This section covers everything from typing your query functions to gracefully handling error and loading states.
Whether you're a seasoned developer or just dipping your toes into the TypeScript or React Query waters, a wealth of insights awaits you. Ready to dive deep? Let's unlock the transformative benefits of melding TypeScript with React Query!
Setting up React Query with TypeScript: A Step-by-step Guide
Embarking on a journey to integrate React Query with TypeScript? Rest assured, it's not as daunting as it might sound. This section illuminates the pathway, guiding you meticulously from installation to penning down your first typed query.
Project Initialization
Starting from scratch? Easily kick off with a new React project equipped with a TypeScript template. Just use the command:
npx create-react-app my-app --template typescript
If you already have a React project and wish to add TypeScript to it, you can do so by first installing TypeScript as a dev dependency using the command:
npm install --save-dev typescript
Installing React Query
Now that your project's foundation is laid, let’s layer it with React Query. You can anchor it using either npm or yarn. For npm enthusiasts:
npm install react-query
Setting up TypeScript with React Query
Once you've installed React Query, the next step is configuring TypeScript to complement it. Ensure there's a
tsconfig.json
file in your project's root directory. This file would already be present if you initiated your project with the TypeScript template. If not, initiate it with the following command:tsc --init
The essential TypeScript compiler options for React Query integration are
strict: true
—activating a comprehensive range of type-checking—andnoImplicitAny: true
, which prohibiting the use of implicit 'any' types.Writing Your First Typed Query
With everything set up, you can now write your first typed query. Here's a simple example of a typed
useQuery
hook:import { useQuery } from 'react-query'; type Post = { id: string; title: string; body: string; }; async function fetchPosts(): Promise<Post[]> { const response = await fetch('/api/posts'); if (!response.ok) { throw new Error('Problem fetching posts'); } const posts: Post[] = await response.json(); return posts; } function Posts() { const { data, error, isLoading } = useQuery<Post[]>('posts', fetchPosts); if (isLoading) { return <div>Loading...</div>; } if (error) { return <div>Error: {error.message}</div>; } return ( <ul> {data?.map(post => ( <li key={post.id}>{post.title}</li> ))} </ul> ); }
In the above instance, the Post
type defines the structure of a post object. Concurrently, the fetchPosts
function is a typed asynchronous function retrieves posts from a specified API endpoint, promising to conclude with a post array.
The useQuery
hook is applied in the Posts
component associated with the 'posts' key and fetchPosts
function. Its outcome is typed as Post[]
.
These steps will set you up for a smooth and efficient development experience with React Query and TypeScript. The remaining sections of this guide will delve into more advanced concepts and practices to help you harness the full potential of these technologies.
Typing Query and Mutation Hooks
Typing your query and mutation hooks with TypeScript is a practice that will significantly enhance your developer experience.
It ensures type safety when fetching, updating, or deleting data and provides helpful autocompletion and inline documentation in your editor. Let's look at how to provide types for useQuery
and useMutation
, two commonly used hooks in React Query.
Typing
useQuery
HookTo use TypeScript with
useQuery
, you need to provide it with the types of the query function's result, error, and argument. Let's consider the following example where we fetch a list of posts:import { useQuery } from 'react-query'; type Post = { id: string; title: string; body: string; }; async function fetchPosts(): Promise<Post[]> { const response = await fetch('/api/posts'); if (!response.ok) { throw new Error('Problem fetching posts'); } const posts: Post[] = await response.json(); return posts; } function Posts() { const { data, error, isLoading } = useQuery<Post[], Error>('posts', fetchPosts); if (isLoading) { return <div>Loading...</div>; } if (error) { return <div>Error: {error.message}</div>; } return ( <ul> {data?.map(post => ( <li key={post.id}>{post.title}</li> ))} </ul> ); }
In this example, the
useQuery
hook is typed withPost[]
andError
. ThePost[]
type corresponds to the type of data that thefetchPosts
function returns, andError
corresponds to the type of error that the function may throw.Typing
useMutation
HookSimilar to
useQuery
,useMutation
the types of the mutation function's result, error, and argument must be provided. Consider the following example where we update a post:import { useMutation } from 'react-query'; type Post = { id: string; title: string; body: string; }; async function updatePost(post: Post): Promise<Post> { const response = await fetch(`/api/posts/${post.id}`, { method: 'PUT', headers: { 'Content-Type': 'application/json', }, body: JSON.stringify(post), }); if (!response.ok) { throw new Error('Problem updating post'); } const updatedPost: Post = await response.json(); return updatedPost; } function PostEditor({ post }: { post: Post }) { const mutation = useMutation<Post, Error, Post>(updatePost); }
In this example,
useMutation
is typed withPost
,Error
, andPost
. The firstPost
type corresponds to the type of data that theupdatePost
function returns,Error
corresponds to the type of error that the function may throw, and the secondPost
type corresponds to the type of the function's argument.
Providing types for your query and mutation hooks enhances your development workflow with robust type checking, helpful autocompletion, and inline documentation, making fetching, updating, or deleting data safer and more efficient.
Typing Query Function
Providing types for the query function is an integral part of setting up TypeScript with React Query. The query function is the function that you pass to useQuery
or useMutation
, and it's responsible for fetching, updating, or deleting data. Let's explore how to define types for this function.
A query function can have any name; however, it should be an asynchronous function that returns a promise. The promise type should be the same as the type of data you expect to receive from your API.
For instance, if we have an API that returns a list of posts, we might define our post type and query function as follows:
type Post = {
id: string;
title: string;
body: string;
};
async function fetchPosts(): Promise<Post[]> {
const response = await fetch('/api/posts');
if (!response.ok) {
throw new Error('Problem fetching posts');
}
const posts: Post[] = await response.json();
return posts;
}
In this example, fetchPosts
is a query function that returns a promise resolving to Post[]
, an array of Post
objects. The Post
type defines the shape of a post object, which corresponds to the data structure that the API returns.
When we use this query function with useQuery
, we provide Post[]
as the type for useQuery
:
const { data, error, isLoading } = useQuery<Post[], Error>('posts', fetchPosts);
This ensures that data
is typed as Post[] | undefined
, error
is typed as Error | null
, and isLoading
is typed as boolean
. Now, TypeScript knows the types of these variables, and it can provide autocompletion and type checking, making our code more reliable and easier to work with.
Similarly, you can provide types for mutation functions when using useMutation
. Remember, the types you define should always align with the data structure you expect from your API.
TypeScript can catch type-related errors early in development by typing your query functions, leading to more robust and reliable code.
TypeScript and React Query's Infinite Queries
Another advanced feature to consider is the infinite queries provided by React Query, which allow us to handle paginated data from APIs by automatically managing page cursors and concatenating page data.
As with standard queries, using TypeScript with infinite queries provides robust type-checking and improves our developer experience. Let's look at how to type responses and variables for infinite queries.
Defining Our Data Types
Suppose we're fetching paginated posts from an API. We would first define the type of our
Post
andPage
data.type Post = { id: string; title: string; body: string; }; type Page = { posts: Post[]; nextPage: number; hasMore: boolean; };
In this example, each
Page
object includes an array ofPost
objects, anextPage
number, and ahasMore
boolean indicating if there are more pages to fetch.Typing the Fetch Function
We then define our fetch function, which should return a
Promise<Page>
.async function fetchPosts(pageNumber = 0): Promise<Page> { const response = await fetch(`/api/posts?page=${pageNumber}`); if (!response.ok) { throw new Error('Problem fetching posts'); } const page: Page = await response.json(); return page; }
Typing
useInfiniteQuery
HookFinally, we can use
useInfiniteQuery
with our fetch function and provide thePage
andError
types to the Hook.const { data, error, fetchNextPage, hasNextPage, isFetchingNextPage, isLoading, } = useInfiniteQuery<Page, Error>('posts', fetchPosts, { getNextPageParam: (lastPage) => lastPage.nextPage, });
In this example,
useInfiniteQuery
returns an object containing methods and variables for managing and displaying the infinite list of posts. By providing thePage
andError
types touseInfiniteQuery
, we ensure type safety and get helpful autocompletion and inline documentation for these methods and variables.
When you use TypeScript with React Query's Infinite Queries, you can handle paginated data more confidently. The robust typing system minimizes bugs, enhancing your development process.
Handling Error and Loading States with TypeScript
Effective handling of error and loading states is critical to any application fetching server data. Combined with TypeScript, React Query provides an efficient way to handle these states, making your code more robust and readable. This section will illustrate how to type the error
and isLoading
variables returned by useQuery.
Let's consider an example where we are fetching a list of posts.
First, we define our data type and the fetch function:
type Post = {
id: string;
title: string;
body: string;
};
async function fetchPosts(): Promise<Post[]> {
const response = await fetch('/api/posts');
if (!response.ok) {
throw new Error('Problem fetching posts');
}
const posts: Post[] = await response.json();
return posts;
}
Next, we use useQuery
in our component and provide types for the query result and error:
const { data, error, isLoading } = useQuery<Post[], Error>('posts', fetchPosts);
By providing types to useQuery
, we can effectively manage the loading and error states:
function Posts() {
const { data, error, isLoading } = useQuery<Post[], Error>('posts', fetchPosts);
if (isLoading) {
return <div>Loading...</div>;
}
if (error) {
return <div>Error: {error.message}</div>;
}
if (!data || data.length === 0) {
return <div>No posts available at the moment.</div>;
}
return (
<ul>
{data.map(post => (
<li key={post.id}>{post.title}</li>
))}
</ul>
);
}
In this example, isLoading
is a boolean that indicates whether the query is in a "loading" state. If isLoading
is true, we display a loading message. Once the data is loaded, isLoading
becomes false.
error
is of type Error | null
and will contain an error object if the query fails for some reason. If there's an error, we display the error message.
Using TypeScript to type your error and loading states makes your code more robust by ensuring that you handle the correct data types. Additionally, it improves the developer experience by providing helpful autocompletion and inline documentation in your code editor.
TypeScript with React Query's Devtools
For those unfamiliar, React Query Devtools is an indispensable debugging and monitoring tool specifically tailored for React Query. It gives developers a robust interface to visualize, debug, and interact with their application's queries and mutations. This makes it an invaluable asset for anyone using React Query.
When combined with TypeScript, the experience is further elevated. TypeScript further enhances this experience by providing strong type safety and autocompletion, which can be particularly useful when debugging the state of your queries and mutations.
This section will cover how TypeScript can be utilized effectively with React Query Devtools.
To get started, let's assume you have an application where you are fetching a list of users. Let's define the type for our User
and use useQuery
to fetch the data.
type User = {
id: string;
name: string;
email: string;
};
const { data, error, isLoading } = useQuery<User[], Error>('users', fetchUsers);
Now, to install and include React Query Devtools for your project, consider following these steps:
- Install the dev tools package:
npm install --save react-query/devtools
- Include the
ReactQueryDevtools
Component in your main component:
import { ReactQueryDevtools } from 'react-query/devtools';
function App() {
return (
<QueryClientProvider client={queryClient}>
{/* rest of your application */}
<ReactQueryDevtools initialIsOpen={false} />
</QueryClientProvider>
);
}
When you run your application, you can access React Query Devtools, which displays the state of all your queries and mutations. If you click on the 'users' query, you will see the query state, including the data fetched, the loading state, and any errors.
Because we are using TypeScript, the data shown in the Devtools is strongly typed. This means that if you inspect the query data in your code editor, you can see the type information, which can help you understand the data structure and fix any type-related issues.
Furthermore, TypeScript will ensure that any changes you make in your data types will be reflected in the Devtools, helping to keep your types and data consistent.
In conclusion, using TypeScript with React Query's Devtools provides a robust debugging toolset. It helps you catch type-related errors, understand your data structure better, and keep your data types and actual data consistent.
Optimistic Updates with TypeScript
Before delving into the specifics of implementing optimistic updates with TypeScript, let's briefly understand what "optimistic updates" mean. In the world of web applications, there's often a delay between sending a request to a server and receiving a response.
Optimistic updates are a UI pattern where we simulate the desired result of an action immediately, without waiting for the server's confirmation. This provides users with a perception of speed and responsiveness.
However, if the server's response differs from our optimistic prediction, we would correct the UI to reflect the actual state.
it enhances the user experience by providing immediate feedback before receiving server responses. Combined with TypeScript, you benefit from a rigorous typing system ensuring data integrity.
Consider an example where you're updating a post. Define the data type first:
type Post = {
id: string;
title: string;
body: string;
};
Then, an updatePost
Mutation function:
async function updatePost(post: Post): Promise<Post> {
// function implementation
}
The next step involves creating an optimistic update when calling the mutation. This would look something like this:
const mutation = useMutation<Post, Error, Post>(updatePost, {
onMutate: async (newPost) => {
const previousPosts = queryClient.getQueryData<Post[]>('posts');
queryClient.setQueryData<Post[]>('posts', (oldPosts) =>
oldPosts?.map(post => (post.id === newPost.id ? newPost : post))
);
return { previousPosts };
},
onError: (error, newPost, context) => {
if (context?.previousPosts) {
queryClient.setQueryData('posts', context.previousPosts);
}
},
onSettled: () => {
queryClient.invalidateQueries('posts');
},
});
This way, TypeScript assists in managing types while working with React Query's optimistic updates, ensuring a robust and efficient coding experience.
Using TypeScript with React Query's useQueryClient Hook
React Query's useQueryClient
Hook is instrumental in directly accessing the QueryClient
instance and its methods within your components. With TypeScript, you can strongly type these interactions, adding robustness and clarity to your code.
Here's an example of using useQueryClient
with TypeScript:
import { useQueryClient, QueryClient } from 'react-query';
const MyComponent = () => {
const queryClient: QueryClient = useQueryClient();
const posts: Post[] | undefined = queryClient.getQueryData('posts');
return (
// component markup
);
};
In the above code, queryClient
is explicitly typed as QueryClient
, ensuring type safety when accessing its methods. The getQueryData
method is typed to return Post[] | undefined
, meaning it could either return an array of Post
objects or undefined
if the data is not yet available.
Conclusion
To recap, this article provided an in-depth exploration of how to utilize TypeScript alongside React Query. It covered various aspects, including setting up a project, typing query and mutation hooks, typing the query function, handling error and loading states, using infinite queries, and implementing optimistic updates.
Practical instances were given to demonstrate the usage of TypeScript in different contexts of React Query, including the usage of the Devtools and useQueryClient
hook.
Using TypeScript with React Query offers many benefits. It promotes more robust, understandable, and maintainable code, reduces the risk of runtime errors, and enhances the developer experience with features like autocompletion and inline documentation.
Moreover, it aligns well with React Query's philosophy of staying as declarative and intuitive as possible. Embrace TypeScript with React Query to bring your data-fetching capabilities to the next level while maintaining a strongly typed and robust codebase.
Subscribe to my newsletter
Read articles from lokosman directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
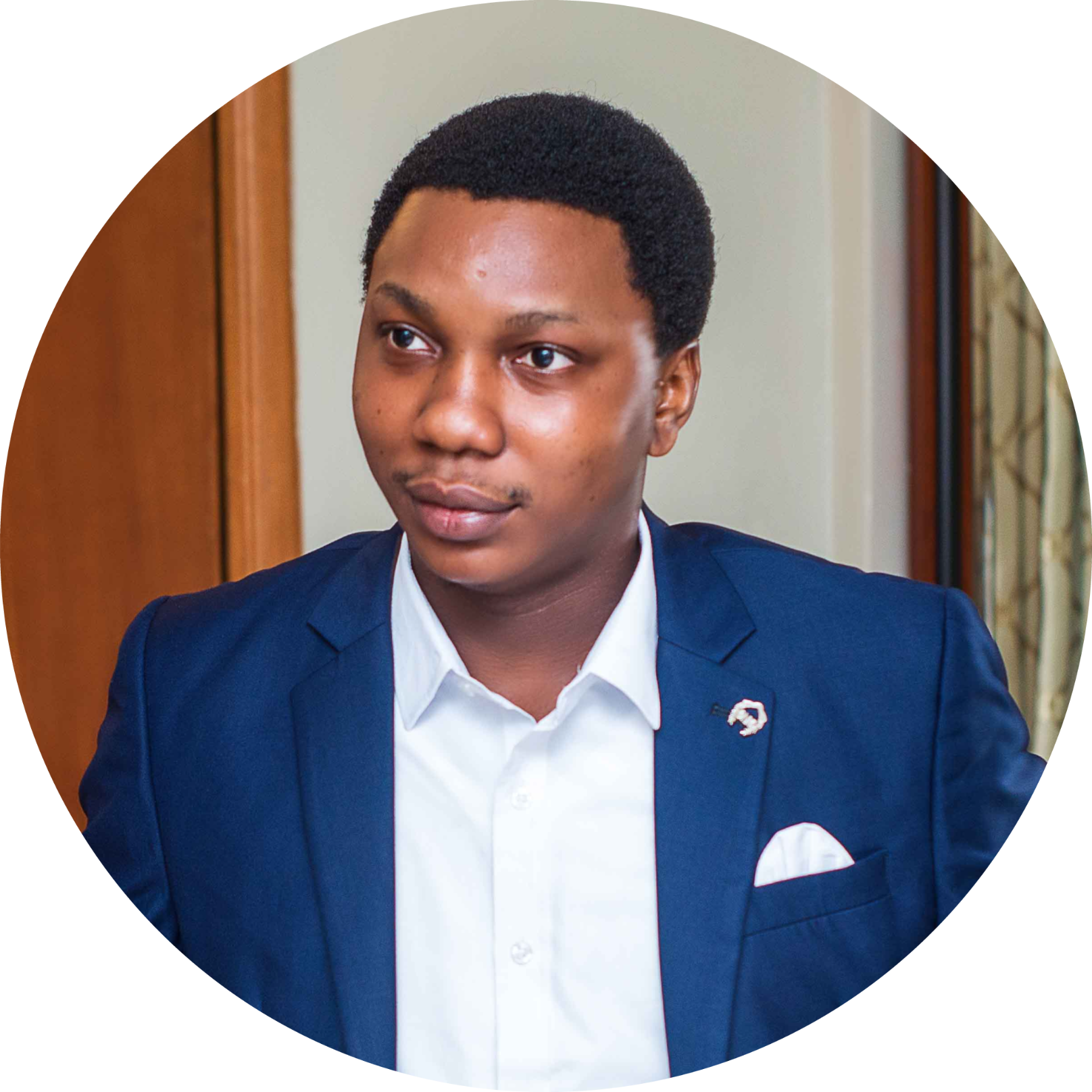
lokosman
lokosman
👋 Greetings, tech lovers! My expertise lies in Java backend engineering, focusing on refining microservices architecture. Web development, for me, is an endless expedition of discovery 🌌. When I share insights through my writings ✍️, it's a beacon of my passion. Each piece is a guiding lighthouse 🗼, hoping to enlighten your path and inspire your tech journey.